Introducing react-router-nav-prompt ๐
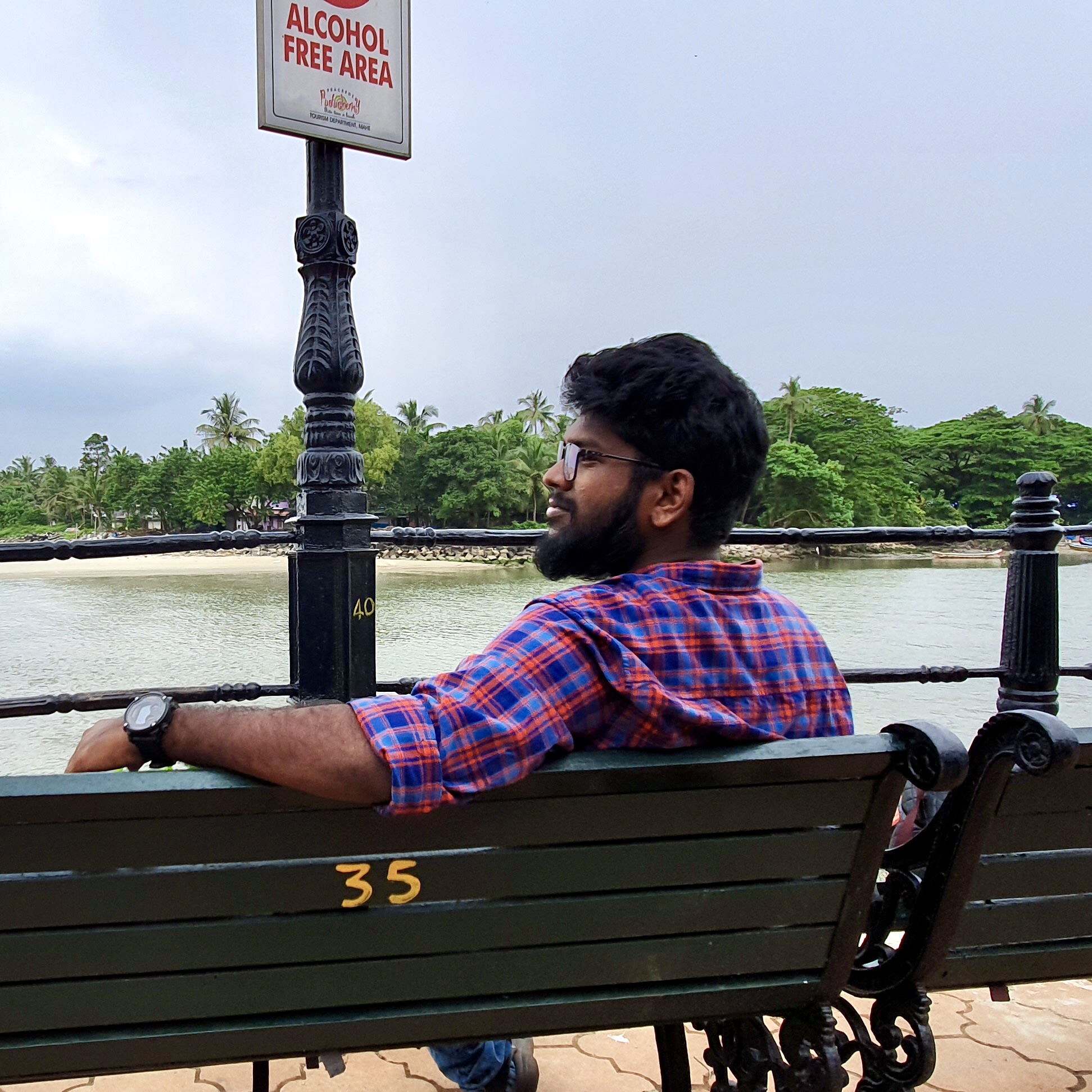
Today, I've decided to make my new npm package react-router-nav-prompt
public!
react-router-nav-prompt
exposes simple APIs based on hooks & render props to confirm user navigation while using React Router. The package is intended to give the flexibility to show any UI when a user tries to leave a route, instead of the un-configurable <Prompt/>
component provided by React Router. It exposes a hook for React v16.8+ functional components and a component for use with both functional & class components.
Usage
useNavPrompt
:
import { useNavPrompt } from 'react-router-nav-prompt';
import Modal from 'your-component-library';
const FormComponent () => {
const [email, setEmail] = useState('');
const {
blocked,
hidePrompt,
navigate,
} = useNavPrompt({
shouldBlock: Boolean(email),
});
if (blocked) {
return (
<Modal>
<div>
Are you sure you want to leave this page?
</div>
<button onClick={hidePrompt}>Stay & fill the form</button>
<button onClick={navigate}>Continue without saving</button>
</Modal>
);
}
return (
<div>
<input
type="text"
onChange={(ev) => setEmail(ev.target.value)}
value={email}
/>
</div>
);
};
NavPrompt
:
import { NavPrompt } from 'react-router-nav-prompt';
import Modal from 'your-component-library';
const FormComponent () => {
const [email, setEmail] = useState('');
const {
blocked,
hidePrompt,
navigate,
} = useNavPrompt({
shouldBlock: Boolean(email),
});
return (
<NavPrompt>
{({
blocked,
hidePrompt,
navigate,
}) => (
blocked
? (
<Modal>
<div>Are you sure you want to leave this page?</div>
<button onClick={hidePrompt}>Stay & fill the form</button>
<button onClick={navigate}>Continue without saving</button>
</Modal>
) : (
<div>
<input
type="text"
onChange={(ev) => setEmail(ev.target.value)}
value={email}
/>
</div>
)
)}
</NavPrompt>
);
};
Feel free to try the package out or see the code at https://github.com/danedavid/react-router-nav-prompt.
It's still at v0.4 as I have currently tested it only with BrowserRouter
& haven't checked inter operability with various APIs like HashRouter
, MemoryRouter
as well as usage with React Native. Feel free to reach out to me or raise an issue!
* mic drop *
Subscribe to my newsletter
Read articles from Dane David directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
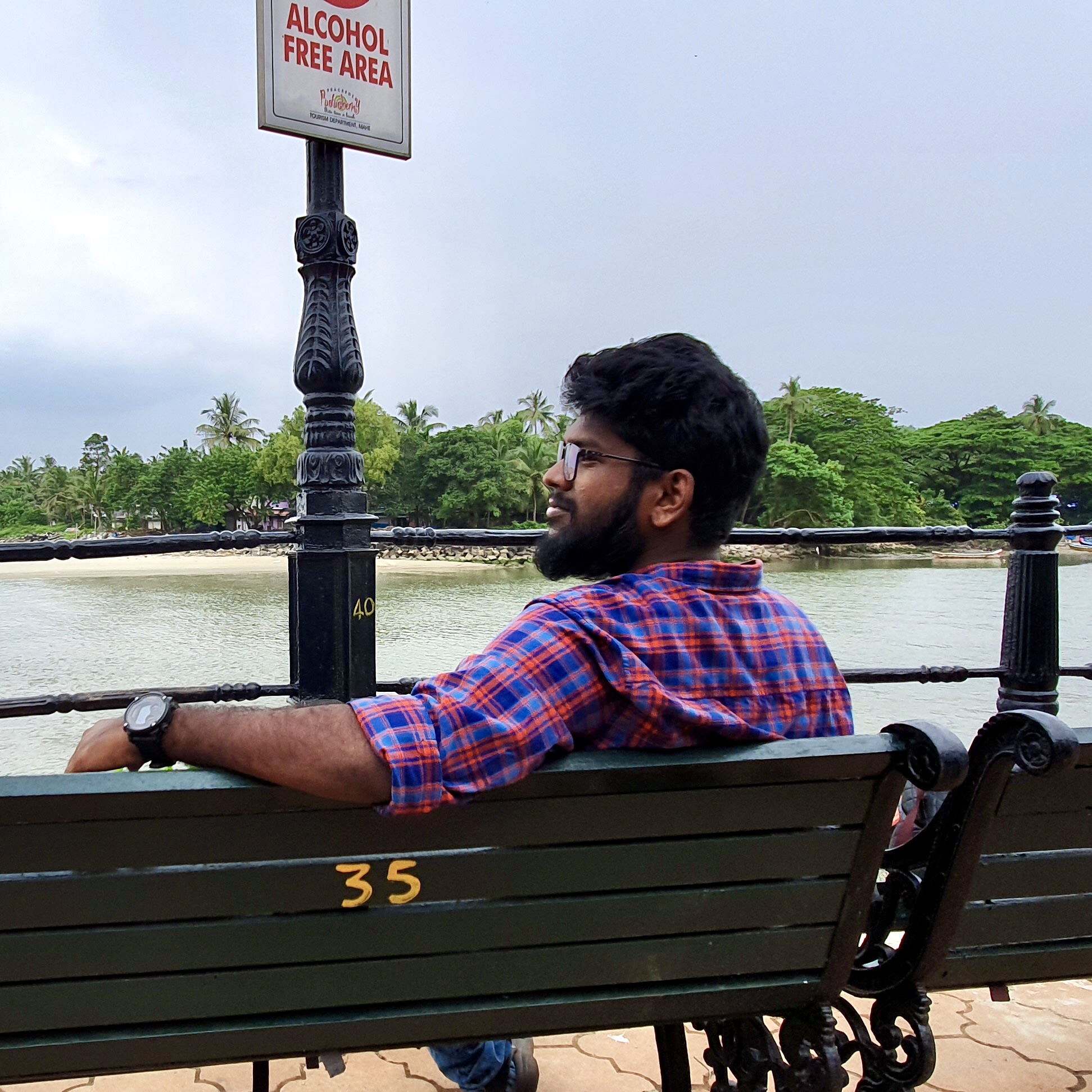
Dane David
Dane David
Full Stack Dev working with React & Node.js