How to install and use Handlebars with express

2 min read
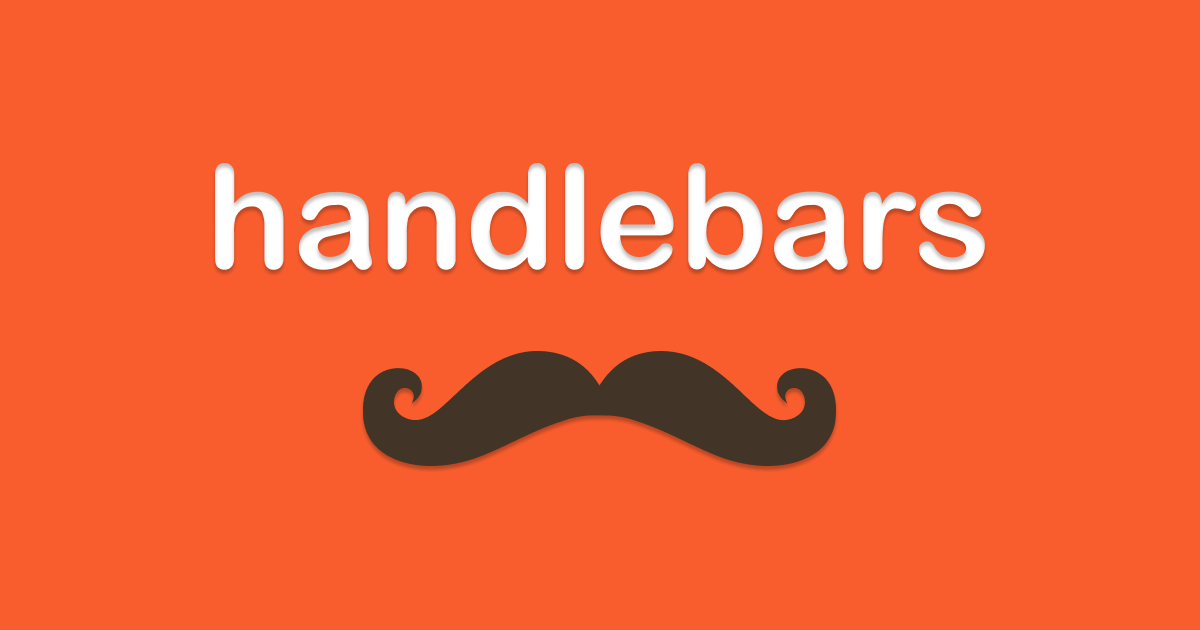
Requirements:
node
express
nodemon
Install
$ yarn add express-handlebars
Files:
src
├── node_modules
└── app.js
In app.js create express server:
const express = require('express');
const app = express();
const handlebars = require('express-handlebars');
app.listen(8080);
Create handlebars in views
folder:
/src
├── /node_modules
├── app.js
└── /views
└── layouts
└── main.hbs
main.hbs
<html>
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Handlebars exemple</title>
</head>
<body>{{{ body }}}</body>
</html>
Configure in app.js
[...]
app.engine('hbs', handlebars({ extName: 'hbs }));
app.set('view engine', 'hbs');
[...]
Create exemple in views
folder:
/src
├── /node_modules
├── app.js
└── /views
├── layouts
│ └── main.hbs
└── exemple.hbs
Create routes:
app.get('/form', function(request, response) {
response.render('exemple');
});
Create exemple.hbs
<h1>Form exemple</h1>
<table>
<tr>
<th>Name</th>
<th>Email</th>
</tr>
{{#each someJson}}
<tr>
<td>{{ name }}</td>
<td>{{ email }}</td>
</tr>
{{/each}}
</table>
Change in app.js
const someJson = require('./some_json.json');
app.get('/form', function(request, response){
response.render('exemple', { someJson: someJson });
});
11
Subscribe to my newsletter
Read articles from Nicolas Oliveira directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nicolas Oliveira
Nicolas Oliveira
It is in the fire of hell of bugs that the legends of programming are forged Currently I study Information Systems at the State University of Minas Gerais and create projects involving several stacks and crazy ideas that I think around.