Master the Arrow Function

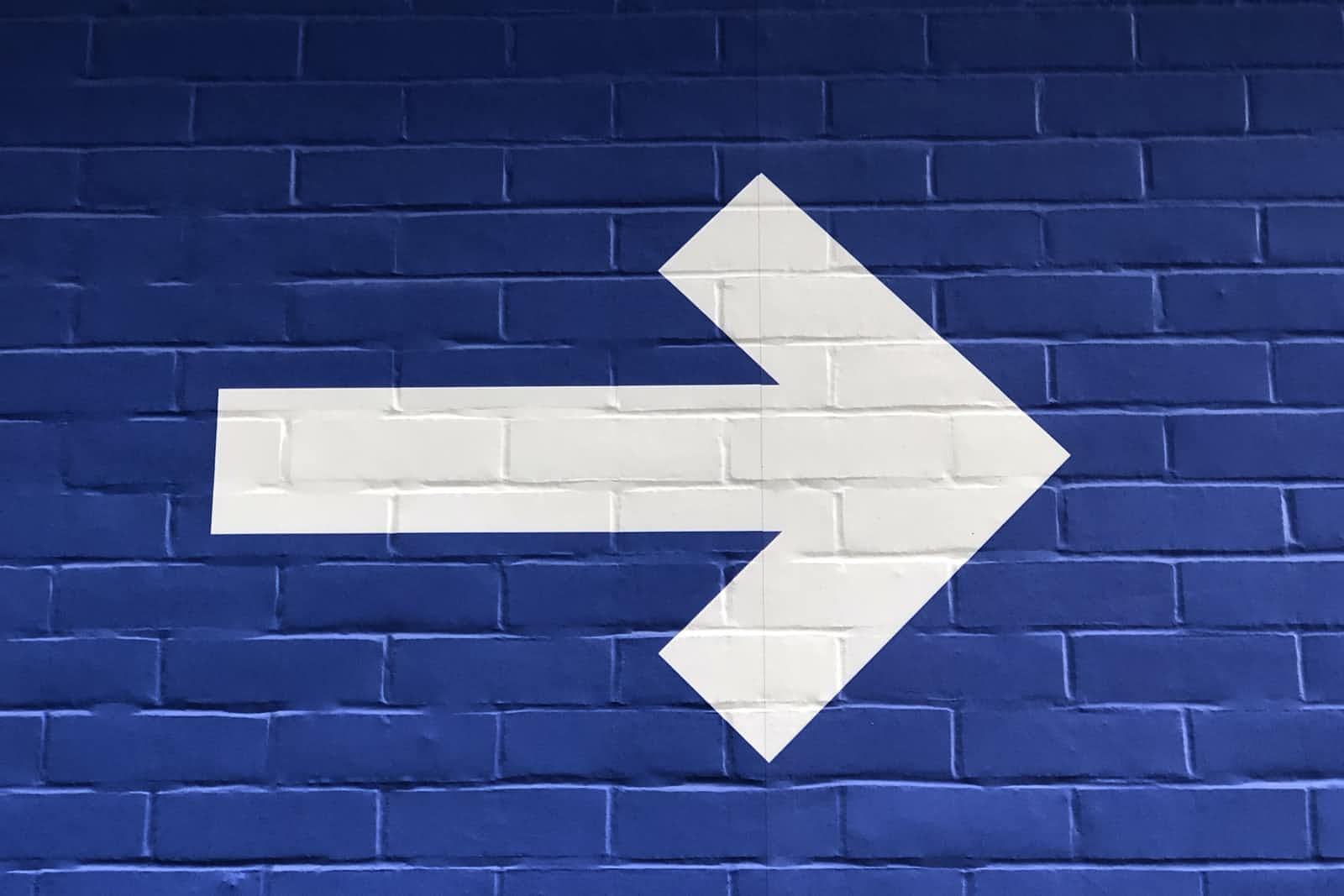
Arrow Function
Arrow functions were introduced in ES6 version of JavaScript. It is a new way to write an anonymous function with some extra benefits and limitations. We cannot use arrow function in all the situations though.
Let’s understand syntax better
There are three conditions, if arrow function has,
- No parameter
- One parameter
- Two or more parameter
No parameter
- We don’t use
function
keyword in the arrow function - We use parentheses if there is no parameter
- No need to use curly brackets and
return
keyword if there is single expression. Everything after the=>
is assumed to be areturn
.
//traditional function
function sum(){
return 2 + 3;
}
console.log(sum()) //5
//arrow function
//ex1
const sum = () => {return 2 + 3};
console.log(sum()) //5
//ex2
const sum = () => 2 + 3;
console.log(sum()) //5
One parameter
- In ex1, you can see that we have used parentheses around the parameter and the output is 2 same as ex2. But, in ex2, we are not using the parentheses. And, that is the magic of arrow function. We can remove the parentheses if there is only one parameter in arrow function and we can remove
return
too because there is only single expression as mentioned above.
//arrow function
//ex 1
const sum = (num) => {
return num + num;
};
console.log(sum(2)); //4
//ex2
const sum = num => num + num;
console.log(sum(2)); //4
Two or more parameters
- We have to use parentheses around the parameters If there is two or more than two parameters
- Now, you must be thinking, why we have used
return
this time. Remember, we have just learned, if there is more than one line of code inside the arrow function then we have to use curly brackets andreturn
keyword.
//arrow function
const sum = (num1, num2) => {
const res = num1 + num2;
return res;
}
console.log(sum(2, 4)); //6
Immediately invoked
JavaScript allows us to declare and invoke the arrow function at the same time, this is called immediately invoked functions.
//syntax
(() => /* code line */ )();
"this" binding
Unlike, traditional function, arrow functions do not have their own binding to this
variable and arguments
. Instead it resolved in lexical scope of that function. Let's understand this by an example:
//traditional function or regular function
const obj = {
name: "javascript",
getName: function () {
console.log(this.name);
function func2() {
console.log(this.name);
}
func2();
},
};
obj.getName();
- first
console.log
will return javascript becausethis
is binding withobj
and not with global scope. - second
console.log
will returnundefined
becausethis
is binding with global scope and not withobj
. And this happened becausefunc2
is independent function here.
This is a bug in javascript and that's why arrow function came as a solution of this problem.
const obj2 = {
name: "javascript",
getName: function () {
console.log(this.name);
func2 = () => {
console.log(this.name);
};
func2();
},
};
obj2.getName();
Here, both the console.log
will return javascript. Because arrow function refers to lexical this
. Means, arrow function inside the regular function will bind with regular function scope. That's a rule.
Note: We cannot write getName
function as an arrow function because according to the rule arrow function will look for the regular function and since arrow function is not inside any regular function it will throw an error. This is the limitation of arrow function.
Constructor
Arrow functions cannot be used as a constructor because arrow function is declare in variable.
constructor: constructor is nothing but a template function which we can be used again and again for the same work with different inputs.
function Person(firstName, lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
const name1 = new Person("Elon", "Musk");
const name2 = new Person("Juhi", "Juhi")
console.log(name1); // {firstName: "Elon", lastName: "Musk"}
console.log(name2); // {firstName: "Juhi", lastName: "Juhi"}
Also, constructor functions and regular functions are hoisted but arrow functions are not. That's another limitation of an arrow function.
hoisting: a function or variable which we can call before their initialisation
Argument object
We cannot access arguments object inside an arrow function but in regular function we can do that. To solve this problem, ES6 provides spread operator.
spread operator: spread an object or array using three dots. example: ...obj
Conclusion
Hopefully this article helped you learn about JavaScript arrow functions. But, to make your understanding strong, write the code by yourself and use debugger to check how your code working behind the scene in arrow function. You can connect with me on LinkedIn and GitHub
Subscribe to my newsletter
Read articles from Savita Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Savita Verma
Savita Verma
Everything you've ever wanted is on the other side of fear." - George Addair This quote sums up the significant pivot I have made in my professional life from the comfort of being in the not technical profession to getting on the roller coaster ride of programming and bugs. Prior to that, I was helping the CEO of NavGurukul in communication with students and partner companies & organisations. Built a few dashboards to help in students' academic, team productivity, and logistics work. Worked in strategy building for the volunteer and CodeStar program of NavGurukul.