Prime Number Checker Using Python
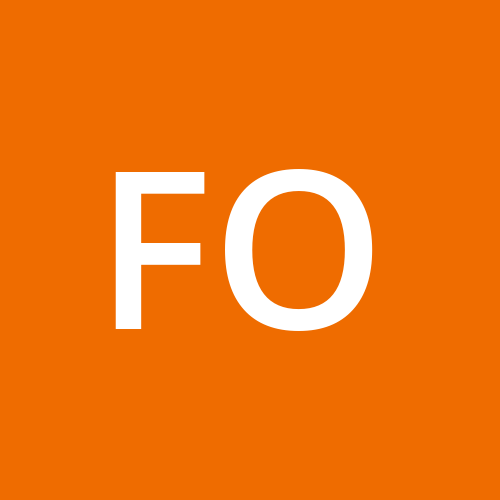

ECX 30 Days of Code and Design
Day 9
Is Prime?
Task
Write a function that takes an integer as input and determines whether it is a prime number or not. A prime number is a positive integer divisible only by one and itself. Nonetheless, 1 is not a prime number.
My Approach
This is the code below. I would explain the code in bits throughout this article.
def prime_number(int_input):
"""Checks if a user's input is either a prime or composite number."""
prime = True
# Negative numbers are not prime numbers
if int_input < 0:
print('Negative numbers are not prime numbers.')
prime = False
# 0 and 1 are not prime numbers
if int_input == 0 or int_input == 1:
print(str(int_input) + ' is a composite (not a prime) number.')
prime = False
# Divide the number inputted by the user from two to the int_input - 1
for i in range(int_input):
# Prevent ZeroDivisionError and division by 1
if i == 0 or i == 1:
continue
# Check if the number is a composite (not a prime) number
if int_input % i == 0:
print(str(int_input) + ' is a composite (not a prime) number.')
prime = False
break
# Print if the number is prime
if prime is True:
print(str(int_input) + ' is a prime number.')
# User's input
try:
print(' Prime Number Checker '.center(30, '*'))
print('Check if a number is a prime or composite number.')
user_input = int(input('Enter number: '))
# Function call
prime_number(user_input)
except ValueError:
print('Invalid input! Input only integers')
First, we create the function, prime_number()
, which is to take an integer parameter. We then create a variable, prime, which we equate to True. If the number the user inputs is a prime number, prime stays True, else it is made False.
def prime_number(int_input):
"""Checks if a user's input is either a prime or composite number."""
prime = True
Next, we check if the user's input is a negative number (negative numbers are not prime numbers), zero, or one.
if int_input < 0:
print('Negative numbers are not prime numbers.')
prime = False
if int_input == 0 or int_input == 1:
print(str(int_input) + ' is a composite (not a prime) number.')
prime = False
Next, we divide the user's input by numbers from 2 to (user input - 1). We won't divide the user's input by 0 or 1. If we get a quotient without a remainder, it is a composite (not a prime) number, thus prime is made False, and the loop breaks.
for i in range(int_input):
if i == 0 or i == 1:
continue
if int_input % i == 0:
print(str(int_input) + ' is a composite (not a prime) number.')
prime = False
break
If the number is not composite, it is a prime number and it is printed out.
if prime is True:
print(str(int_input) + ' is a prime number.')
Finally, we prompt the user to input the number they want to check if it is a prime number or not and we call the prime_number()
function with the user's input as its argument. A try except block is used to catch value error and prevent crash of the program.
try:
print(' Prime Number Checker '.center(30, '*'))
print('Check if a number is a prime or composite number.')
user_input = int(input('Enter number: '))
# Function call
prime_number(user_input)
except ValueError:
print('Invalid input! Input only integers')
Output
Negative Number
**** Prime Number Checker ****
Check if a number is a prime or composite number.
Enter number: -20
Negative numbers are not prime numbers.
Composite Number
**** Prime Number Checker ****
Check if a number is a prime or composite number.
Enter number: 125
125 is a composite (not a prime) number.
Prime Number
**** Prime Number Checker ****
Check if a number is a prime or composite number.
Enter number: 11
11 is a prime number.
Run code in Replit
Subscribe to my newsletter
Read articles from Favour Olumese directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
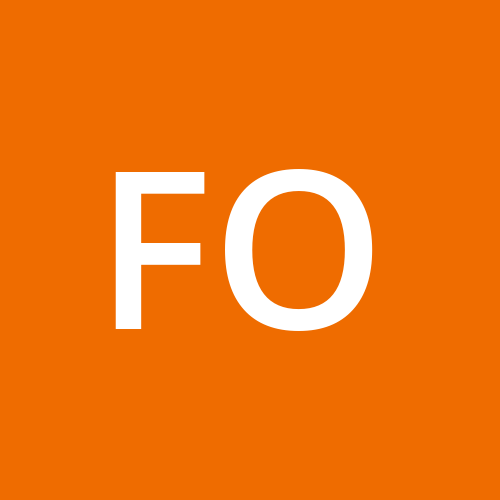
Favour Olumese
Favour Olumese
Hello, I am Favour. I am a Python enthusiast. The Coding Process is a place where I document and consolidate what I learn on my journey towards becoming a programmer. Kindly join me. I am also a poet and non-fiction writer. You could visit https://favourolumese.medium.com to read my writings. Thank you.