Introduction to Diffing and Reconciliation in React
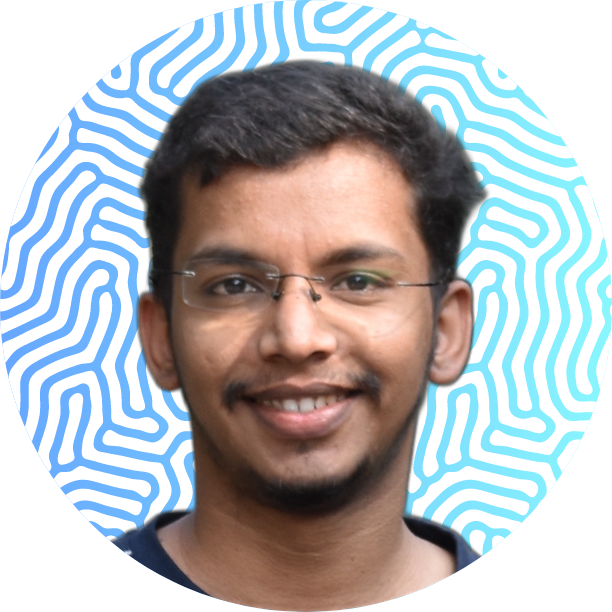
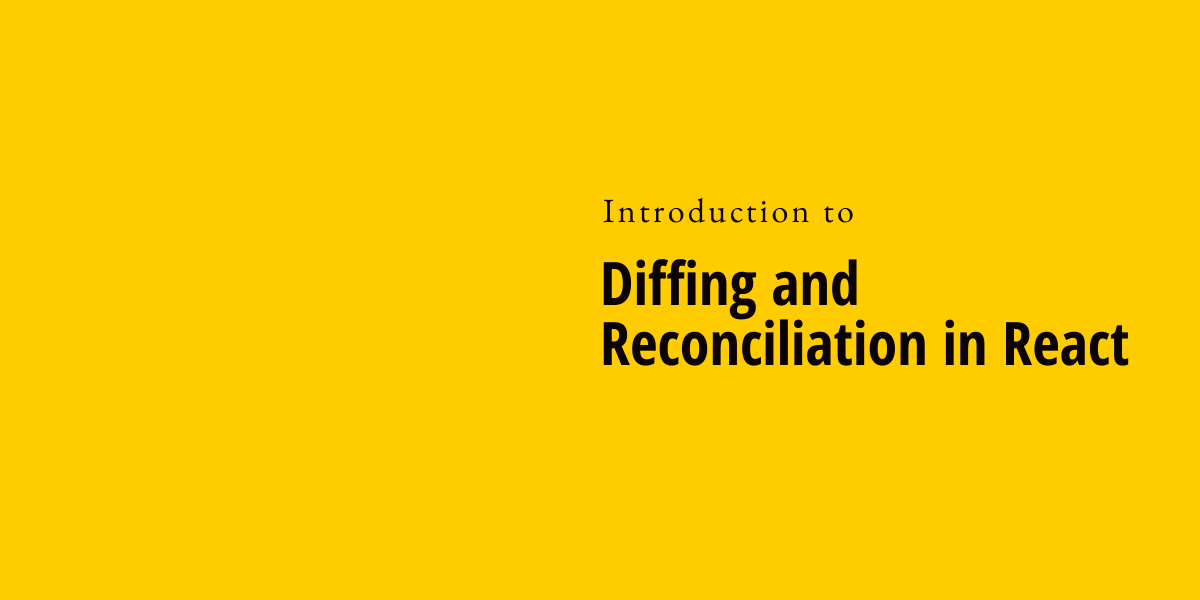
React is fast! And Diffing and Reconciliation surely deserve some credit for it.
Before understanding Diffing and Reconciliation, let's get a brief overview of how React works.
DOM, Virtual DOM and React
Document Object Model (DOM) is a representation of a webpage in terms of nodes and objects. Scripting language like JavaScript uses DOM to access and manipulate a webpage. But since DOM operations are intensive, React uses a something called Virtual DOM, a lightweight copy of real DOM. But Virtual DOM needs to be synced with the Real DOM in order to actually see the changes. This is what Diffing and Reconciliation is all about.
Diffing and Reconciliation
In React, View is a function of State. State changes -> View changes. As discussed above, instead of directly manipulating the DOM, React first updates the Virtual DOM to reflect the changes in View. Let's see how this happens with the help of an example
Virtual DOM before state change
<div>
<p> One </p>
<p> Two </p>
<p> Three </p>
</div>
Virtual DOM after state change
<div>
<p> One </p>
<p> Two </p>
<p> Ten </p>
</div>
Here, React identifies that only last <p>
element has changed and updates only the required node. This update in the Virtual DOM is done according to diffing algorithm like this -
React checks for the type of the changed element,
- if type is different, it creates a new tree for that particular element
- if type is same, it looks for change in attributes and updates only required changes instead of creating new tree for the element
As the Virtual DOM is updated, React compares Virtual DOM before and after the state update and decides which nodes need to be updated.
Once React identifies all and only changes to be done, it updates the Real DOM with least number of operations. This process of syncing the Virtual DOM and Real DOM is called Reconciliation.
Bonus: Why we must use unique keys in the elements?
Consider a different updated state for the example above,
Virtual DOM after state change
<div>
<p> Ten </p>
<p> One </p>
<p> Two </p>
<p> Three </p>
</div>
Let's dry run the diffing algorithm in this case,
Here, React will get inside div
and will encounter a new first element. Now, as the first element itself has changed, React will assume all div
child elements to have changed and it'll go on to rebuild the whole div
. But this is not an optimized solution and we need a way to identify only changed elements. This is where unique keys come into picture. If elements have unique keys, React compares the keys from two Virtual DOMs (before and after state change) and updates required nodes only.
Summary
- React minimizes DOM manipulations with the help of Virtual DOM
- Diffing is a process by which Virtual DOM is updated with state change
- Reconciliation is the optimized way of syncing Virtual DOM with Real DOM
- Having unique key for elements helps React identify the changed elements and avoid unnecessary DOM manipulations
Subscribe to my newsletter
Read articles from Tanay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
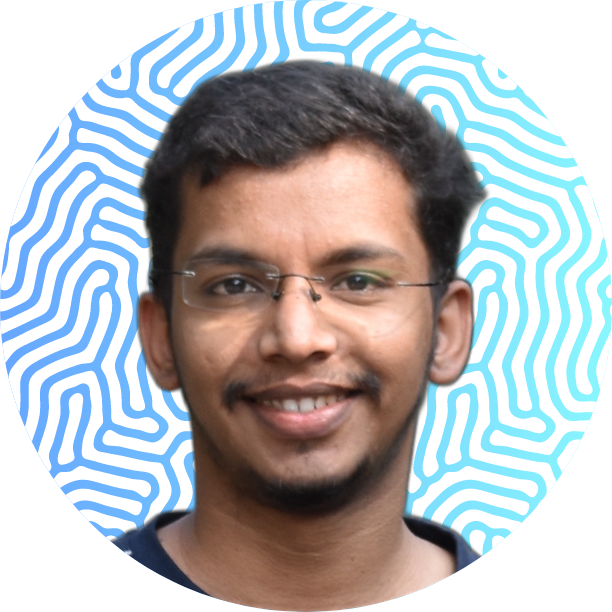