Python Practice, and trying not to feel old. Today I Learned: Wednesday 15 June 2022
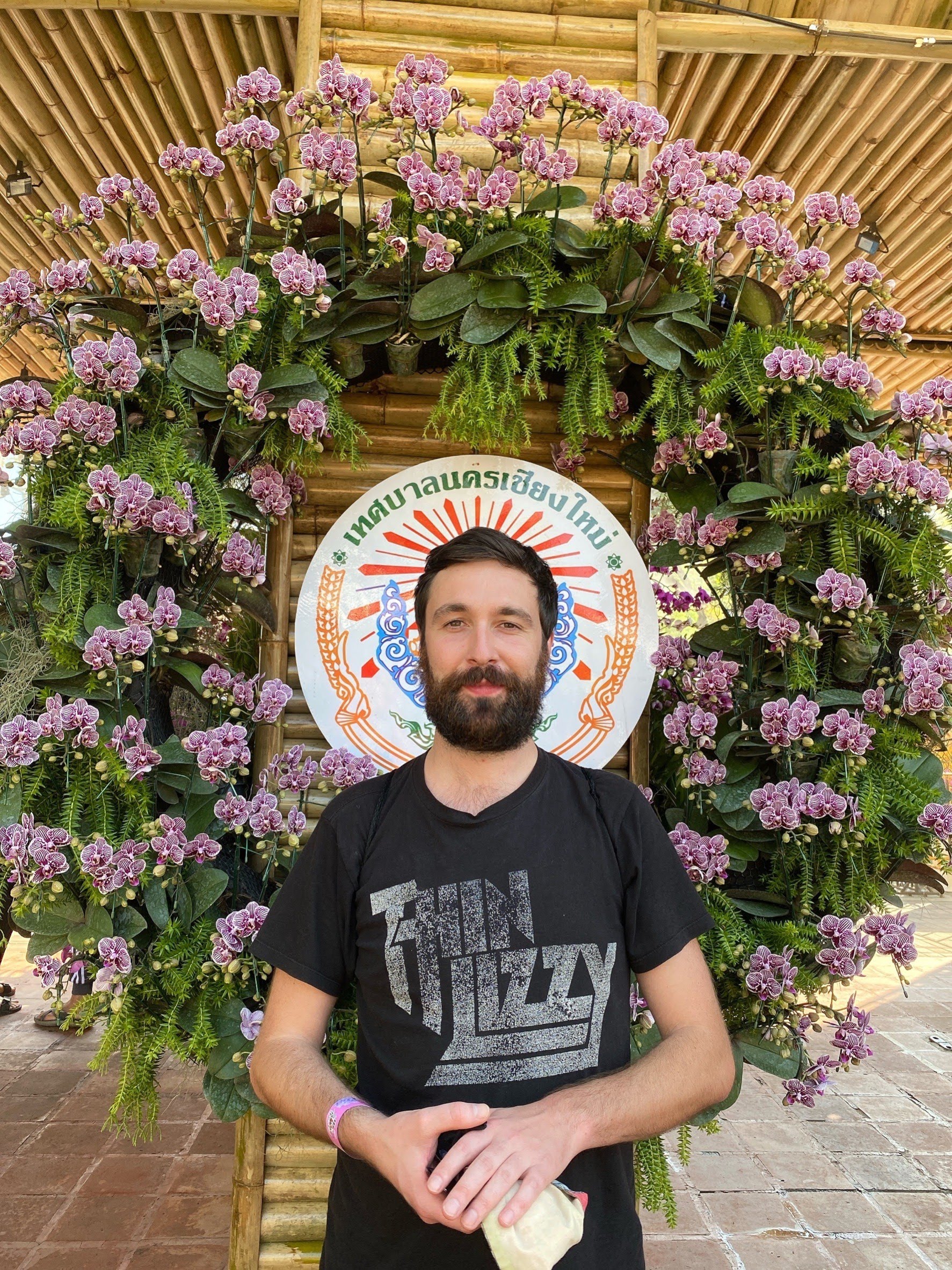
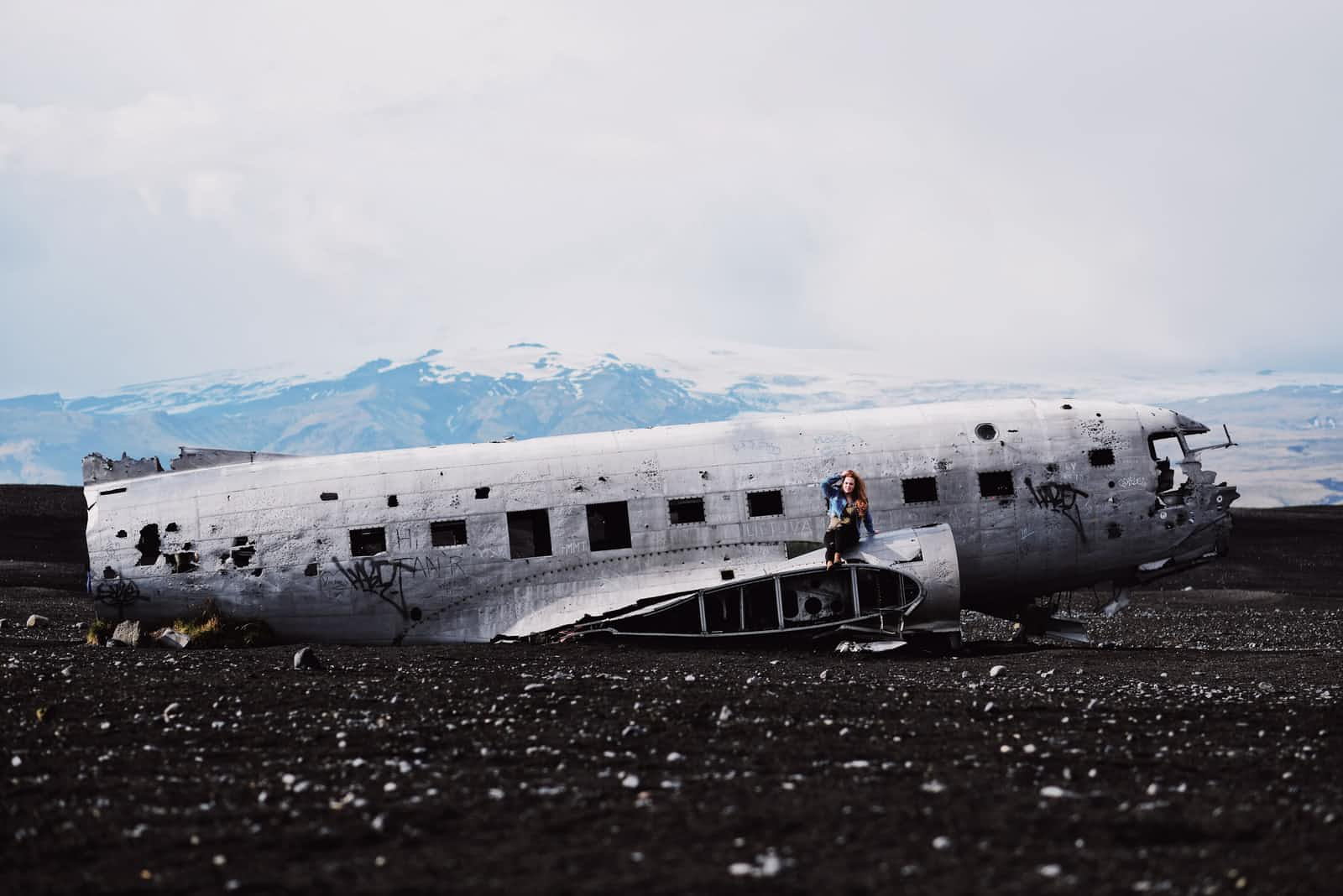
Still working through Codecademy's Python 2 course.
while loops are similar to if statements.
They will continue to loop while the condition is true.
while/else loops will execute the else block once the loop condition evaluates to false.
A trailing comma (,) after a print statement in a loop seems to force text to be printed out horizontally. If the trailing comma is left out, it seems to print on anew line for every character.
The 'zip()' function is used to iterate over two lists at once.
Here is it used in a for loop:
- for a, b in zip(list_a, list_b):
When working with booleans, it is important to capitalize the first letters from 'True' and 'False'. Python will not recognise 'true' and 'false'.
'list' is a reserved name, you cannot name a variable 'list'.
I got stuck for an hour on their factorial calculation practice:
https://www.codecademy.com/courses/learn-python/lessons/practice-makes-perfect/exercises/factorial
I was trying to use str(), map() and list(), combined with for and while loops. No joy.
Eventually I tried using range() and it worked out.
def factorial(x):
factorial = x
for n in range(1, x):
factorial = factorial * n
print factorial
return factorial
factorial(5)
It will take me some time to wrap my head around lists starting the index at 0.
The next practice was for testing if a number is prime:
https://www.codecademy.com/courses/learn-python/lessons/practice-makes-perfect/exercises/isprime
Pretty frustrating. Turns out by errors were down to improper indentation.
- Indentation is important in Python, I'll be sure to keep and eye on it now.
Codecademy's in browser compiler is frustratingly slow.
Python 'if' conditions must repeat the ternary operator everytime when checking string comparisons.
eg. if x == "apple" or "orange":
This wont work.
I will need to put:
- if x == "apple" or x == "orange"
When using the .find() function, it will return -1 if the word not found.
sentence = "What is the story?"
sentence.find("word")
- This will return -1, since the string "word" is not found within the string name 'sentence'.
Currently watching this video and trying not to feel like I wasted my youth with videogames:
I learned to spin up a server with flask, you need to enter 'run flask' into the terminal.
- I was confused about that when doing the flask RESTful tutorial from a few days ago. I'll take a look at that tutorial again now.
'Jinja' is some sort of tool to render images in HTML.
- Supposedly when you use Jinja, you need to use double curly braces {{}}
Startbootstrap.com is another site with free bootstrap templates.
I just heard 'dist' mentioned again. I still don't know what that is.
Again, I need to reiterate how frustrating codecademy's IDE is. Often times when I run some code, I receive the error: "Oops, something went wrong. Try again"
If it was some error with compiling on their end, thats fine. However I would like it if they specified that, as opposed to me guessing if there's something wrong with the syntax of my code.
Usually, to fix this problem, I need to copy the code, reset the workspace, and paste the code in, and run the code.
This is frustrating when trying to debug.
Thanks for reading about my day, Niall Harrington
Subscribe to my newsletter
Read articles from Niall Harrington directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
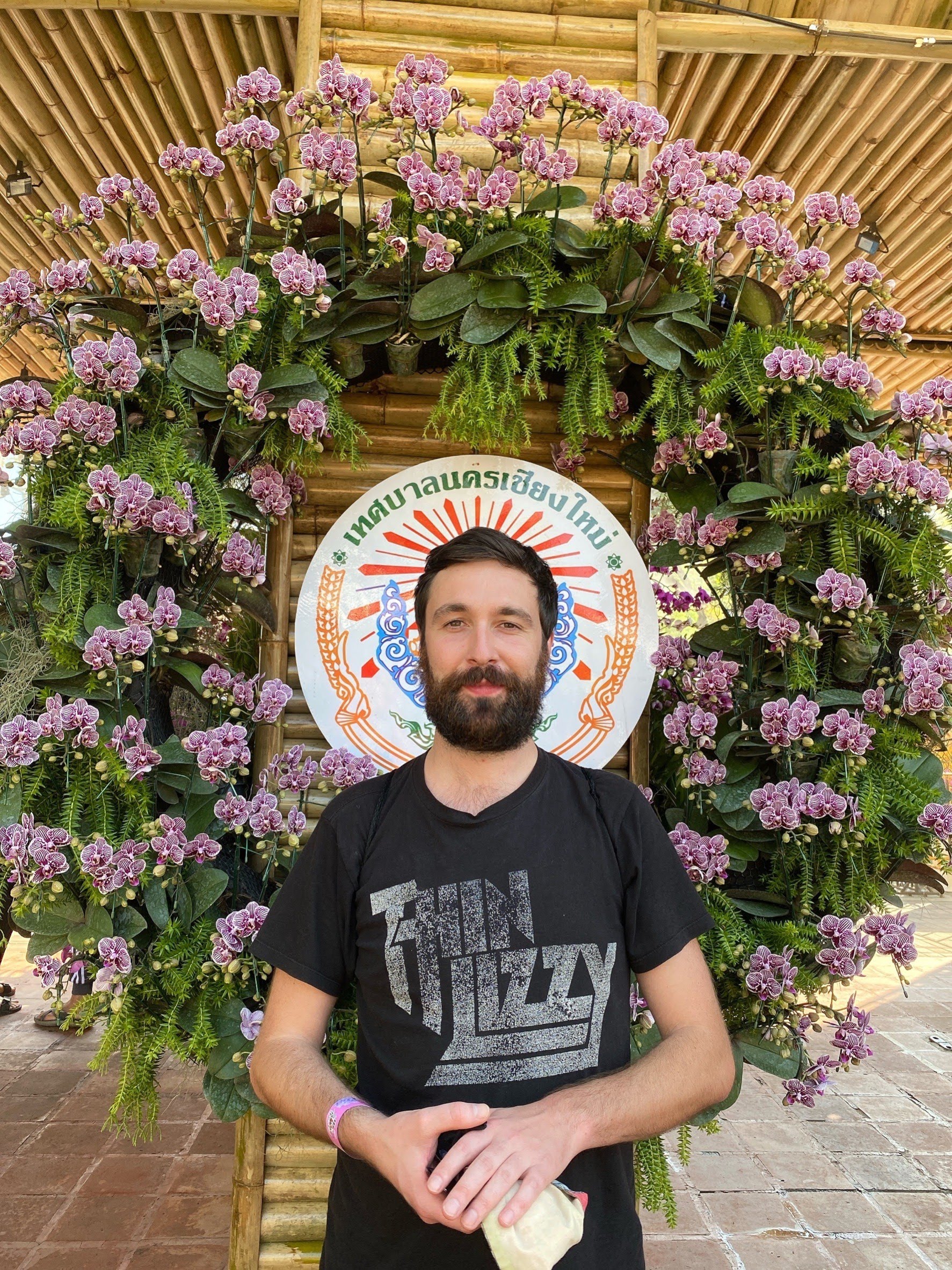
Niall Harrington
Niall Harrington
Hello everyone, I'm a recent graduate of Blockchain & Backend Development at York University, Toronto. I started this blog to document my career change journey from bartender to a developer. Since starting this blog in 2022, I have been hired as a research assistant and front-end developer at York's Digital Currencies Project. We are currently working on developing a way to estimate CO2 emissions associated with bitcoin mining. Thanks for joining me.