Leetcode 75: Day 3: 21. Merge Two Sorted Lists
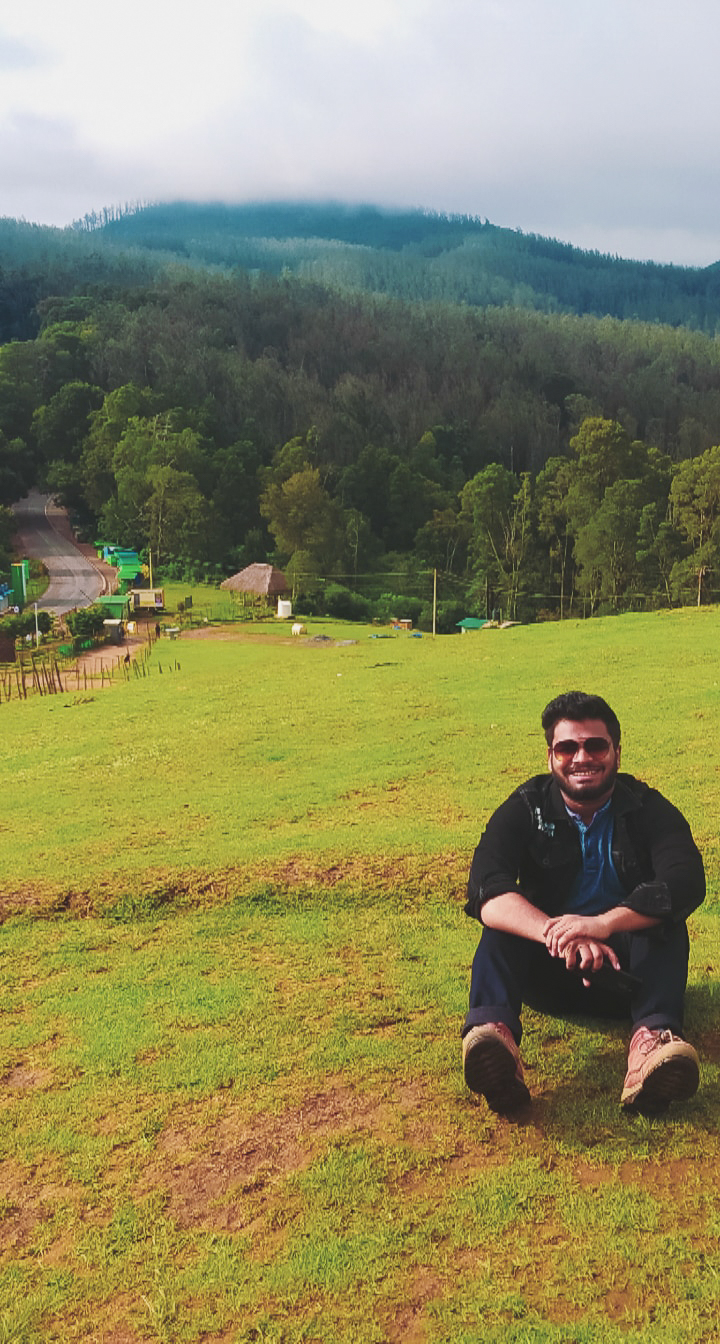
Question:
You are given the heads of two sorted linked lists list1 and list2.
Merge the two lists in a one sorted list. The list should be made by splicing together the nodes of the first two lists.
Return the head of the merged linked list.
Example 1:
Input: list1 = [1,2,4], list2 = [1,3,4] Output: [1,1,2,3,4,4]
Example 2:
Input: list1 = [], list2 = [] Output: []
Example 3:
Input: list1 = [], list2 = [0] Output: [0]
Constraints:
The number of nodes in both lists is in the range [0, 50]. -100 <= Node.val <= 100 Both list1 and list2 are sorted in non-decreasing order.
Solution:
- Use recursion to solve the subproblems.
- Compare the first nodes of both the lists.
- Once the smaller node is found, pass it to the recursive function (with new list starting from the second node of that list: list.next node).
Code:
class Solution {
public ListNode mergeTwoLists(ListNode l1, ListNode l2) {
if(l1 == null) return l2;
if(l2 == null) return l1;
if(l1.val < l2.val){
l1.next = mergeTwoLists(l1.next, l2);
return l1;
} else{
l2.next = mergeTwoLists(l1, l2.next);
return l2;
}
}
}
Complexity:
- Time: O(m + n)
- Space: O(1)
Subscribe to my newsletter
Read articles from Rahul Saxena directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
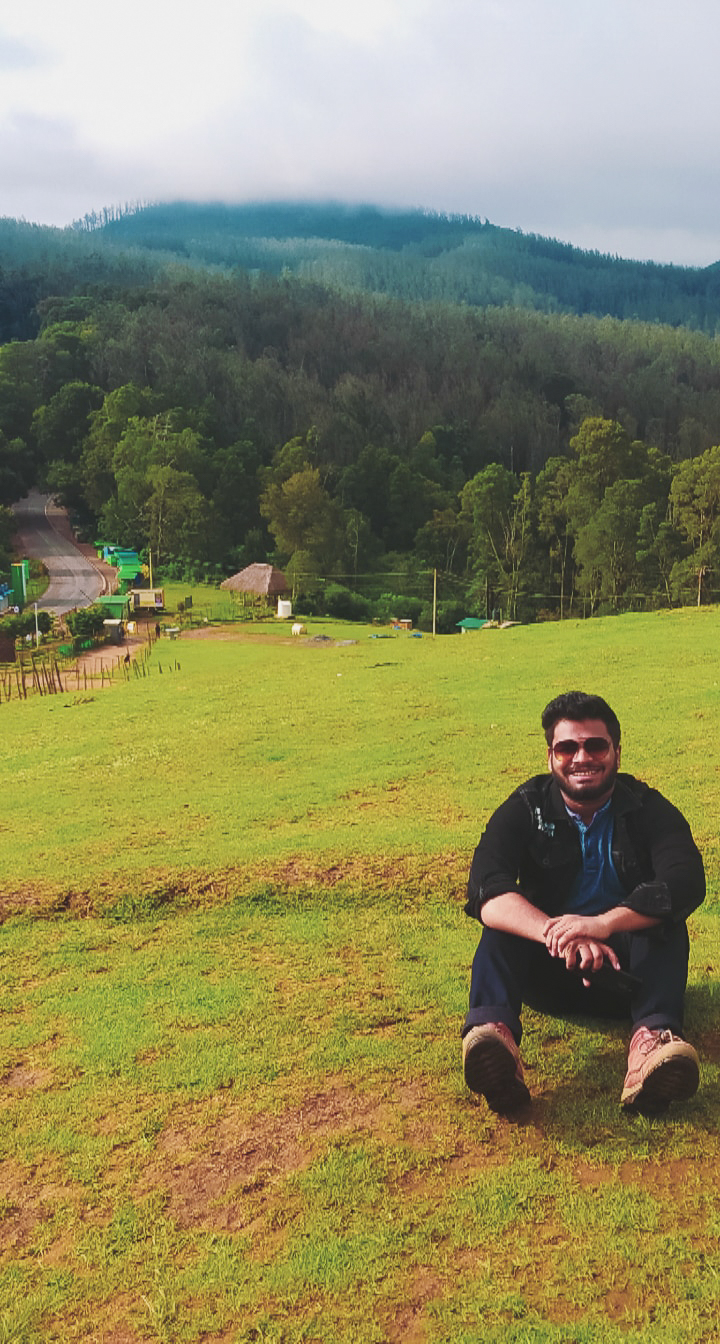
Rahul Saxena
Rahul Saxena
I’m a Master’s student in Computer Science at the University of Massachusetts Amherst, specializing in Computer Vision and Natural Language Processing. I focus on enhancing image captioning, visual question answering (VQA), and text-to-image generation using deep learning. I’m seeking Fall 2024 internships, co-op opportunities, and full-time roles starting in 2025. With over four years of software development experience in healthcare and fintech, including roles at PayPal and Philips, I excel in C++, Java, Python, and JavaScript, with a preference for Python and C++. Outside of work, I’m passionate about photography and trail running, having participated in several marathons and ultra events. I’m open to connecting with professionals and recruiters who value collaboration. Reach out to me at rahulsaxena@umass.edu or connect on LinkedIn.