Finishing one course and starting another. The road to a PCEP certificate. Today I Learned: Saturday 18 June 2022
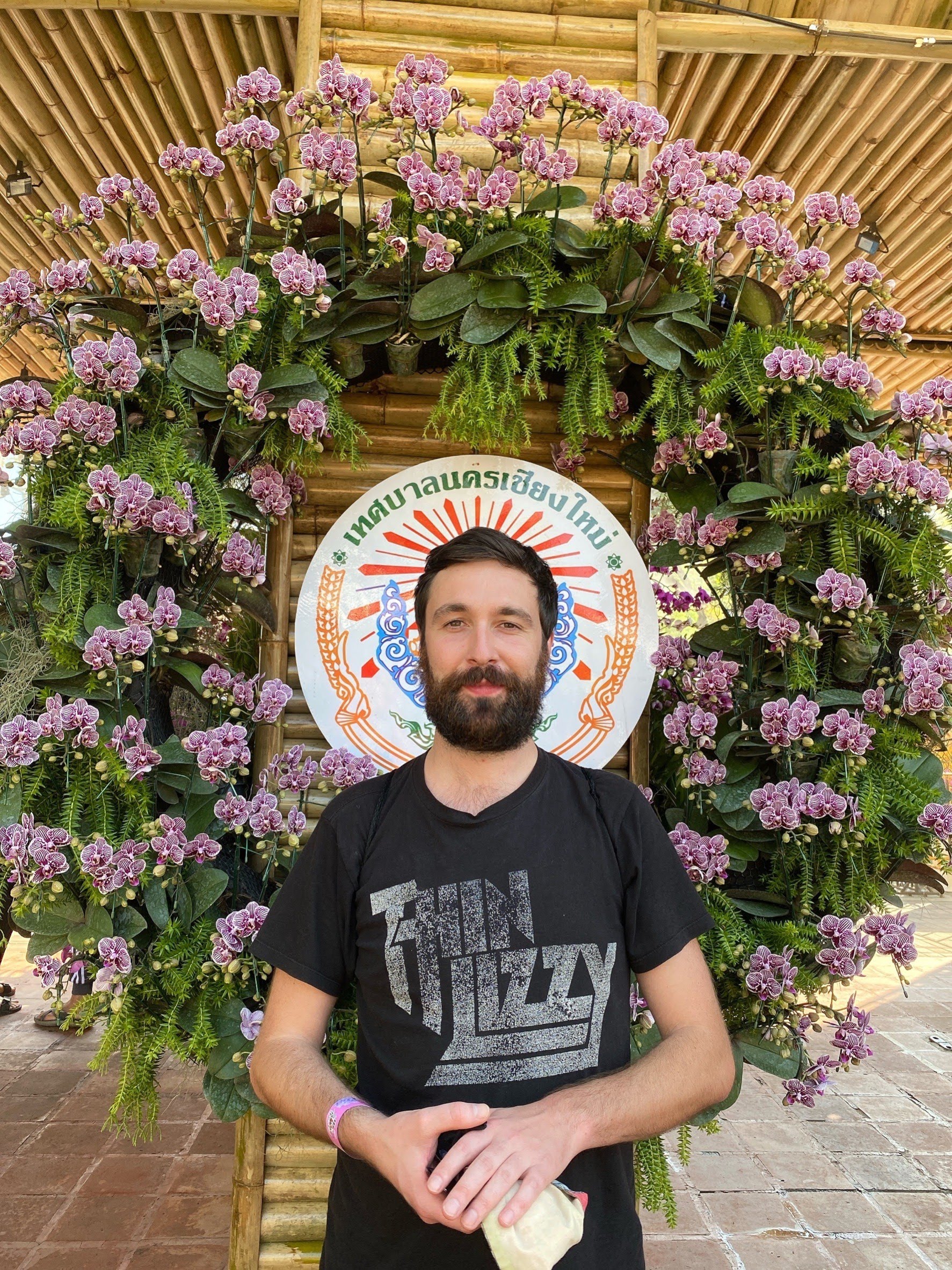
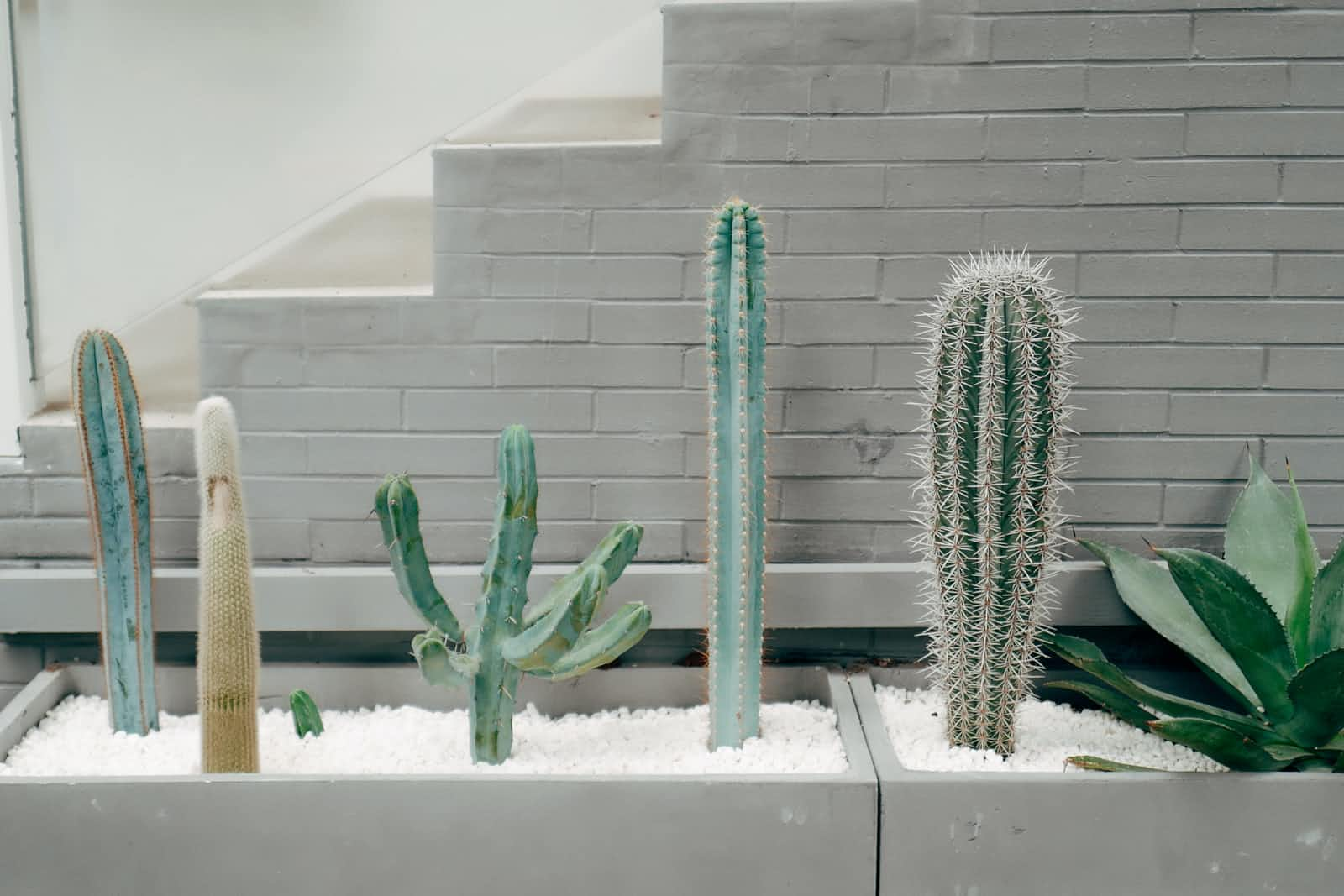
I'm on the last hurdle of codecademy's Python 2 course. Now I'm learning about classes.
The 'pass' keyword is used in the body of a class. It's not used for anything, just used to prevent an error from happening because the body is empty.
- The 'self' variable refers to the object being created. It turns out that this 'self' word is just a Python convention, there is nothing special about it.
It is overwhelmingly common to use 'self' as the first parameter in init().
- I think init() is used to initialize a class. I could be wrong. The guide says that the first parameter entered into the init() function is used to refer to the object being created. Hence why they use the word 'self'. Again, any word can be used, but it is convention to use the word 'self'.
The init() function exists by default, so you dont need to specify it in the class if you want to create an instance of the class.
Instead, if you write init() into the class, you are overwriting the default version.
- There are three different types of variables when it comes to accessibility:
- global variables
- available everywhere
- member variables
- available to members of a certain class.
- instance variables
- only available to particular instances of a class.
- The same principle applies to functions.
- When creating a new class, you can make it inherit the properties of an older class by passing the older class into it:
- **class new_class(old_class)**
- The '*sum()*' function is only for adding iterable variables such as: lists, dictionaries and tuples.
- It cannot be used like this '**sum(number1, number2, number3**)'
- When creating a new class, I should always pass in the argument '*object*' into the parenthesis. This means that the class will inherit all the properties of an object, which is the simplest, most basic class.
- When creating an instance of a class, you don't need to enter '*self*' as the first argument. This will be added automatically. Eg:
- **my_car = Car("Delorean", "silver", 88)**
- There is another method in python classes called **__repr__()**. It is used to represent something. I don't know exactly how it works yet.
- There is a python function called **open()**, which is used to open a file and write to it, as opposed to printing to the console. It takes 2 arguments, the first is the name of the file, and the second is the mode it is in. For example:
- **f = open("output.txt", "w")**
- The above command opens a file named output.txt in 'w' mode. "w" stands for 'write'.
- Another mode to use is 'r+', which allows you to read AND write it.
- 'a' stands for append mode
- 'r' stands for read-only mode
- 'w' stands for write-only mode
- The **f.read()** function will print the contents of the file to the console.
- **f.write('text here')** function allows you to write to the file stored under variable 'f'.
- **f.close()** is used to close the file when you are done. The guide says it is important to do this every time.
- Guide says if you write to a file without closing it, the data won't make it to the target file.
- I'm not sure if this issue will affect **read()** functions?
- Codecademy course finished!
Now I'm looking into Python certification.
The Python Institute has a certificate called PCEP. It's an entry-level certification. Toronto Public Library offers free training here:\ https://www.netacad.com/portal/web/self-enroll/m/course-1220668
It's 75 hours to train for both the entry-level PCEP and associate-level PCAP.
Ok, I've started the training for the certificate.
There is a difference between code compilation and code interpretation.
Python is an interpreted language.
It was created by one man from Haarlem in the Netherlands, named Guido van Rossum. The language is named after Monty Python's Flying Circus.
The two direct competitors of Python are Perl and Ruby.
Python 2 and Python 3 are not compatible with each other.
Although 3 is a better version of 2, 2 has not been discontinued yet because there are too many applications already built with it so it wouldn't make sense to discard it.
The previous course I did with Codecademy was in Python 2. This current course is Python 3.
Each Python language is written in C. Which makes it easy to port to other platforms that can compile and run C.
Cython is an alternative to Python that compiles the code in C, to make it run faster.
Jython is Python written in Java. Python 2 is only supported, not Python 3.
PyPy is Python written in a Python-like language named RPython (Restricted Python). It is used for the development of Python, and Python 3 is supported, unlike Jython.
Python3 includes IDLE: Integrated Development and Learning Environment.
Python requires that there cannot be more than one instruction per line.
\ is the escape character. When used inside strings, '\n' will skip to a new line.
The print() function can also accept keyword arguments. They have to be put after the last positional argument.
The 'end =' keyword is one of these. It looks like it makes the next print function output to the same, current line.
- Actually, I think it just appends a specific string to the end of the variable.
The 'sep =' keyword replaces the separation space between string with the inputted string. Eg.
- print("My", "name", "is", "Monty", "Python.", sep="-")
This will print:
- My-name-is-Monty-Python.
By default, is it a space instead ' '.
Python 3.8 has 69 built-in functions.
A literal is data whose values are determined by the literal itself.
- 123 is a literal, 'c' is not.
Since Python 3.6, you can use underscores _ in integers to make them more readable:
So 11111111 is the same as 11_111_111.
Also, you don't need to put a + before a number to show that it is positive. But it is possible.
Python can also hand octal and hexadecimal numbers.
0o123 is an example of an octal number equal to 83. I don't know the purpose of octal numbers yet.
0x123 is an example of a hexadecimal number equal to 291. I also don't know what the purpose of a hexadecimal number is, but I have seen it used for crypto-wallets.
A 0 in a float can be omitted if it is the only digit in front of or after the floating-point. Eg.
0.4 is the same as .4.
5.0 is the same as 5.
You can also use 'e' or 'E' for an exponent. An exponent means 'times ten to the power of'. Eg.
'3E8' means 3 x 108
Think of it like '3 with 8 zeroes after it.'
Note:
the exponent (the value after the E) has to be an integer;
the base (the value in front of the E) may be an integer.
If you want quotation marks "" to appear in a string, you have two options:
use the escape character before the ". Eg.
- string = "She said /"Yes!/""
Use an apostrophe instead of quotation marks to make the string. Eg.
- string = 'She said "Yes!"'
Number bases:
Binary numbers have a base of 2. It is only made up of 0 and 1s. The decimal number 10 is equivalent to 1010 in binary.
Octal numbers have a base of 8. Decimal 10 is equivalent to octal 0o12.
Hexadecimal numbers have a base of 16. Decimal 10 is equivalent to 0xA.
When using numerical operators, if one argument is a float, then the result will be a float. Eg.
3 * 3 = 9
3 * 3.0 = 9.0
3.0 * 3.0 = 9.0
Using the division arithmetic / will always result in a float, even if two integers are used as arguments. Eg.
- 6 / 2 = 3.0
To get around this, you can use the integer divisional operator //.
- 6 // 2 = 3
Also, if you use an integer divisional // on a number with a floating-point. It will always result in a float with the number rounded down to .0.
6 // 3.5 = 1
Note that the rounding will always go to the lesser integer, even if it is for example 4.8
Integer division is also known as floor division.
Multiplications precede additions. Eg
2 + 3 * 5
In the above line, the multiplication will be performed first. The final result is 17.
Operators with equal priority use left-side binding. So they are performed from the left to the right. Eg.
9 6 2
The above line equates to (96)2
Exponentiation operators use right-side binding however. Eg
print(2 2 3)
results in 2 (2 3)
256
There are two different types of + and -, unary and binary.
binary operators are used for addition and subtraction. Eg.
5 - 3
It requires two arguments, one on each side. On the left, it's called the minuend, and on the right, it's called the subtrahend.
unary operators change the sign on a number. - put in front of 15 makes it -15, a negative number.
The priority of operators goes like this:
1: **
2: +, - (In unary format)
3: *, /, //, %
4: +, - (In binary format)
A program that doesn't read a user's input is referred to as a deaf program.
The input() function is used to receive user's input from the console.
When printing varibles, you only use + when you are trying to print strings only. Think of the + like glue. If there are any other data types in the message, use a ',' instead.
When you try to multiply a string, it just replicates the string by the number.
- "James" * 3 = "JamesJamesJames"
Thanks for reading this far! Niall
Subscribe to my newsletter
Read articles from Niall Harrington directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
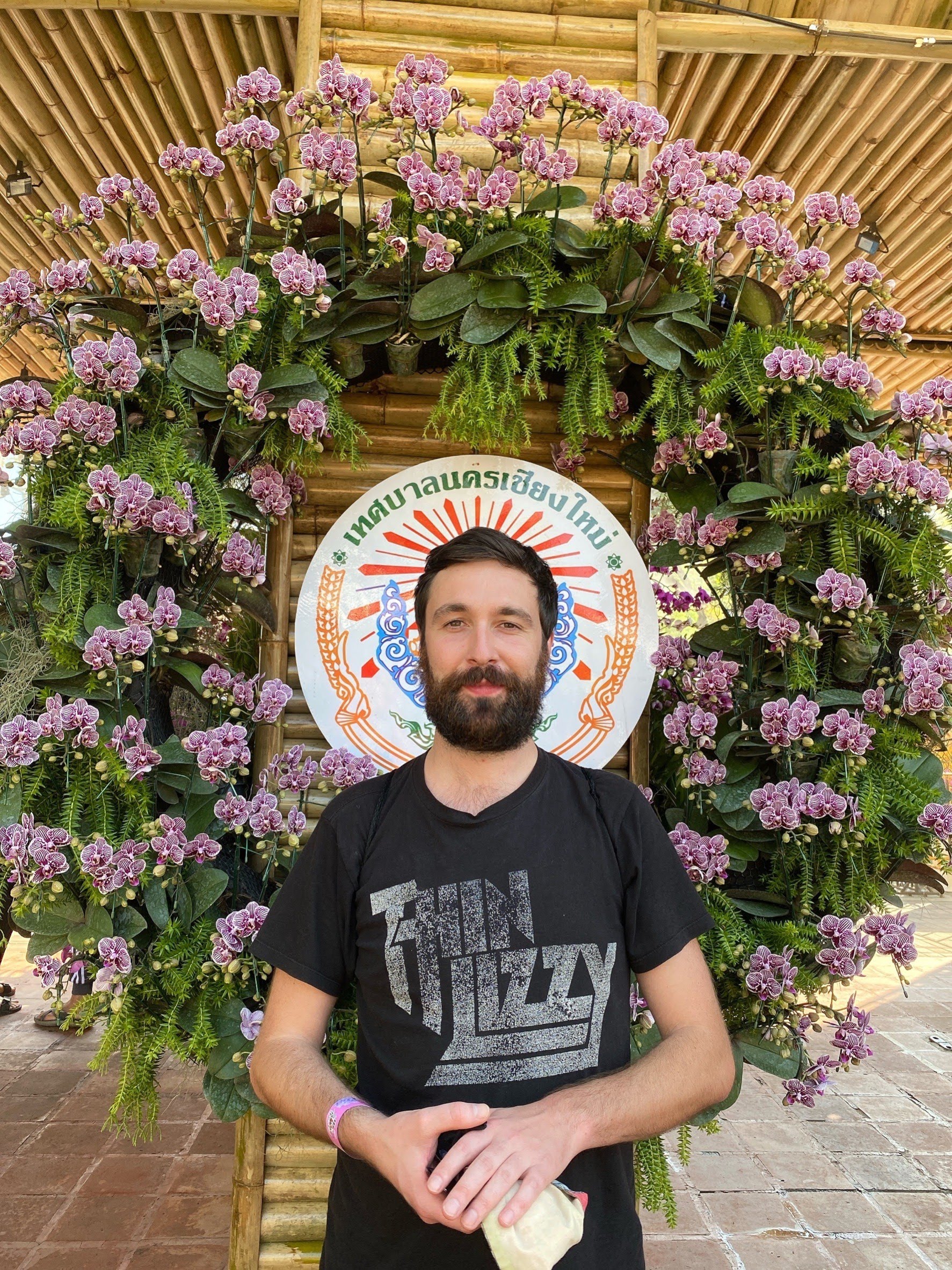
Niall Harrington
Niall Harrington
Hello everyone, I'm a recent graduate of Blockchain & Backend Development at York University, Toronto. I started this blog to document my career change journey from bartender to a developer. Since starting this blog in 2022, I have been hired as a research assistant and front-end developer at York's Digital Currencies Project. We are currently working on developing a way to estimate CO2 emissions associated with bitcoin mining. Thanks for joining me.