TypeScript program to get all separate digits of a number
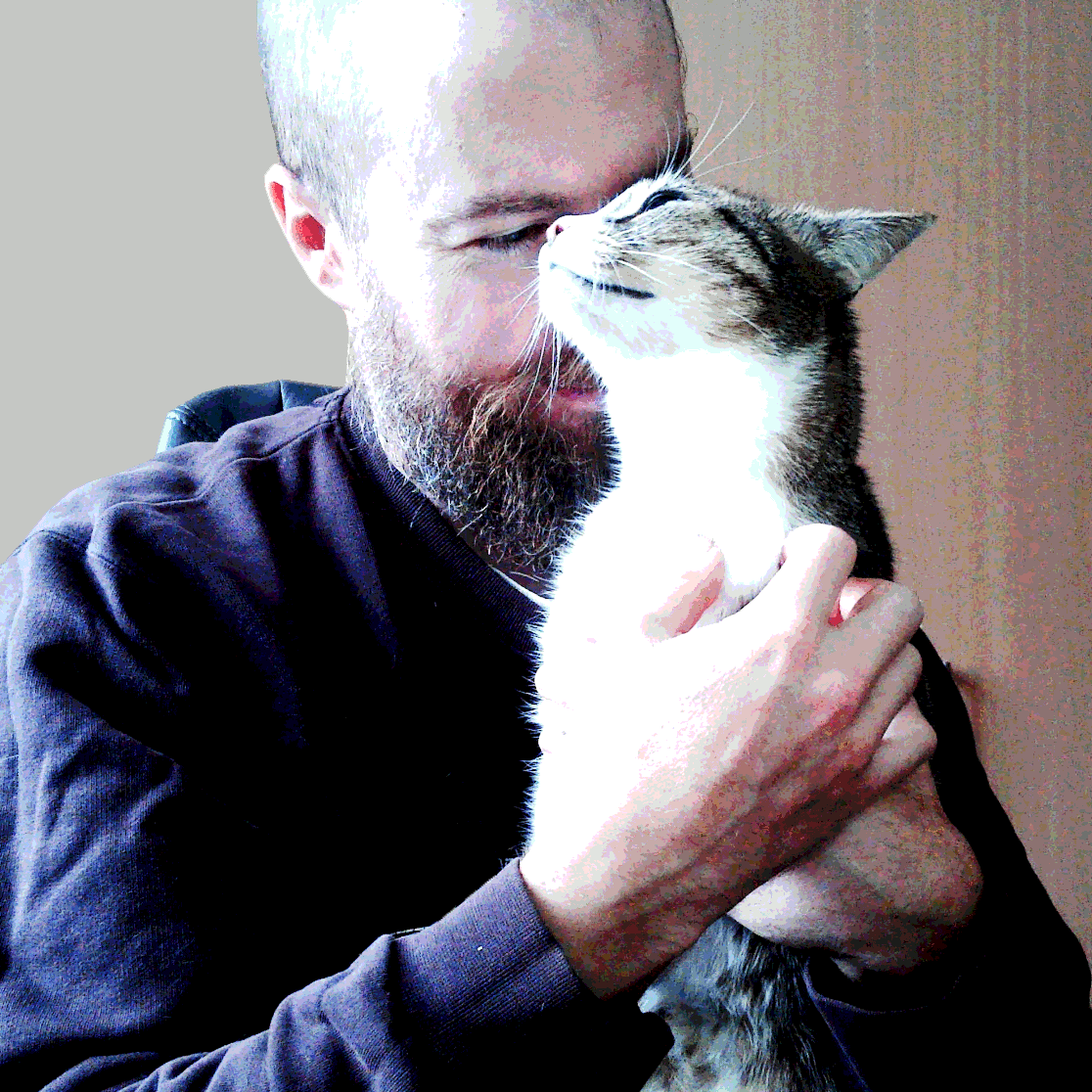
2 min read
Do you need to "extract" each digit of a given number? Then this little TypeScript program is probably exactly what you're looking for. I have inserted some important comments about the "safe" input range, so make sure you are aware of it before using it in critical segments.
/**
* @param num A whole number in the range -21474836489 .. 21474836479
* @returns A number[] with each digit of 'num' separated.
*/
function separateDigits(num: number): number[]
{
let arr: number[] = [];
let lastDigit: number;
let i = 0;
while (num !== 0)
{
lastDigit = num % 10;
arr[i] = lastDigit;
i++;
// Update num to num/10 to cut off last digit:
num = toInt32(num / 10);
}
return arr.reverse();
}
/**
* Fast bitwise operation to truncate a floating point number to get an Int32 value.
* The ~~ bitwise operator is used, where an overflow occurs if the number is too large.
* The safe range is [-2147483648..2147483647].
* @param f A (floating point) number in the range [-2147483648.999..2147483647.999]
* @returns Input 'f' truncated to Int32.
*/
function toInt32(f: number): number
{
// Note that type "number" in JS is always "float" internally, so this func works with both.
// A safe integer division in JavaScript is Math.floor(x/y);
return ~~f;
}
console.log( separateDigits(3412520) );
// Output:
// [3, 4, 1, 2, 5, 2, 0]
Last update: 2022-06-30
0
Subscribe to my newsletter
Read articles from Jan Prazak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
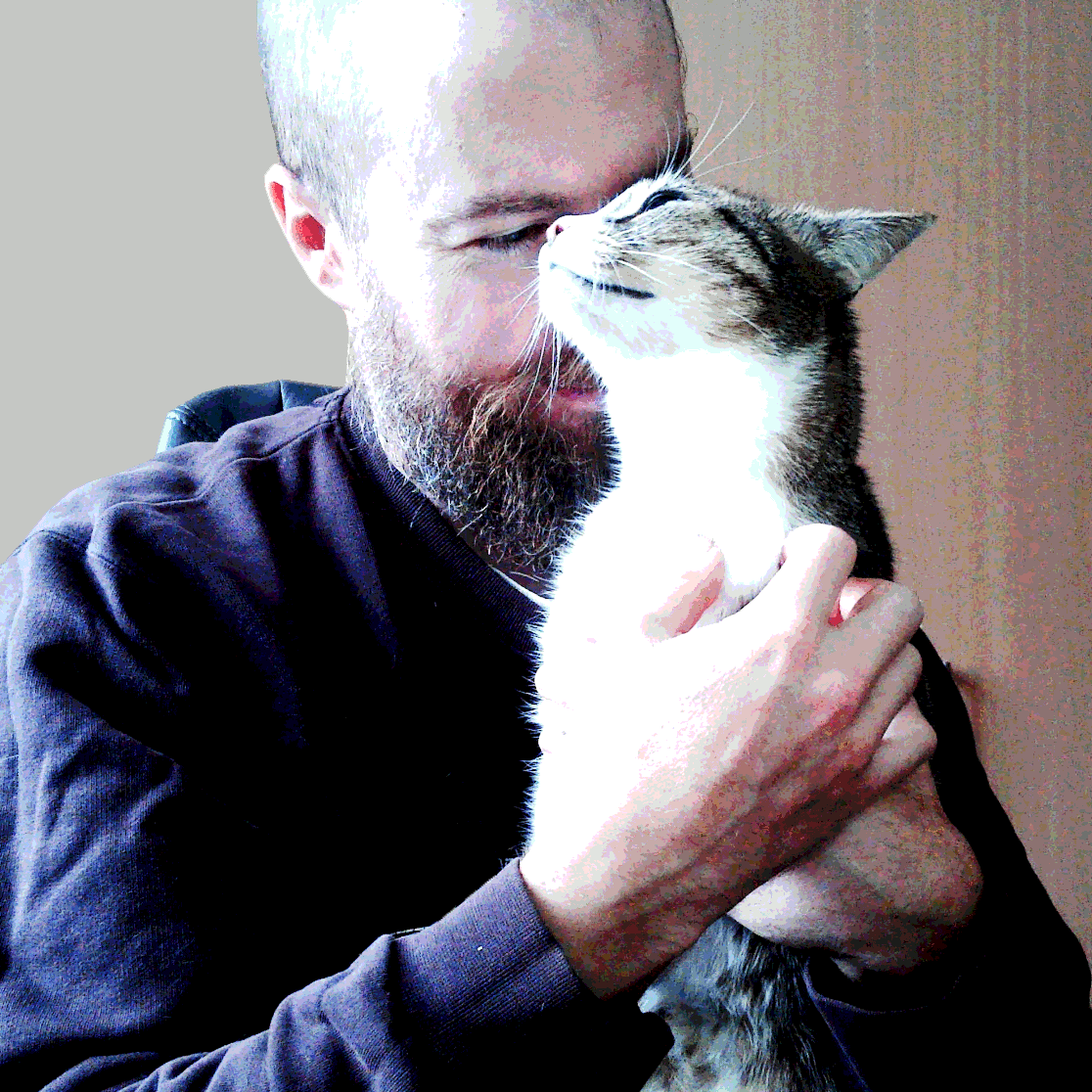
Jan Prazak
Jan Prazak
Webdeveloper, HTML+CSS since 2005, JavaScript since 2015, TypeScript since 2020. I don't consider myself to be an expert; always learning (and forgetting 😸), trying to keep things simple.