2319. Check if Matrix Is X-Matrix

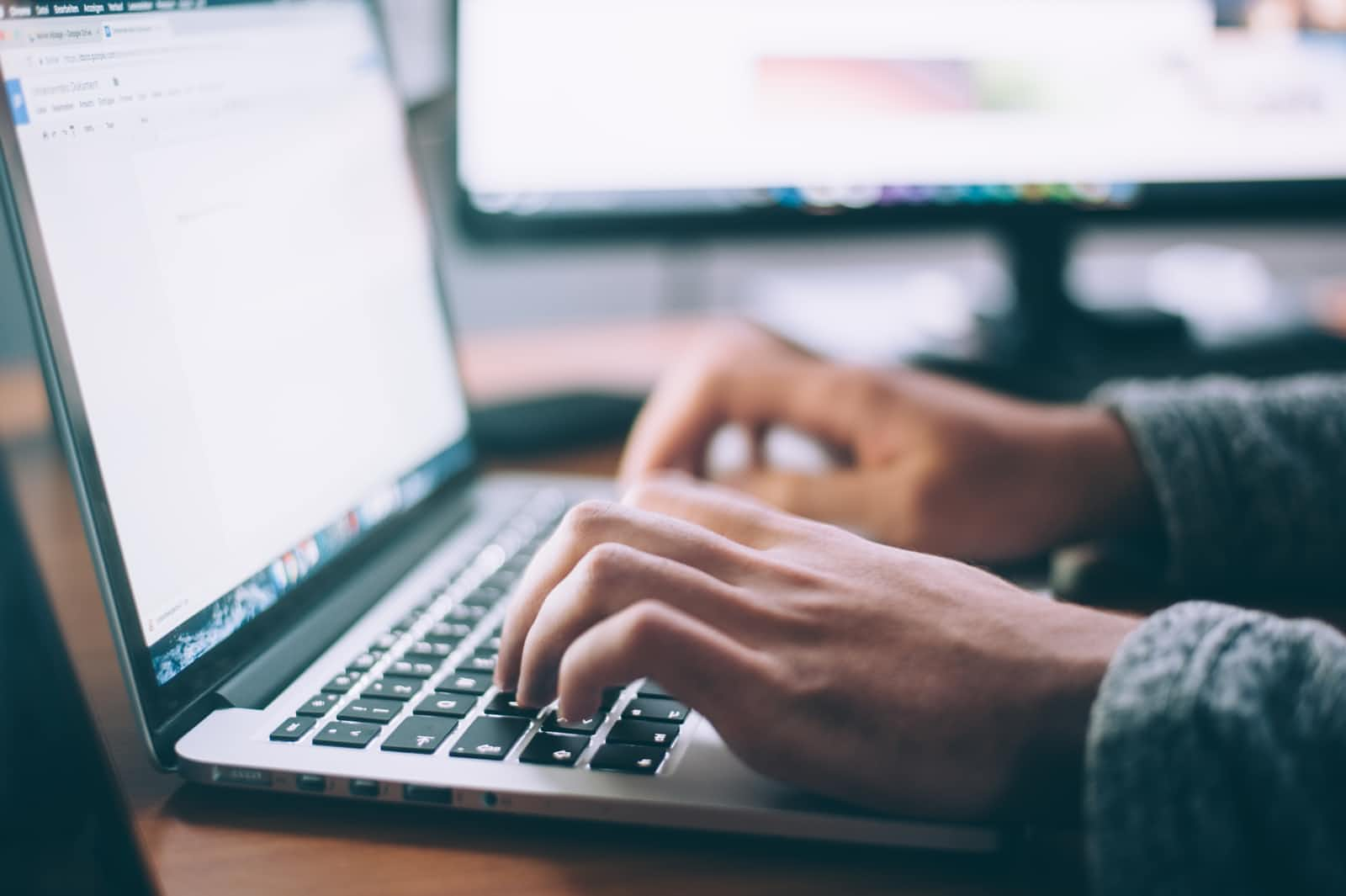
Problem
My Attempt
Brainstorming
X-Matrix All the elements in the diagonals of the matrix are non-zero. All other elements are 0.
So, it need to have 0 in all positions.
num, 0,0,0,num
0,num,0,num,0
0,0,num,0,0
0,num,0,num,0
num, 0,0,0,num
n == grid.length == grid[i].length
3 <= n <= 100
0 <= grid[i][j] <= 105
should count nums. it less than 0's
5/2=2 2/2=1
10/2=5
1000000001
0100000010
0010000100
0001001000
0000110000
0001001000
0010000100
0100000010
1000000001
loop until length/2 for nums. if(i==length/2) x=-1
i+x to check pos.
Result code
class Solution {
public boolean checkXMatrix(int[][] grid) {
int len = grid.length;
for (int i = 0; i < (grid.length / 2); ++i) {
if (!chkArray(grid[i], i + 1)) return false;
if (!chkArray(grid[len - 1 - i], i + 1)) return false;
}
return true;
}
public static boolean chkArray(int[] grid, int count) {
for (int i = 0; i < grid.length; ++i) {
if (grid[i] != 0) {
if (i != (count - 1) || i != (grid.length - count)) {
return false;
}
}
}
return true;
}
}
Wrong Answer
Study
chkArray method was wrong. logic was fine. but the code is wrong. I needed 20mins more+IDE to fix it. need to practice hard code.
Solution2 is using Recursion. For me, this is not easy to understand.. need to study.
Solution (time, space)
O(n/2), O(1)
class Solution {
public boolean checkXMatrix(int[][] grid) {
for (int i = 0; i < (grid.length / 2); ++i) {
if (!chkArray(grid[i], i + 1)) return false;
if (!chkArray(grid[grid.length - 1 - i], i + 1)) return false;
}
return true;
}
public static boolean chkArray(int[] grid, int count) {
for (int i = 0; i < grid.length; ++i) {
if (i != (count - 1) && i != (grid.length - count)) {
if (grid[i] != 0) return false;
} else {
if (grid[i] == 0) return false;
}
}
return true;
}
}
Runtime: 1 ms, faster than 99.85% of Java online submissions for Check if Matrix Is X-Matrix. Memory Usage: 53.9 MB, less than 83.86% of Java online submissions for Check if Matrix Is X-Matrix.
Solution2 (time, space)
O(n/2), O(1)
class Solution {
public boolean checkXMatrix(int[][] grid) {
return checkRect(grid, 0, grid.length - 1);
}
boolean checkRect(int[][] grid, int first, int last) {
if (grid[first][first] == 0 ||
grid[first][last] == 0 ||
grid[last][first] == 0 ||
grid[last][last] == 0) return false;
for (int i = first + 1; i < last; i++) {
if (grid[first][i] != 0 ||
grid[last][i] != 0 ||
grid[i][first] != 0 ||
grid[i][last] != 0) return false;
}
if (first + 1 >= last) return true;
return checkRect(grid, first + 1, last - 1);
}
}
Subscribe to my newsletter
Read articles from Han directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
