Python String Formats

2 min read
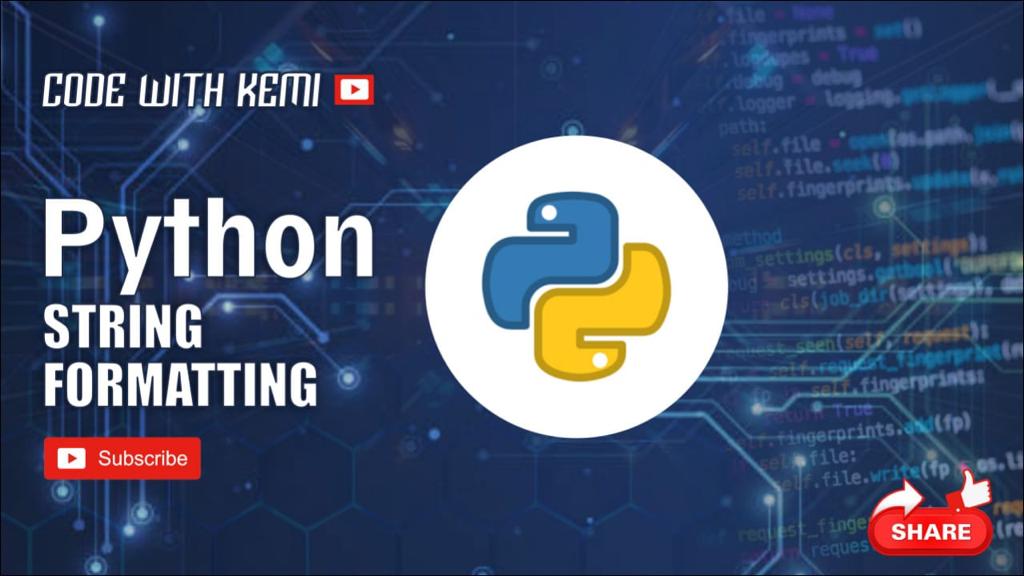
There are couple of ways you could format your strings. Formatting your code makes it flexible for use. Here are some ways you could format your code:
The use of %
% is used to format different kinds of inputs. Here are a couple of ways you could use it. %d is used to format digits. %s is used to format strings. %x is used for hexadecimal number.
Example:
x = 'looked'
print('Aisha %s and %s around'%('walked', x))
The % placeholder gets replaced by the string value defined. So the first %s gets replaced by 'walked' and the second %s gets replaces by 'looked'.
num = 7
print('There are %d days in a week'%num)
%d is a format for digits. num values replaces the placeholder for digits.
>>>hex_num = 50159747054
>>>print('there is a %x error'%hex_num)
'there is a badc0ffee error'
Format method (str.format)
name = 'Bill'
>>>‘Hello {}’.format(name)
Hello Bill
>>>print('My name is {name}, my age is {age}, my location is {location}'.format(name='Smith', age=13, location='Africa'))
My name is Smith, my age is 13, my location is Africa
>>>print('My name is {0}, my age is {1}, my location is {2}'.format('Smith',13, 'Africa'))
My name is Smith, my age is 13, my location is Africa
String Interpolation/ f-string
name = ‘Will’
>>> print(f'The author\'s name is {name}')
The author's name is Will
>>> a = 5
>>> b = 7
>>> print(f'The multiple of {a} and {b} is {(a * b)}.')
The multiple of 5 and 7 is 35.
The use of Template
>>>from string import Template
>>>name = 'Richie'
>>>t = Template('Hey, $name')
>>>t.substitute(name=name)
'Hey, Richie'
The use of +
>>>firstname = 'Will'
>>>lastname = 'Salah'
>>>print(firstname +' ' +lastname)
Will Salah
The use of ,
>>>firstname = 'Will'
>>>lastname = 'Salah'
>>>print(firstname , lastname)
Will Salah
1
Subscribe to my newsletter
Read articles from Rukayat Balogun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
