Valid Palindrome - Leetcode
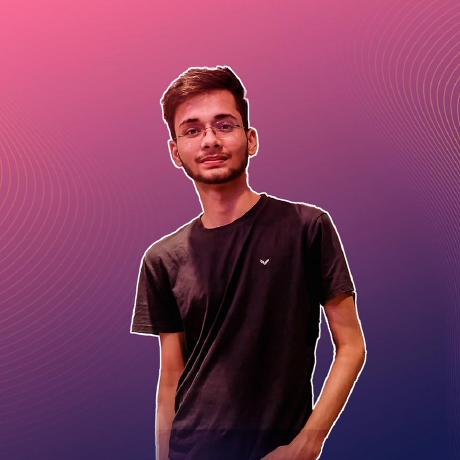
Table of contents
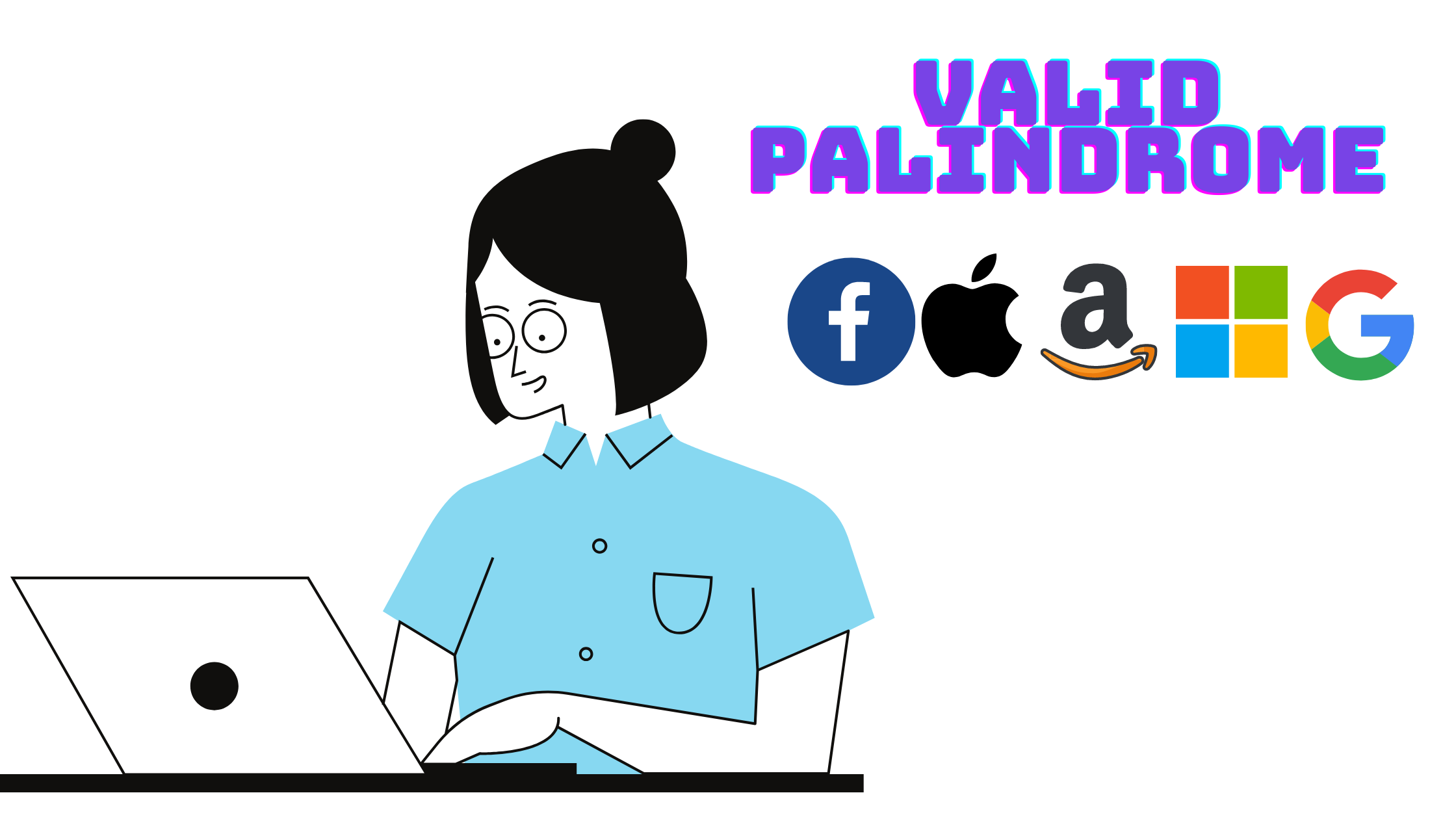
Introduction
In this article of the series we're covering the valid palindrome problem from leetcode. We'll be covering all the problems with explanation, code, time and space complexity in Grind 75 sheet. Grind75 is a curated list of Algorithmic questions from LeetCode that have been very famous from quite a long time. These question have been asked in top tech companies such as Google, Microsoft, Amazon, Facebook, Netflix, Apple, and other top tier companies as well. Do follow for further updates.
Question
A phrase is a palindrome if, after converting all uppercase letters into lowercase letters and removing all non-alphanumeric characters, it reads the same forward and backward. Alphanumeric characters include letters and numbers.
Given a string s
, return true
if it is a palindrome, or false
otherwise.
Example 1:
**Input**: s = "A man, a plan, a canal: Panama"
**Output**: true
**Explanation**: "amanaplanacanalpanama" is a palindrome.
Example 2:
**Input**: s = "race a car"
**Output**: false
**Explanation**: "raceacar" is not a palindrome.
Example 3:
**Input**: s = " "
**Output**: true
**Explanation**: s is an empty string "" after removing non-alphanumeric characters.
Since an empty string reads the same forward and backward, it is a palindrome.
Explanation
The first thing we note here is that we only considering AlphaNumeric. So we can ignore all the special characters other than Alphabets and Numbers.
We'll use two pointer approach to tackle this problem.
Take a start
variable and a end
variable, and initialize it with 0
and s.length()-1
respectively.
After that loop through the string till out start < end
Now we need to check if the character at start and end is either AlphaNumeric Character or not. For that we'll use built in method in java Character.isLetterOrDigit()
, this'll return true if our character is AlphaNumeric else it'll return false.
If character is not AlphaNumeric we'll increase our start and end after checking for both respectively.
If both case fails that means our current character is AlphaNumeric, now we only need to check if character at both the positions are same or not. If they aren't same return false
right away otherwise continue doing the same.
Code
public boolean isPalindrome(String s) {
int start = 0, end = s.length()-1;
while(start < end) {
char c1 = s.charAt(start);
char c2 = s.charAt(end);
if(!Character.isLetterOrDigit(c1)) {
start++;
}
else if(!Character.isLetterOrDigit(c2)) {
end--;
}
else{
if(Character.toLowerCase(c1) != Character.toLowerCase(c2)) {
return false;
}
start++; end--;
}
}
return true;
}
Time Complexity - O(N), as we'll end up scanning whole string in worst case when no character of string match.
Space Complexity - O(1), we're not using any extra space.
Subscribe to my newsletter
Read articles from Aryan Srivastava directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
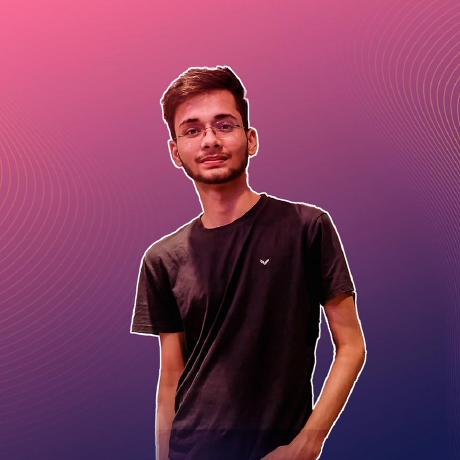