Python Sets
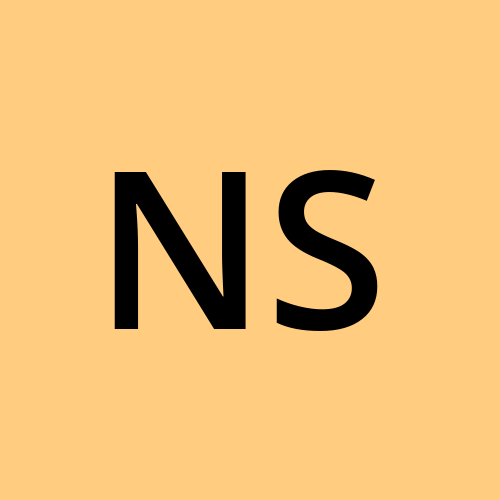
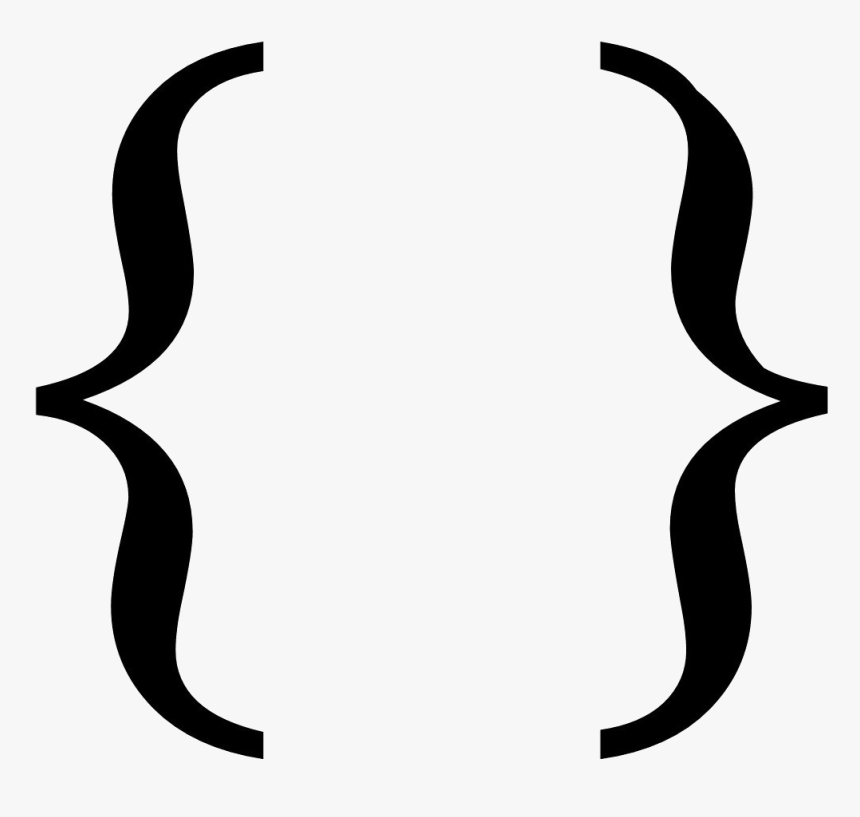
What are Sets?
Sets are used to store multiple items in a single variable.
myset = {"bigsmoke", "isnt", "hacker"}
A set is a collection which is unordered and unindexed and do not allow duplicate values.
len()
We can use the function len(set1) to find the length of set.
set1 = {"bigsmoke", "hulk", "thor"} print(len(set1)) #4
Access Set Items (using loops)
We can’t use indexing in sets to access a item. But we can use for loop to do this.
set1 = {"bigsmoke", "hulk", "thor"} for i in set1: print(i)
add()
To add one item to a set use the add() method.
set1 = {"bigsmoke", "hulk", "thor"} set1.add("iron man") print(set1) # OUTPUT # {"bigsmoke", "hulk", "thor", "iron man"}
set1 = {"bigsmoke", "hulk", "thor"} set2 = {"batman", "superman", "joker"} set3 = set1.update(set2) print(set3)
set1 = {"bigsmoke", "hulk", "thor"} set1.remove("bigsmoke") print(set1)
Delete an Entire Set
The del keyword will delete the set completely.
set1 = {"bigsmoke", "hulk", "thor"} del set1 print(set1) # You will get an error while running this function as "set1" is not defined
Clear an Entire Set
The clear() function will clear all the elements present in the set.
set1 = {"bigsmoke", "hulk", "thor"} set1.clear() print(set1)
Copy of a set
The copy() function will copy all the elements from one set to another.
set1 = {"bigsmoke", "hulk", "thor"} set2 = set1.copy() print(set2)
Difference between two sets
The difference() function returns a set containing the difference between two or more sets.
set1 = {apple, banana, mango} set2 = {microsoft, apple, oneplus} set3 = set1.difference(set2) print(set3)
Printing single time appeared in both sets
The difference_update() removes the items in this set that are also included in another, specified set.
set1 = {"apple", "banana", "mango"} set2 = {"microsoft", "apple", "oneplus"} set3 = set1.difference_update(set2) print(set3)
Removing the specified item
The discard() function removes the specified item
fruits = {"apple", "banana", "cherry"} fruits.discard("banana") print(fruits) # {"apple", "cherry"}
Printing the intersection of two or more sets
The intersection() function returns a set, that is the intersection of two or more sets
x = {"apple", "banana", "cherry"} y = {"google", "microsoft", "apple"} z = x.intersection(y) print(z) # {"apple"}
Removing item that is not present in both sets
The intersection_update() function removes the items in this set that are not present in other, specified set(s)
x = {"apple", "banana", "cherry"} y = {"google", "microsoft", "apple"} x.intersection_update(y) print(x) # As apple is common in both sets # {"apple"}
Intersection of two sets possible or not
The isdisjoint() function returns whether two sets have an intersection or not
x = {"apple", "banana", "cherry"} y = {"google", "microsoft", "facebook"} z = x.isdisjoint(y) print(z) # Returns True if no common/same value is present in both sets. # True
To check whether set1 is present in set2
The issubset() function returns whether another set contains this set or not
x = {"a", "b", "c"} y = {"f", "e", "d", "c", "b", "a"} z = x.issubset(y) print(z) # Returns True if x set is present in y set # True
To check whether set2 is present in set1
The issuperset() function returns whether set 1 contains set 2 or not
x = {"f", "e", "d", "c", "b", "a"} y = {"a", "b", "c"} z = x.issuperset(y) print(z) # Return True if set y is present in set x # True
Removing an element from the set
The pop() function removes a random element from the set
fruits = {"apple", "banana", "cherry"} fruits.pop() print(fruits) # It can remove random element so you can't say any specific value
Removing a specific element from the set
The remove() function removes the specified element
fruits = {"apple", "banana", "cherry"} fruits.remove("banana") print(fruits) # Removes banana from the set # {"apple", "cherry"}
To find symmetric difference between two sets
The symmetric_difference() function returns a set with the symmetric differences of two sets
x = {"apple", "banana", "cherry"} y = {"google", "microsoft", "apple"} z = x.symmetric_difference(y) print(z) # It returns values that are different in both sets # {"banana", "cherry", "google", "microsoft"}
To insert symmetric difference elements from both sets
The symmetric_difference_update() function inserts the symmetric differences from this set and another
x = {"apple", "banana", "cherry"} y = {"google", "microsoft", "apple"} x.symmetric_difference_update(y) print(x) # It functions same as symmetric_difference() function # {"banana", "google", "cherry", "microsoft"}
To add elements from both sets
The union() function returns a set, containing the union of sets
set1 = {"bigsmoke", "hulk", "thor"} set2 = {"batman", "superman", "joker"} set3 = set1.union(set2) print(set3) # It unifies both the sets #{"bigsmoke", "hulk", "thor", "batman", "superman", "joker"}
Updating set with another set
The update() function updates the set with another set, or any other iterable
x = {"apple", "banana", "cherry"} y = {"google", "microsoft", "apple"} x.update(y) print(x) # It combines two sets into one # {"apple", "banana", "cherry", "google", "microsoft"}
Subscribe to my newsletter
Read articles from Namya Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
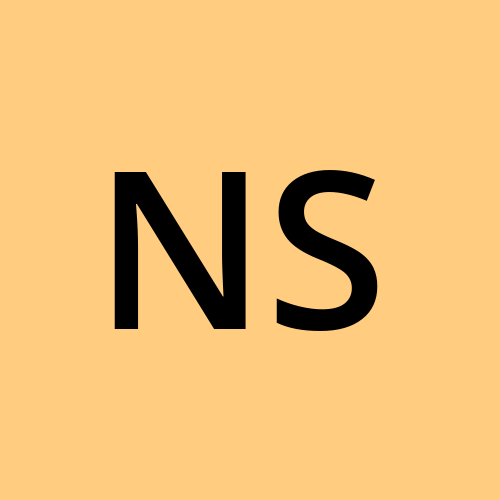
Namya Shah
Namya Shah
I am a developer who is very enthusiast about technology and coding.