Python String Methods
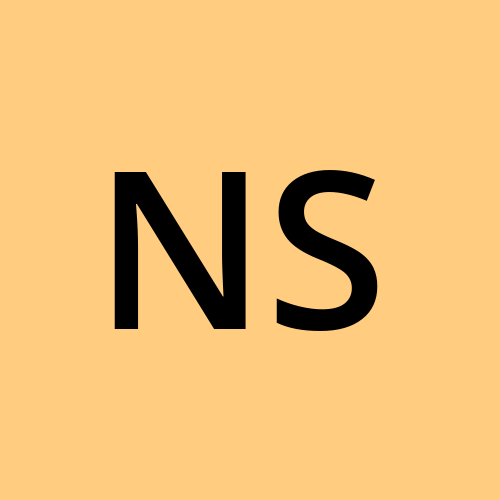
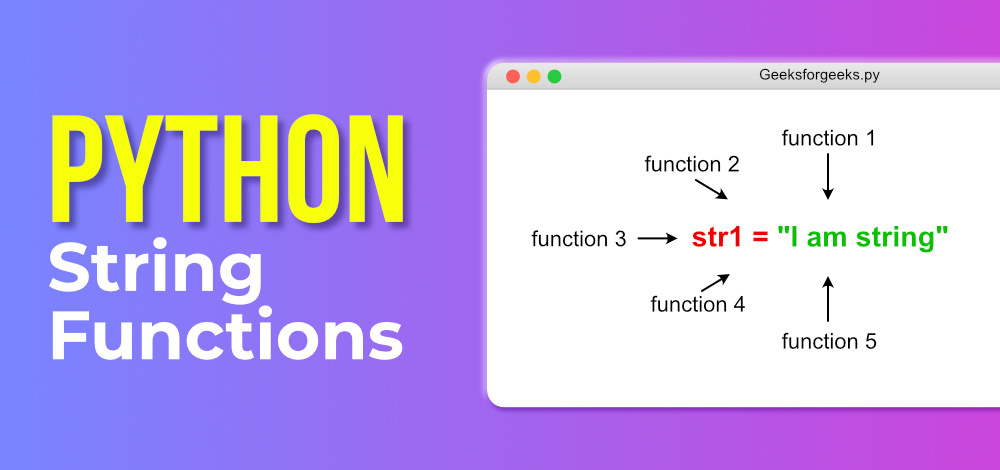
String is an immutable sequence data type.
It is the sequence of Unicode characters wrapped inside single, double, or triple quotes.
Functions
capitalize()
Converts the first character to upper case
txt = "hello world!" x = txt.capitalize() print(x)
casefold()
Converts string into lower case
txt = "Hello, World!" x = txt.casefold() print(x)
center()
Returns a centered string
txt = "mercedes" x = txt.center(10) print(x)
count()
Returns the number of times a specified value occurs in a string
txt = "I love buggatti, buggatti is my favourite company." x = txt.count("buggatti") print(x)
encode()
Returns an encoded version of the string
txt = "I love buggatti, buggatti is my favourite company." x = txt.encode() print(x)
endswith()
Returns true if the string ends with specified value
txt = "I love buggatti, buggatti is my favourite company." x = txt.endswith(".") print(x)
expandtabs()
Sets the tab size of the string
txt = "I love buggatti, buggatti is my favourite company." x = txt.expandtabs(3) print(x)
find()
Searches the string for a specified value and returns the position of where it was found
txt = "I love buggatti, buggatti is my favourite company." x = txt.find("buggatti") print(x)
format()
Formats specified values in a string
txt = "For only {price:.2f} dollars!" print(txt.format(price = 49))
join()
- Convers the elements of an iterable into a string
company = ("Mercedes", "Buggatti", "Pagani") x = "vs".join(company) print(x)
lower()
- Converts string into lower case
txt = "HELLO, WORLD!" x = txt.lower() print(x)
maketrans()
- Returns a translation table to be used in translations
txt = "HELLO, WORLD!" x = txt.maketrans("WORLD", "PEOPLE")) print(x)
replace()
- Returns a string where a specified value is replaced with a specified value
txt = "HELLO, WORLD!" x = txt.replace("WORLD", "PEOPLE")) print(x)
split()
- Splits the string at the specified separator, and returns a list
txt = "Welcome to my world!" x = txt.split() print(x)
splitlines()
- Splits the string at line breaks and returns a list
txt = "Welcome to my world!" x = txt.splitlines() print(x)
startswith()
- Returns True if the string starts with the specified value
txt = "Welcome to my world!" x = txt.startswith("Welcome") print(x)
strip()
- Returns a trimmed version of the string
txt = " People " x = txt.strip() print("Welcome", x)
swapcase()
- Swap cases, lower case becomes upper case and vice versa
txt = "Welcome to my WORLD!" x = txt.swapcase() print(x)
title()
- Converts the first character of each word to upper case
txt = "welcome to my world!" x = txt.title() print(x)
upper()
- Converts a string into upper case
txt = "Welcome to my world!" x = txt.upper() print(x)
String Checker
isalnum()
Returns True if all characters in the string are alphanumeric
txt = "AstonMartin007" x = txt.isalnum() print(x)
isalpha()
Returns True if all characters in the string are in the alphabet
txt = "SpaceX" x = txt.isalpha() print(x)
isascii()
Returns True if all characters in the string are ascii characters
txt = "AstonMartin007" x = txt.isascii() print(x)
isdecimal()
Returns True if all characters in the string are decimals
txt = "\u0033" #unicode for 3 x = txt.isdecimal() print(x)
isdigit()
Returns True if all characters in the string are digits/numbers
txt = "2580" x = txt.isdigit() print(x)
isidentifier()
Returns True if the string is an identifier
txt = "Demo" x = txt.isidentifier() print(x)
islower()
Returns True if all characters in the string are lower case
txt = "song" x = txt.islower() print(x)
isprintable()
Returns True if all characters in the string are printable
txt = "2580" x = txt.isprintable() print(x)
isspace()
Returns True if all characters in the string are whitespaces
txt = " " x = txt.isspace() print(x)
istitle()
- Returns True if the string follows the rules of a title
txt = "This is my code" x = txt.istitle() print(x)
isupper()
- Returns True if all characters in the string are upper case
txt = "CODE" x = txt.isupper() print(x)
Subscribe to my newsletter
Read articles from Namya Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
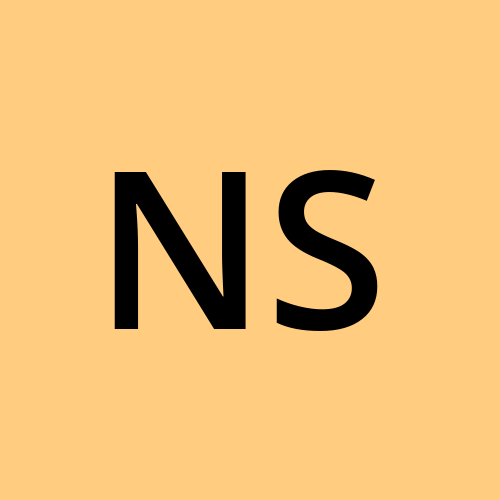
Namya Shah
Namya Shah
I am a developer who is very enthusiast about technology and coding.