[Unity] Electric Energy

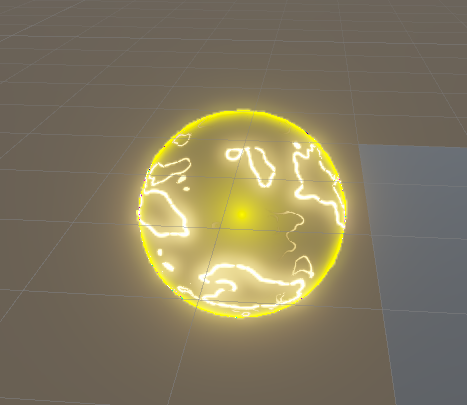
How I started
Recently, I've been thinking about creating an FPS wave game where the player defeats a big wave of monsters. Typical. Since I loved fantasy, I wanted one of the skills to be about shooting magical, and what can be more magical than a glowing electricity ball? Here's how I did it.
Disclaimer
Unity Version: 2021.3.6f1 Render Pipeline: Universal Render Pipeline
A great amount of my project comes from Gabriel Aguiar Prod. He does a great job at making game VFX tutorials. I highly suggest it to people looking for a more in-depth understanding of unity game effects. Videos Referenced:
- Unity Shader Graph - Electricity Shader Effect Tutorial
- Unity VFX Graph - Hits and Impact Effects Tutorial
Electricity Shader
The great thing about this method is that you don't need any textures--it's all created from generated textures. This also means the electricity effect can be used in different ways, which I will talk about later.
{Pic 1}
{Pic 2}
{Pic 3}
How it works
Two noises move in different directions. By adding two noises, the textures become very complex. (Pic 1) Then, the final noise is put into a 'Rectangle' node. This causes the rectangle texture to deform and become similar to sparking electricity. (Pic 2) Lastly, the edges are smoothened using the reverse of the 'polar coordinates' node, which makes a white circle. This means that the outer parts are softened. (Pic 3)
Applying it into a material
After the shader is created, I create a material based on the shader (by right-clicking on the shader and creating 'material' via the menu). This material can be used in other parts of the game.
Using the material
For example, the material can be used in a particle system to create electricity sparking effect. This is also what Gabriel did in his tutorial. However, what I did was I applied the material as the second material of a ball.
Shader for the ball
Obviously, this didn't look very interesting, so I made a different shader for the ball.
How it works
I used the fresnel effect, where the edge of the material gets highlighted. If I only used it once, the center of the ball becomes empty, so I added a reversed fresnel effect with a normal fresnel effect to create this effect.
Final Product
In the end, a simple electric ball was created. After that, I added a rigidbody to the ball, created a small explosion effect with VFX, and wala! A paralyzing ball!
Code for the electric ball
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.VFX;
public class ElectricBall : MonoBehaviour
{
private Rigidbody rb;
[SerializeField]
private float speed = 2;
[SerializeField]
private Material electric;
[SerializeField]
private GameObject flashPrefab;
// Start is called before the first frame update
void Start()
{
rb = GetComponent<Rigidbody>();
}
// Update is called once per frame
void Update()
{
rb.velocity = Vector3.forward * speed;
}
private void OnCollisionEnter(Collision other) {
Material[] origials = other.gameObject.GetComponent<Renderer>().materials;
int matCount = origials.Length;
Material[] materials = new Material[matCount + 1];
for (int i = 0; i < matCount; i++)
{
materials[i] = origials[i];
}
materials[matCount] = electric;
other.gameObject.GetComponent<Renderer>().materials = materials;
GameObject flash = Instantiate(flashPrefab, transform.position, Quaternion.identity);
flash.GetComponent<VisualEffect>().Play();
Destroy(gameObject);
}
}
Subscribe to my newsletter
Read articles from Taehyun Han directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
