Understanding CSS Selectors
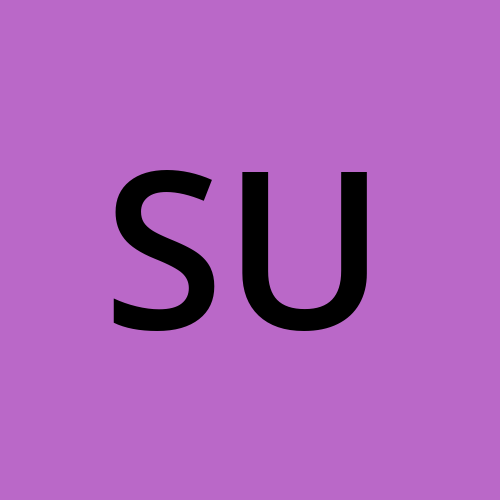
Table of contents
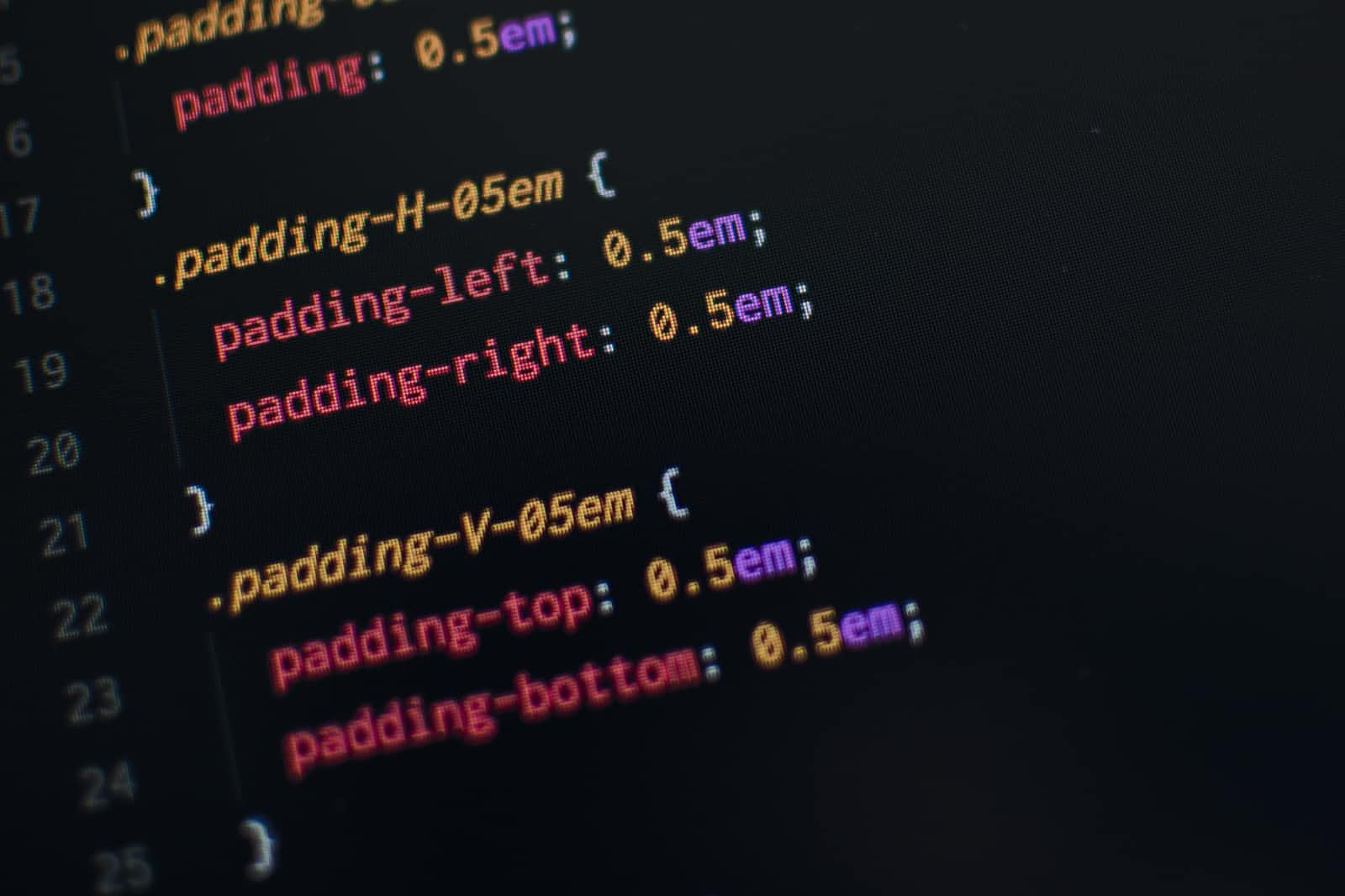
In this article, You'll be provided a good understanding of CSS Selectors. Knowing about the Selectors can make one's life easy while styling a complex html page.
CSS Selectors are used to target html elements that we want to style with CSS. Let us dive into the different kind of selectors usage with some code snippets.
Basic Selectors
Universal Selector : Universal selector is used to apply css styles to all the elements that are there in the HTML page. An Asterisk()* is used in the stylesheet to target all the elements.
//HTML
<body>
<section>
<h1>I turned into Orange</h1>
</section>
<div>
<h3>I too turned into Orange, But why ?</h3>
<p>So am I. That's due to universal selector</p>
</div>
</body>
//CSS
* {
color: orange;
}
ID Selector : ID selectors helpful to style HTML elements based on the value of its id attribute. A hash(#) is used as prefix to the id value on stylesheet while targeting that element
//HTML
<body>
<div>
<h3 id="text">I turned into Orange</h3>
<p>I will remain in my original color</p>
</div>
</body>
//CSS
#text {
color: orange;
}
Class Selector : Similar to ID Selector, Class selectors are also used to target one or more HTML elements based on value of its class attribute. A dot(.) is used as prefix to the class name on stylesheet.
//HTML
<body>
<div>
<h3 class="text">I turned into Orange</h3>
<p>I will remain in my original color</p>
</div>
</body>
//CSS
.text {
color: orange;
}
Type Selector : Type selectors are used to target HTML elements of that particular type. HTML elements matching that type will get effected with applied styles
//HTML
<body>
<div>
<h3>I will remain in my original color</h3>
<p>I turned into Orange</p>
</div>
</body>
//CSS
p {
color: orange;
}
Group Selector : Group selectors are very useful when we want to apply same styles to different type of HTML elements. This will reduce the size of our code. We can mention all the types on style sheet separated by a comma(,).
//HTML
<body>
<div>
<h3>I turned into orange..</h3>
<p>I too turned into orange!!</p>
</div>
</body>
//CSS
p, h3 {
color: orange;
}
Combinators
Descendant combinator : Descendant combinators are used to target the matching descendants of an HTML element. The syntax on stylesheet will be element name followed by a space(" ") and then the descendant you want to target.
//HTML
<body>
<section>
<div class="text">
I turned into orange..
</div>
<p>I will remain in my original color.</p>
</section>
</body>
//CSS
section div {
color: orange;
}
Child Selector : Child Selectors are used to target the immediate matching children of an HTML element. The syntax will be Parent element followed by greater than (>) and Child element that needs to be targeted.
//HTML
<body>
<section>
<div class="text">
<h1> I turned into orange.. </h1>
<p>I will remain in my original color.</p>
</div>
</section>
</body>
//CSS
.text > h1 {
color: orange;
}
Adjacent Sibling Selector : Adjacent Sibling Selectors are used to target the immediate next sibling of an HTML element. The syntax will be element above our target element followed by a plus(+) sign and target element.
//HTML
<body>
<div>
<h1>I will remain in my original color.</h1>
<p>I will remain in my original color.</p>
</div>
<p>I turned into Orange as I am next to div</p>
<p>I will remain in my original color.</p>
</body>
//CSS
.div + p {
color: orange;
}
General Sibling Selector : General Sibling Selectors are used to target the all next immediate siblings of an HTML element. The syntax will be element above our target elements followed by a tilt(~) and target element.
//HTML
<body>
<div>
<h1>I will remain in my original color.</h1>
<p>I will remain in my original color.</p>
</div>
<p>I turned into Orange as I exist after div</p>
<h3>I will remain in my original color.</h3>
<p>I turned into Orange as I exist after div</p>
</body>
//CSS
.div ~ p {
color: orange;
}
Pseudo Selectors
Pseudo Classes : Pseudo Classes lets you to apply styles when the element is in a particular state. These are seen very often while using hover effects. The Syntax will be the selector followed by a colon(:) and pseudo class.
//HTML
<body>
<button>Hover Me</button>
</body>
//CSS
button:hover{
background-color: orange;
}
//output
Pseudo Elements : Pseudo Elements lets you to style at specified parts of content . Pseudo elements has a syntax where the element is pre-fixed a double colon(::)
//HTML
<body>
<p> a html tag</p>
</body>
//CSS
p::before{
content: 'This is';
}
//output - This is a html tag
I'm leaving a couple of resources for you to dig deep and explore more on Selectors.
Subscribe to my newsletter
Read articles from Surya Uppalapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
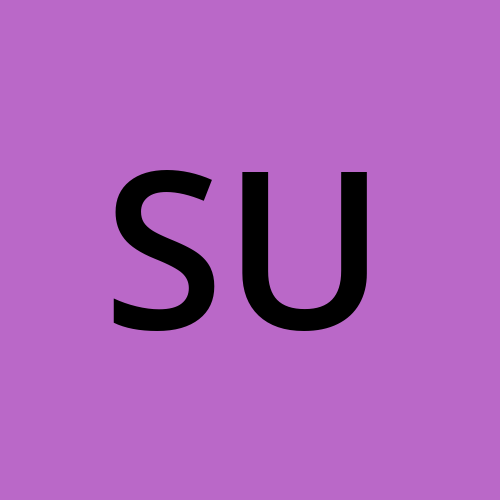
Surya Uppalapati
Surya Uppalapati
Software Developer | MERN | AWS