Read+Create QR Code with Python
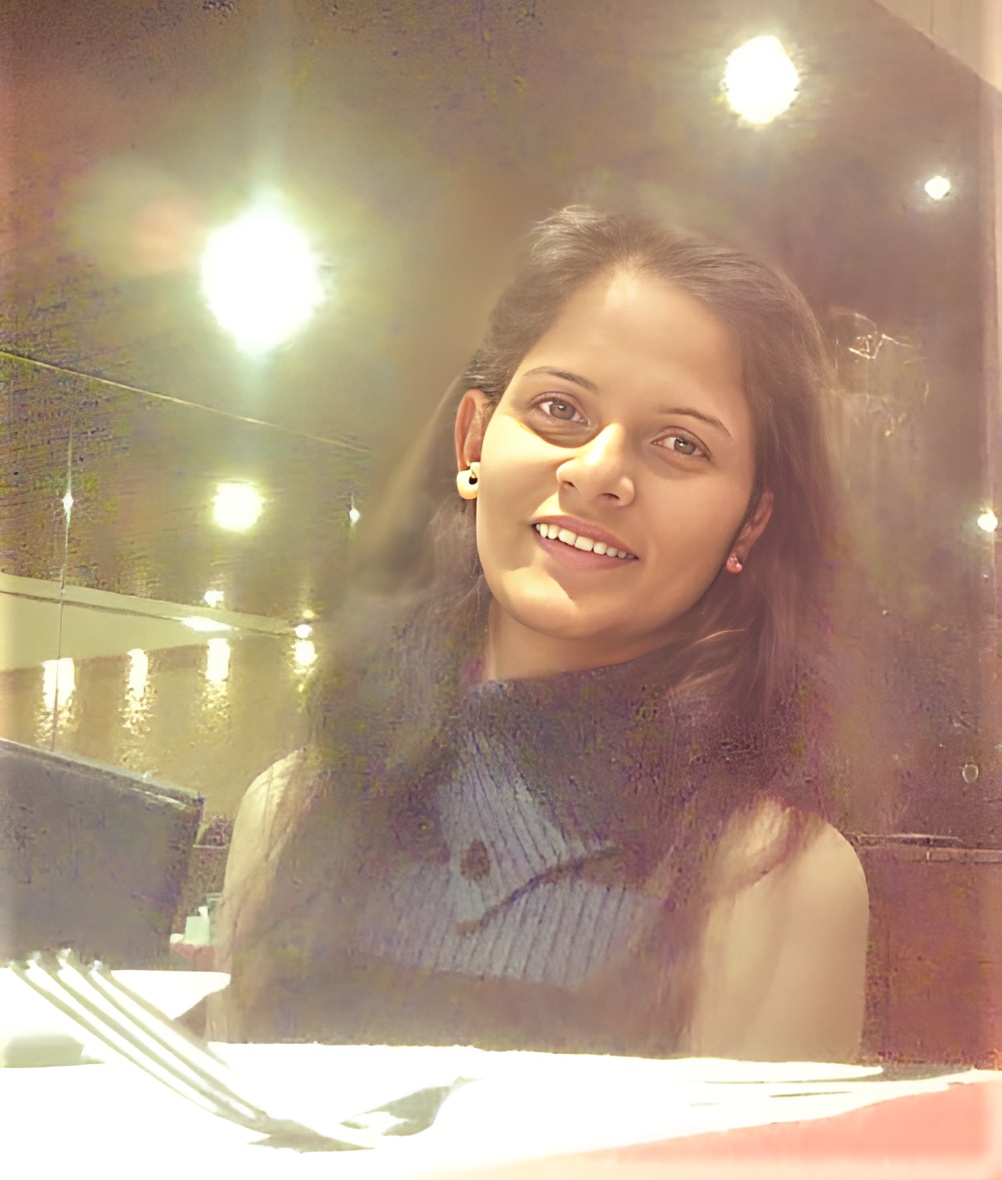
Table of contents
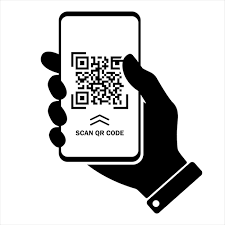
QR Code stands for Quick Response Code. It was developed by a Japanese Automotive Company Denso Wave in 1994. It works similarly to that of a barcode but, is much faster as it processes the information within few seconds. Unlike a barcode reader that is required to read information embedded in barcodes, QR Codes do not require specials devices and can be accessed by a smartphone camera also.
QR Code can be used in many ways like to show product details, track product delivery, to show menu to customers in restaurants,to get access to any webinar or any other site or link, for accessing social media platforms and to transfer money (Gpay,PhonePay,BharatPay,Paytm).
Advantages:-
- Small in size:- QR-Codes are usually very small in size. They will require minimal space on your ad banner, pamphlet, product, or any other place you wish to use it.
- Easier to scan:- QR-Code can be scanned by our mobile camera and does not require any special device to interpret the message.
- High storage capacity:- QR-Code can store a good amount of information. Commonly, they can store up to 7089 digits or 4296 characters. They can store data in the form of image, video, URL, etc.
- Works even when damaged:- It is possible that if we print a QR-Code on paper or any wrapper, some portion might get damaged a bit. However, QR-Code will work even if only 30% of the code is unreadable.
Disadvantages:-
- Not secure:- Any person with a smartphone can get access to information stored in code. It is not recommended to make QR-Codes of confidential data.
Unfamiliarity:- In India, not every person is familiar with the use of a QR-Code on billboard or pamphlets. One might need to convey the message further so that it can be of use.
Now as we discussed much about QR code, its time to jump into actual coding session.
- first will cover how to create QR code then will go ahead with QR code reading.
So Lets start.....
for this code I am using VSCode as code editor and python as a language.
1)Create QR Code
We will be using 'qrcode' package for generating QR code. The first step is installing the package using pip command.
pip install qrcode
- Import module
- Create Qrcode with qrcode.make()
- Save into image
Example 1:
simple example to create qr code with default parameters
#Import module
import qrcode
#Generate QR Code
img=qrcode.make('www.google.com')
img.save('qrimage.png')
one can use smartphone to scan above QR code and easily access anything associated with that qrcode easily without any hassle .
Example 2:
QR code can be customized using QRCode object which has the following parameters:
i. version: There are 40 versions of QR code which controls the size of the code. 1 being the smallest and 40 being the largest. Version 1 will create a 21X21 matrix QR Code.
ii. error_correction: This parameter controls the Error Correction used for the QR code. This varies from 7% to 30% error correction as below. ERROR_CORRECT_L: up to 7% ERROR_CORRECT_M: up to 15% ERROR_CORRECT_Q: up to 25% ERROR_CORRECT_H: up to 30%
iii. box_size: This parameter controls the number of pixels in each box of the QR code
iv. border: This parameter controls the thickness of the border. The default border is 4 pixels thick.
The QRCode object has the following functions which can be used to create the QR Code.
i. add data: The content of the QR code can be passed as an argument to this function.
ii. make: If you are not sure about which version of QR code to use, the version can be set automatically by : a. setting version parameter to None and b. seting fit parameter of make to True.
iii. make image: This function generates the QR code. It can also be used to set the fill color and background color of the QR code using fill_color and back_color arguments.
#Import module
import qrcode
print("QR CODE GENERATOR")
a= input("Enter link which you of want to make qr code")
#set QR code parameteres
qr = qrcode.QRCode(
version = 10,
box_size = 5,
border = 1
)
#storing input given into data variable
data = a
qr.add_data(data)
#Generate QR Code
qr.make(fit = True)
#set QR code properties
img = qr.make_image(fill="black",back_color = "white")
#save QR code
img.save("QrCodeImage.png")
python qr.py
QR CODE GENERATOR
Enter link which you of want to make qr code: gmail.com
2.Read QR code
To read QR code ,we will be using opencv. for using opencv first have install it using following command:
pip install cv2
But you may face problem after hitting this command as below:
PS C:\Yashaswini Suryawanshi\Development\Projects> pip install cv2
ERROR: Could not find a version that satisfies the requirement cv2 (from versions: none)
ERROR: No matching distribution found for cv2
WARNING: You are using pip version 22.0.4; however, version 22.1.2 is available.
You should consider upgrading via the 'C:\Users\Yashaswini suryawanshi\AppData\Local\Microsoft\WindowsApps\PythonSoftwareFoundation.Python.3.10_qbz5n2kfra8p0\python.exe -m pip install --upgrade pip' command.
PS C:\Yashaswini Suryawanshi\Development\Projects> pip install --upgrade pip
Requirement already satisfied: pip in c:\program files\windowsapps\pythonsoftwarefoundation.python.3.10_3.10.1520.0_x64__qbz5n2kfra8p0\lib\site-packages (22.0.4)
Collecting pip
Downloading pip-22.1.2-py3-none-any.whl (2.1 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 2.1/2.1 MB 9.7 MB/s eta 0:00:00
Installing collected packages: pip
WARNING: The scripts pip.exe, pip3.10.exe and pip3.exe are installed in 'C:\Users\Yashaswini suryawanshi\AppData\Local\Packages\PythonSoftwareFoundation.Python.3.10_qbz5n2kfra8p0\LocalCache\local-packages\Python310\Scripts' which is not on PATH.
Consider adding this directory to PATH or, if you prefer to suppress this warning, use --no-warn-script-location.
Successfully installed pip-22.1.2
WARNING: You are using pip version 22.0.4; however, version 22.1.2 is available.
You should consider upgrading via the 'C:\Users\Yashaswini suryawanshi\AppData\Local\Microsoft\WindowsApps\PythonSoftwareFoundation.Python.3.10_qbz5n2kfra8p0\python.exe -m pip install --upgrade pip' command.
PS C:\Yashaswini Suryawanshi\Development\Projects> pip install cv2
ERROR: Could not find a version that satisfies the requirement cv2 (from versions: none)
ERROR: No matching distribution found for cv2
So to solve this problem you can go ahead with following command:
pip3 install opencv-python
PS C:\Yashaswini Suryawanshi\Development\Projects> pip3 install opencv-python
Collecting opencv-python
Downloading opencv_python-4.6.0.66-cp36-abi3-win_amd64.whl (35.6 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 35.6/35.6 MB 10.4 MB/s eta 0:00:00
Collecting numpy>=1.17.3
Downloading numpy-1.23.1-cp310-cp310-win_amd64.whl (14.6 MB)
━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━━ 14.6/14.6 MB 16.8 MB/s eta 0:00:00
Installing collected packages: numpy, opencv-python
WARNING: The script f2py.exe is installed in 'C:\Users\y.y.suryawanshi\AppData\Local\Packages\PythonSoftwareFoundation.Python.3.10_qbz5n2kfra8p0\LocalCache\local-packages\Python310\Scripts' which is not on PATH.
Consider adding this directory to PATH or, if you prefer to suppress this warning, use --no-warn-script-location.
Successfully installed numpy-1.23.1 opencv-python-4.6.0.66
Now we are good to continue our code writting.
QR code can be decoded using detectAndDecode function of QRCodeDetector object of OpenCV.
The detectAndDecode function returns the content of the QR code, coordinates of the corners of the box and binarized QR code.
- You can refer OpenCV doc
- for more information on reading QR code using OpenCV.
img1=cv2.imread("QrCodeImage.png")
det=cv2.QRCodeDetector()
val, pts, st_code=det.detectAndDecode(img1)
print(val)
Output:
https://www.google.com
Subscribe to my newsletter
Read articles from Yashaswini Suryawanshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
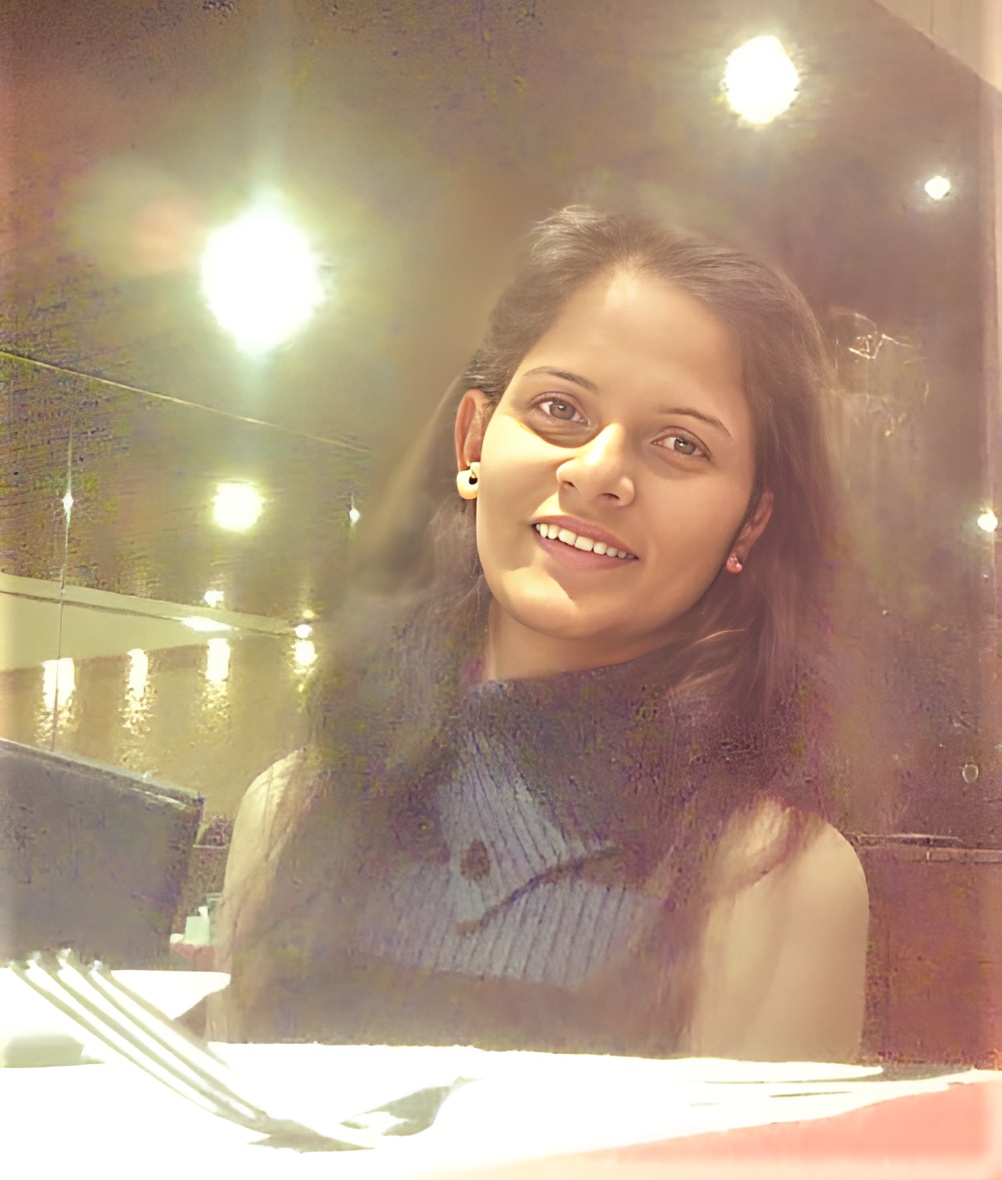