Hide the entity/model class field value in SpringBoot app
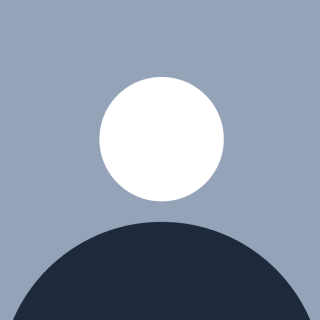
Table of contents
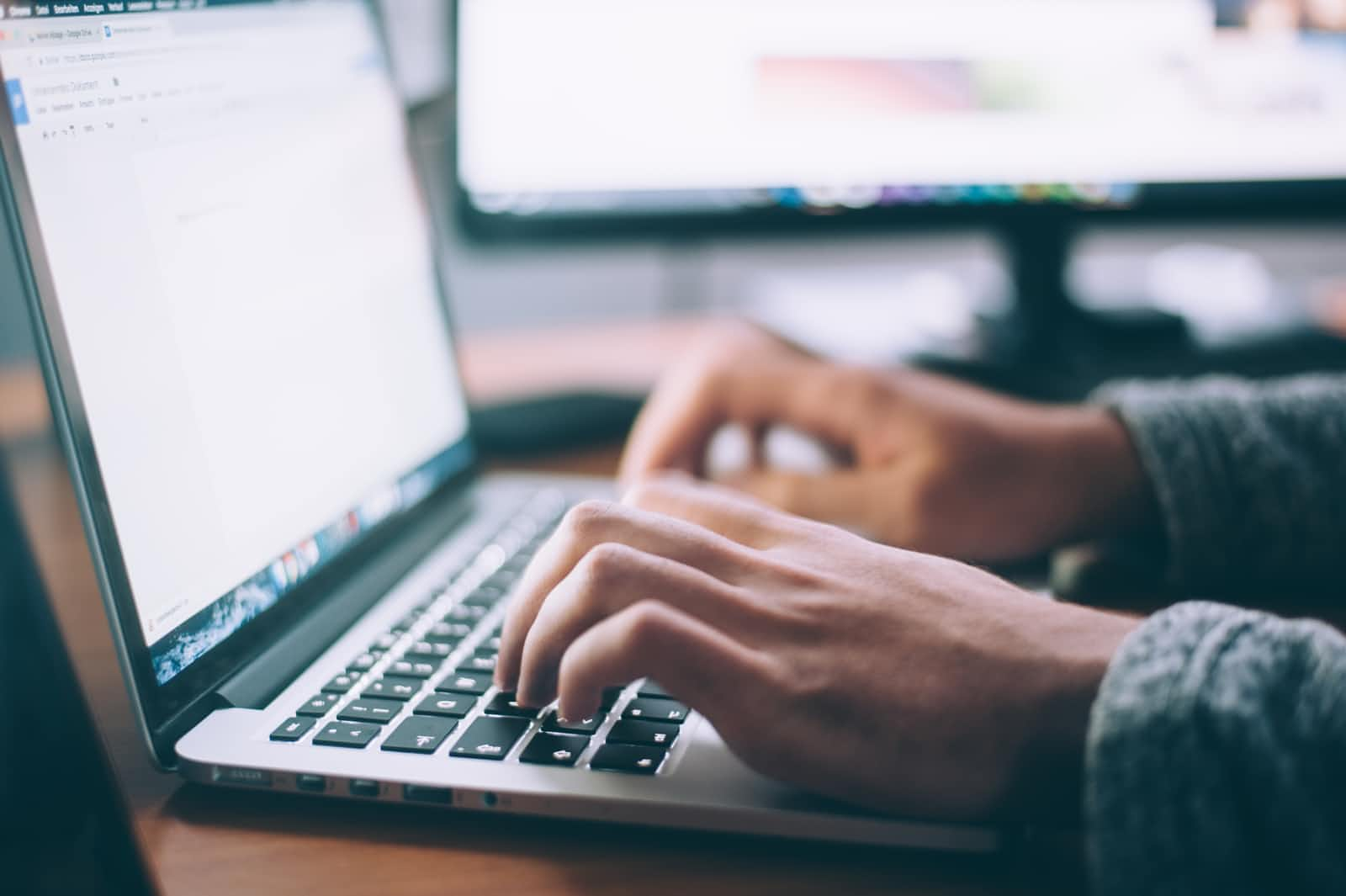
Introduction: It's easy to hide the field value from the REST API call using the annotation JsonIgnore, all you need is the field that you want to hide the variable/field. Let's take an example UserCredentials java class below:
package com.example.restful.webservice.demorestservice.credentials;
import com.fasterxml.jackson.annotation.JsonIgnore;
public class UserCredentials {
private int id;
@JsonIgnore
private String password;
@JsonIgnore
private String securityQuestion1;
@JsonIgnore
private String securityQuestion2;
@JsonIgnore
private String securityQuestion3;
public UserCredentials(int id, String password, String securityQuestion1, String securityQuestion2, String securityQuestion3) {
this.id = id;
this.password = password;
this.securityQuestion1 = securityQuestion1;
this.securityQuestion2 = securityQuestion2;
this.securityQuestion3 = securityQuestion3;
}
public String getSecurityQuestion1() {
return securityQuestion1;
}
public String getSecurityQuestion2() {
return securityQuestion2;
}
public String getSecurityQuestion3() {
return securityQuestion3;
}
public void setId(int id) {
this.id = id;
}
public int getId() {
return id;
}
public void setPassword(String password) {
this.password = password;
}
public String getPassword() {
return password;
}
}
Steps for implementing the JsonIgnore annotation: Add the JsonIgnore annotation to the field/variable that you want to implement as shown below code snippet
@JsonIgnore
private String password;
@JsonIgnore
private String securityQuestion1;
@JsonIgnore
private String securityQuestion2;
@JsonIgnore
private String securityQuestion3;
controller class that has only GET http method look like below:
@GetMapping("/user-credentials-info")
private UserCredentials userCredentials() {
return new UserCredentials(12345, "something", "what's your birth city", "what's your mother's maiden name", "what's your first job role");
}
hit your url that you have configured for your application controller class REST call method, in this case mine is localhost:8080/user-credentials-info
and the as expected the only field that doesn't have the @JsonIgnore
annotation not being added should come as the response as shown below:
Remove the @JsonIgnore
annotation to see the actual results:
private int id;
private String password;
private String securityQuestion1;
private String securityQuestion2;
private String securityQuestion3;
hit the url localhost:8080/user-credentials-info
. As expected the field value should come back as the argument value that is being passed.
Subscribe to my newsletter
Read articles from Sandeep Beegudem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Sandeep Beegudem
Sandeep Beegudem
I am a Java Software Engineer from San Antonio, Texas.