CSS Position
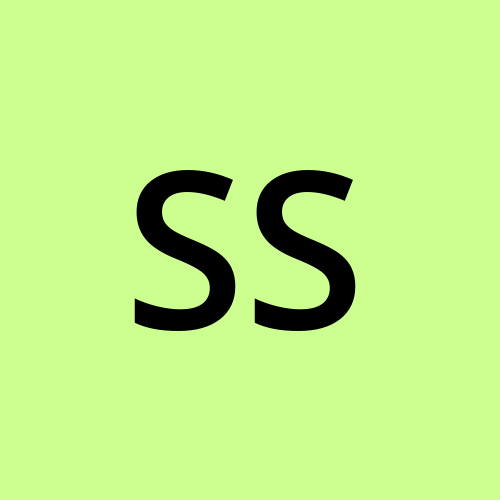
Table of contents
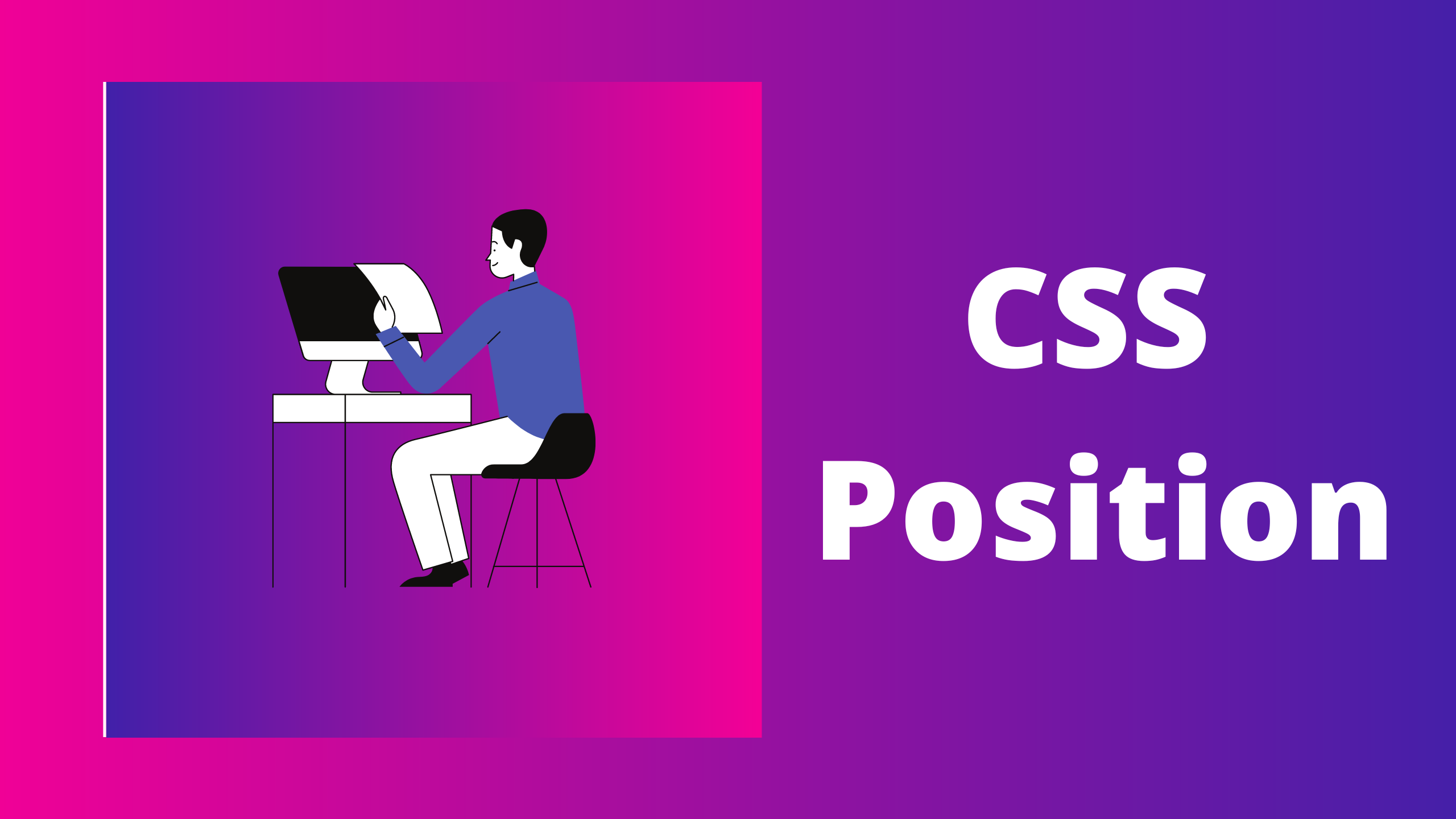
The css position property tells us how to position html elements in a web page. Position in a web page means top, right, bottom and left. Types of css positions are:
- Static
- Relative
- Absolute
- Fixed
- Sticky
- z-index
We will also discuss the below trick later
Trick: how to use absolute + relative + transform translate (how to put them together to get things centered)
Note: We will use the same example throughout the article with few changes.
To understand the positions let's take an example:
Example 1: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>CSS Position</title>
</head>
<body>
<div class="container">
<h1>Hello guys</h1>
<h3>Welcome to the world of programming.</h3>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Rem quia error nesciunt possimus asperiores nostrum facilis reiciendis ad ex, dicta, eveniet alias quibusdam? Fuga beatae dolorum aut, quo vitae aspernatur consequatur eum eos temporibus molestias nemo, alias qui cum quaerat molestiae possimus nesciunt. Sit recusandae, esse voluptatem facilis culpa quam, laudantium, nulla sequi praesentium accusantium aut perspiciatis error. Ea consectetur minus, fugit earum vitae excepturi temporibus! Distinctio magni, tempore consectetur accusantium porro saepe inventore iure quae, alias quidem, totam incidunt temporibus quos explicabo itaque dignissimos deleniti autem repellat. Repellat, ipsa, molestiae distinctio tenetur qui eius a exercitationem perferendis, reprehenderit inventore dicta! Provident itaque ut excepturi omnis nesciunt explicabo veritatis et eligendi dolorum culpa! Aspernatur atque animi aliquam magni dolor obcaecati corporis molestiae dolorem tenetur soluta dolore, aut nulla aperiam rem nesciunt similique distinctio quod! Tempore dolores suscipit modi error voluptatibus eaque ullam repellat voluptate obcaecati maxime. Ab ea explicabo commodi iste amet soluta, praesentium tenetur voluptas quae sequi repellendus ut. Exercitationem vero, repudiandae nostrum quibusdam odit consequatur. Ratione consequatur facere quia nulla est! Assumenda molestias commodi saepe repellendus at facere quibusdam libero veniam officiis laudantium! Veritatis quaerat voluptas doloremque dolor repellendus iste aut tempore. Aliquid incidunt consequuntur veniam illo magni!</p>
</div>
<div class="box">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Consectetur obcaecati nisi qui non expedita reiciendis voluptatem debitis, voluptatum ex, facilis maxime dicta veritatis dolor saepe, sequi repellendus numquam culpa tempore exercitationem laborum! Voluptatem dolores hic itaque aliquam fugit reiciendis illum perspiciatis ut aspernatur animi tempora amet aliquid, ducimus veritatis eius voluptate eaque commodi quaerat repellendus molestias quae blanditiis, odio tenetur! Perspiciatis optio minima in necessitatibus nemo, eveniet, a et itaque architecto nobis voluptas eaque voluptatibus sit veniam aliquid cum nisi ut commodi consectetur esse deleniti quam ipsa omnis eum. Rem, necessitatibus, quos exercitationem optio omnis dolorum repellat incidunt et fugiat corrupti vero maiores neque tempore voluptate reprehenderit nisi accusantium rerum ratione ad perferendis cumque minus ducimus? Rem deserunt nam, provident sit minus quam laborum! Odio omnis necessitatibus eaque in, aliquid laboriosam consectetur, dolores velit corrupti asperiores magni. Tempore fugiat repudiandae velit esse, aliquid molestias sapiente provident recusandae id debitis doloribus odit inventore cupiditate tenetur earum nam amet aperiam? Pariatur iste ab, recusandae, deleniti in molestiae omnis, porro ipsam nisi aliquid facere? Natus soluta esse ipsum molestias asperiores, illum cupiditate, numquam eaque, ullam accusantium sed placeat deserunt nisi repellendus distinctio odio harum? Provident quaerat deleniti, itaque rem impedit perspiciatis corporis minus enim vitae. Porro repudiandae veritatis at repellendus exercitationem, reiciendis dolor unde hic minus non quos quisquam, libero eaque. Id quod vero iusto sapiente ipsum tenetur quis consequuntur corrupti libero nam sequi, ducimus neque consectetur ullam esse saepe, enim eligendi vel molestiae? Rem voluptatum, veritatis aspernatur sit soluta aliquam ipsum est id, expedita et eos excepturi quasi fuga totam. Veritatis sit nobis praesentium id dignissimos velit recusandae repellat aspernatur! Maiores neque consequatur illum, ab nisi asperiores, tenetur obcaecati molestiae nostrum odio sequi animi, minima numquam et veniam iste. Recusandae porro molestiae, repellat deserunt fugit reiciendis corrupti placeat, maxime, ducimus tempora dignissimos.
</div>
</body>
</html>
style.css
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
border: 8pxsolid blueviolet;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgrey;
}
Time for the output,
Using the above example, we will understand the position.
How does the flow of html's document?
In HTML document flow, block level elements are stacked one after the other, while in inline the elements are arranged horizontally.
1. Static
In this, the elements do not move from their original position.
Position of html elements is static by default. On your web page you can do right click-> inspect -> computed->show all->search for position.
see the output below,
Now I change the position of h1 in the above example1's style sheet as static.
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: static;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgrey;
}
Now go to the web page again check for position, there will be no change in output.
We can say in static the position of elements remain same. When give a static position, the elements of html work in their normal flow and do not change position on the webpage, that you can see in above output.
2. Relative
If we give relative position to any element of html then it can come out from its normal flow and move left, right, top and bottom on the web page. It moves from its original position. Let's understand this by an example,
Example 2: In above Example1 , I changed the position of h1 as relative so now I can use left, right, top, bottom with this.
Let's try for the top position.
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: relative;
top:60px;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgrey;
}
Time for the output,
Now h1 has moved 60px from its top.
Let's try for the left position,
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: relative;
left:60px;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgrey;
}
Time for the output,
h1 has moved 60px from the left side to the right side.
Similarly you can try for bottom and right position.
3. Absolute
See the below example before explaining the absolute.
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: absolute;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
You will notice something has happened strange in the above output, let's understand it,
Let's talk about html's document flow, in above output by default its flow is from top to bottom because I have block elements in above example.
Note: For Inline vs Block level elements search on the internet by yourself.
When I used the relative position, my h1 element was inside the document flow. But as I will change its position from relative to absolute so now the h1 element will be outside the document flow.
You can see in above output after doing absolute position, the h1 element has gone out of document flow.
Now you can position it anywhere on the whole page. It will consider the whole page, not its original position.
For example if I take top: 0px it will behave like below,
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: absolute;
top: 0px;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
If we give right: 0px so it will behave like below,
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: absolute;
top: 0px;
right: 0px;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
In absolute position element will come out from its document flow to viewport. We can give it to position top, left, right and bottom on anywhere on the entire webpage.
Trick: Now we will discuss about this trick.
absolute + relative + transform translate (how to put them together to get things centered)
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>CSS Position</title>
</head>
<body>
<div class="container">
<h1>Hello guys</h1>
</div>
<div class="box">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Consectetur obcaecati nisi qui non expedita reiciendis voluptatem debitis, voluptatum ex, facilis maxime dicta veritatis dolor saepe, sequi repellendus numquam culpa tempore exercitationem laborum! Voluptatem dolores hic itaque aliquam fugit reiciendis illum perspiciatis ut aspernatur animi tempora amet aliquid, ducimus veritatis eius voluptate eaque commodi quaerat repellendus molestias quae blanditiis, odio tenetur! Perspiciatis optio minima in necessitatibus nemo, eveniet, a et itaque architecto nobis voluptas eaque voluptatibus sit veniam aliquid cum nisi ut commodi consectetur esse deleniti quam ipsa omnis eum. Rem, necessitatibus, quos exercitationem optio omnis dolorum repellat incidunt et fugiat corrupti vero maiores neque tempore voluptate reprehenderit nisi accusantium rerum ratione ad perferendis cumque minus ducimus? Rem deserunt nam, provident sit minus quam laborum! Odio omnis necessitatibus eaque in, aliquid laboriosam consectetur, dolores velit corrupti asperiores magni. Tempore fugiat repudiandae velit esse, aliquid molestias sapiente provident recusandae id debitis doloribus odit inventore cupiditate tenetur earum nam amet aperiam? Pariatur iste ab, recusandae, deleniti in molestiae omnis, porro ipsam nisi aliquid facere? Natus soluta esse ipsum molestias asperiores, illum cupiditate, numquam eaque, ullam accusantium sed placeat deserunt nisi repellendus distinctio odio harum? Provident quaerat deleniti, itaque rem impedit perspiciatis corporis minus enim vitae. Porro repudiandae veritatis at repellendus exercitationem, reiciendis dolor unde hic minus non quos quisquam, libero eaque. Id quod vero iusto sapiente ipsum tenetur quis consequuntur corrupti libero nam sequi, ducimus neque consectetur ullam esse saepe, enim eligendi vel molestiae? Rem voluptatum, veritatis aspernatur sit soluta aliquam ipsum est id, expedita et eos excepturi quasi fuga totam. Veritatis sit nobis praesentium id dignissimos velit recusandae repellat aspernatur! Maiores neque consequatur illum, ab nisi asperiores, tenetur obcaecati molestiae nostrum odio sequi animi, minima numquam et veniam iste. Recusandae porro molestiae, repellat deserunt fugit reiciendis corrupti placeat, maxime, ducimus tempora dignissimos.
</div>
</body>
</html>
style.css
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
height: 600px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: absolute;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
I have to bring h1 to the center in above example, for this there is a trick which is written above, h1 parent is container i.e div, give relative position to this container means parent has to give relative position, and the element which has to be placed in the center has to be given absolute position. In our case we will give the absolute position to h1.
When previously given the absolute position to h1 it was setting its position relative to the whole page. Now h1 will set its position relative to its parent. After this transform:translate() property will use to bring it to the center as given below.
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
position: relative;
width: 800px;
height: 300px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%,-50%);
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
4. Fixed
Let's say h3 content is content that you always want to keep on top like navbar, for this we will make it fixed position. In fixed position the element comes out of its normal document flow, and also we can use top, left, bottom and right value with this. In our example, h3 will come out of its normal document flow in the fixed position, now it will always be up, even if you scroll the page. If I give top: 0px it will behave like as below,
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
height: 500px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
}
.container h3{
background: black;
color: #ffffff;
position: fixed;
top: 0px;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
Remember: Sometimes it happens that when we use fixed position with an element ,the element dose not visible, it hides behind the other elements. Because we have given the value of z-index to the other elements. So in such a situation, which element is to be kept above what you have to do is to increase the z-index value of the element. In example I am giving it z-index=10; By doing this the element will always be on the top. The default value of z-index is auto(0 zero).
.container h1{
border: 4px solid green;
background: lightcoral;
}
.container h3{
background: black;
color: #ffffff;
position: fixed;
top: 0px;
z-index:10;
}
5. Sticky
In sticky position the element will remain in the normal document flow. By default this will work like relative position. Here I can use top, left, bottom and right value with this. When I give top: 0px; as you start scrolling the page and when it goes from top to 0px then it will be sticky.
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
height: 500px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
}
.container h3{
background: black;
color: #ffffff;
position: sticky;
top:0px;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
In above example h3's parent is div, after scrolling when you come out the div sticky effect will gone on the web page. At the time of using sticky position remember the element to whom you want to be sticky don't keep it inside any div, keep it separately outside like in below example,
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>CSS Position</title>
</head>
<body>
<div class="container">
<h1>Hello guys</h1>
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Rem quia error nesciunt possimus asperiores nostrum facilis reiciendis ad ex, dicta, eveniet alias quibusdam? Fuga beatae dolorum aut, quo vitae aspernatur consequatur eum eos temporibus molestias nemo, alias qui cum quaerat molestiae possimus nesciunt. Sit recusandae, esse voluptatem facilis culpa quam, laudantium, nulla sequi praesentium accusantium aut perspiciatis error. Ea consectetur minus, fugit earum vitae excepturi temporibus! Distinctio magni, tempore consectetur accusantium porro saepe inventore iure quae, alias quidem, totam incidunt temporibus quos explicabo itaque dignissimos deleniti autem repellat. Repellat, ipsa, molestiae distinctio tenetur qui eius a exercitationem perferendis, reprehenderit inventore dicta! Provident itaque ut excepturi omnis nesciunt explicabo veritatis et eligendi dolorum culpa! Aspernatur atque animi aliquam magni dolor obcaecati corporis molestiae dolorem tenetur soluta dolore, aut nulla aperiam rem nesciunt similique distinctio quod! Tempore dolores suscipit modi error voluptatibus eaque ullam repellat voluptate obcaecati maxime. Ab ea explicabo commodi iste amet soluta, praesentium tenetur voluptas quae sequi repellendus ut. Exercitationem vero, repudiandae nostrum quibusdam odit consequatur. Ratione consequatur facere quia nulla est! Assumenda molestias commodi saepe repellendus at facere quibusdam libero veniam officiis laudantium! Veritatis quaerat voluptas doloremque dolor repellendus iste aut tempore. Aliquid incidunt consequuntur veniam illo magni!</p>
</div>
<h3>Welcome to the world of programming.</h3>
<div class="box">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Consectetur obcaecati nisi qui non expedita reiciendis voluptatem debitis, voluptatum ex, facilis maxime dicta veritatis dolor saepe, sequi repellendus numquam culpa tempore exercitationem laborum! Voluptatem dolores hic itaque aliquam fugit reiciendis illum perspiciatis ut aspernatur animi tempora amet aliquid, ducimus veritatis eius voluptate eaque commodi quaerat repellendus molestias quae blanditiis, odio tenetur! Perspiciatis optio minima in necessitatibus nemo, eveniet, a et itaque architecto nobis voluptas eaque voluptatibus sit veniam aliquid cum nisi ut commodi consectetur esse deleniti quam ipsa omnis eum. Rem, necessitatibus, quos exercitationem optio omnis dolorum repellat incidunt et fugiat corrupti vero maiores neque tempore voluptate reprehenderit nisi accusantium rerum ratione ad perferendis cumque minus ducimus? Rem deserunt nam, provident sit minus quam laborum! Odio omnis necessitatibus eaque in, aliquid laboriosam consectetur, dolores velit corrupti asperiores magni. Tempore fugiat repudiandae velit esse, aliquid molestias sapiente provident recusandae id debitis doloribus odit inventore cupiditate tenetur earum nam amet aperiam? Pariatur iste ab, recusandae, deleniti in molestiae omnis, porro ipsam nisi aliquid facere? Natus soluta esse ipsum molestias asperiores, illum cupiditate, numquam eaque, ullam accusantium sed placeat deserunt nisi repellendus distinctio odio harum? Provident quaerat deleniti, itaque rem impedit perspiciatis corporis minus enim vitae. Porro repudiandae veritatis at repellendus exercitationem, reiciendis dolor unde hic minus non quos quisquam, libero eaque. Id quod vero iusto sapiente ipsum tenetur quis consequuntur corrupti libero nam sequi, ducimus neque consectetur ullam esse saepe, enim eligendi vel molestiae? Rem voluptatum, veritatis aspernatur sit soluta aliquam ipsum est id, expedita et eos excepturi quasi fuga totam. Veritatis sit nobis praesentium id dignissimos velit recusandae repellat aspernatur! Maiores neque consequatur illum, ab nisi asperiores, tenetur obcaecati molestiae nostrum odio sequi animi, minima numquam et veniam iste. Recusandae porro molestiae, repellat deserunt fugit reiciendis corrupti placeat, maxime, ducimus tempora dignissimos.
</div>
<p>lorem500</p>
</body>
</html>
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
height: 500px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
}
h3{
background: black;
color: #ffffff;
position: sticky;
top:0px;
}
.container p{
border: 4px solid red;
background: lightgray
}
Time for the output,
6. z-index
z-index is a property. Each element of html having this property. Each element by default has z-index auto i.e zero.
if we move any element then it will come on top of other element.
Let's take an example,
In Exmple 1 do below changes in style.css file
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position: relative;
top: 160px;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
background: lightgrey;
}
Time for the output,
You can see h1 has come on paragraph element. If we give z-index: 0; property to h1 then output will be same as above.
If an element having higher z-index it will come on another element, as in above output.
For example I want to put h1 behind the paragraph element.
h1 tag having 0(zero) z-index by default, p tag also having 0(zero) z-index by default.
Example 6: In Exmple 1 do below changes in style.css file
css
body{
font-family: Arial,Helvetica,sans-serif;
}
.container{
width: 800px;
border: 8px solid blue;
margin-top: 50px;
margin-bottom: 50px;
}
.container h1{
border: 4px solid green;
background: lightcoral;
position:relative;
top: 160px;
z-index: -1;
}
.container h3{
background: black;
color: #ffffff;
}
.container p{
border: 4px solid red;
/* background is transparent */
background: rgb(211, 211, 211,0.286);
}
Time for the output,
Now you can see h1 tag went behind p tag, because we have given -1 value to z-index.
That’s all for now. I hope this article clears your concepts of CSS position.
Next in this series, I’ll be writing on CSS Selectors.
If you liked this article, consider following me.
```
Subscribe to my newsletter
Read articles from shumaila sami directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
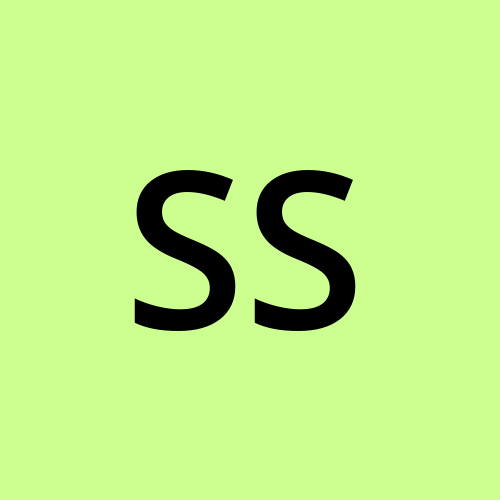