Burst Particles at Runtime with Unity
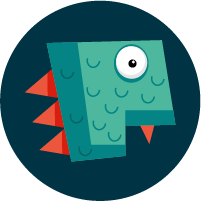
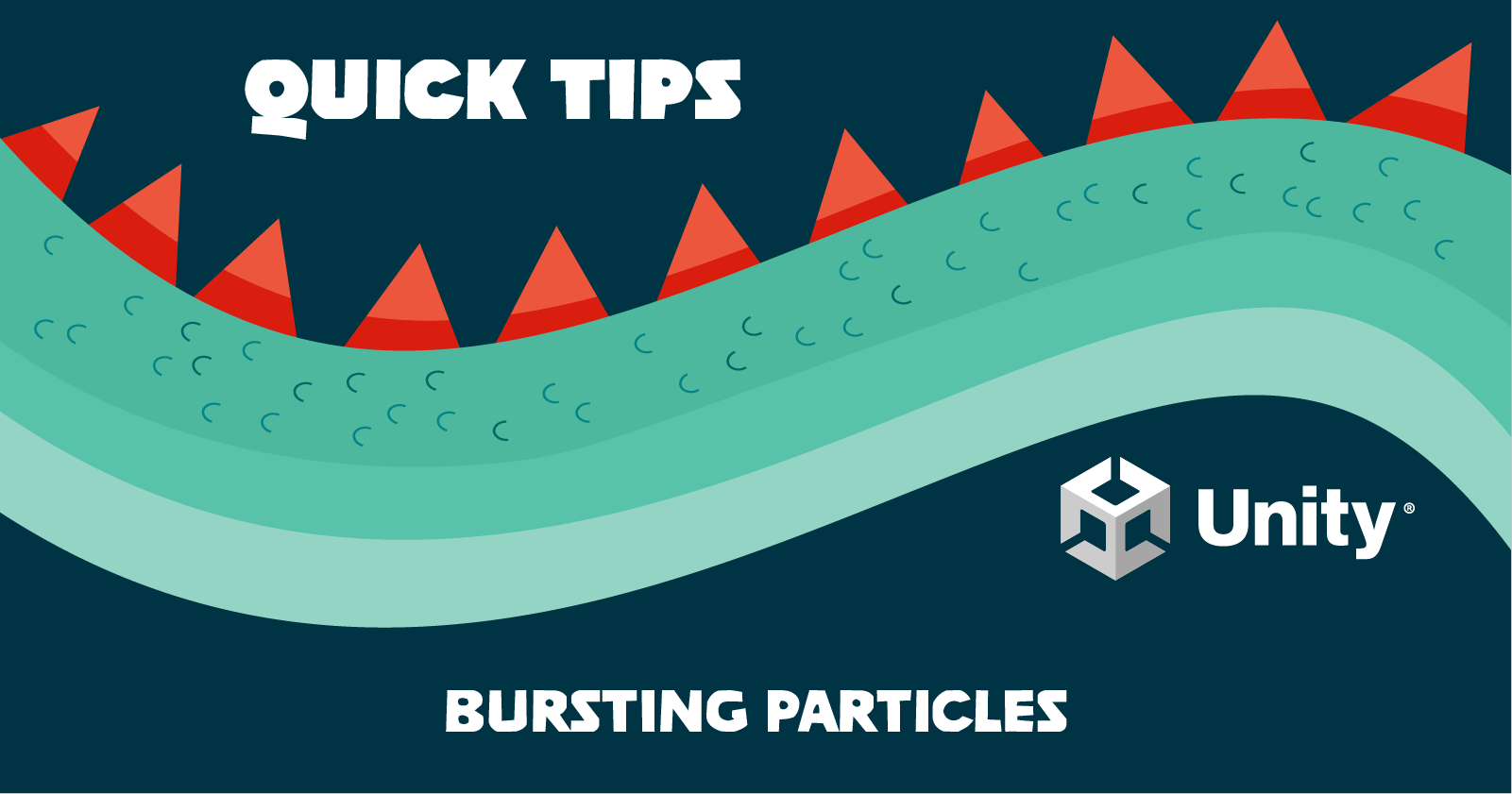
Problem:
I want to emit a specific number of particles from a ParticleSystem at runtime. For example, in the game CatBoarder, for every spin the player completed during a jump, I wanted to emit a particle for each.
Solution
- Unity 2020+
Add a ParticleSystem to the GameObject that will be emitting particles. Uncheck Looping and Play on Awake.
Under Emissions, configure Bursts to only emit one particle.
Add the code below as a component, then call Emit
with the number of particles to emit.
public class BurstEffect: MonoBehaviour
{
private ParticleSystem _particles;
private ParticleSystem.Burst _burst;
private void Awake()
{
_particles = GetComponent<ParticleSystem>();
_burst = _particles.emission.GetBurst(0);
}
public void Emit(int score)
{
_burst.count = score;
_particles.emission.SetBurst(0, _burst);
_particles.Play();
}
}
The results... a happy cat after 2 spins!
Further Reading:
- https://docs.unity3d.com/Manual/PartSysEmissionModule.html
Subscribe to my newsletter
Read articles from Steve Mcilwain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
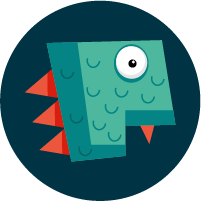
Steve Mcilwain
Steve Mcilwain
Indie Game Studio Owner | Game Designer | Game Developer | Game Artist | Cloud Architect | Technology Leader | Product Manager | Entrepreneur