TypeScript project: How does a bank calculate the interest rate?

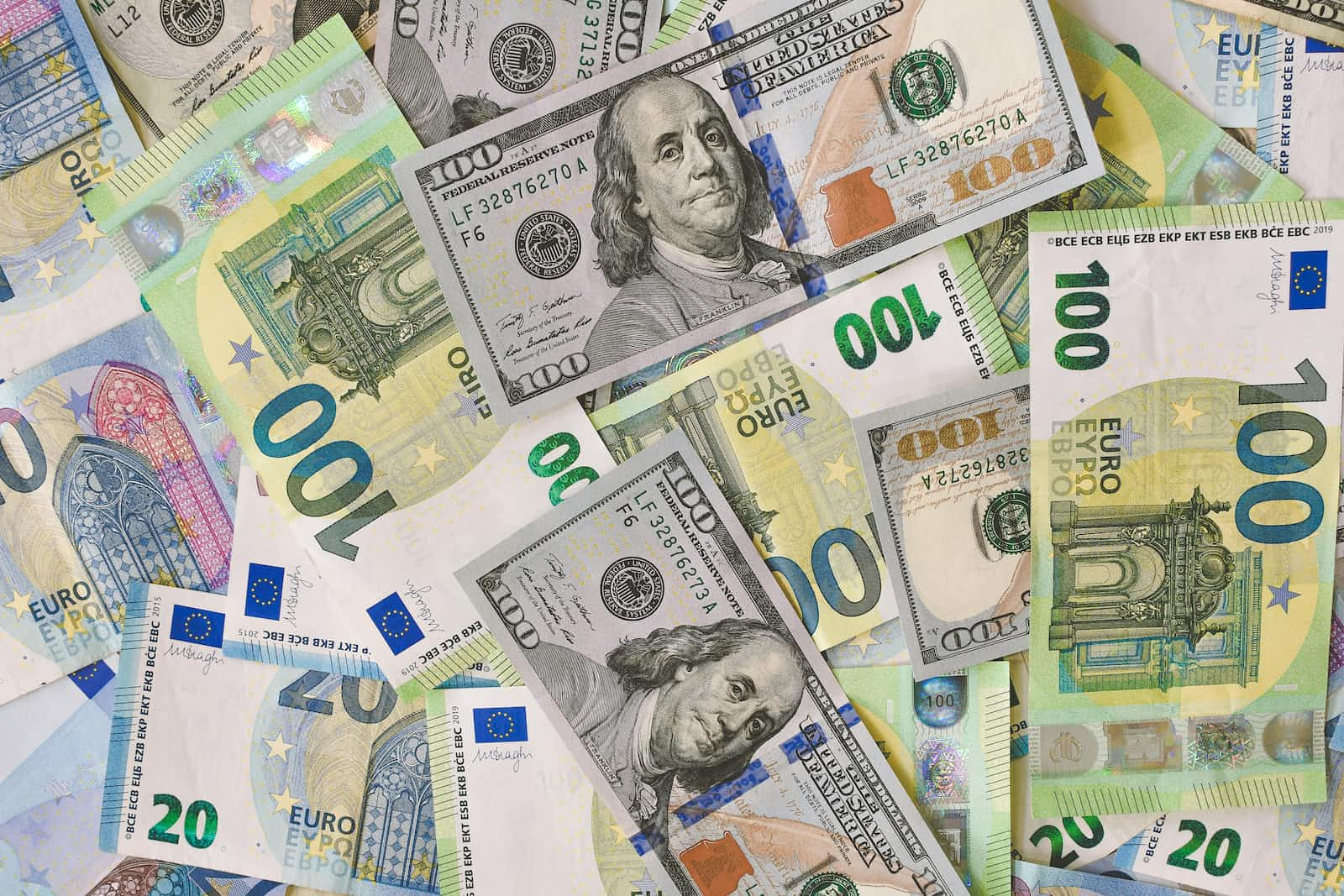
After reading the book Principles: Life & Work by Ray Dalio I wanted to translate my Financial math problem into computer code. In this post, I share my approach of converting a most common financial math problem into a computer code with TypeScript language.
Table of contents
Simple interest
Simple interest means you earn interest only on the principal amount of money.
Compound interest
To put it simply, compounding means when your earning interest will also earn interest over time. If you want to learn more about compound interest you can read this post.
Explanations
Selecting HTML form element and input elements with the data attribute.
const FORM = document.querySelector(
'[data-time="bank-form"]'
)! as HTMLFormElement;
const DOM = {
totalAmount: document.querySelector(
'[data-time="total-amount"]'
) as HTMLInputElement,
interest: document.querySelector(
'[data-time="interest"]'
) as HTMLInputElement,
year: document.querySelector('[data-time="year"]') as HTMLInputElement,
compound: document.querySelector(
'[data-time="compound-frequency"]'
) as HTMLInputElement,
renderContainer: document.querySelector(
'[data-time="render"]'
) as HTMLElement
};
Firstly, preventing the form from submissions with preventDefault(). Secondly, retrieving input field value. Thirdly, instantiating BankCalculation class. Finally, if the user forgets to fill out any of the input boxes then show an alert, otherwise use myFutureAmount method of BankCalculation class.
FORM.addEventListener("submit", (event) => {
event.preventDefault();
const total_amount: string = DOM.totalAmount.value;
const interest: number = Number(DOM.interest.value);
const year: number = Number(DOM.year.value);
const compound: number = Number(DOM.compound.value);
const bankCalculation = new BankCalculation(
total_amount,
year,
interest,
compound
);
if (total_amount !== "" && interest !== 0 && year !== 0) {
bankCalculation.myFutureAmount(DOM.renderContainer);
} else {
alert("Fill out all the input box.");
}
});
Creating BankCalculation class with four initial properties: "amount", "year", "interest", and "compound". Basically, whenever we try to create an instance from the class we must provide these four properties as arguments.
class BankCalculation {
amount: string;
year: number;
interest: number;
compound: number;
constructor(
amount: string,
year: number,
interest: number,
compound: number
) {
this.amount = amount;
this.year = year;
this.interest = interest / 100;
this.compound = compound;
}
}
Returning the formula (Deposit amount (1+i)^n or Deposit amount (1+i/m)^nm) based on compound interest.
private getTotalInterestFormulaUI(): string {
return `${this.getInterestFormulaUI()}${this.getYearFormulaUI()}`;
}
protected getInterestFormulaUI() {
if (this.isCompound()) {
return "(1+i/m)";
}
return "(1+i)";
}
protected getYearFormulaUI() {
if (this.isCompound()) {
return `<sup>nm</sup>`;
}
return `<sup>n</sup>`;
}
Placing actual value of the formula.
private getTotalInterestValueUI(): string {
return `${this.getInterestValueUI()}${this.getYearValueUI()}`;
}
protected getInterestValueUI() {
if (this.isCompound()) {
return `(1+${this.interest}/${this.compound})`;
}
return `(1+${this.interest})`;
}
protected getYearValueUI() {
if (this.isCompound()) {
return `<sup>${this.year}×${this.compound}</sup>`;
}
return `<sup>${this.year}</sup>`;
}
Use the compound formula if the bank provides compound interest, otherwise use the simple formula and return interest value.
protected getCalcInterest(): number {
let interest: number = 1 + this.interest;
if (this.isCompound()) {
interest = 1 + this.interest / this.compound;
}
return Number(interest.toFixed(5));
}
Calculate the interest rate according to the number of years you are going to deposit your money in the bank. If the bank provides compound interest use the compound formula, otherwise use the simple formula.
protected getCalcInterestWithPower(): number {
let year: number = this.year;
const interest: number = this.getCalcInterest();
let calcPower: number = interest ** year;
if (this.isCompound()) {
year = year * this.compound;
calcPower = interest ** year;
}
return Number(calcPower.toFixed(5));
}
If the compound value is greater than 1, return true, otherwise, return false.
protected isCompound(): boolean {
return this.compound > 1 ? true : false;
}
Calling functions
myFutureAmount(renderArea: HTMLElement): void {
const totalInterestUI: string = `${this.getTotalInterestFormulaUI()}`;
const formula = `${this.baseTemplate(
`Principal amount ${totalInterestUI}`
)}`;
const setValue = `${this.baseTemplate(
`${this.amount} ${this.getTotalInterestValueUI()}`
)}`;
const totalInterest: number = this.getCalcInterestWithPower();
const answerUI = `${this.amount} × ${totalInterest}`;
const answer = Math.round(
Number(this.amount.replaceAll(",", "")) * totalInterest
);
this.renderTemplate(
`${formula}${setValue}`,
answerUI,
`$${answer.toLocaleString("en-US")}`,
renderArea
);
}
Rendering formula, calculation, and answer inside the renderArea element.
protected baseTemplate(value: string): string {
return `<div>${value}</div>`;
}
protected renderTemplate(
formula: string,
calculation: string,
answer: string,
renderArea: HTMLElement
) {
renderArea.innerHTML = `
<p class="mb-0">We know,</p>
<div class="pl-4">
${formula}
${this.baseTemplate(calculation)}
${this.baseTemplate(answer)}
</div>`;
}
Simple version.
Final project.
Subscribe to my newsletter
Read articles from ITS MAC directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ITS MAC
ITS MAC
He is a web developer. He develops websites using a mobile-first design approach that is fast, user-friendly, and mobile-optimized. He loves JavaScript, React, Python, and kind of tolerates React with TypeScript, but he is happy to use whatever tool is most suited for the job at hand. He is also interested in Server-Side Rendering (SSR) or Single-Page Applications (SPA). Currently, he is discovering Nextjs for SSR/SPA.