How to remove specific item from array by value in Javascript?
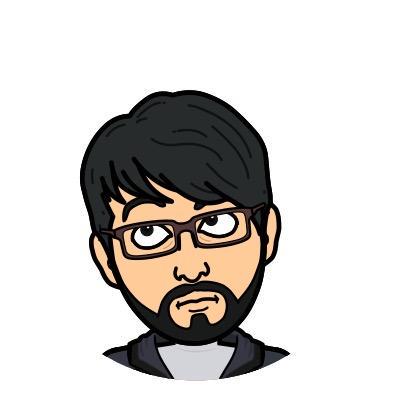
Find the index of the array element you want to remove using indexOf, and then remove that index with splice.
The splice() method changes the contents of an array by removing existing elements and/or adding new elements.
const array = [2, 5, 9];
console.log(array);
const index = array.indexOf(5);
if (index > -1) { // only splice array when item is found
array.splice(index, 1); // 2nd parameter means remove one item only
}
// array = [2, 9]
console.log(array);
The second parameter of splice is the number of elements to remove. Note that splice modifies the array in place and returns a new array containing the elements that have been removed.
Below I'm providing functions which you can easily plug into your code and keep it clean. The first function removes only a single occurrence (i.e. removing the first match of 5 from [2,5,9,1,5,8,5]), while the second function removes all occurrences:
function removeOne(arr, value) {
var index = arr.indexOf(value);
if (index > -1) {
arr.splice(index, 1);
}
return arr;
}
function removeAll(arr, value) {
var i = 0;
while (i < arr.length) {
if (arr[i] === value) {
arr.splice(i, 1);
} else {
++i;
}
}
return arr;
}
// Usage
console.log(removeOne([2,5,9,1,5,8,5], 5))
console.log(removeAll([2,5,9,1,5,8,5], 5))
For those looking for ECMAScript 6 code, I got your back
let value = 3
let arr = [1, 2, 3, 4, 5, 3]
arr = arr.filter(item => item !== value)
console.log(arr)
// [ 1, 2, 4, 5 ]
IMPORTANT ECMAScript 6 () => {} arrow function syntax is not supported in Internet Explorer at all, Chrome before version 45, Firefox before version 22, and Safari before version 10. To use ECMAScript 6 syntax in old browsers you can use BabelJS.
Subscribe to my newsletter
Read articles from Vajid Kagdi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
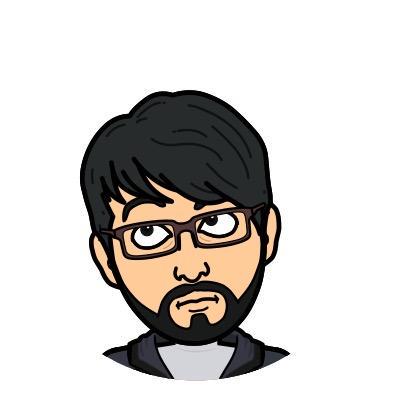
Vajid Kagdi
Vajid Kagdi
Graduated with a Master’s degree in Software Engineering from San Jose State University in December 2019 and currently working at PayPal on the Customer Journey Platform. I enjoy generating new ideas and devising feasible solutions to broadly relevant problems. My colleagues would describe me as a driven, resourceful individual who maintains a positive, proactive attitude when faced with adversity. Interested to work in areas related to Distributed Systems, Cloud Infrastructure and Micro-Services. I get a lot of satisfaction from the constant learning and puzzle solving that comes with my profession and contributing to a successful and worthwhile product. My expertise includes project design and management, data analysis and interpretation, and the development and implementation of software products.