Have A Ride With Some Concept Of Python
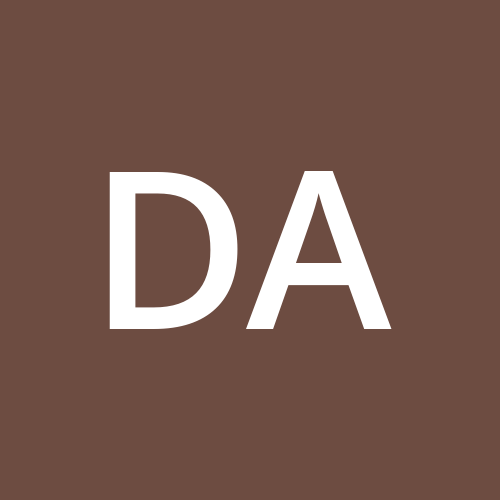
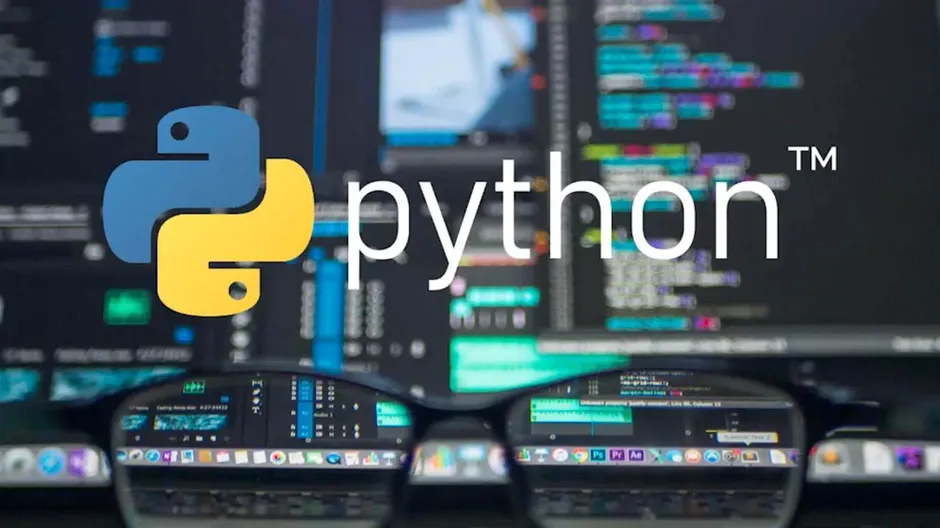
Welcome to my first article in my first series ever : "Python"
In this article , I'll be introducing you a few Python concept!😊
If you have just started learning python or you are thinking of doing so,you can start learning without worrying , as soon you will realize that it is very different programming language.
Unlike other programming languages such as C,C++,JAVA.Python is high-level,interpreted language,this means that instead of compiling your code to bytecode and then loading it,a Python interpreter reads your source code and execute it directly.
this article will help you understand what variables and expression are in context of coding.
Strap in ,folks. Keep all hands, feet, arms, legs inside the ride. We're about to learn some Python 😎 Let's get started!
Variables In Python
Python variables is container with the name to storing data. It is the place where you can store data for use at a later stage in your program.A variable is a label that you can assign value to it. For Example: See below ⬇️
message = 'Hello World'
print(message)
Output:
Hello World
in this example, message is variable it holds the string 'Hello World' . The print () function shows the message Hello World to the screen
Declaring and assigning values to variables is super easy in Python! Here's an example below ⬇️
website='myntra.com'
print(website)
Output:
myntra.com
I assigned a value myntra.com to the variable website. Then, I printed out the value assigned website i.e. myntra.com Now if you want to change the value of your variable, that is also super easy! Check it out⬇️
# assign initial value to website
website = 'myntra.com'
print(website)
# assigning a new value to website
website = 'udemy.com'
print(website)
Output:
udemy.com
But, what if you need to assign multiple values to multiple variables? Look below⬇️
a, b, c = 20, 12.2, 'Anime'
print (a)
print (b)
print (c)
Output:
20
12.2
Anime
You can also assign the same values to multiple variable!
a=b=c='Marvel'
print(a)
print(b)
print(c)
Output:
Marvel
Marvel
Marvel
Control Flow
There comes situations in real life when we need to make some decisions and based on these decisions, we decide what should we do next. Similarly in programming we need to make some decisions and based on these decisions we will execute the next block of code. Decision-making statements in programming languages decide the direction of the flow of program execution.
Two types of Control Flow in Python
1.Conditionals
2.Loops
1.Conditionals
If Statement
If statement is the most commonly used conditional statement in programming languages.it decides whether the certain statements need to be executed or not.it checks for a given condition, If the condition is true then, the set of code present inside the "if" block will be executed otherwise not.
The If condition evaluates a boolean expression and execute the block of code only when the boolean expression becomes TRUE. For Example See below⬇️
passing_Score = 60
my_Score = 67
if(my_Score >= passing_Score):
print('You passed the exam')
print('Congratulations')
Output:
You passed the exam
Congratulations
if-else Statement
If-else statement evaluates the Boolean expression. If the condition is TRUE then, the code present in the “ if “ block will be executed otherwise the code of the “else“ block will be executed. Check it out⬇️
passing_Score = 50
my_Score = 40
if(my_Score >= passing_Score):
print('Congratulations! You passed the exam')
print('You are passed in the exam')
else:
print('Sorry! You failed the exam, better luck next time')
Output:
Sorry! You failed the exam, better luck next time
elif Statement
we have one more conditional statement called "elif" statements. "elif" statement is used to check multiple conditions only if the given condition is false. It’s similar to an "if-else" statement and the only difference is that in "else" we will not check the condition but in "elif" we will check the condition.
"elif" statements are similar to "if-else” statements but "elif" statements evaluate multiple condition. For Example Look Below⬇️
num = -7
if (num > 0):
print('Number is positive')
elif (num < 0):
print('Number is negative')
else:
print('Number is Zero')
Output:
Number is negative
Nested If-else Statement
When an if a statement is present inside another if statement, it is called a nested IF statement. This situation occurs when you have to filter a variable multiple times. A nested if is an if statement that is the target of another if statement. Check it out⬇️
x = 50
if x > 20:
print('Above twenty')
if x > 40:
print('and also above 40')
else:
print('but not above 40')
Output:
Above twenty
and also above 40
2. Loops
Python programming language provides the following types of loops to handle looping requirements.Loops in Python allow us to execute a group of statements several times. Python provides three ways for executing the loops.
1. While Loop
while loop is used to execute a block of statements repeatedly until a given condition is satisfied. And when the condition becomes false, the line immediately after the loop in the program is executed. For Example See Below⬇️
count = 0
while (count < 3):
count = count + 1
print('Hello Friends')
Output:
Hello Friends
Hello Friends
Hello Friends
2. For Loop
Executes a sequence of statements multiple times and abbreviates the code that manages the loop variable. Here's an example⬇️
numbers = [6, 5, 3, 8, 4,]
sum = 0
for val in numbers:
sum = sum+val
print('The sum is', sum)
Output:
The sum is 26
3. Nested Loop
The Python programming language allows programmers to use one looping statement inside another looping statement.
Like so⬇️
rows = 6
for i in range(1, rows + 1):
for j in range(1, i + 1):
print("*", end=' ')
print('')
Output:
*
* *
* * *
* * * *
* * * * *
* * * * * *
That's it! You made it to the end! Thanks for reading! 😃
Subscribe to my newsletter
Read articles from Daniya Ahmad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
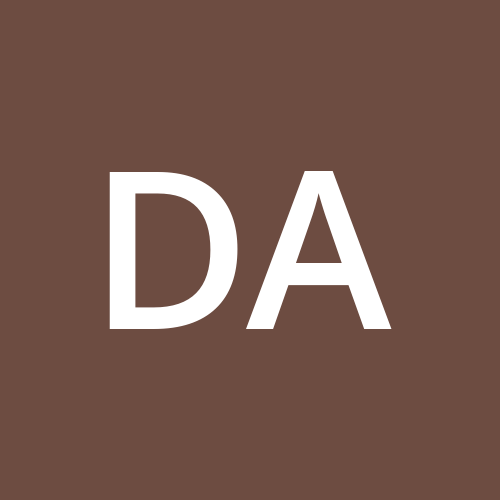