How to solve factorial problem using recursion?
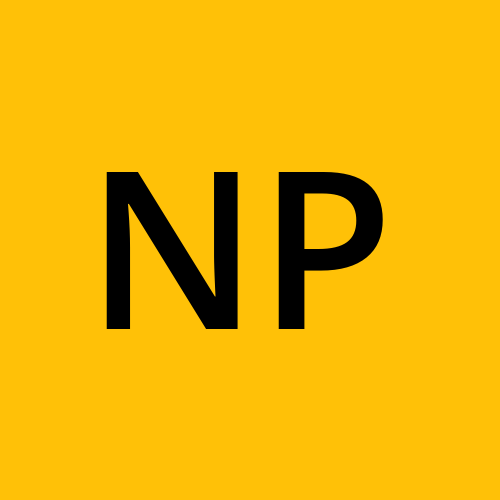
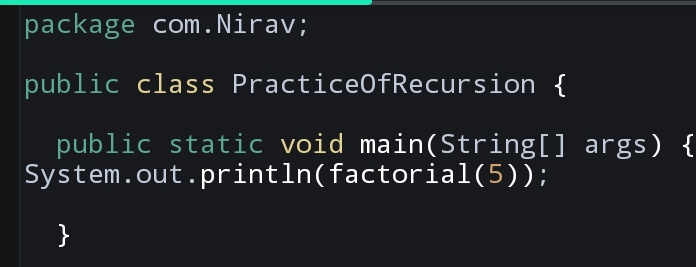
Hello developer's
Today i share my experience of solving factorial problem using recursion.
First of all what is recursion?
Recursion is a function which recall itself.
How to solve factorial problem using recursion?
First of all we try to divide this problem in small problems Like 3 ! = 3 2 1 We can break this in small problems 3 ! = 3 2 ! 2 ! = 2 1 ! Factorial of 1 is 1.
You can see here that what is common ?
First you can see that factorial of 3 is equal to 3 factorial of 2 Second thing is factorial of 2 is equal to 2 factorial of 1 So you can see that factorial of num is equal to num * factorial of num - 1
Now you find common thing now what condition we can add you should see that we are calculating factorial by reducing num so we can add that if num is equal to 1 than stop calling function again and again.
What are you passing in function ?
You are passing (num - 1)
Here is code
public class PracticeOfRecursion {
public static void main(String[] args) {
System.out.println(factorial(5));
}
static int factorial(int n){
// adding condition because at the end we want know that factorial of 1 is 1
if (n == 1){
return 1;
}
// returning function it self because the factorial is always be multiplication of 1 till n
return n * factorial(n - 1);
// this function is returning fuction it self with factorial of n - 1
//like if we want to get factorial of 3
// factorial of 3 = 3 * factorial of 2
// factorial of 2 = 2 * factorial of 1
// we know that factorial of is equal to 1 and also factorial of 1 means argument of function is 1 so it will hit our if codition and it that's finish because function have only one statement
}
}
Subscribe to my newsletter
Read articles from Nirav Prajapati directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
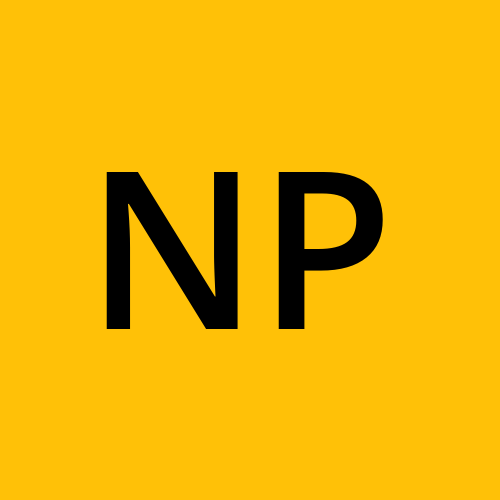
Nirav Prajapati
Nirav Prajapati
Hi there, My name is Nirav. I am from India. Currently I am learning Java and Dsa. I can code in Html, Css, Javascript, Python ⭐ My projects: https://pro-quiz-app.herokuapp.com https://guessnumberofficial.netlify.app ✨ GitHub: https://github.com/Niravprajapati1/