Scrimba's Gift Selector Challenge
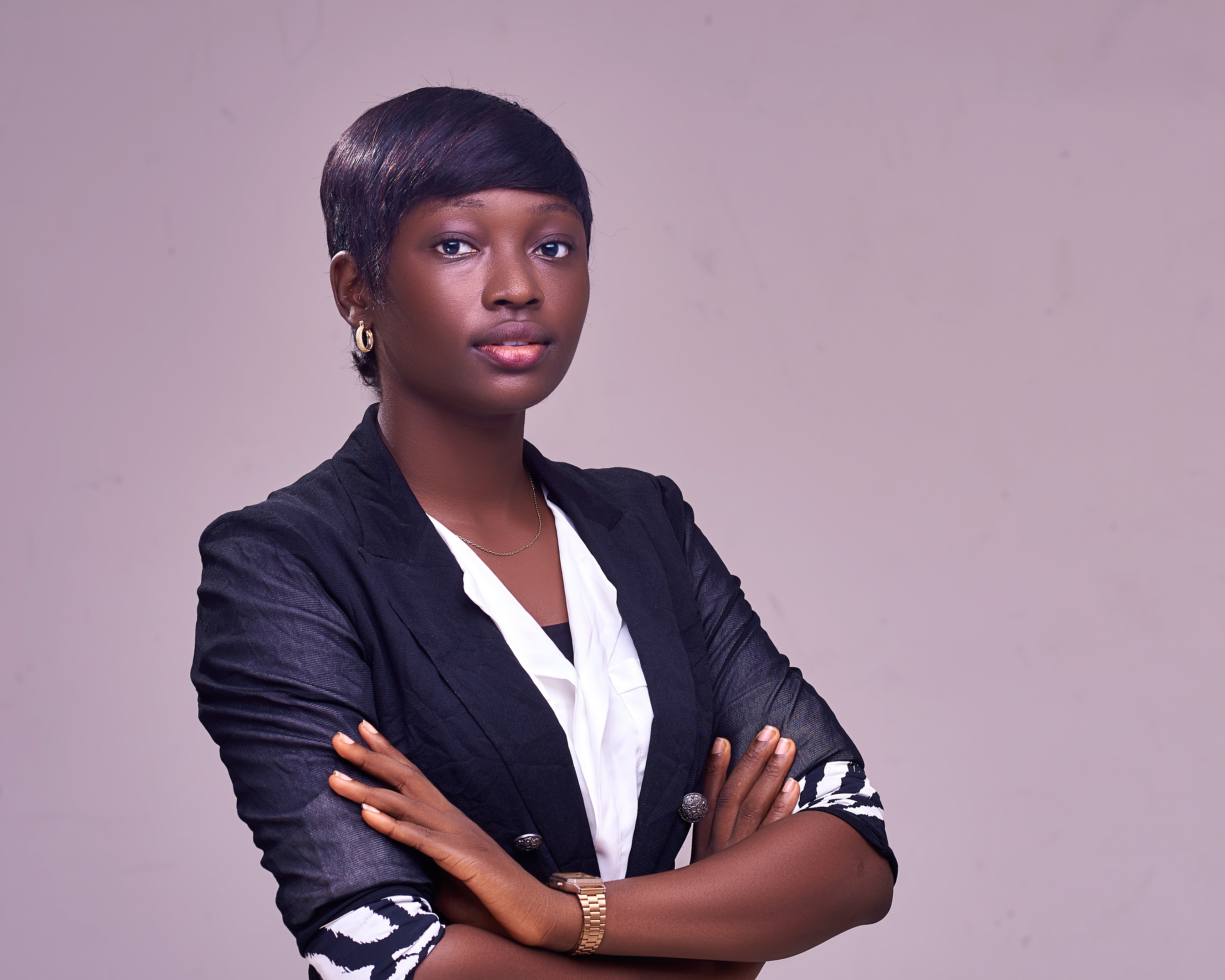
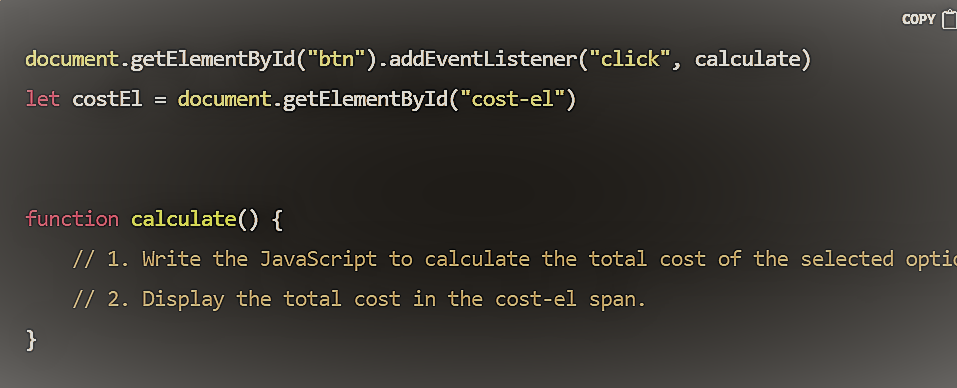
Following my quest to complete a 100daysOfCode challenge, I tried my hands on Scrimba's Gift Selector Challenge with basic approach. This solution is readable to beginners who are trying their hands on challenges to upscale the knowledge of javascript.
Following this step by step will give you a functioning gift selector as seen here.
First, get the starter files from this starter files for challenge to get the HTML and CSS files.
We will be exploring concepts like:
Getting the value attribute of an Option tag of a select element (You can check out MDN documentation of this).
Converting a string to a number using the parseInt function.
Displaying a mathematical output in an element.
In your script.js
you'll see a template made for you to input the solution:
document.getElementById("btn").addEventListener("click", calculate)
let costEl = document.getElementById("cost-el")
function calculate() {
// 1. Write the JavaScript to calculate the total cost of the selected options from the form.
// 2. Display the total cost in the cost-el span.
}
The challenge is to find the solution to the instructions in the comments.
To calculate the total cost, we will first retrieve the value of a selected option. To do this we will first get the elements either by class, Id or selector but we will be using ID SELECTORS for this solution. To get the element for one of the dropdown, we write
var getFoodValue = document.getElementById("food-select");
Next, we get the value from the element using
var foodValue = getFoodValue.options[getFoodValue.selectedIndex].value;
Remember to write this inside the calculate function already declared.
Here, we will see that there is a common word FOOD in these declarations because we are only on the food-select
part now.
At this point, if you console.log(foodValue)
you'll get the chosen output when you click on the calculate function. However, the output is a string which mathematical functions will not calculate as a number even if it is a number, it is of a string data type.
var foodValueToNumber = parseInt(foodValue);
The above declaration converts the value to a number or integer data type (to know more on data types).
Since we need the values of both dropdowns, repeat the same steps for the transport-select
ID. You should have this:
function calculate() {
// 1. Write the JavaScript to calculate the total cost of the selected options from the form.
// for food
var getFoodValue = document.getElementById("food-select");
var foodValue = getFoodValue.options[getFoodValue.selectedIndex].value;
var foodValueToNumber = parseInt(foodValue);
// for transport
var getTransportValue = document.getElementById("transport-select");
var transportValue = getTransportValue.options[getTransportValue.selectedIndex].value;
var transportValueToNumber = parseInt(transportValue);
}
If you've followed those steps and you get accurate results when you console.log
, then CONGRATULATIONS, You're almost set with your gift selector.
Now, to get the final result so as to conclude the first instruction,
// total gift cost
var totalCost = foodValueToNumber + transportValueToNumber;
The above will give the sum of both values, you can console.log(totalCost)
to be sure you're in sync.
Remember from the start, costEl
was declared
let costEl = document.getElementById("cost-el")
This is the output display area provided and to route your output to display on the given section add
// 2. Display the total cost in the cost-el span.
costEl.innerHTML = totalCost;
This will display the sum just after the paragraph
with content Total gift cost:
Hooray ๐๐ฅณ๐!! Your Gift Selector is good to go.
You can add more options to the given options and play around with the values.
If you have any issues, check here for the codebase of the complete solution.
CIAO!
Subscribe to my newsletter
Read articles from Anita Idemudia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
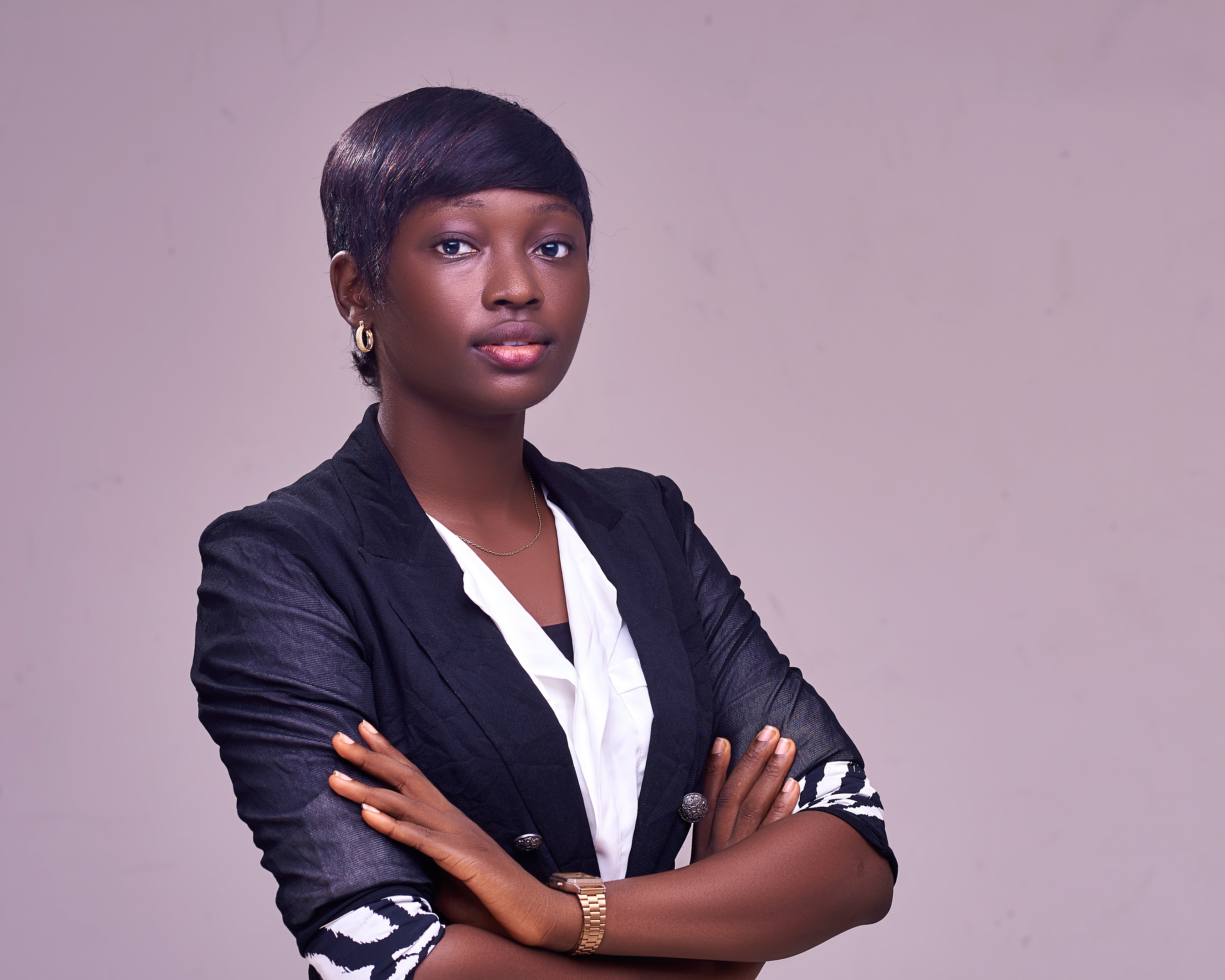
Anita Idemudia
Anita Idemudia
I'm a Frontend Engineer and Technical Content Writer. I'm passionate about gaining knowledge, documentation, and creating content.