How to create an animated sphere for your website

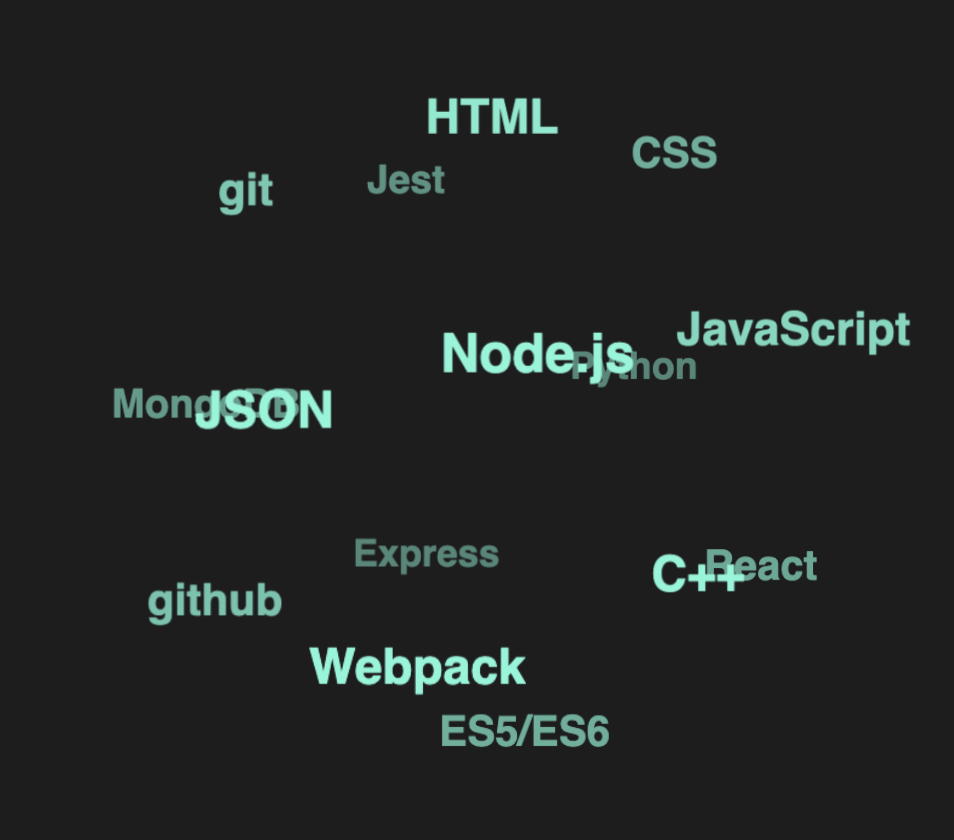
I've seen some websites using an animated sphere like the following one:
So I made my own research and I bring you a tutorial on how to create one of this spheres. You can implement it either using Vanilla JavaScript or using React.JS.
I want to clarify that I didn't write the code for this effect. I just implement it in my site. The original code is from TagCloud and was written by Cong Ming. Please visit and star his project on Github if you find it useful.
Now... let's get started.
Rotating sphere with vanilla JS and plain HTML
HTML
Create a container to hold the TagCloud
<div class="content"></div>
Import TagCloud.js script in the document
<script src="https://cdn.jsdelivr.net/npm/TagCloud@2.2.0/dist/TagCloud.min.js"></script>
And that's all about the HTML.
CSS
You can use Raw CSS to customize how the text in the sphere looks like:
.tagcloud {
font-family: 'Poppins', sans-serif;
font-size: 20px;
font-weight: 650;
margin-left: 30%;
}
.tagcloud--item:hover {
color: #36454F;
}
JavaScript
Define the tags you want to display. Use a JS array:
const myTags = [ 'JavaScript', 'CSS', 'HTML', 'C', 'C++', 'React', 'Python', 'Java', 'git', 'django', 'Node.js', 'OpenCV', 'GCP', 'MySQL', 'jQuery', ];
Render a default TagCloud :
var tagCloud = TagCloud('.content', myTags);
If you need to, config the TagCloud behavior this way:
var tagCloud = TagCloud('.content', myTags,{
// radius in px
radius: 300,
// animation speed
// slow, normal, fast
maxSpeed: 'fast',
initSpeed: 'fast',
// 0 = top
// 90 = left
// 135 = right-bottom
direction: 135,
// interact with cursor move on mouse out
keep: true
});
The result should be something like this:
Rotating sphere with React.JS.
You can use TagCloud by installing it into your project using any of the following options:
npm install TagCloud
- import directly from https://cdn.skypack.dev/TagCloud@2.2.0
In this tutorial we are going to do it using the direct link.
For HTML, you only need an element for the React app to render:
<div id="root"></div>
CSS
Again, you can customize your CSS the same way we did as in plain HTML.
@import url('https://fonts.googleapis.com/css2?family=Poppins&display=swap');
body {
background-color: #111827;
}
.tagcloud {
font-family: 'Poppins', sans-serif;
font-size: 20px;
margin: auto;
width: 50%;
color: #79f7d9;
}
.tagcloud--item:hover {
color: #e94152;
}
JavaScript.
You need to create your component and use the TagCloud inside a useEffect hook (since we only need the sphere to render once):
There is a problem when using React useEffect and React.StrictMode at the same time. Depending on your React version, useEffect will run twice (thus, generating two spheres). I think this happens in version 17 and above. Anyway, this double execution will only happen in development mode. Please read the doc in order to know more about why this happens.
However, if this is the case for you, and React.useEffect runs twice, you can avoid that behavior by using useRef hook.
This is how your componen should look like:
import React, { useRef } from "https://cdn.skypack.dev/react@17.0.1";
import ReactDOM from "https://cdn.skypack.dev/react-dom@17.0.1";
// If you install TagCloud via npm:
//import TagCloud from "TagCloud";
import TagCloud from "https://cdn.skypack.dev/TagCloud@2.2.0";
const App = () => {
const sphereMounted = useRef(false); // useRef hook: Avoids behavior where useeffect runs twice in development mode.
React.useEffect(() => {
if(sphereMounted.current === false){
// Again, define your tags
const myTags = [
'JavaScript', 'CSS', 'HTML',
'Node.js', 'C++', 'React',
'Python', 'Jest', 'git',
'JSON', 'Webpack', 'ES5/ES6',
'Express', 'MongoDB', 'github',
];
// Render a tagCloud with custom configuration
var tagCloud = TagCloud('.sphere', myTags,{
// radius in px
radius: 200,
// animation speed
// slow, normal, fast
maxSpeed: 'fast',
initSpeed: 'fast',
// 0 = top
// 90 = left
// 135 = right-bottom
direction: 135,
// interact with cursor move on mouse out
keep: true,
});
}
return () => sphereMounted.current = true; // useRef
}, []);
// This is what will render the tagcloud:
return(
<span className="sphere"></span>
);
}
ReactDOM.render(<App />,
document.getElementById("root"))
Result is this:
And that's all for today! Hope you find it useful.
Subscribe to my newsletter
Read articles from Miguel Hernández directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
