Median of Two Sorted Arrays
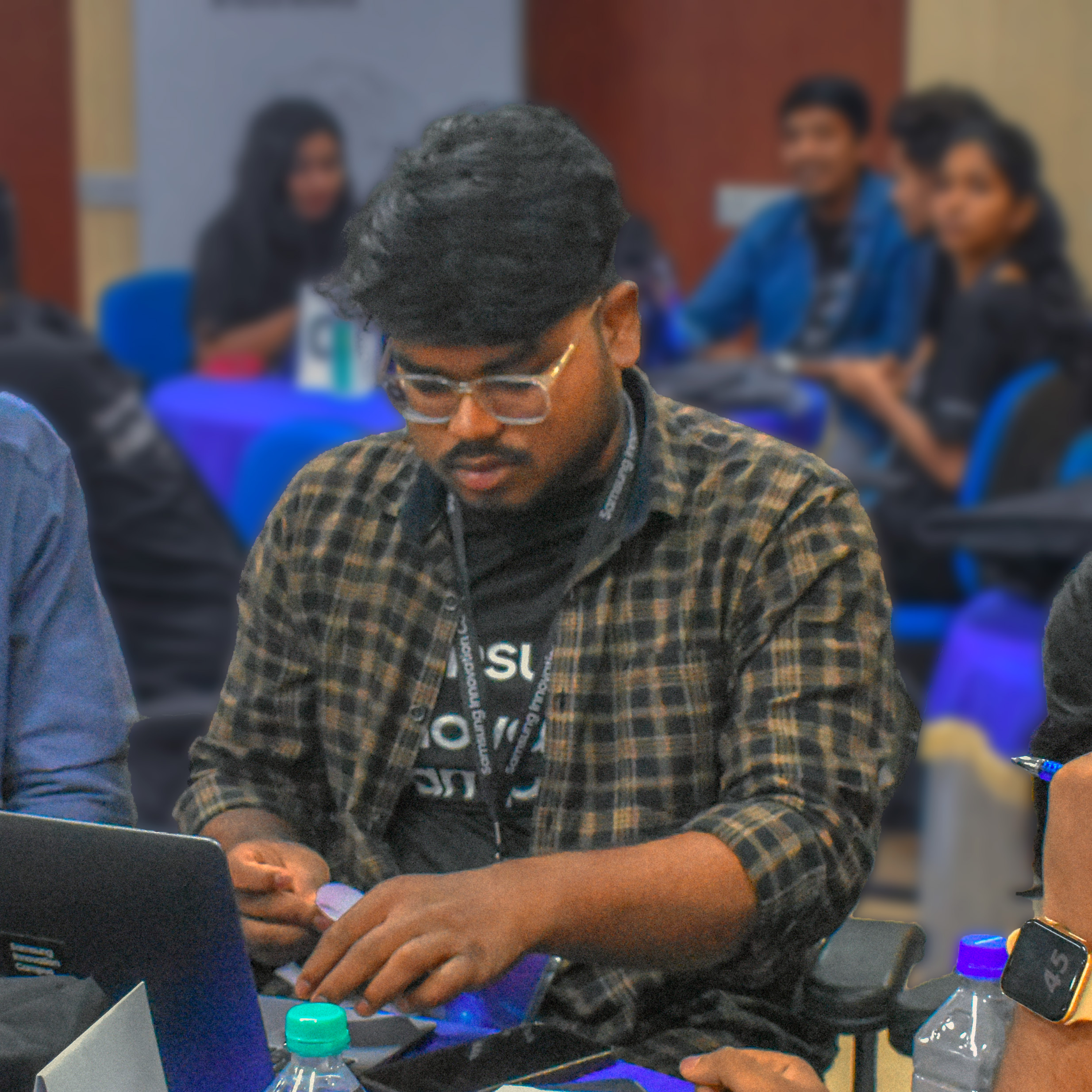
Given two sorted arrays nums1 and nums2 of size m and n respectively, return the median of the two sorted arrays.
The overall run time complexity should be O(log (m+n)).
Example 1:
Input: nums1 = [1,3], nums2 = [2] Output: 2.00000 Explanation: merged array = [1,2,3] and median is 2. Example 2:
Input: nums1 = [1,2], nums2 = [3,4] Output: 2.50000 Explanation: merged array = [1,2,3,4] and median is (2 + 3) / 2 = 2.5.
Constraints:
nums1.length == m nums2.length == n 0 <= m <= 1000 0 <= n <= 1000 1 <= m + n <= 2000 -106 <= nums1[i], nums2[i] <= 106
Approach: first merge the two arrays, then sort them. when the length of the array is odd median will be the middle element of the array when the length of the array is even median will be the average of the middle two elements. Code:
using System;
namespace TwoMedian
{
class Program
{
public double FindMedianSortedArrays(int[] nums1, int[] nums2)
{
int[] x = new int[nums1.Length + nums2.Length];
nums1.CopyTo(x, 0);
nums2.CopyTo(x, nums1.Length);
Array.Sort(x);
int m = x.Length;
double y;
if (m % 2 != 0)
{
y = x[m / 2];
}
else
{
int c = x[m / 2] + x[m / 2 - 1];
double d = Convert.ToDouble(c);
y = d / 2;
}
return y;
}
static void Main(string[] args)
{
int[] num1 = { 1, 2 };
int[] num2 = { 3, 4 };
Program obj = new Program();
double u=obj.FindMedianSortedArrays(num1,num2);
Console.WriteLine(u);
}
}
}
Subscribe to my newsletter
Read articles from Dhananjay Sahoo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
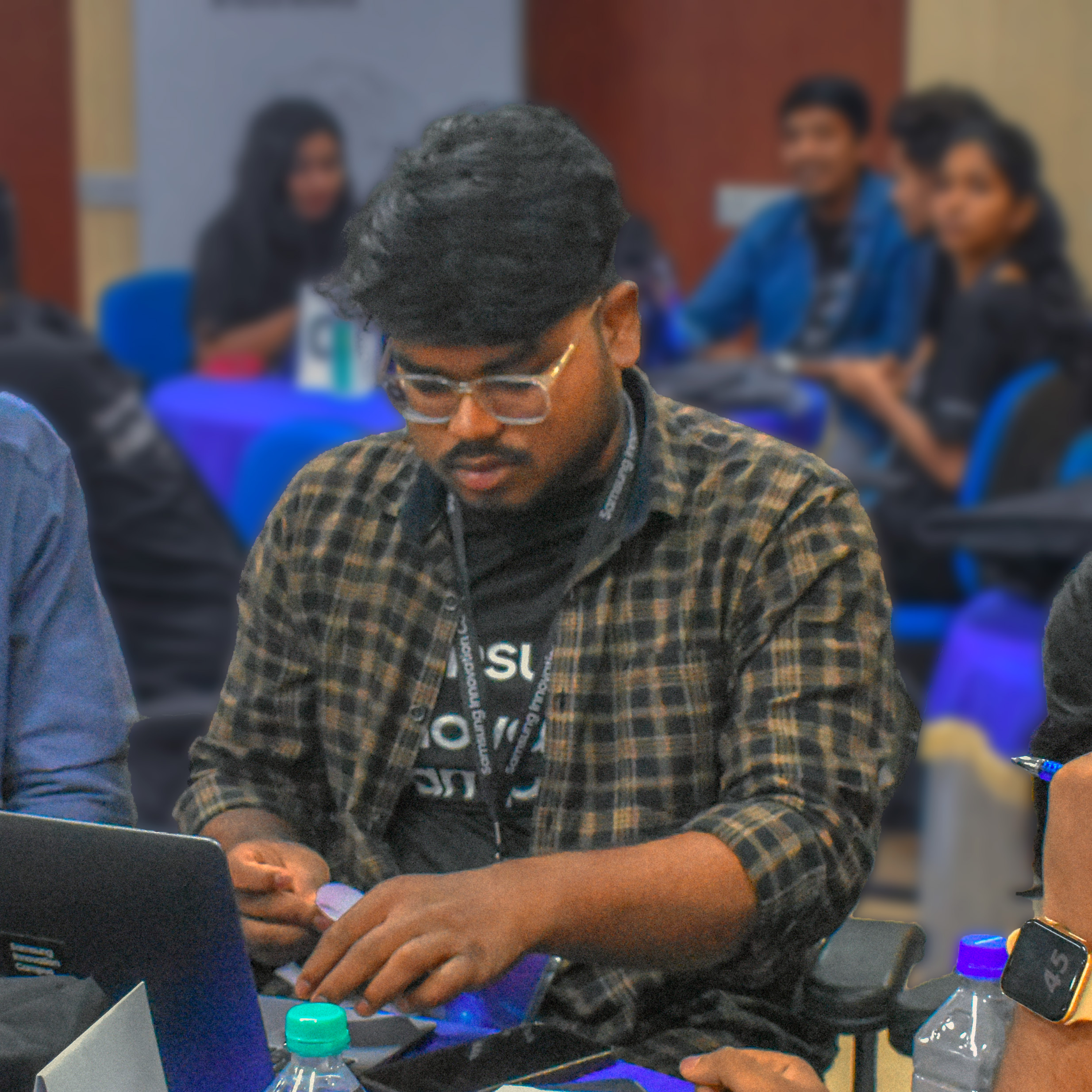
Dhananjay Sahoo
Dhananjay Sahoo
I am studying Computer Science in the 3rd year of graduation.