Problem: Sudoku Solver
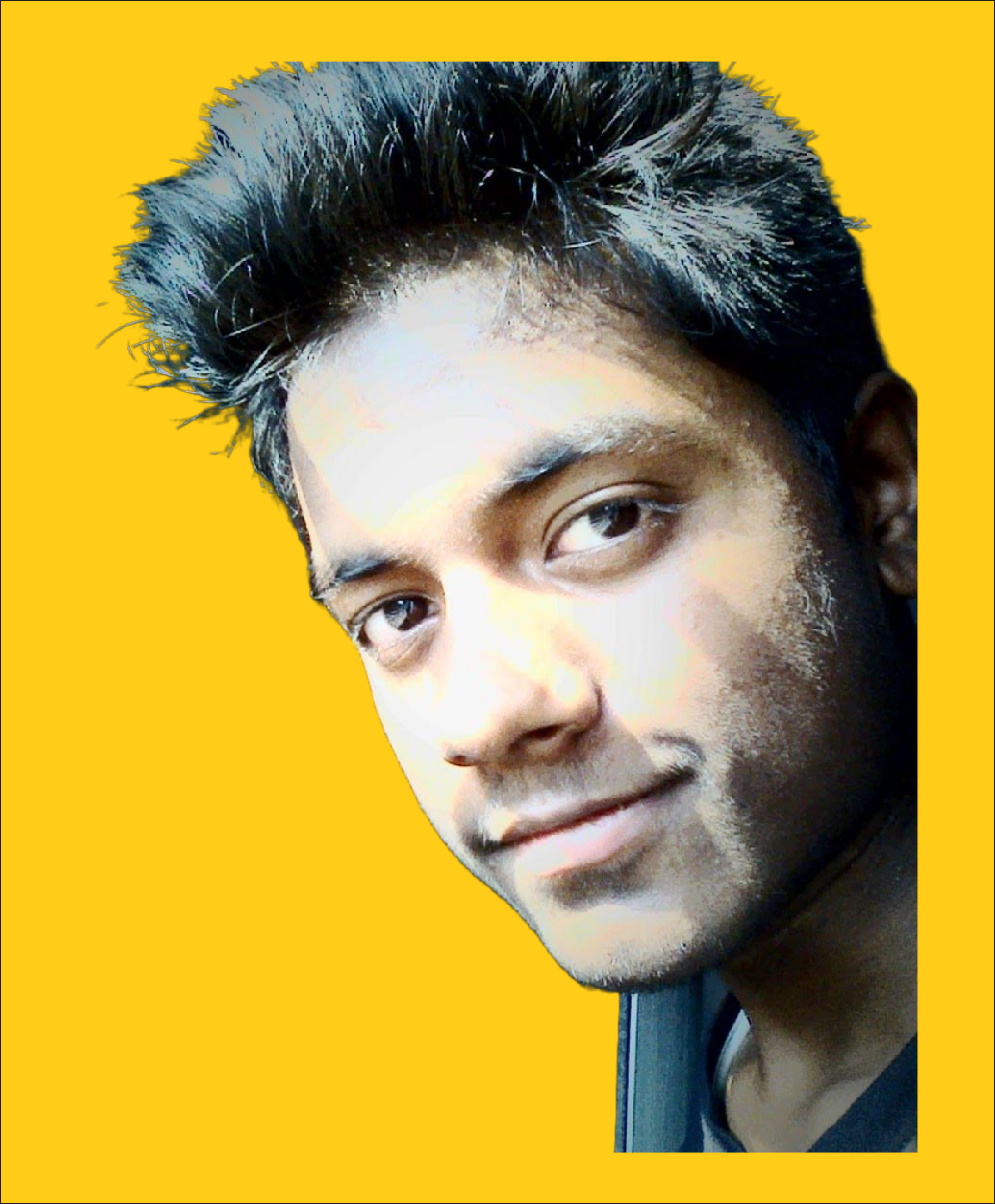
2 min read
Write a program to solve a Sudoku puzzle by filling the empty cells.
A sudoku solution must satisfy all of the following rules:
Each of the digits 1-9 must occur exactly once in each row. Each of the digits 1-9 must occur exactly once in each column. Each of the digits 1-9 must occur exactly once in each of the 9 3x3 sub-boxes of the grid.
The '.' character indicates empty cells.
class Solution {
boolean done = false;
void solve(char[][] board, int row, int col) {
if (col == board[0].length) {
row = row + 1;
col = 0;
}
if (row == board.length) {
done = true;
return;
}
if (board[row][col] == '.') {
for (int i = 1; i <= 9; i++) {
if (isSafe(row, col, (char) (i + '0'), board)) {
board[row][col] = (char) (i + '0');
solve(board, row, col + 1);
if (!done)
board[row][col] = '.';
}
}
} else
solve(board, row, col + 1);
}
private boolean isSafe(int row, int col, char val, char[][] board) {
for (int i = 0; i < board.length; i++) {
if (board[i][col] == val)
return false;
}
for (int i = 0; i < board[0].length; i++) {
if (board[row][i] == val)
return false;
}
int sR = (row / 3) * 3;
int eR = sR + 3;
int sC = (col / 3) * 3;
int eC = sC + 3;
for (int i = sR; i < eR; i++) {
for (int j = sC; j < eC; j++) {
if (board[i][j] == val)
return false;
}
}
return true;
}
public void solveSudoku(char[][] board) {
solve(board, 0, 0);
}
}
1
Subscribe to my newsletter
Read articles from ANKUSH KUMAR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
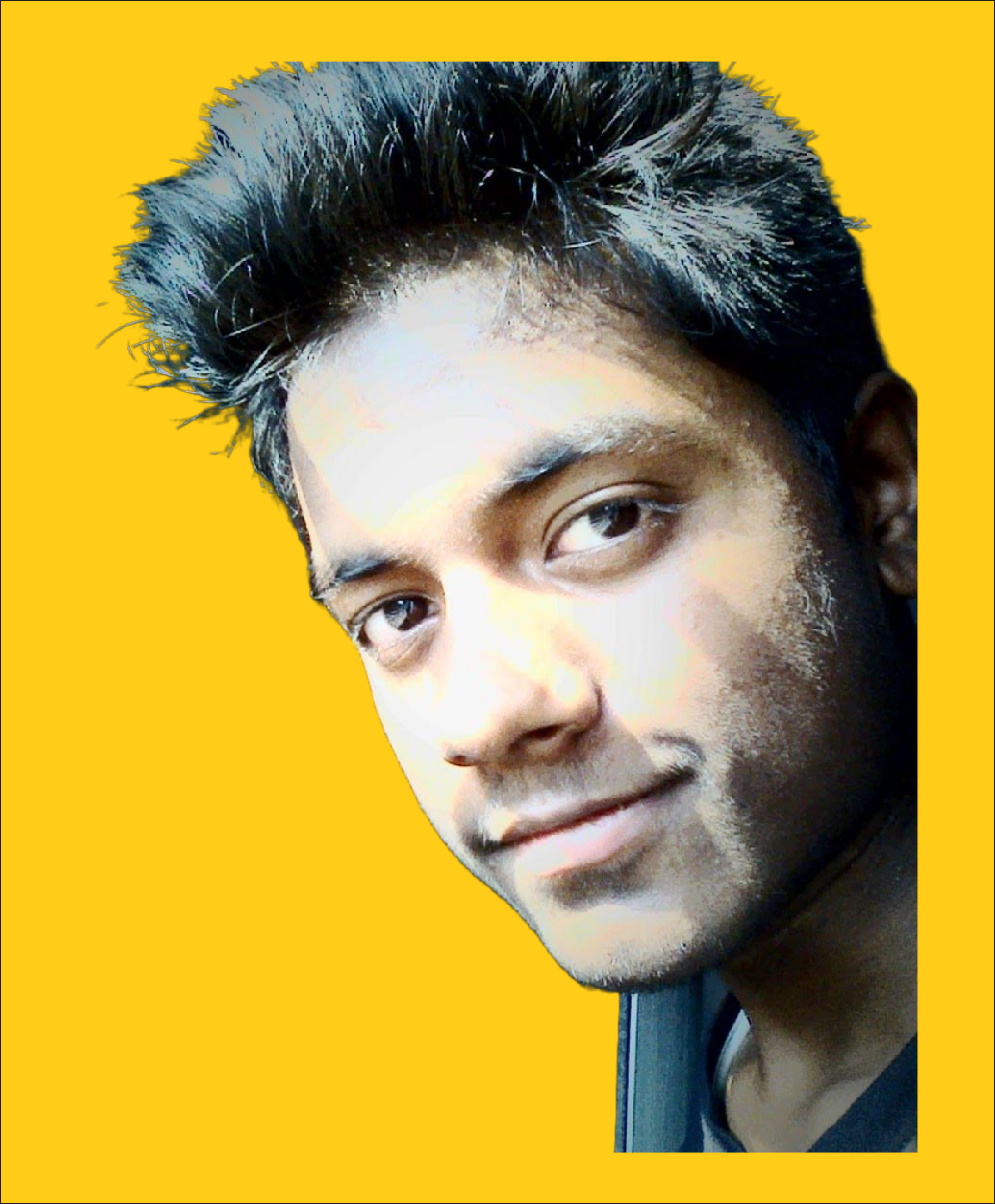
ANKUSH KUMAR
ANKUSH KUMAR
({MERN Stack Developer at mackph}) | <Ex-Internshala Student Partner(ISP 16)> | <5 star coder at Hackerrank> | <Self employed at Mackph> | <GSSoC '22 Contributor> | <Ex-Campus Ambassador at International MUN>