Array In JavaScript
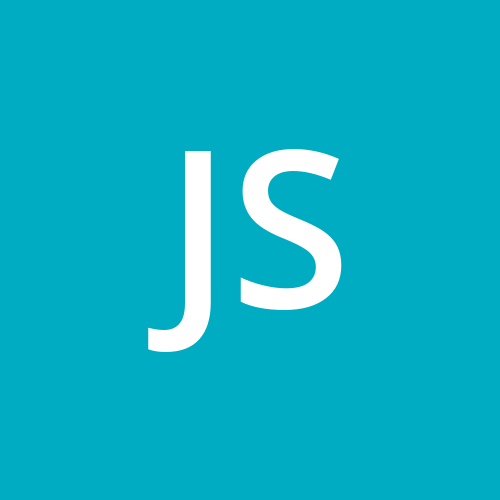
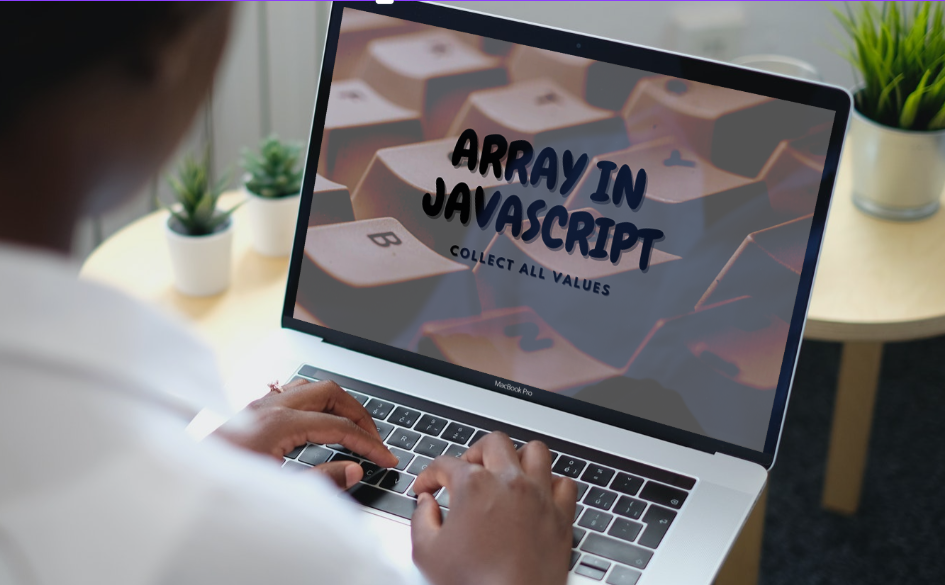
In this article , we take a quick revision about Arrays in JavaScript.
Introduction Of Array
In other programming language, array is a collection of elements of similar datatype in a single variable name but In JavaScript, Array is an object that is the collection of elements of different datatype in a single variable name i.e., it collect mixture of elements of different data type.
Declaration of array
Three different methods are used to declare an array in JavaScript:-
METHOD 1
Syntax
variable_name array_name= [ element1, element2,....]
// Initializing while declaring
const student_detail= ["Rohit", "Btech", "CS33", 245251, "Delhi"];
METHOD 2
Syntax
variable_name array_name= new Array(element1, element2,....)
// Initializing while declaring
// Array directly using new keyword
const student_detail=new Array("Rohit", "Btech", "CS33", 245251, "Delhi");
METHOD 3
Syntax
variable_name array_name= new Array(length)
// Array constructor using new keyword
const student_detail=new Array(5);
// assign values
student_detail[0] = "Rohit" ;
student_detail[1] = "Btech";
student_detail[2] = "CS33" ;
student_detail[3] = 245251 ;
student_detail[3] ="Delhi" ;
Basic Method To Access The Value Of Array
Syntax: To access all of the element :
console.log(array_name);
console.log(student_detail);
Syntax: To access any particular element of an index:
console.log(array_name[index];
console.log(student_detail[0]);
Replacing Array Element
code:
const student_detail=new Array("Rohit", "Btech", "CS33", 245251, "Delhi");
console.log(student_detail);
student_detail[0] = "Ram" ;
console.log(student_detail);
output:
["Rohit", "Btech", "CS33", 245251, "Delhi"]
["Ram", "Btech", "CS33", 245251, "Delhi"]
Length property of an Array
The property length of an array gives the number of the elements that would be stored in the array.
Syntax:
variable_name name = array_name.length;
Basic Array Methods In JavaScript
concat() Method:
The concat() Method is used in javascript to combine two or more arrays and give another array.
isArray() Method:
The isArray() Method is used to check whether the passed argument is an array or not.
at() Method:
The at() Method take the index value of an array and return the element of that index.
push() Method:
The push() Method is used to push element at the end of the array.
pop() Method:
The pop() Method is used to remove element from the end of the array.
unshift() Method:
The unshift() Method is used to add elements at the front of array.
shift() Method:
The shift() Method is used to remove elements at the front of array.
splice() Method:
The splice() Method is used to remove and insert elements from one index to other index i.e., remove or add element at a particular location.
Subscribe to my newsletter
Read articles from jyoti singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
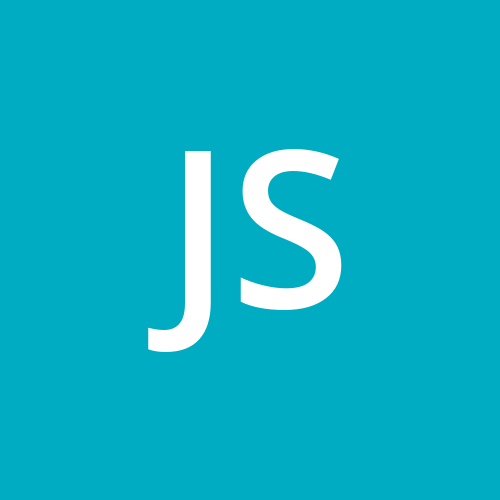