JavaScript Array and its Methods
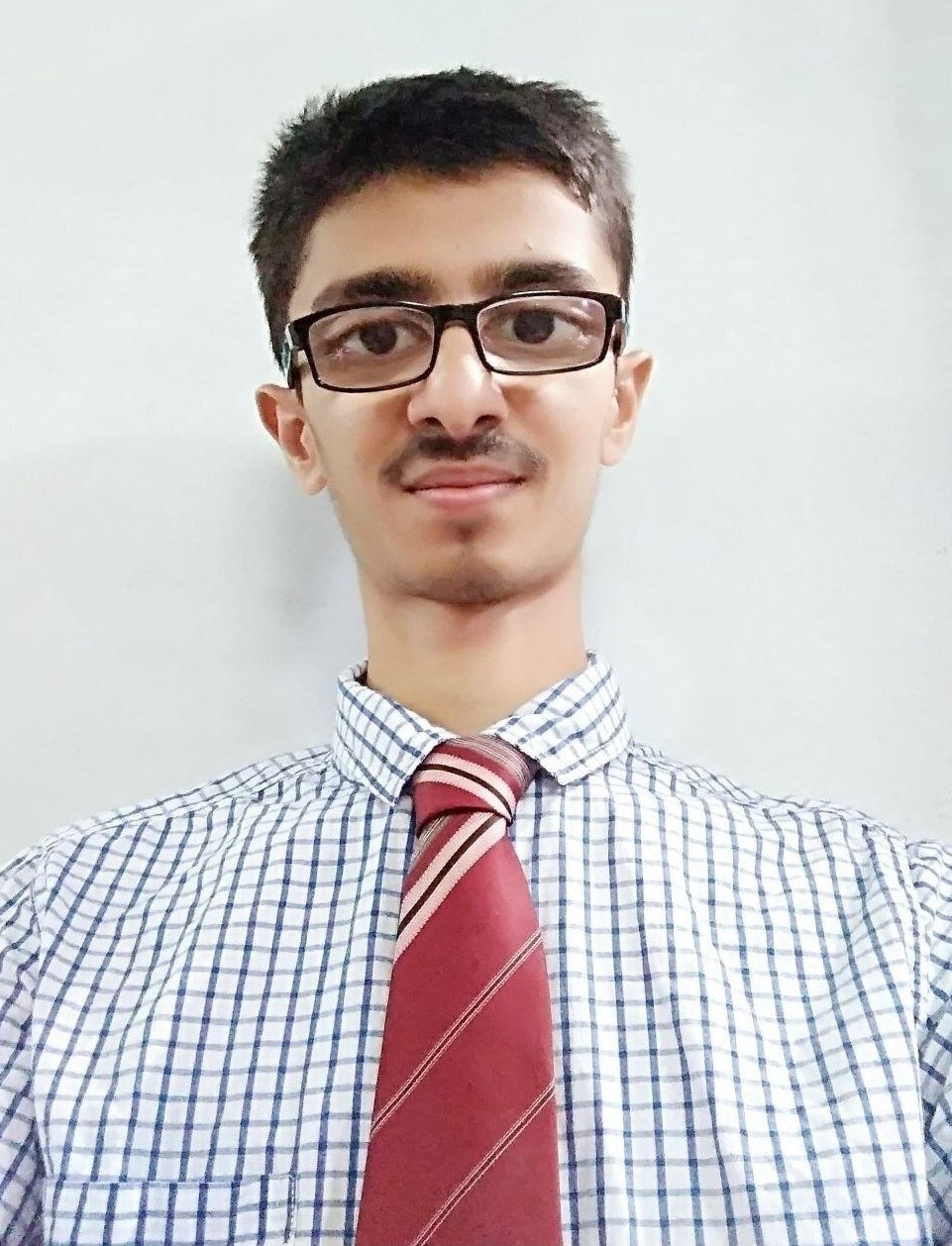
In this article I will explain what is an array and why to use an array and what are some of its methods-
An array is a special variable, which can hold more than one value-
const fruits = ["Apple", "Orange", "Banana"];
This is an array of cars which has three values in it.
If you have a list of items (a list of car names, for example), storing the cars in single variables could look like this:
let fruit1 = "Apple";
let fruit2 = "Orange";
let fruit3 = "Banana";
However, what if you want to loop through the cars and find a specific one? And what if you had not 3 cars, but 300?
The solution is an Array!
An array can hold many values under a single name, and you can access the values by referring to an index number.
Syntax of an Array:
const array_name = [item1, item2, ...];
Array indexes start with 0:
- [0] is the first array element
- [1] is the second
- [2] is the third
Methods of Array-
1)toString Method- This JavaScript method toString() converts an array to a string of (comma separated) array values.
Example-
const fruits = [
"Apple",
"Orange ",
"Grapes ",
"Strawberry ",
"Cherry "
];
console.log(fruits.toString());
// Apple,Orange ,Grapes ,Strawberry ,Cherry
2).push() Method-
Push method adds provided elements at the end of an Array. you can pass multiple parameters in push() method. let's see an example.
Example-
const vegetables = [
"Carrots ",
"Potatoes ",
"Chilli "
];
vegetables.push("Broccoli ")
console.log(vegetables);
// [ 'Carrots ', 'Potatoes ', 'Chilli ', 'Broccoli ' ]
see here, I pushed Broccoli , and it's added at the last index of array, this method will modify the original array and this method return number which is the new length of the array after pushing given elements.
3).pop() Method-
The pop method will remove the last element of an Array. Let's understand it by example.
Example
const vegetables = [
"Carrots ",
"Potatoes ",
"Chilli "
];
let removedVegetable = vegetables.pop()
console.log(`${removedVegetable} removed from Array.`);
console.log(vegetables);
// Chilli removed from Array.
// [ 'Carrots ', 'Potatoes ' ]
see here, Chilli is at the last index of an Array, so the pop method removed it, and it will modify the original Array. this method will return the removed item from an array.
4).includes() Method-
This method takes two parameters, one is searchString and another is startIndex which is optional. if the provided element is present in the array then it will return True else false
Example
const fruits = [
"Apple ",
"Orange ",
"Grapes ",
"Mango "
];
let apple = fruits.includes("Apple");
console.log(apple);
// returns true
5).indexOf() Method-
This method takes two parameters one is searchString and another is startIndex which is optional. if the provided element is present in the array then it will return the index of that element, and if the element is not present in the Array then it will return -1.
Example-
const vegetables = [
"Carrots ",
"Potatoes ",
"Chilli "
];
console.log(vegetables.indexOf("Chilli "));
// 2
Conclusion-
Although there are many methods of an array.For more information you can visit W3Schools.
Thanks for reading.Follow for more.And don't forget to hit a ๐ if you find this article helpful. Happy learning! ๐
Subscribe to my newsletter
Read articles from Ayush Nighoskar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
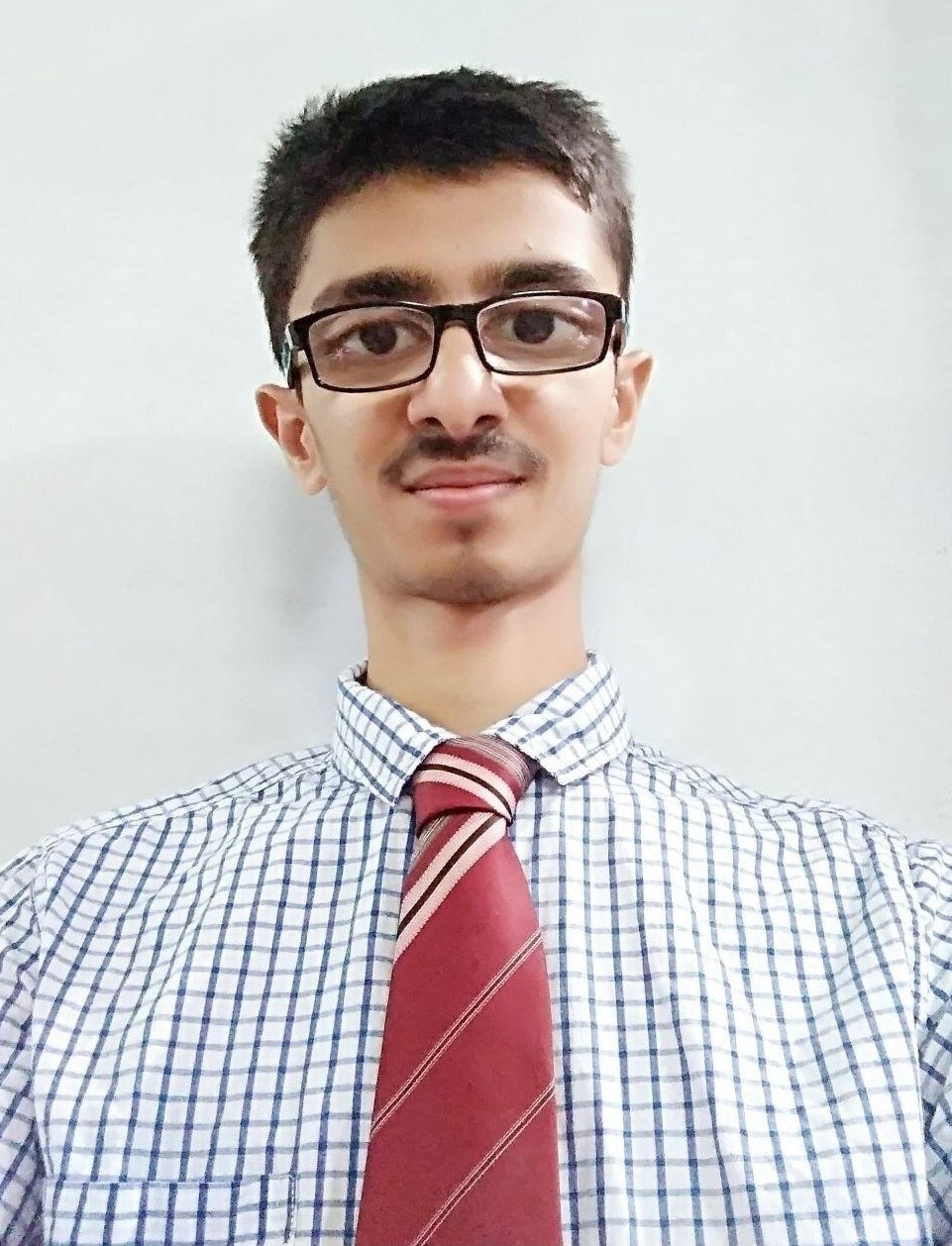
Ayush Nighoskar
Ayush Nighoskar
Experienced Software Developer interested in Web Development (MERN stack). Along with my technical skill sets, I possess clear verbal and written communication skills and in due time, am capable enough to do the assigned presentation and solve problems. I am always eager to learn more and improve my skills in various aspects of my career along with the organization.Are you interested in staying up-to-date with the latest tech trends and insights? Follow me for more thought-provoking tech articles that will help you expand your knowledge and skills as a developer.