JavaScript Array
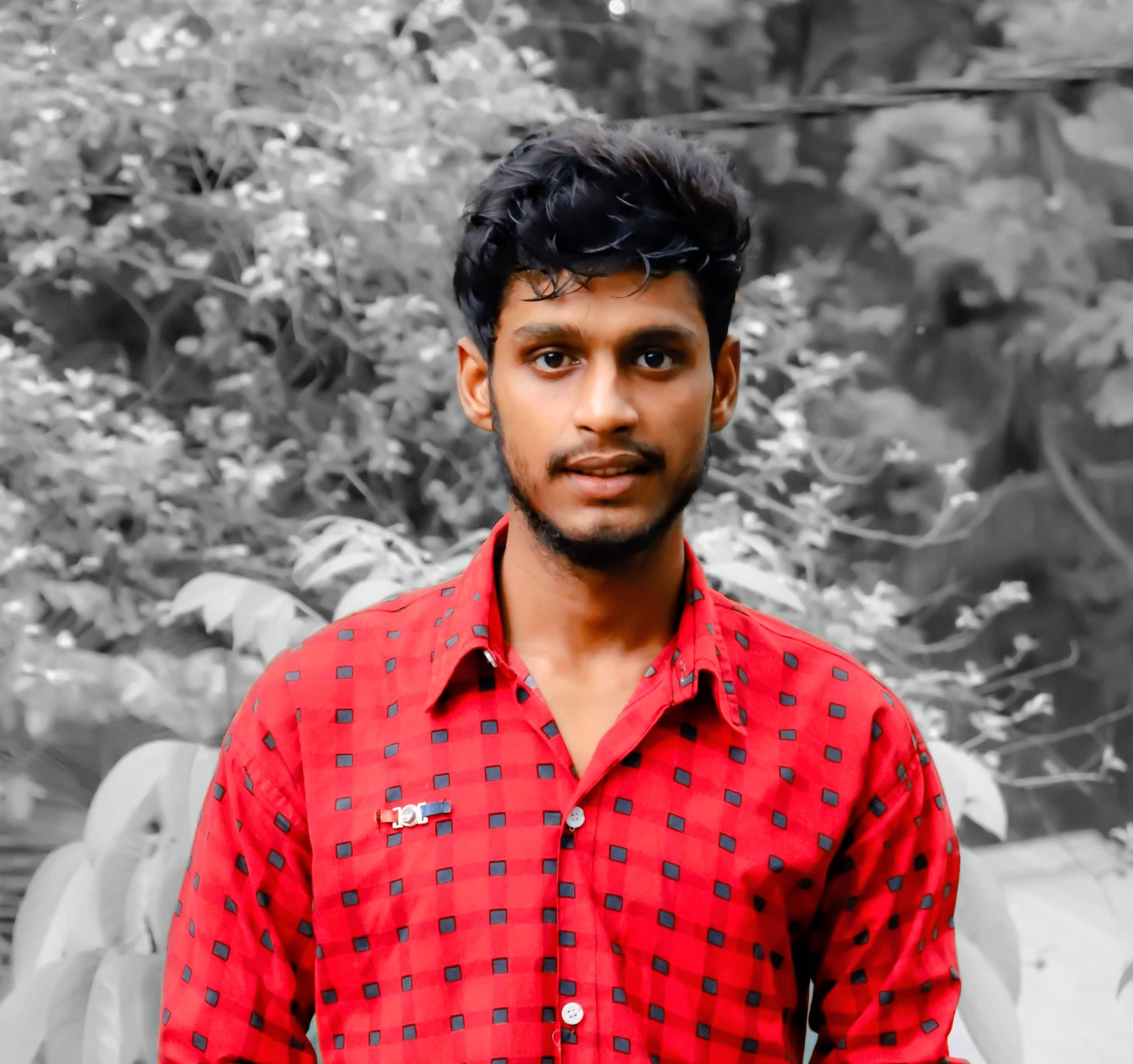
2 min read
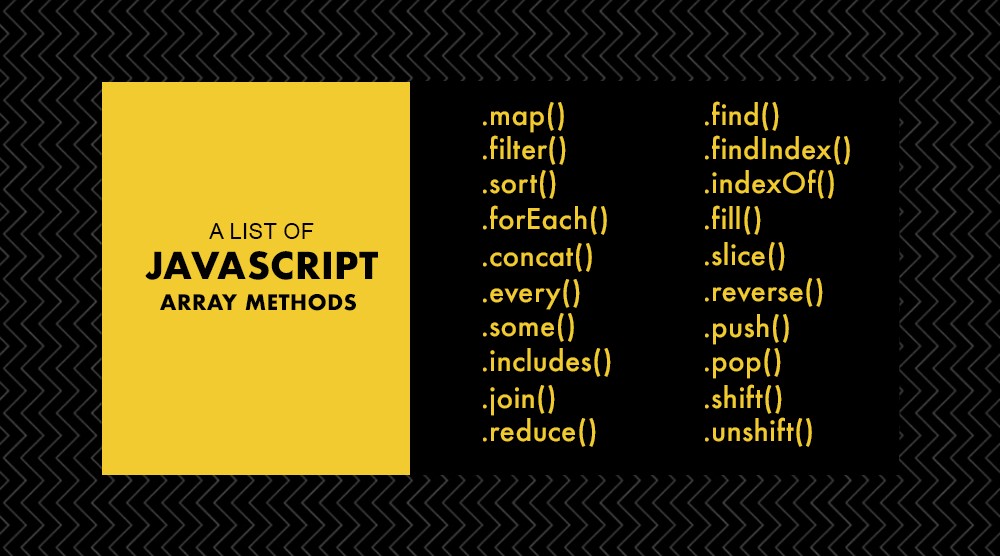
What is Array ?
An array is a special variable, which can hold more than one value.
let newArray = [12, "Koushik", null, false, 100]
All Array Methods In JavaScript
I will Discuss about some of them.
How to Create an Array ?
let newArray = [1, 2, 3, 4, 5];
let newArray =new Array("Koushik", "Saha", true);
Array Methods :
For output of methods, you have to run below codes in your code editor, and this is best practice.
1. Array Length -
let newArray = [1, 2, 3, 4, 5];
console.log(newArray.length);
it is a array property. Not a method.
2. Array.Push( ) -
This method adds elements at the end of an array.
let newArray = [ ];
newArray.push(10);
newArray.push(12, "Koushik");
console.log(newArray);
3. Array.Pop( ) -
This method removes elements from the end of the array.
let newArray = [1, 2, 3, 4];
newArray.pop();
console.log(newArray)
4. Array.Shift( ) -
It is the opposite of array.pop(). It removes elements from the front of the array.
let newArray = [1, 2, 3, 4];
newArray.shift();
console.log(newArray)
5. Array.UnShift( ) -
It is the opposite of array.push(). It add elements from the front of the array.
let newArray = [1, 2, 3, 4];
newArray.unshift();
console.log(newArray)
# How to Grab Elemnts from an Array :
Array Index start from 0.
let newArray = [1, 2, 3, 4];
// Grabing first element.๐
console.log(newArray[0]);
// Grabing second element.๐
console.log(newArray[1]);
// Grabing third element.๐
console.log(newArray[2]);
// Grabing last element.๐
console.log(newArray[newArray.length - 1]);
1
Subscribe to my newsletter
Read articles from Koushik Saha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
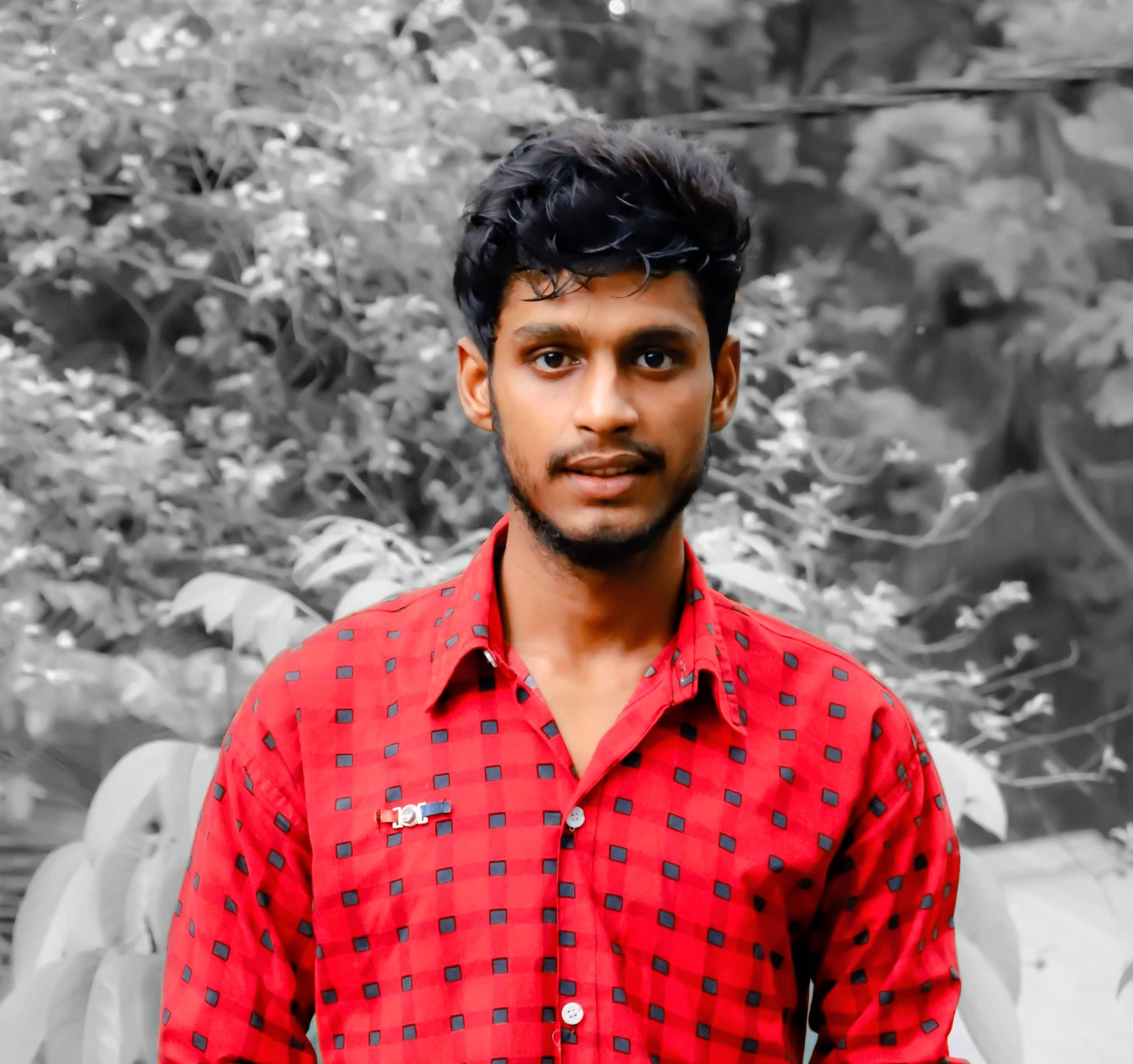
Koushik Saha
Koushik Saha
A Good Learner. And Web Developer.