JavaScript: call(), bind() & apply() methods with examples
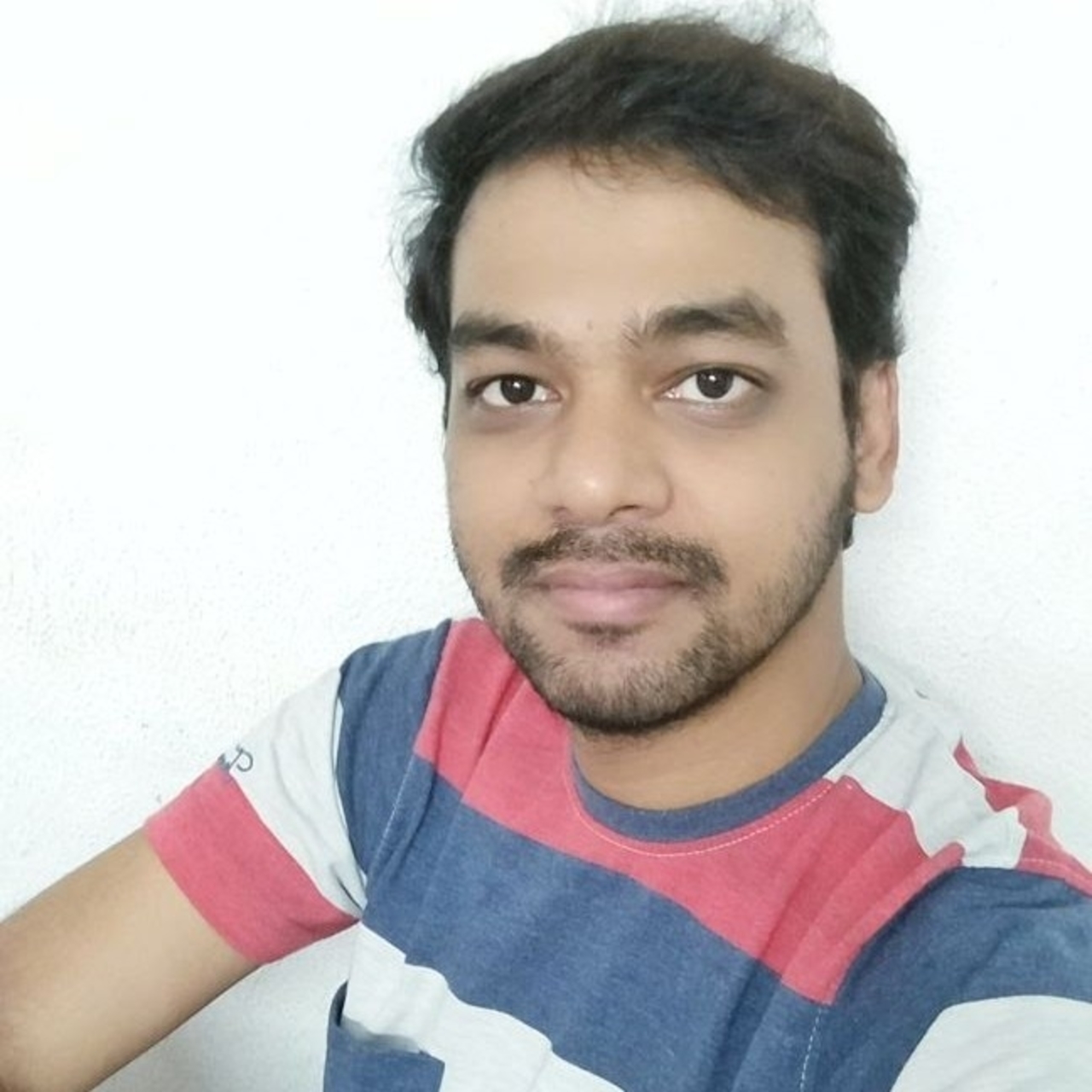
1 min read
Table of contents
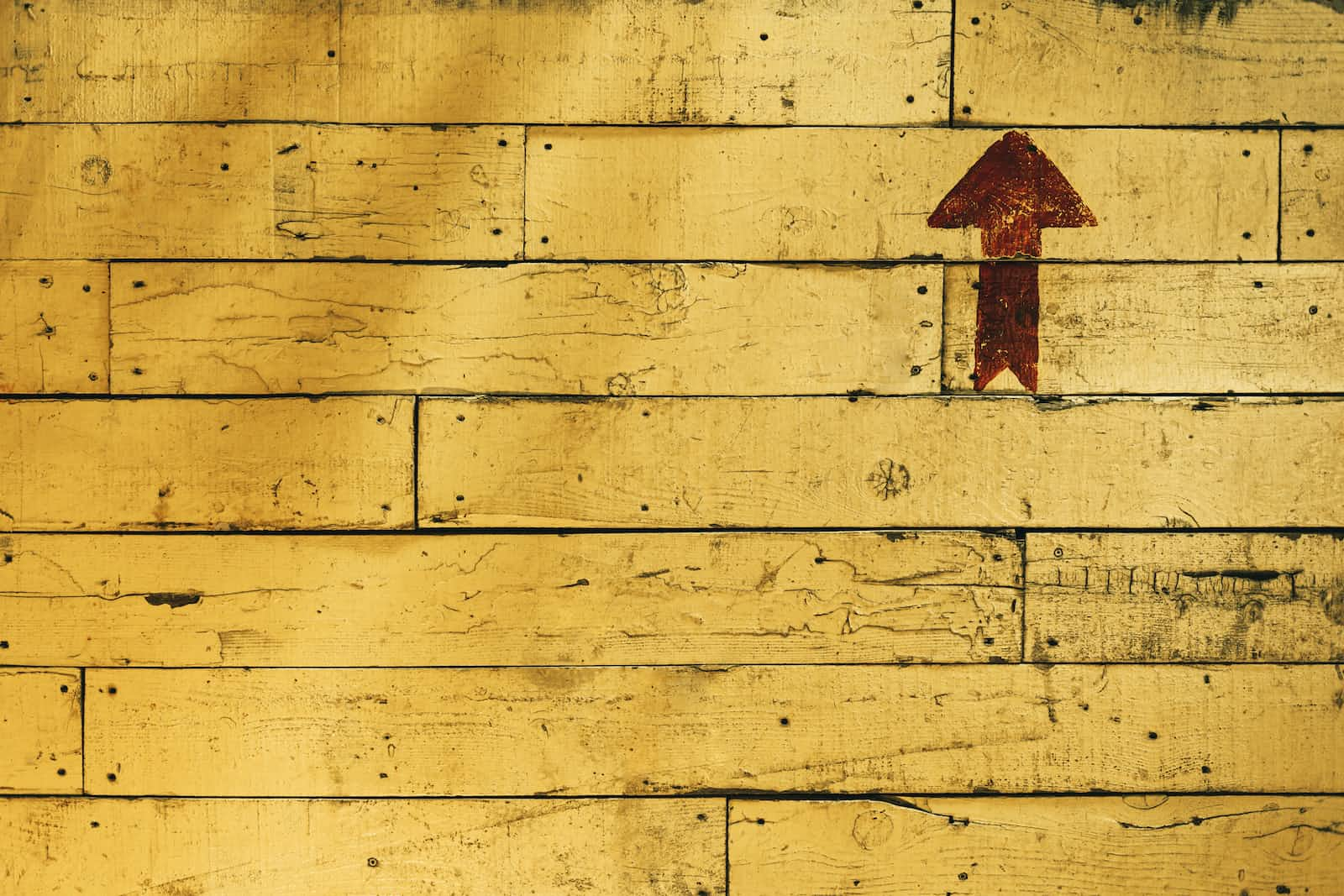
// Call method
let employeeOne = {
fullName: 'John Doe',
age: 24,
salary: 40000,
salaryHike(hike) {
this.salary += hike
}
};
let employeeTwo = {
fullName: 'Sarah Green',
age: 26,
salary: 60000
};
console.log("Call method");
console.log(employeeTwo.salary);
employeeOne.salaryHike.call(employeeTwo, 10000);
console.log(employeeTwo.salary);
console.log('---------------------------------------------------');
// Apply method
let employeeThree = {
fullName: 'John Doe',
age: 24,
salary: 40000,
salaryHike(hike) {
this.salary += hike
}
};
let employeeFour = {
fullName: 'Sarah Green',
age: 26,
salary: 60000
};
console.log("Apply method");
console.log(employeeFour.salary);
employeeThree.salaryHike.apply(employeeFour, [10000]);
console.log(employeeFour.salary);
console.log('---------------------------------------------------');
// Bind method
let employeeFive = {
fullName: 'John Doe',
age: 24,
salary: 40000,
salaryHike(hike) {
this.salary += hike
}
};
let employeeSix = {
fullName: 'Sarah Green',
age: 26,
salary: 60000
};
console.log("Bind method");
console.log(employeeFive.salary);
const hikeEmployeeSix = employeeFive.salaryHike.bind(employeeSix, 10000);
hikeEmployeeSix();
console.log(employeeSix.salary);
Output:
Call method
60000
70000
-------------------
Apply method
60000
70000
-------------------
Bind method
40000
70000
0
Subscribe to my newsletter
Read articles from Hari Krishna Anem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
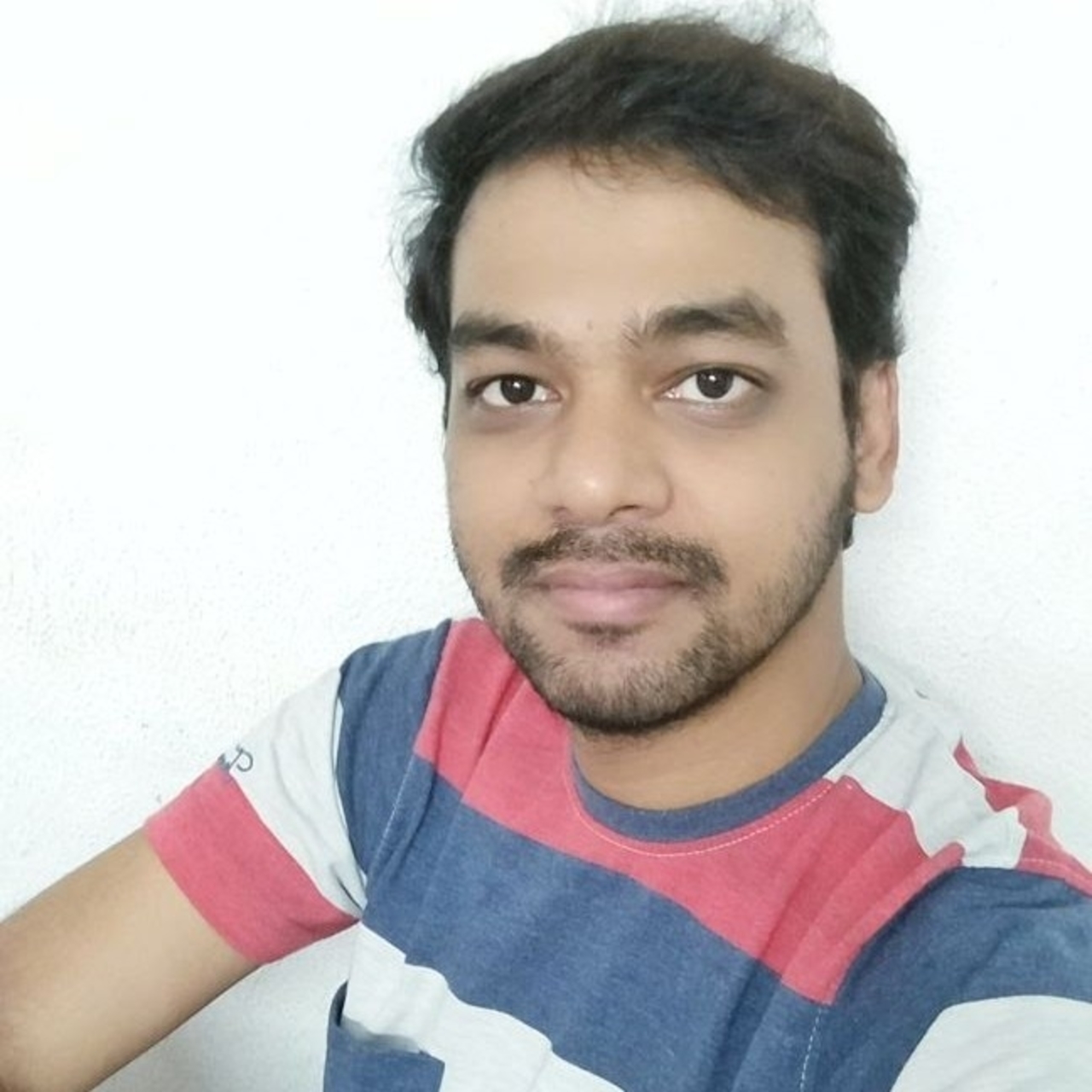
Hari Krishna Anem
Hari Krishna Anem
Full stack developer (ReactJS, NodeJS, JavaScript, PHP, SQL)