How to find pathname of any URL - Javascript
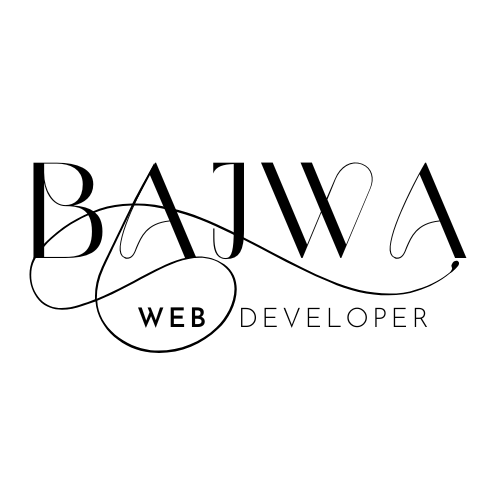
In this article I will share the knowledge of converting a string
into URL
and manipulating the properties of Uniform Resource Locator (URL).
Let's start ๐
Scenario 1
We need current pathname
from URL of the current webpage to consume it in our application.
Solution
We can get URL of the current webpage with window object. The following code gives us the location
object of the current document.
console.log(window.location);
The window.location
will return the following Location object as a read-only result.
{
hash: ""
host: "https://devbajwa.com"
hostname: "https://devbajwa.com"
href: "https://devbajwa.com/programming/languages/javascript/objects"
origin: "https://devbajwa.com"
pathname: "/programming/languages/javascript/objects"
port: ""
protocol: "https:"
search: ""
}
window.location
object has several properties which carries the information about the location of the current document.
Now, suppose the following URL is current document https://devbajwa.com/programming/languages/javascript/objects
.
href
window.location.href
gives us the absolute URL of the current document.
console.log(window.location.href)
->
"https://devbajwa.com/programming/languages/javascript/objects"
pathname
Similarly we can access the pathname
with window.location.pathname
property. This is also referred as relative URL.
console.log(window.location.pathname)
->
"/programming/languages/javascript/objects"
Scenario 2
We fetch
JSON data from an API call and receive a response with an absolute URL. We need pathname
of that absolute URL.
Since the response of an API call is in string
format and not from the window.location
object, we don't have access the location
or pathname
property for that string
value(URL).
Example
const absoluteURL = "https://devbajwa.com/programming/languages/javascript/objects";
console.log(absoluteURL.location);
->
undefined
If we try to access location
object of our custom string
format URL, we will get undefined
response.
Solution
We can leverage modern URL API available that accesses and manipulates URLs. The new URL(string)
parses the string
format URL and provides access to the integral parts through its properties.
Simply pass the string
formatted URL to the new URL()
function and the resulted value is a URL
now. We have access to various properties.
href
const absoluteURL = new URL("https://devbajwa.com/programming/languages/javascript/objects");
console.log(absoluteURL.href);
->
"https://devbajwa.com/programming/languages/javascript/objects"
pathname
const absoluteURL = new URL("https://devbajwa.com/programming/languages/javascript/objects");
console.log(absoluteURL.pathname);
->
"/programming/languages/javascript/objects"
Codepen
Conclusion
Modern Javascript is becoming more declarative now a days. We should leverage the available modern Web APIs to make our code more readable and developer friendly. However, keep check on the browser support before use :)
Subscribe to my newsletter
Read articles from Waqas Naeem Bajwa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
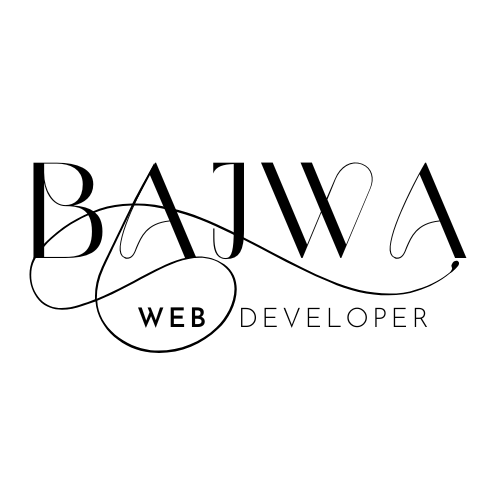