Javascript Strings & Its Method

Table of contents
- What is String
- How String stored in memory
- String Constant Pool (SCP)
- Strings objects are immutable
- String Methods
- 1. length
- 2. indexOf()
- 3. lastIndexOf()
- 4. startsWith()
- 5. endsWith()
- 6. toUpperCase()
- 7. toLowerCase()
- 8. includes()
- 9. repeat()
- 10. charAt()
- 11. substring()
- 12. padStart()
- 13. padEnd()
- 14. match()
- 15. matchAll()
- 16. search()
- 17. localeCompare()
- 18. replace()
- 19. replaceAll()
- 20. concat()
- 21. split()
- 22. trim()
- 23. slice()
- Common methods name in Array & String
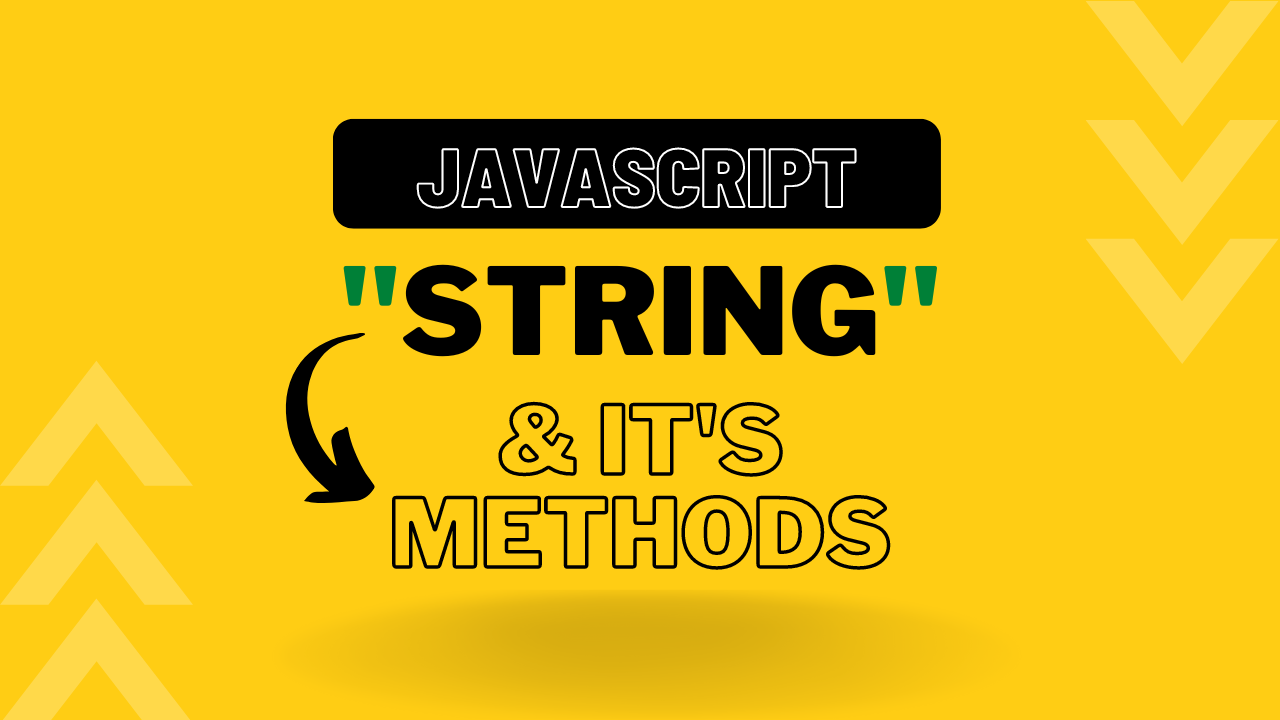
The string data type is the most common in programming languages. Most of cases we have to work on strings, rather than numbers or others. That's why knowing concepts and methods about string always makes you confident.
In this article, I will discuss What is a string, what happens when you perform any operation on a string, and at the end detailed discussion of string methods with examples.
What is String
In a programming language, we work with different types of data/values such as integer
, float
, boolean
, text/string
etc. That type of value which is actually a character or sequence of characters is called string
in the programming language. A person's name, address, about all information is treated as string type.
In javascript, we can declare a string two ways.
let str1 = "Adnan";
let str2 = new String("Adnan");
console.log(str1); // Adnan
console.log(str2.valueOf()); // Adnan
Both variables str1 & str2 store string data. But their memory representation is different and their value is the same but not they are. let's see an example,
let str1 = "Adnan";
let str2 = "Adnan";
console.log(str1 === str2); // true
let str3 = new String("Adnan");
console.log(str1 === str3); // false
How String stored in memory
We can create a variable for the string two ways & they are not the same. Now I would like to discuss why they are different and their memory representation.
At first, the string is an object type so all strings are stored in a memory area called Heap
, and their reference variable is stored in a memory area called stack
, Why do I say reference variable instead of the variable? because a variable is store a value inside his memory address but the reference variable stores only his reference address, not the reference itself.
let x = 5; // a variable who holds 5 inside his memory address
let str = new String("Adnan"); // a reference variable that holds string reference's address, not reference itself
Now, If the reference variable is stored in his reference address then where is the reference stored?
I hope it is understandable for all where the string objects are stored and why their variables are called reference variables. Now the most interesting part comes, I said strings are objects and they are stored in the memory heap area. but creating a string object using new
or single/double quotations that aren't stored in the same place.
All the string objects created directly using single/double quotations are stored in the String Constant Pool (SCP)
which is inside in heap area. Let's dive deep into SCP.
String Constant Pool (SCP)
SCP is an area where stored string objects and all the string objects are unique. Unique means if a string object is created and its value is "Adnan"
then if another object is created in the same as "Adnan"
then inside SCP there will be only one object which holds the string value "Adnan".
let str1 = "Adnan";
let str2 = "Adnan";
Both str1 & str2 are holding a string object which is "Adnan", but the two objects contain the same string value that's why only one string object will be created inside the SCP area and str1 & str2 both refer to the same object.
let str1 = "Adnan";
let str2 = "Adnan";
console.log(str1 === str2); // true
As they are referring same string object that's why the output shows true.
Now, what happened if we create a string object using new String()
? let's dive deep into it.
If we create a string object using the new
keyword then it will create a string object in the SCP area if the same string object doesn't exist in the SCP area and it will also create the same string object outside the SCP area and inside the heap memory area. Now you can understand what happened if you use a new keyword instead of double/single quotations.
let str = new String("Adnan");
It will create two string objects, one will be stored inside the SCP area and one object will be stored inside the heap memory area.
let str1 = new String("Adnan");
let str2 = new String("Adnan");
console.log(str1 === str2); // false
Why false? because they refer to the different string objects which is stored inside the heap memory area, not the SCP area.
let's take one last example then you will be confident about string objects and how they are stored in memory.
let str1 = "Adnan";
let str2 = "Adnan";
console.log(str1 === str2); // true
let str3 = new String("Adnan");
let str4 = new String("Adnan");
console.log(str3 === str4); // false
First, two reference variables will refer to the same object which is stored inside the SCP area, that's why the first output shows true. The last two reference variables will refer to different string objects inside the heap memory area and that's why the second output shows false.
That's all about javascript string and how it's stored inside the memory. This SCP is also available in java programming. But you might be thinking about why SCP needs and why the same value's string object doesn't create more than one time in the SCP area. Let's discuss it.
Strings objects are immutable
In javascript, string objects are immutable means you can't change the content of a string object and they are not mutable. So, If we try to perform any operations in a string object to change its content, then it will create another new string object inside the SCP area with updated content. That means we never change the string object, if we try to, then it will create a new string object with updated content.
let str = "Adnan";
str.toLocaleUpperCase();
console.log(str); // Adnan
It doesn't change the main string object, but it will return a new string object with that updated content;
let str1 = "Adnan";
let str2 = str1.toLocaleUpperCase();
console.log(str1); // Adnan
console.log(str2); // ADNAN
Why String objects are immutable? because one string object is referred to multiple reference variables, So if one reference variable can change the string object's content then it will affect all the reference variables also. That's why Strings are immutable.
The last confusion was why SCP is needed. The main benefit is it took less memory space to store objects. If there is no SCP area, then every string object with the same content will be created and it took more and more memory space for this, and then there is no need to string immutable concept because every reference variable is referred to as its own string object.
String Methods
1. length
First of all, it's not a method, it's a property of the string object and stores the total number of characters of the string object.
Return type: number
let str = "Adnan";
console.log(str.length); // 5
2. indexOf()
The indexOf() method simply returns the first occurrence of the search value. The second argument which is where to start searching is by default 0.
The syntax is: indexOf( search value, where to start the searching )
Return type: number
let str = "Hello World";
console.log(str.indexOf("o")); // 4
3. lastIndexOf()
This method is the opposite of the indexOf() method. It simply returns the last occurrence of the search value.
The syntax is: lastIndexOf( search value, where to start the searching but it will count from the last index )
Return type: number
let str = "Hello World";
console.log(str.lastIndexOf("o")); // 7
4. startsWith()
The startsWith() method simply checks if the argument's value is found from the start index. If found then return true
, otherwise false
.
The syntax is: startsWith( search value )
Return type: boolean
let str = "Hello World";
console.log(str.startsWith("Hel")); // true
console.log(str.startsWith("Heo")); // false
5. endsWith()
This method is simply the opposite of startsWith() method and simply checks if the provided value matches the last index and returns true or false.
The syntax is: endsWith( search value )
Return type: boolean
let str = "Hello World";
console.log(str.endsWith("rld")); // true
console.log(str.endsWith("rll")); // false
6. toUpperCase()
This method simply makes a new string object with all uppercase letters based on the current string object's content and returns the new string object which contains all uppercase letters.
The syntax is: toUpperCase()
Return type: new string object
let str1 = "Adnan";
let str2 = str1.toUpperCase();
console.log(str1); // Adnan
console.log(str2); // ADNAN
7. toLowerCase()
This method is the opposite of toUpperCase() method and returns a new string object which contains all lowercase letters.
The syntax is: toLowerCase()
Return type: new string object
let str1 = "Adnan";
let str2 = str1.toLowerCase();
console.log(str1); // Adnan
console.log(str2); // adnan
8. includes()
This method will check if the provided argument's string is available in the main string object. If found then returns true
otherwise false
. The second argument is by default 0 if not provided.
The syntax is: includes( searching value, which index to start checking )
Return type: boolean
let str = "This article is related to javascript";
console.log(str.includes("is"); // true
9. repeat()
Returns a string by repeating it at a given argument's times. That means a new string object will be created and its content will be repeated by passing the argument's times.
The syntax is: repeat( how many times repeat the content )
Return type: new string object
let str1 = "Adnan";
let str2 = str1.repeat(2);
console.log(str1); // Adnan
console.log(str2); // AdnanAdnan
10. charAt()
Simply returns the specific index character which is provided by the argument.
The syntax is: charAt( provide index number )
Return type: a string character, not an object
let str = "Adnan";
console.log(str.charAt(4)); // n
11. substring()
This method returns a new string object based on the argument's range. We need to pass where to start and end index and this method will return a new string object which has that specific range's content. This substring() method will be copying the content for the new string object from starting index to the ending index, but the ending index is not included.
The syntax is: substring( starting index, ending index )
Return type: new string object
let str1 = "This article is related on javascript";
let str2 = str1.substring(5, 12);
console.log(str1); // This article is related on javascript
console.log(str2); // article
12. padStart()
This method will create and return a new string object with provided argument length and padding character. So, you have to pass what new length of the string and which character you want to padding start with, by default it is blank space " "
.
Note:
this method will add padding characters at the start if there is found any space.
The syntax is: padStart( new length, padding character )
Return type: new string object
let str1 = "Adnan";
let str2 = str1.padStart(10, "+");
console.log(str1); // Adnan
console.log(str2); // +++++Adnan
13. padEnd()
It is the opposite of the padStart() method and adds padding characters at the end instead of the start.
The syntax is: padEnd( new length, padding character )
Return type: new string object
let str1 = "Adnan";
let str2 = str1.padEnd(10, "+");
console.log(str1); // Adnan
console.log(str2); // Adnan+++++
14. match()
This method returns an array of matched strings based on a regular expression argument. That means, we have to pass a regex as an argument. It will return an array with the matches otherwise null
.
The syntax is: match( regular expression-regex )
Return type: an array of strings
let str1 = "Adnan Sarkar";
let str2 = str1.match(/[A-Z]/g);
console.log(str1); // Adnan Sarkar
console.log(str2); // [ 'A', 'S' ]
15. matchAll()
The matchAll() method returns an iterator of matched strings based on a regular expression argument.
The syntax is: matchAll( regular expression-regex )
Return type: an iterator object
let str1 = "Adnan Sarkar";
let str2 = str1.matchAll(/[A-Z]/g);
console.log(str1); // Adnan Sarkar
for (let str3 of str2) {
console.log(str3);
}
// output:
// [ 'A', index: 0, input: 'Adnan Sarkar', groups: undefined ]
// [ 'S', index: 6, input: 'Adnan Sarkar', groups: undefined ]
16. search()
This search() method also takes a regular expression as an argument. It will return the index of the first match between the regular expression and the given string and returns -1 if no match was found.
The syntax is: matchAll( regular expression-regex )
Return type: number
let str1 = "Adnan Sarkar";
console.log(str1.search(/[A-Z]/g)); // 0
console.log(str1.search(/[S]/g)); // 6
console.log(str1.search(/[0-9]/g)); // -1
17. localeCompare()
The localeCompare() method checks are the compared string is equivalent or sorted before or sorted after. If equivalent then returns 0
, if sorted before then -1 or any negative number
and if sorted after then 1 or any positive number
. In this method, there are two optional parameters, which are for customizing what formatting conventions to use.
The syntax is: localeCompare( compare string, locales, options )
Return type: number
console.log("abc".localeCompare("abc")); // 0
console.log("abc".localeCompare("bcd")); // -1
console.log("bcd".localeCompare("abc")); // 1
18. replace()
This method will return a new string object (because we are trying to change the immutable string object) with updated content.
The syntax is: replace( target string/regex, replace value )
Return type: new string object
let str1 = "Hello World";
let str2 = str1.replace("World", "Everyone");
console.log(str1); // Hello World
console.log(str2); // Hello Everyone
19. replaceAll()
This method will replace all the matches with a replacing value and return a new string.
The syntax is: replaceAll( target string/regex, replace value )
let str1 = "Hello World World";
let str2 = str1.replaceAll("World", "Everyone"); // It will give an error
console.log(str1); // Hello World World
console.log(str2); // Hello Everyone Everyone
Note:
Today there is no replaceAll() method in javascript for the string objects. You can use the replace()
method and pass the argument as a regex using the /replace value/g
g-flag (global) to replace all the occurrences. Still, MDN has a details discussion about String.prototype.replaceAll().
20. concat()
The contact() method simply does the concatenation / combined the main string object with provided string in the argument and returns a new string object.
The syntax is: concat( new string arguments separated with comma )
Return type: new string object
let str1 = "Adnan";
let str2 = str1.concat(" Sarkar");
console.log(str1); // Adnan
console.log(str2); // Adnan Sarkar
21. split()
This method will return an array where each element will separate by the argument's separator in the main string object. There is an optional second argument that indicates the limit of splitting.
The syntax is: split( separator character, limit of splitting )
Return type: an array of strings
let str1 = "Adnan Sarkar";
let str2 = str1.split(" ");
console.log(str1); // Adnan Sarkar
console.log(str2); // [ 'Adnan', 'Sarkar' ]
22. trim()
The trim() method will remove more than one whitespace from the start and the end of the content and return the new string object. It will work when content has extra whitespace, otherwise, return the main string object instead of creating a new one.
The syntax is: trim()
Return type: new string object
let str1 = " Adnan Sarkar";
let str2 = str1.trim();
console.log(str1); // Adnan Sarkar
console.log(str2); // Adnan Sarkar
23. slice()
The slice() method will extract the content from the string object and returns a new string object with extracted values. In this method, we have to pass the starting index, and it's mandatory, the second argument is where to end the extraction and it's by default value is the ending index. If we pass the ending index then it will not extract the ending index, extract from the starting index to before the ending index.
The syntax is: slice( starting index, ending index which is not included )
Return type: new string object
let str1 = "Adnan Sarkar";
let str2 = str1.slice(0, 5);
console.log(str1); // Adnan Sarkar
console.log(str2); // Adnan
Common methods name in Array & String
- indexOf()
- lastIndexOf()
- includes()
- concat()
- slice()
Their working mechanism is the same but they are not the same.
I tried to explain the most common string method which is regularly used in javascript. I hope you can understand one thing very clearly which is no one can change the string object because of immutability. If any method tries to change, it will create a new string object inside the SCP area
. So, if you use them, then try to catch the new string object inside a new reference variable. Thank you.
📢 My Social Links
Subscribe to my newsletter
Read articles from Adnan Sarkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Adnan Sarkar
Adnan Sarkar
Hello there, I'm Adnan Sarkar, currently studying BSCSE at United International University. At present, I'm learning full-stack javascript.