Control Flow Statements
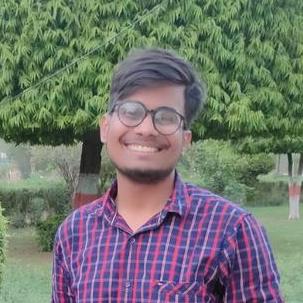
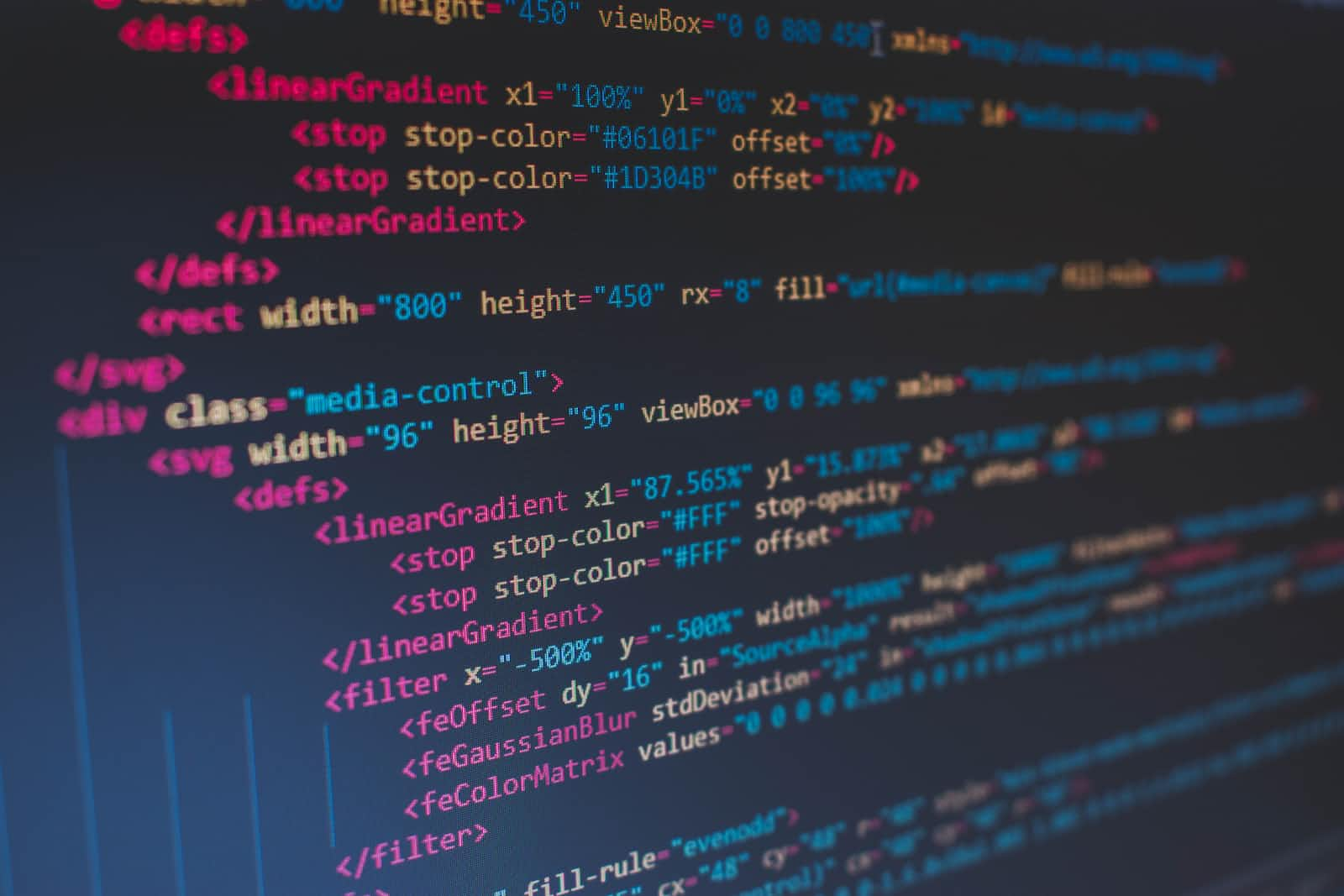
One of the basic things that help us to solve various programming problems are control flow statements. Now, every programmer at one point during the start of his programming journey might have wondered how am I gonna write a code that would give different results based on different conditions? And then we all came across "Control Flow Statements".
First of all, let's discuss something very basic such as defining control flow statements. According to MDN web docs
"The control flow is the order in which the computer executes statements in a script."
Well that covered the technical definition but to understand it better let's discuss about it in an easy way. We all know that code that we write is executed in top-down manner, to alter the course of our code execution according to our requirement, for example say getting different outputs for different conditions i.e. change the flow of our code from going through single path to multiple paths. For doing that we use some basic control flow structures.
Control flow structures can be broadly classified into two categories:
- Conditional Control Flow Statements.
- Lopping Control Flow Statements.
Conditional Control Flow Statements.
When the flow of control of a program is changed based on some condition using control statements, it is termed conditional flow of control.
In simpler terms, conditional control flow statements direct the flow of the program according to the conditions that we specify. Some of the most commonly used statements are:
- if
- if...else
- else if
- switch
If Statement
The if statement is the basic conditional statement which consists of a condition a block of code to be executed when the condition is fulfilled.
Syntax:
if (expression) {
Statements //Block of code to be executed
}
If....Else Statement
The if...else statement is similar to the if statement the only difference being the code inside the else block executing rather than moving to the next code line if the condition is not fulfilled.
Syntax:
if (condition) {
Statement(s) // if the condition is fulfilled
} else {
Statement(s) // if condition is not fulfilled
}
Else if Statement
The else if statement allows us to include a variety of conditions. The control checks the expression from top to bottom, goes to the next if one is false, and eventually finishes with the else statement, which acts as a default statement when none of the conditions are met.
Syntax:
if (condition 1) {
Statement(s) // if condition 1 is met
} else if (condition 2) {
Statement(s) // if condition 2 is met
} else if (condition 3) {
Statement(s) // if condition 3 is met
} else {
Statement(s) // if no condition is met
}
Switch Statement
Switch statements are similar to if-else-if statements. The switch statement contains multiple blocks of code called cases and a single case is executed based on the variable which is being switched. Default statement is executed when any of the case doesn't match the value of expression. Break statement terminates the switch block when the condition is satisfied.
Syntax:
switch (expression) {
case condition 1: statement(s)
break;
case condition 2: statement(s)
break;
case condition n: statement(s)
break;
default: statement(s) // executed when none of the conditions are met
}
Looping Control Flow Statements.
A loop is utilized for executing a block of statements more than once until a specific condition is fulfilled.
So in a situation which requires reusing the same block of code again and again we use loops. A great example of this is when we have to write a program to print a table of any given number. They are of the following types:
- For
- While
- Do While
For Loop
The for loop is one of the most commonly used loops in all the programming languages which continues to loop till the specified condition is met.
Syntax:
for (initialExpression; condition; updateExpression) {
// Body
}
Here,
For is the conditional statement. The initial variable from which the looping begins is referred to as initialExpression. Condition is where the conditions are written. The initial variable is either increased or decreased in the updateExpression.
While execution the loop checks the updateExpression with the condition statement after each iteration and if the condition is true loops again till the condition becomes false.
While Loop
The while loop works similar to the for loop i.e. loops till the conditions specified are met, the only difference being the initial variable is given before the looping statement.
Syntax:
while (condition) {
// Body
}
Here,
While is the conditional statement. Condition is where the conditions are written which when met terminates the loop.
Do While Loop
The do while loop is similar to while loop which can be easily seen from the syntax but the only notable difference is that it executes the instruction first and then checks the condition, basically this loop runs at least for 1 time before terminating. Syntax:
do
{
// Body
} while (condition)
Here,
The do represents that the loop structure is of do while. The condition is where the control checks if the variable fulfils it.
Conclusion
Well that sums up all the control flow statements that we commonly use while programming. I hope this blog was able to give you a brief idea about the control flow in the computer programming and types of statements involved in it to alter it according to our need.
Subscribe to my newsletter
Read articles from Aaditya Khantal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
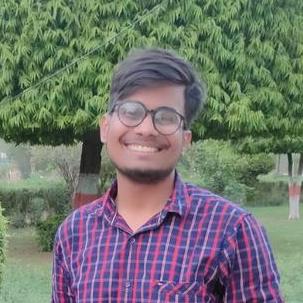