JavaScript Interview Prep Cheatsheet

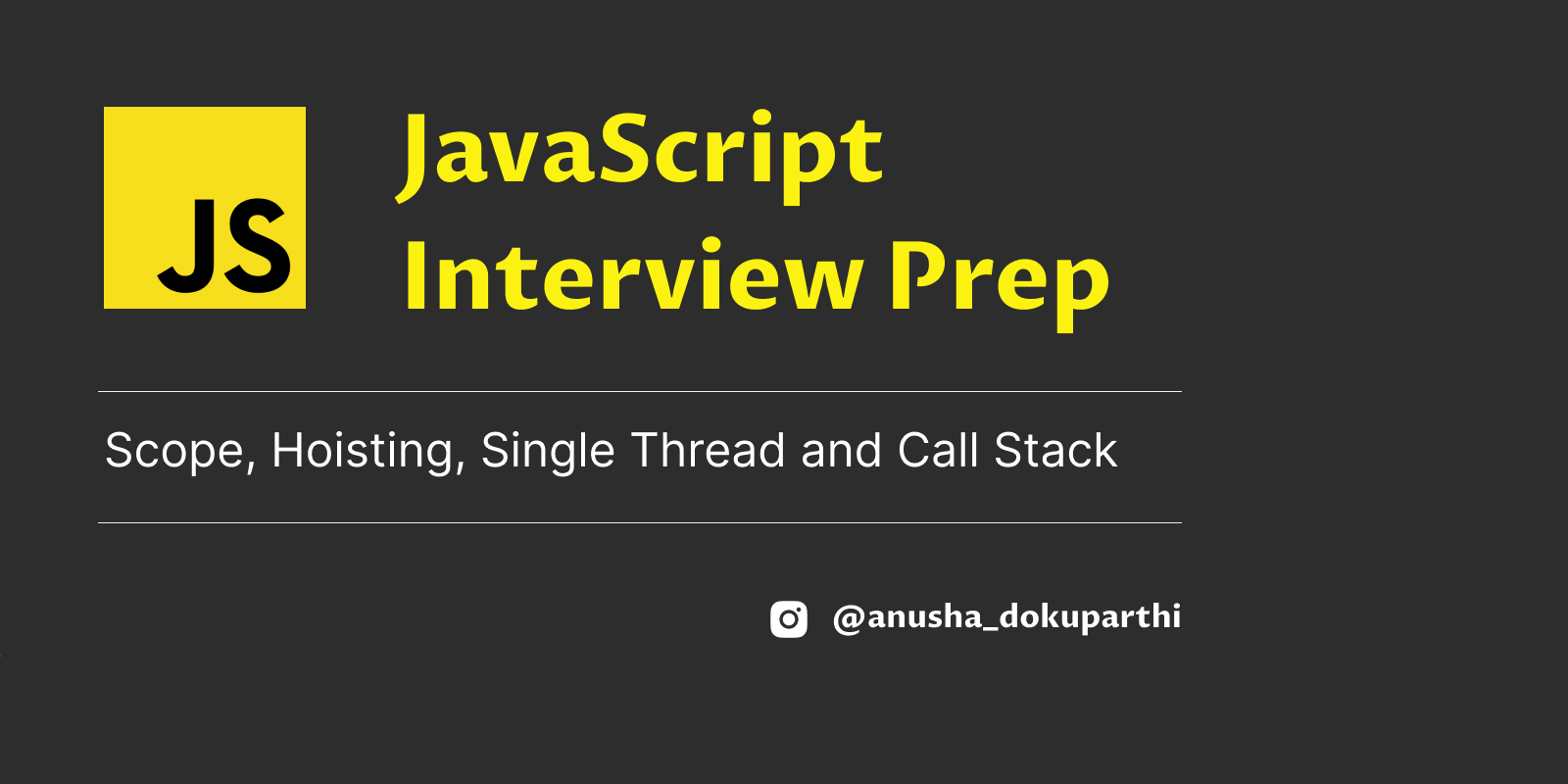
Hello Guys.. Today we are going to discuss about 4 main topics in JavaScript which you can expect in any interview.
Scope
Hoisting
Single thread
Call stack
Scope
What is scope? Scope can be defined as an area where an element can be accessed or available to use. In programming generally we talk about scope of variables and functions.
Before the introduction of ES6 all the variables has global scope, module scope and functional scope. In order to make it more interesting ES6 has introduced let and const datatypes which has changed the whole game play of the scope in the JavaScript.
So, now we have 4 types of scope.
Global Scope
Module Scope
Functional Scope
Block Scope
Global Scope
A variable declared outside of all the functions and blocks is called global variable and it is accessible or visible across the all the functions within the file is called global scope.
Example of Global scope
var name = 'Anusha Dokuparthi';
function getName(){
console.log(name);
}
function getUser(){
console.log(`Current loggedin user is ${name}`);
}
//Output:
//Anusha Dokuparthi
//Current loggedin user is Anusha Dokuparthi
Module Scope
Module scope refers to the access of variable within the current module that means if we create name as global variable in user.js and trying to access this variable in activity.js it will throw an error. We have to import the variables before accessing it in another module.
Example of Module Scope
//superheros.js
var hero = 'Iron man';
var power = 'Suit';
export { hero, power };
//marvelmovie.js
import { hero, power } from "./superheros";
function ironMan3(){
console.log(`Main lead is ${hero} and his power is ${power}`);
}
//Output:
//Main lead is Iron man and his power is Suit
Functional Scope
Functional scope can be referred as visibility or accessibility of variables within the function that the variable has been created.
Example of Functional Scope
function playCricket(){
var overScore = 7;
console.log(overScore);
}
console.log(overScore);
//output:
//Uncaught Reference Error
Note: We can't access the function variables outside of the function without calling them
function playCricket(){
var overScore = 7;
console.log(overScore);
}
playCricket();
//output:
//7
Block Scope
Block scope refers to the visibility or accessibility of the variables within the block of statements is called block scope. This is the new concept which mainly focusses on the let and const datatypes
Example of Block Scope
Example with var
function greet() {
let a = 'hello';
if(a == 'hello'){
var b = 'world'; //now b can be accessed from this statement until the end of function
}
console.log(a + ' ' + b);
}
greet();
Output:
hello world
Example with let
function greet() {
let a = 'hello';
if(a == 'hello'){
let b = 'world'; //now b can be accessed within this if block
}
console.log(a + ' ' + b);
}
greet();
Lexical Scope
When we drive deep into the scope of a variable we can hear the term called lexical scope. Before understanding the lexical scope let's try to understand the word is lexical.
Definition of Lexical:
Lexical itself refers definition that means anything related to creating words, expressions, or variables is termed lexical. In simple terms the place where elements were created.
Now, let's talk about lexical scope in programming language...
Lexical scope refers to an area or place where the variables were defined or created.
Example of lexical scope
const candyBox = 8; //lexical scope
function candyCount(){
console.log(candyBox); // Gives 8 because it has global scope
}
candyCount();
//Output
//8
Hoisting
In a program all the variables or functions that are initialized and tried to access before the declaration can be considered as Hoisting
Example of Hoisting with var
//Display harrypotter house
house = 'Gryffindor'
console.log(house);
var house;
Output
Gryffindor
Example of Hoisting with const
//Display harrypotter house
house = 'Gryffindor'
console.log(house);
const house;
Output
That means when the program is loaded all the variables and functions got scanned and remembered.
Program knew that the variable exists in the memory but when tried to access before declaration it gives the reference error and this behavior is called hoisting. Surprisingly this error doesn't occur in the case of var.
So, in JavaScript we can consider var as notorious datatype and after the introduction of let and const people are avoiding to use var for writing error free code
Single Thread
JavaScript is single threaded language that means all the statements in a program will execute in sequential fashion.
Let's try to understand by solving this Challenge.
Mom is coming home within 1 hour. Household chores needs to be finished.
- Clean house, 2. Wash clothes, 3. Clean utensils
Example:
//Mom is coming home
console.log('Cleaning house');
setTimeout(() => {
console.log('Wash clothes in washing machine');
}, 3000);
console.log('Cleaning utensils');
Output
Cleaning house
Cleaning utensils
Wash clothes in washing machine
When the program is executing, it places the first statement in the call stack which gets executed and prints Cleaning house in the console and pop out of the stack. Now, it places the second statement in the call stack and tries to execute the statement but it has setTimeout() function and can't be executed immediately so it pops out the function and puts in the WebAPI to get executed there. Since the call stack is now again empty, it places the third statement in the stack and executes it thus prints Cleaning utensils in the console.
Meanwhile, the WebAPI executes the timeout function and places the code in the callback queue. The eventloop checks if the call stack is empty or not if it is empty then it keep the third statement in the call stack otherwise it waits till the stack is empty
Call Stack
Javascript engine has
Memory Heap
Callstack
Memory heap: It is responsible to allocate the memory that is used by a program
Callstack: Before get into the details of the call stack. Let's understand what is stack?
Stack is a place where we keep the things in vertical fashion and follows the First In Last Out approach
The mechanism of interpreter of JavaScript to keep track of all the calls of multiple functions and calls with in the function
When a function is called the execution will go to that function and resumes only when that is finished
When any function calls another function then it add to the call stack and keeps there to carry out the action
If the function exceeds the stack then it causes stack overflow error
Example:
//baking a cake
function prepareBatter(){
console.log('Prepare batter');
}
function bakeCake(){
console.log('Bake the cake in oven');
}
function decorateCake(){
console.log('Decorate the cake with cherry toppings');
}
function birthdayCake(){
prepareBatter();
bakeCake();
decorateCake();
}
birthdayCake();
Output
Prepare batter
Bake the cake in oven
Decorate the cake with cherry toppings
Subscribe to my newsletter
Read articles from Anusha Dokuparthi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
