Python Built In Functions
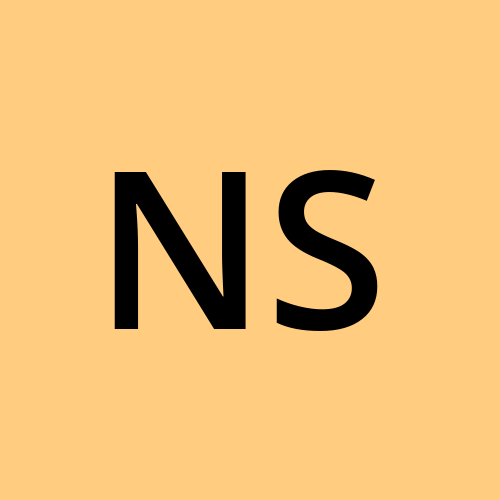
8 min read
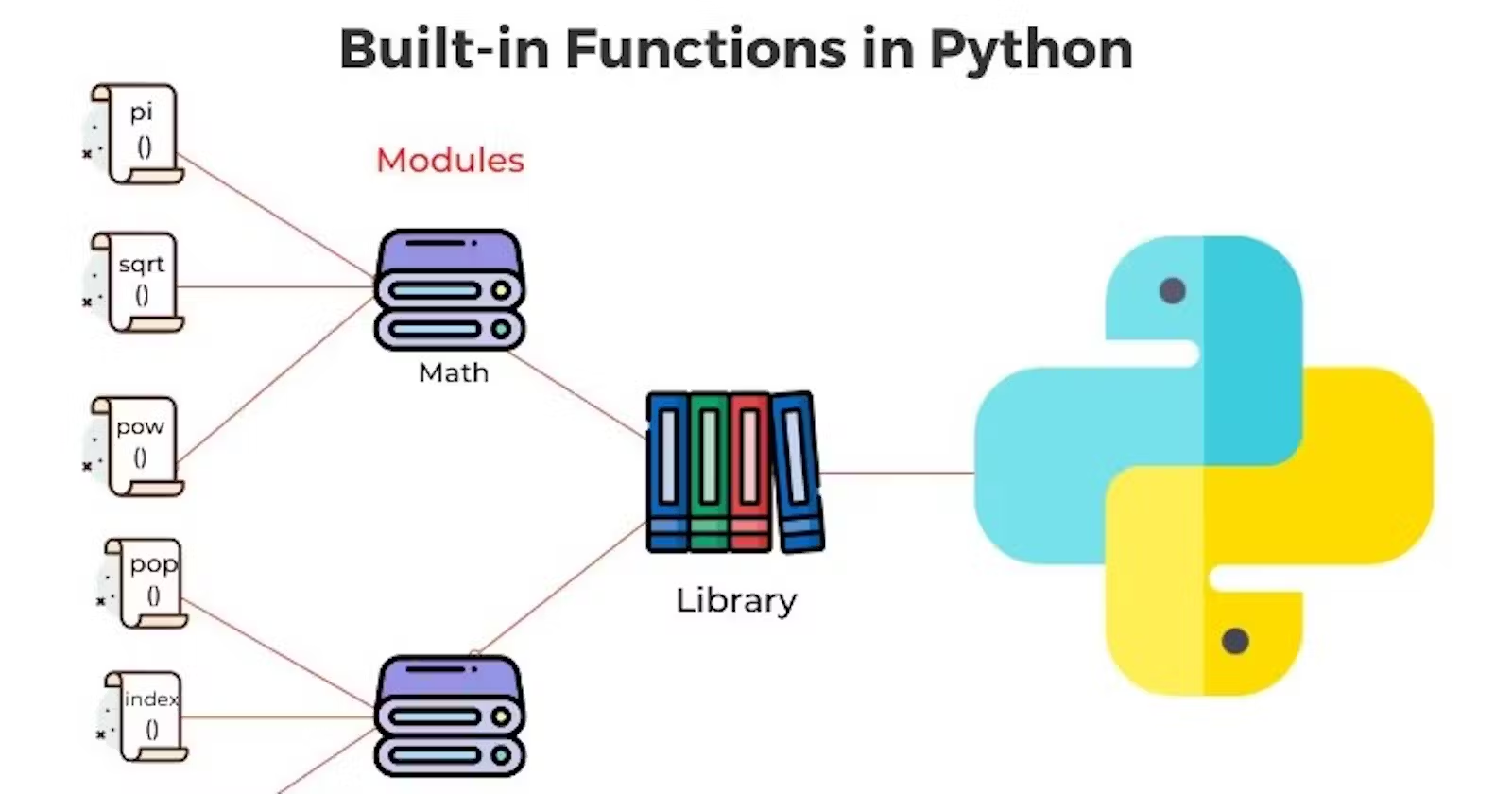
abs()
# Returns the absolute value of a number x = abs(-7.25) print(x) # OUTPUT # 7.25
all()
# Returns True if all items in an iterable object are true mylist = [True, True, True] x = all(mylist) print(x) # OUTPUT # True
any()
# Returns True if any item in an iterable object is true mylist = [False, True, False] x = any(mylist) print(x) # OUTPUT # True
ascii()
# Returns a readable version of an object. Replaces none-ascii characters with escape character x = ascii("My name is Ståle") print(x) # OUTPUT # 'My name is St\e5le'
bin()
# Returns the binary version of a number x = bin(36) print(x) # OUTPUT # 0b100100
bool()
# Returns the boolean value of the specified object x = bool(1) print(x) # OUTPUT # True
bytearray()
# Returns an array of bytes x = bytearray(4) print(x) # OUTPUT # bytearray(b'\x00\x00\x00\x00')
bytes()
# Returns a bytes object x = bytes(4) print(x) # OUTPUT # b'\x00\x00\x00\x00'
callable()
# Returns True if the specified object is callable, otherwise False def x(): a = 5 print(callable(x)) # OUTPUT # True
chr()
# Returns a character from the specified Unicode code. x = chr(97) print(x) # OUTPUT # a
classmethod()
# Converts a method into a class method
compile()
# Returns the specified source as an object, ready to be executed x = compile('print(55)', 'test', 'eval') exec(x) # OUTPUT # 55
complex()
# Returns a complex number x = complex(3, 5) print(x) # OUTPUT # (3+5j)
delattr()
# Deletes the specified attribute (property or method) from the specified object class Person: name = "John" age = 36 country = "Norway" delattr(Person, 'age') print(x) # OUTPUT # The Person object will no longer contain an "age" property
dict()
# Returns a dictionary (Array) x = dict(name = "John", age = 36, country = "Norway") print(x) # OUTPUT # {'name': 'John', 'age': 36, 'country': 'Norway'}
dir()
# Returns a list of the specified object's properties and methods class Person: name = "John" age = 36 country = "Norway" print(dir(Person)) # OUTPUT # ['__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__', 'age', 'country', 'name']
divmod()
# Returns the quotient and the remainder when argument1 is divided by argument2 x = divmod(5, 2) print(x) # OUTPUT # (2, 1)
enumerate()
# Takes a collection (e.g. a tuple) and returns it as an enumerate object x = ('apple', 'banana', 'cherry') y = enumerate(x) # OUTPUT # [(0, 'apple'), (1, 'banana'), (2, 'cherry')]
eval()
# Evaluates and executes an expression x = 'print(55)' eval(x) # OUTPUT # 55
exec()
# Executes the specified code (or object) x = 'name = "John"\nprint(name)' exec(x) # OUTPUT # John
filter()
# Use a filter function to exclude items in an iterable object ages = [5, 12, 17, 18, 24, 32] def myFunc(x): if x < 18: return False else: return True adults = filter(myFunc, ages) for x in adults: print(x) # OUTPUT # 18 # 24 # 32
float()
# Returns a floating point number x = float(3) print(x) # OUTPUT # 3.0
format()
# Format the number 0.5 into a percentage value x = format(0.5, '%') print(x) # OUTPUT # 50.000000%
frozenset()
# Returns a frozenset object mylist = ['apple', 'banana', 'cherry'] x = frozenset(mylist) print(x) # OUTPUT # frozenset({'banana', 'cherry', 'apple'})
getattr()
# Returns the value of the specified attribute (property or method) class Person: name = "John" age = 36 country = "Norway" x = getattr(Person, 'age') print(x) # OUTPUT # 36
globals()
# Returns the current global symbol table as a dictionary x = globals() print(x) # OUTPUT # {'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <_frozen_importlib_external.SourceFileLoader object at 0x02A8C2D0>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, '__file__': 'demo_ref_globals.py', '__cached__': None, 'x'_ {...}}
hasattr()
# Returns True if the specified object has the specified attribute (property/method) class Person: name = "John" age = 36 country = "Norway" x = hasattr(Person, 'age') print(x) # OUTPUT # True
hash()
# Returns the hash value of a specified object
help()
# Executes the built-in help system
hex()
# Converts a number into a hexadecimal value x = hex(255) print(x) # OUTPUT # 0xff
id()
# Returns the id of an object x = ('apple', 'banana', 'cherry') y = id(x) # OUTPUT # 75686015
input()
# Allowing user input print('Enter your name:') x = input() print('Hello, ' + x) # OUTPUT # Hello, x
int()
# Returns an integer number x = int(3.5) print(x) # OUTPUT # 3
isinstance()
# Returns True if a specified object is an instance of a specified object x = isinstance(5, int) # OUTPUT # True
issubclass()
# Returns True if a specified class is a subclass of a specified object print(x)
iter()
# Returns an iterator object print(x)
len()
# Returns the length of an object mylist = ["apple", "banana", "cherry"] x = len(mylist) print(x) # OUTPUT # 3
list()
# Returns a list x = list(('apple', 'banana', 'cherry')) print(x) # OUTPUT # ['apple', banana', 'cherry']
locals()
# Returns an updated dictionary of the current local symbol table x = locals() print(x) # OUTPUT # {'__name__': '__main__', '__doc__': None, '__package__': None, '__loader__': <_frozen_importlib_external.SourceFileLoader object at 0x0327C2D0>, '__spec__': None, '__annotations__': {}, '__builtins__': <module 'builtins' (built-in)>, '__file__': 'demo_ref_globals.py', '__cached__': None, 'x'_ {...}}
map()
# Returns the specified iterator with the specified function applied to each item def myfunc(n): return len(n) x = map(myfunc, ('apple', 'banana', 'cherry')) print(x) # OUTPUT # <map object at 0x056D44F0> # [5, 6, 6]
max()
# Returns the largest item in an iterable x = max(5, 10) print(x) # OUTPUT # 10
memoryview()
# Returns a memory view object x = memoryview(b"Hello") print(x) #return the Unicode of the first character print(x[0]) #return the Unicode of the second character print(x[1]) # OUTPUT # <memory at 0x03348FA0> # 72 # 101
min()
# Returns the smallest item in an iterable x = min(5, 10) print(x) # OUTPUT # 5
next()
# Returns the next item in an iterable mylist = iter(["apple", "banana", "cherry"]) x = next(mylist) print(x) x = next(mylist) print(x) x = next(mylist) print(x) # OUTPUT # apple # banana # cherry
object()
# Returns a new object x = object() print(x) # OUTPUT # <object object at 0x14ba79ea9d70>
oct()
# Converts a number into an octal x = oct(12) print(x) # OUTPUT # 0o14
open()
# Opens a file and returns a file object f = open("demofile.txt", "r") print(f.read()) # OUTPUT # Hello! Welcome to demofile.txt # This file is for testing purposes. # Good Luck!
ord()
# Convert an integer representing the Unicode of the specified character x = ord("h") print(x) # OUTPUT # 104
pow()
# Returns the value of x to the power of y x = pow(4, 3) print(x) # OUTPUT # 64
print()
# Prints to the standard output device print("Hello World") # OUTPUT # Hello World
property()
# Gets, sets, deletes a property
range()
# Returns a sequence of numbers, starting from 0 and increments by 1 (by default) x = range(6) for n in x: print(n) # OUTPUT # 0 # 1 # 2 # 3 # 4 # 5
repr()
# Returns a readable version of an object
reversed()
# Returns a reversed iterator alph = ["a", "b", "c", "d"] ralph = reversed(alph) for x in ralph: print(x) # OUTPUT # d # c # b # a
round()
# Rounds a numbers x = round(5.76543, 2) print(x) # OUTPUT # 5.76
set()
# Returns a new set object x = set(('apple', 'banana', 'cherry')) print(x) # OUTPUT # {'apple', 'banana', 'cherry'}
setattr()
# Sets an attribute (property/method) of an object class Person: name = "John" age = 36 country = "Norway" setattr(Person, 'age', 40) # OUTPUT # 40
slice()
# Returns a slice object a = ("a", "b", "c", "d", "e", "f", "g", "h") x = slice(2) print(a[x]) # OUTPUT # ('a', 'b')
sorted()
# Returns a sorted list a = ("b", "g", "a", "d", "f", "c", "h", "e") x = sorted(a) print(x) # OUTPUT # ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h']
staticmethod()
# Converts a method into a static method
str()
# Returns a string object x = str(3.5) print(x) # OUTPUT # "3.5"
sum()
# Sums the items of an iterator a = (1, 2, 3, 4, 5) x = sum(a) print(x) # OUTPUT # 15
super()
# Returns an object that represents the parent class class Parent: def __init__(self, txt): self.message = txt def printmessage(self): print(self.message) class Child(Parent): def __init__(self, txt): super().__init__(txt) x = Child("Hello, and welcome!") x.printmessage() # OUTPUT # Hello, and welcome!
tuple()
# Returns a tuple x = tuple(("apple", "banana", "cherry")) print(x) # OUTPUT # ('banana', 'cherry', 'apple')
type()
# Returns the type of an object a = ('apple', 'banana', 'cherry') b = "Hello World" c = 33 x = type(a) y = type(b) z = type(c) # OUTPUT # tuple # str # int
vars()
# Returns the __dict__ property of an object class Person: name = "John" age = 36 country = "norway" x = vars(Person) print(x) # OUTPUT # {'__module__': '__main__', 'name': 'John', 'age': 36, 'country': 'norway', '__dict__': <attribute '__dict__' of 'Person' objects>, '__weakref__': <attribute '__weakref__' of 'Person' objects>, '__doc__': None}
zip()
# Returns an iterator, from two or more iterators a = ("John", "Charles", "Mike") b = ("Jenny", "Christy", "Monica") x = zip(a, b) print(x) # OUTPUT # (('John', 'Jenny'), ('Charles', 'Christy'), ('Mike', 'Monica'))
10
Subscribe to my newsletter
Read articles from Namya Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
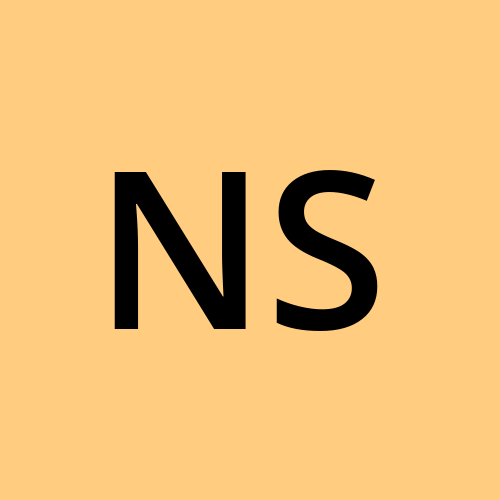
Namya Shah
Namya Shah
I am a developer who is very enthusiast about technology and coding.