React - Center a component horizontally and vertically

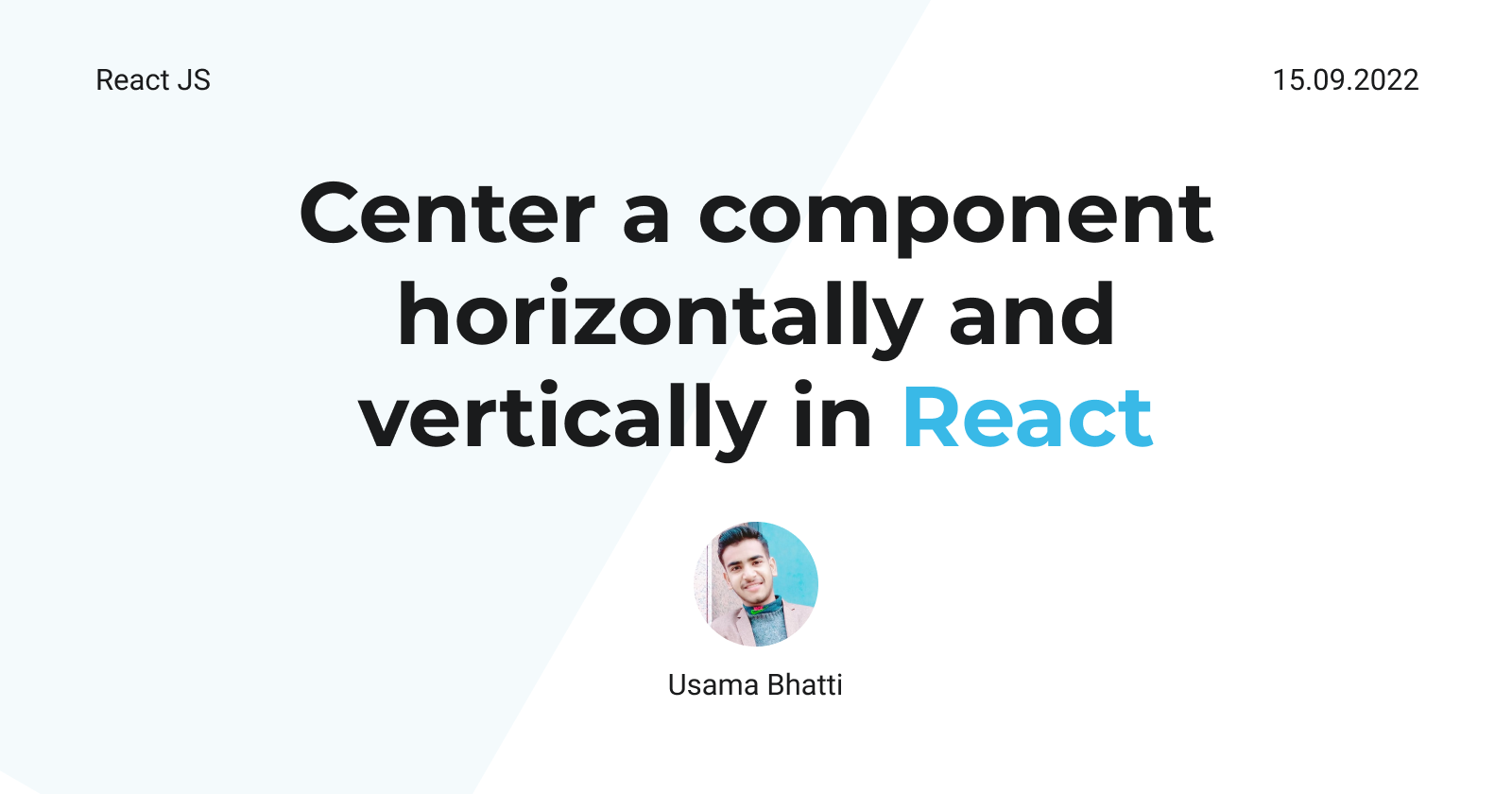
In this tutorial, we are going to learn about how to center a component horizontally and vertically in React with the help of examples.
Consider, we have the following component in our react app:
import React from 'react';
function Home(){
return (
<div className="center">
<h1>Home Component</h1>
</div>
)
}
export default Home;
Centering using simple css
To center a component horizontally and vertically in React, add the display:flex, justify-content: center and align-items: center to the react component CSS class.
‘justify-content: center’ centers the component horizontally.
‘align-items: center’ centers the component vertically.
Here is an example:
import React from 'react';
function Home(){
return (
<div className="center">
<h1>Home Component</h1>
</div>
)
}
export default Home;
.center{
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
Centering using inline css in react
or we can add it inline using the style object in React.
import React from 'react';
function Home(){
return (
<div style={{
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
height: '100vh'
}}>
<h1>Home Component</h1>
</div>
)
}
export default Home;
Centering using absolute position
We can use the absolute positioning in react.js to center the component horizontally and vertically.
Here is an example:
function Home(){
return (
<div style={{
position: 'absolute',
left: '50%',
top: '50%',
transform: 'translate(-50%, -50%)'
}}>
Home Component
</div>
)
}
export default Home;
Here we added position:absolute to the component div element, so the element breaks out from the normal document flow and is positioned to its relative parent (eg: body or parent component).
The left:50% moves the element 50% right from its position.
The top:50% moves the element 50% down from its position.
The translate(-50%, -50%) moves the element 50% up, 50% left of it’s position.
Creating the center component
We can also create a reusable center component in React, so we can reuse it in our app instead of adding the styles every time to the component.
Example:
import React from 'react';
function Center(props){
return (
<div style={{
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
height: '100vh'
}}>
{props.children}
</div>
)
}
export default Center;
Using the Center component:
import React from 'react';
import Center from './center.js'
function Home(){
return (
<div>
<Center>
<h1>Home Component</h1>
</Center>
</div>
)
}
export default Home;
Subscribe to my newsletter
Read articles from Usama Bhatti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
