Discover Javascript Arrays
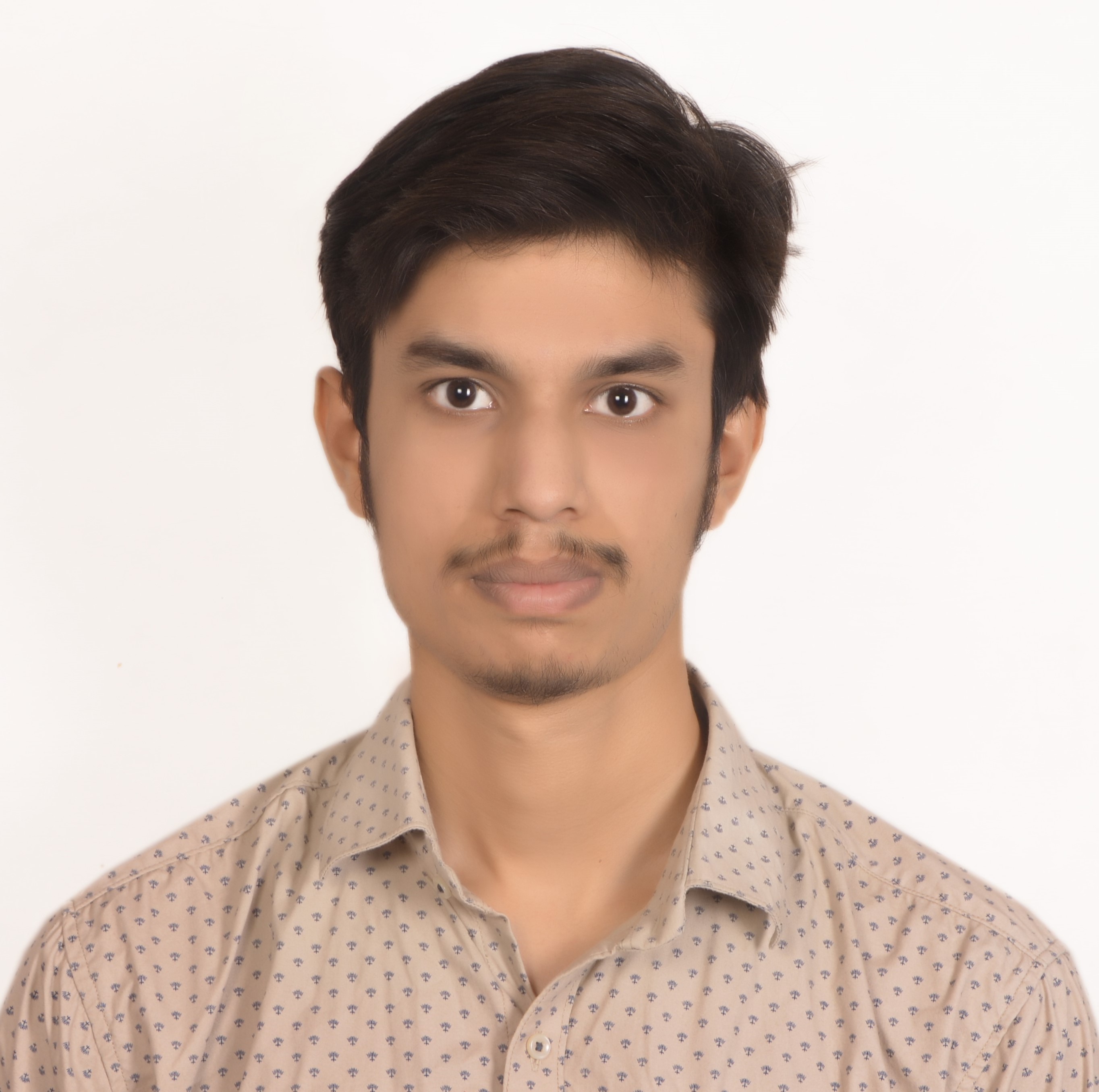
3 min read
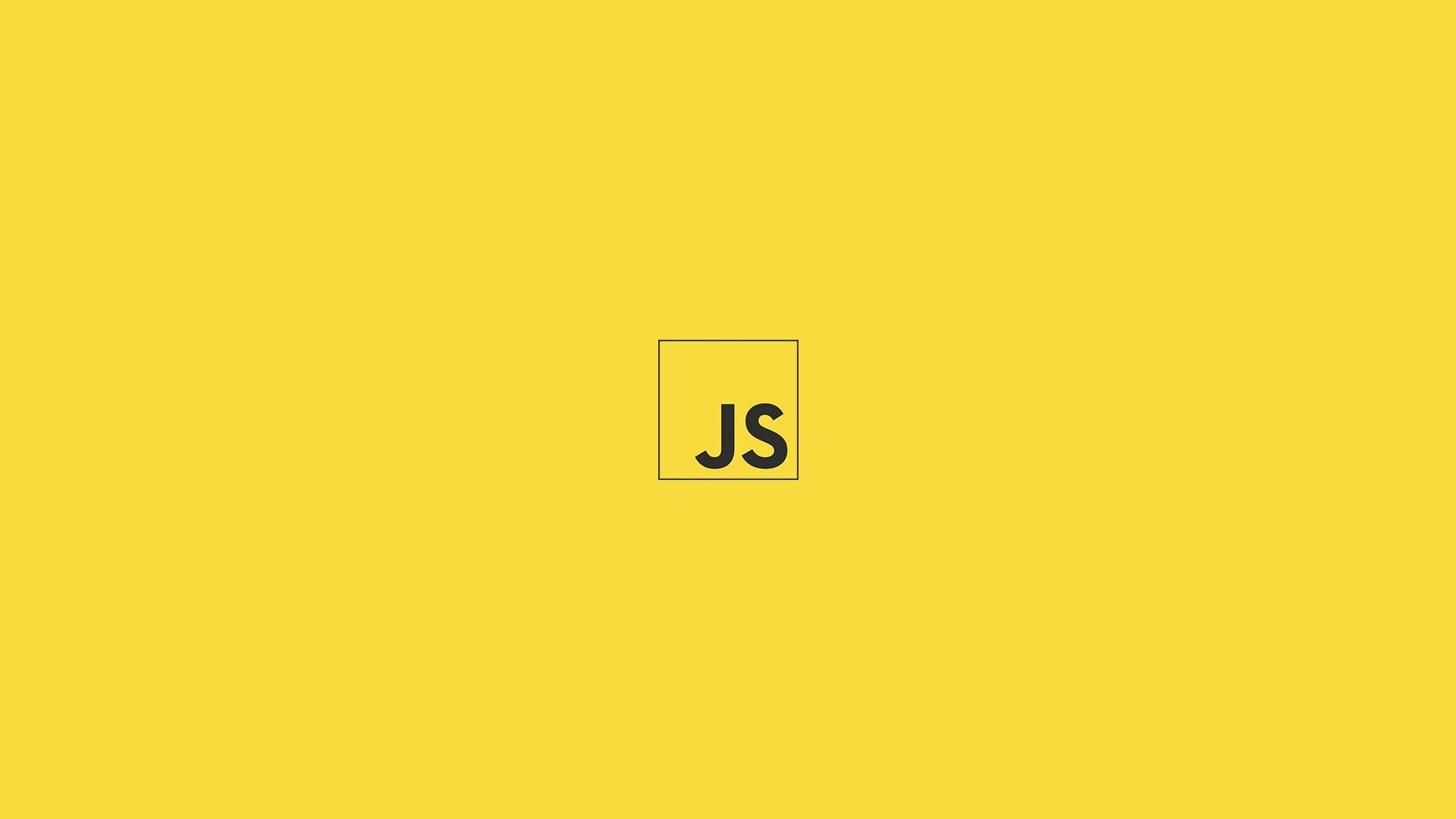
In Javascript world arrays is referred to as an Object , hence it can hold different datatypes inside it.
Advantages of Array
- Arrays represent multiple data items of the same type using a single name
- In arrays, the elements can be accessed randomly by using the index number
- Arrays allocate memory in contiguous memory locations for all its elements
There are 3 ways to construct array in JS.
By array Literal
The syntax of creating array using array literal is given below:
let arr1 = ['a' , 'b' , 3 , 4]
By using 'new' keyword
JavaScript array constructor (new keyword)
Indexing in Array
JavaScript arrays are zero-indexed: the first element of an array is at index 0 , the second is at index 1 , and so on — and the last element is at the value of the array's length property minus 1
So you can see here the first value of an arrays has index 0 , second value has index 1 and so on.
Last index = array.length - 1
Methods to Manupulate Array
- .concat() Consider we have 2 different arrays , arr1 and arr2. So if we want to join two arrays and save those values in new array then we use this method.
- .sort() The sort() sorts the elements of an array. The sort() overwrites the original array. The sort() sorts the elements as strings in alphabetical and ascending order.
- .map() map() creates a new array from calling a function for every array element. map() calls a function once for each element in an array. map() does not execute the function for empty elements. map() does not change the original array.
- .slice() The slice() method returns selected elements in an array, as a new array. The slice() method selects from a given start, up to a (not inclusive) given end. The slice() method does not change the original array.
- .splice() The splice() method adds and/or removes array elements. The splice() method overwrites the original array.
- .fill() The fill() method fills specified elements in an array with a value. The fill() method overwrites the original array. Start and end position can be specified. If not, all elements will be filled.
- .toString() The toString() method returns a string with array values separated by commas. The toString() method does not change the original array.
code

result

code

result

code

result

code

result

syntax
array.splice(index, howmany, item1, ....., itemX)
code

result

syntax
array.fill(value, start, end)
code

result

syntax
array.toString()
code

result

0
Subscribe to my newsletter
Read articles from Swaroop Dharmadhikari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
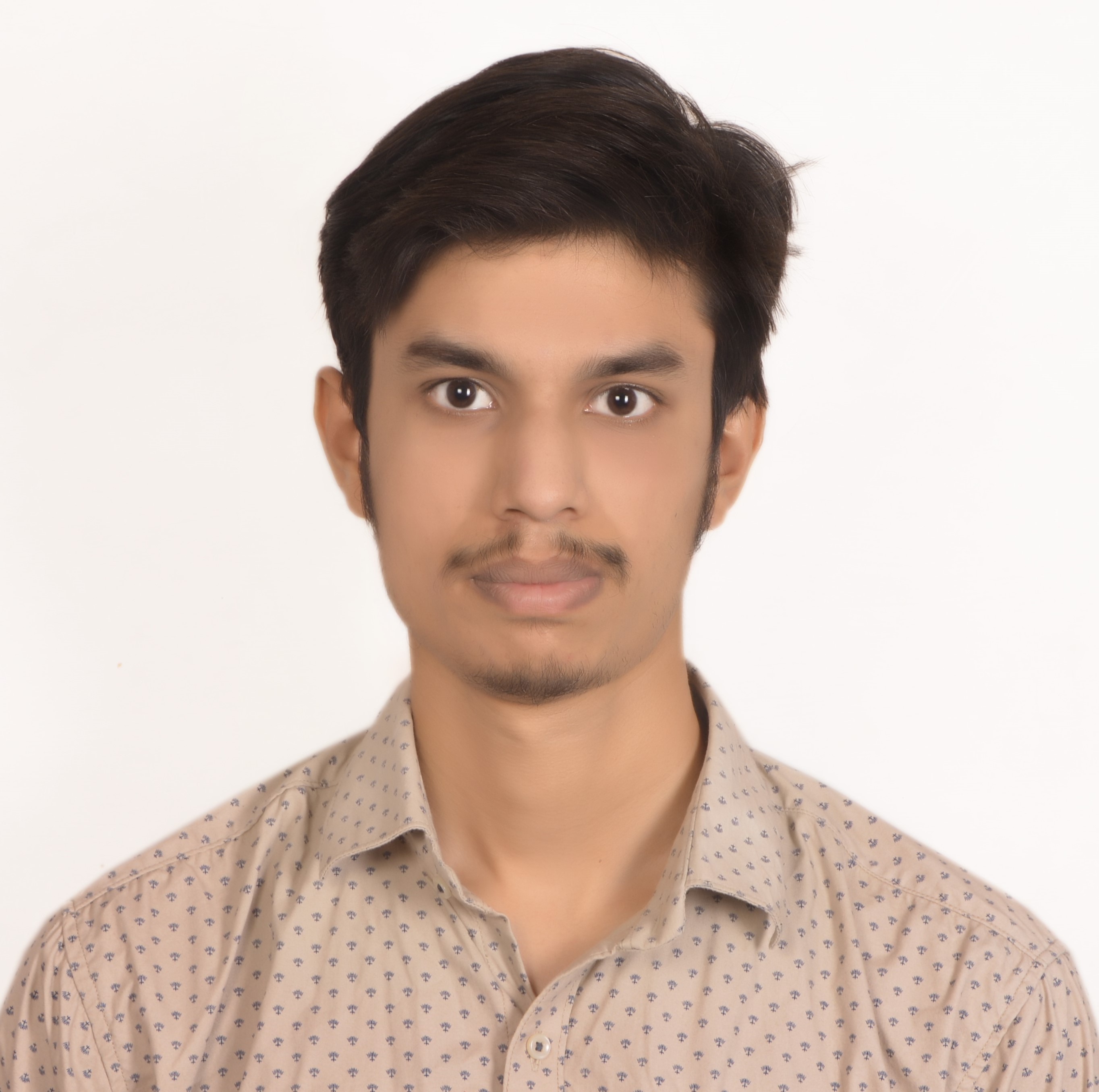