Data Structures and Algorithms (Day-1 #I4G10DaysOfCodeChallenge)

Table of contents
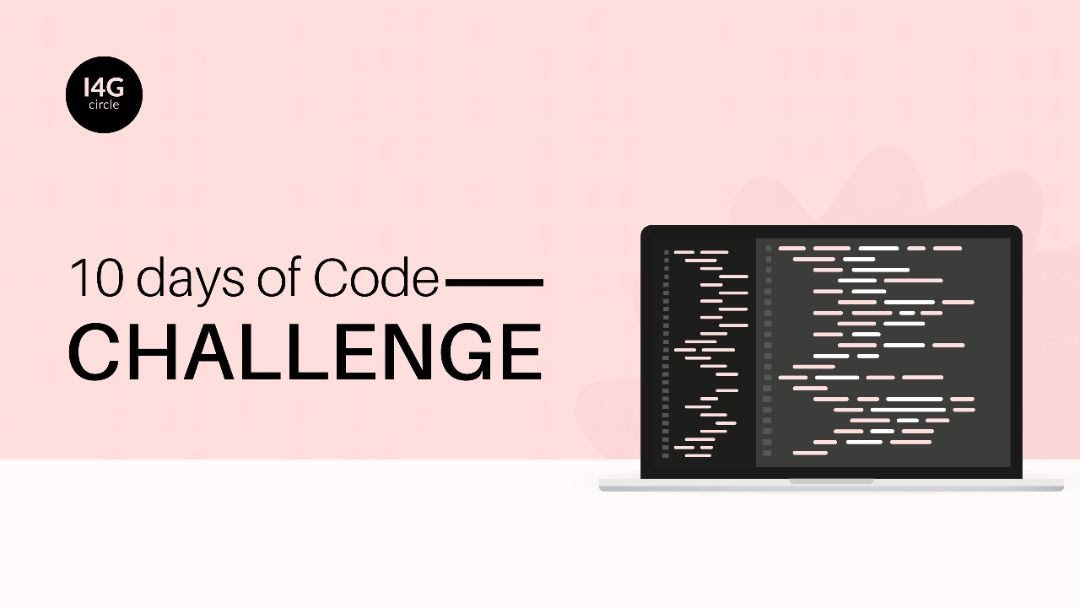
Understanding the question(challenge)
The first process of solving this challenge is understanding the question. Understanding the question gave me basis for me to start trials.
Question:
Given an integer array nums sorted in non-decreasing order, remove the duplicates in-place such that each unique element appears only once. The relative order of the elements should be kept the same.
Since it is impossible to change the length of the array in some languages, you must instead have the result be placed in the first part of the array nums. More formally, if there are k elements after removing the duplicates, then the first k elements of nums should hold the final result. It does not matter what you leave beyond the first k elements.
Return k after placing the final result in the first k slots of nums.
Do not allocate extra space for another array. You must do this by modifying the input array in-place with O(1) extra memory.
Click Here to view the full question alongside example cases.
Solution Process
Like I said earlier, the first step was understanding the question, which did imply that the output array must only contain unique items and also the input array must not be altered/replaced with another array. From this understanding, here are the processes taken to solve the challenge:
- First declare and initialize a counter variable to zero. Ensure that this variable is not a constant as the values changes during the program.
- Next, loop through the input array with ( I used a for loop). The looping should start an index of 1.
- Then start comparing the values of the input array. The comparison starts with the initial value and the next values.The initial value will be of index 0 and the following 1. The counter increases as the scope-chained i index in the for loop also increase.The condition for comparison are as follows:
- if current value is equal to next value or if the current value is equal to the previous value If the above condition is met, the current value is remove from the input array (in JavaScript using .splice())
- Another for loop is then inserted into the current if statement to check if there are any further duplicates. This current for loop ,loops through the modified input array and checks for backward duplicates again with an if statement. Then splices any found duplicates from the modified input array.
- The counter increments at any stage of the outer for loop.
- The function then returns an array of on unique items. Below is a description of the code
var removeDuplicates = (input_array){
declare and initialize counter to zero
for(let i = 1; ...){
if (compares current item with next item or previous item) {
splicing is done
for (let j = 0; ...) {
if (compares current item with previous item) {
splicing is done
}
}
counter increases
}
}
logs modified input array
}
Proof of my solution is here:
Subscribe to my newsletter
Read articles from Victor Ukoha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Victor Ukoha
Victor Ukoha
Student | Backend Developer (Nodejs)