How to install Tailwind CSS in a new Ember app
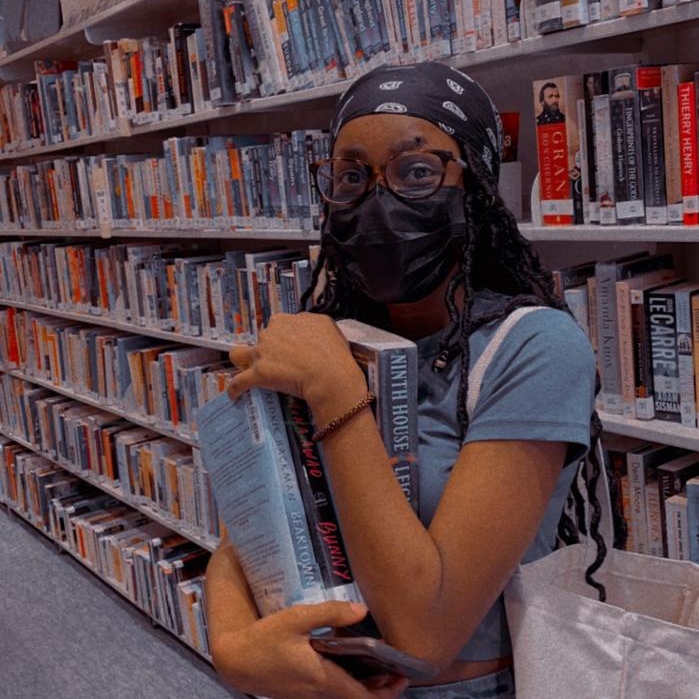
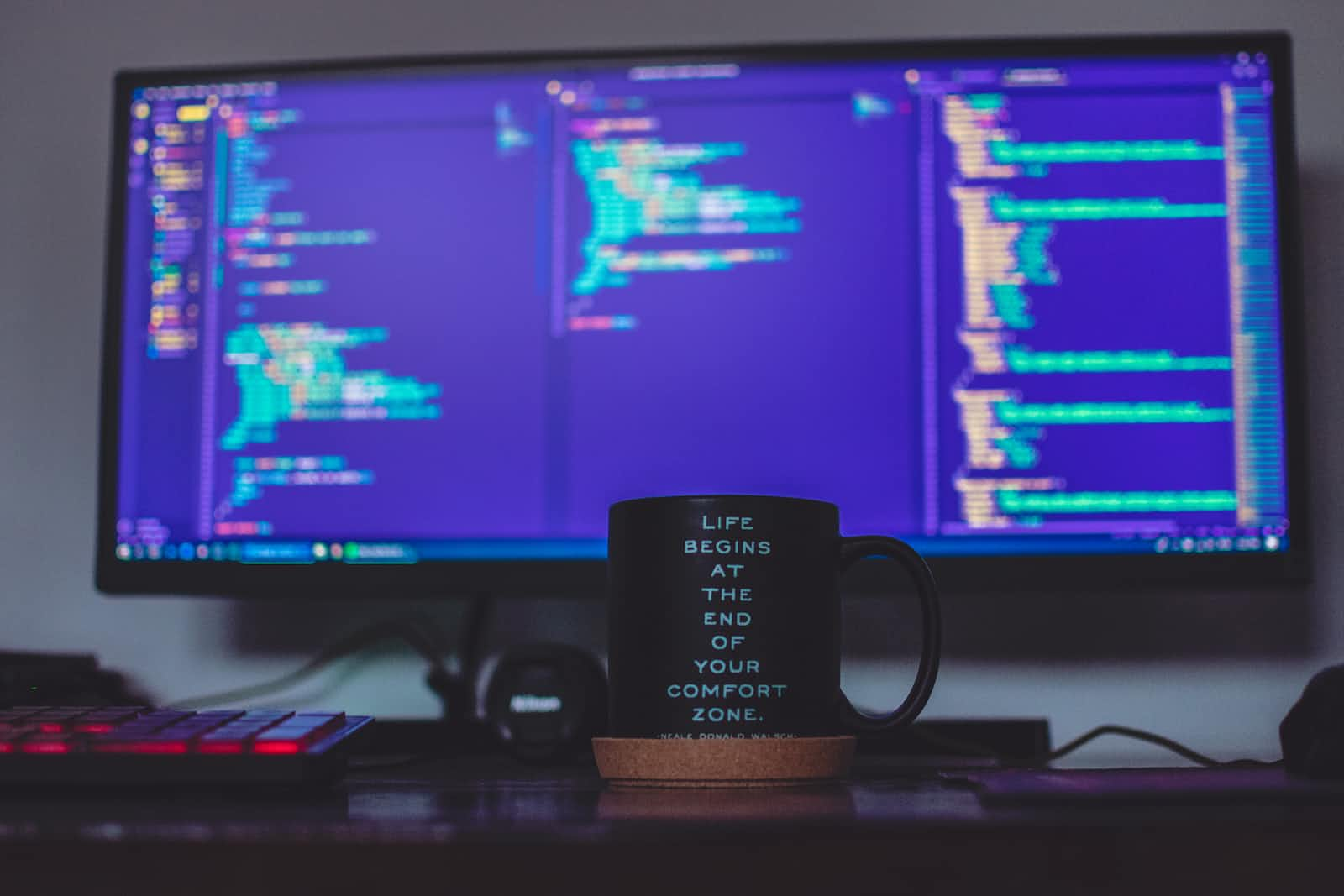
If you're building an Ember application and you want to use Tailwind CSS for your styling, here's a quick guide on how to do that. Follow these steps below to wire up Tailwind CSS in your application:
Run this command in your current app folder:
npm install tailwindcss autoprefixer --save-dev
Next, you'll want to install the PostCSS add-on like so:
ember install ember-cli-postcss
After that, create a Tailwind config folder inside your styles folder like so:
mkdir app/styles/tailwind
Add a
config.js
file inside the Tailwind folder withnpx tailwind init app/styles/tailwind/config.js
. Once you've got that, add this inside your config file:
module.exports = {
content: ['./app/**/*.hbs'],
theme: {
extend: {},
},
plugins: [],
}
- Next, you'll want to update your app.css file by adding this:
@tailwind base;
@tailwind components;
@tailwind utilities;
- Finally, you will want to go into your
ember-build-cli.js
file and edit that to look like so:
'use strict';
const EmberApp = require('ember-cli/lib/broccoli/ember-app');
const autoprefixer = require('autoprefixer');
const tailwind = require('tailwindcss');
module.exports = function (defaults) {
let app = new EmberApp(defaults, {
postcssOptions: {
compile: {
// track changes in template, css, scss, and tailwind config files
cacheInclude: [/.*\.(css|scss|hbs)$/, /.tailwind\/config\.js$/],
plugins: [
{
module: autoprefixer,
options: {},
},
{
module: tailwind,
options: {
config: './app/styles/tailwind/config.js',
},
},
],
},
},
});
return app.toTree();
};
And that's it! You've got Tailwind wired up in your Ember application now and can use any style classes you want.
Thank you for reading! :)
Subscribe to my newsletter
Read articles from Debbie Otuagomah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
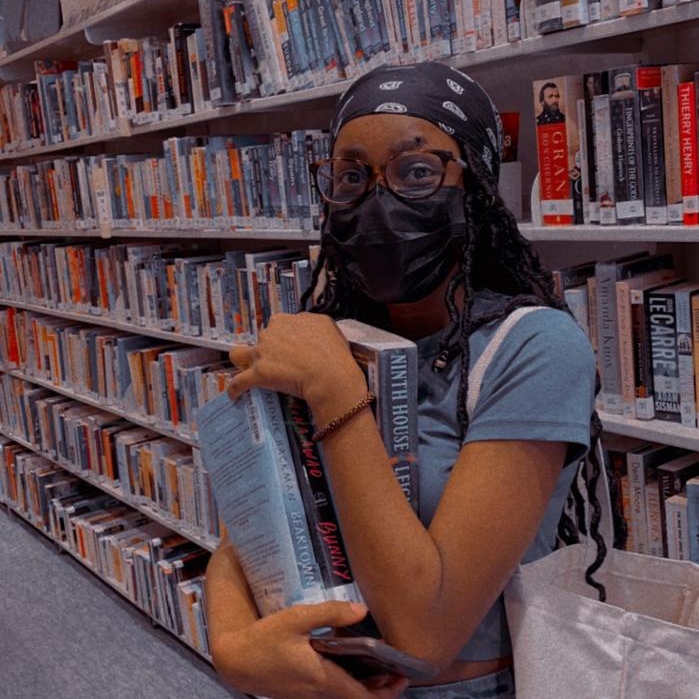
Debbie Otuagomah
Debbie Otuagomah
Hi! I'm Debbie and I'm currently building an array of frontend engineering skills by learning in public. Here's where I write helpful notes about everything JavaScript for my future self.