Mint an NFT using the Hedera Token Service
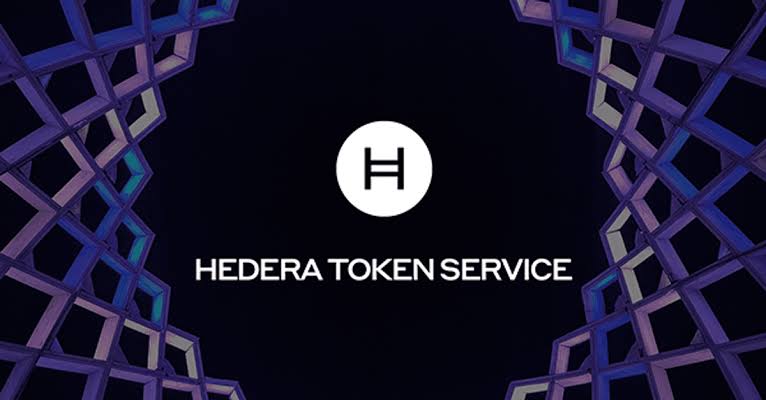
Ready to launch your NFT collection on the Hedera network? The Hedera Token Service give you the power to set up, mint and manage your tokens. And the best part is you don't need to write a smart contract and deploy it.
In this article, we will learn how to:
- Set royalties for our NFTs
- Create a Non-Fungible Token
- Mint an NFT
Go through these getting started resources before following along with this article. It explains how to create a hedera testnet account and set up your development enviroment.
Set Royalty Fees
Whenever a token is sold or transferred, a certain percentage of the fee paid for the token is collected. This is know as a royalty fee.
// Set Royalty Fee
let nftRoyaltyFee = await new CustomRoyaltyFee()
.setNumerator(4)
.setDenominator(10)
.setFeeCollectorAccountId(treasuryId)
.setFallbackFee(new CustomFixedFee().setHbarAmount(new Hbar(200)));
In the code snippet above, we have set the royalty fee to be 0.4 Hbar tokens.
Create a Non-Fungible Token
For this section, it is important to have the images ready for the NFT collection. We will also need the metadata ready too. The metadata and images need to be stored on IPFS or any alternative decentralized storage.
When the files are stored on IPFS, you will be provided with a CID (Content Identifier). The CID is in this format:
"ipfs://bafyreiax45o6ardirmfk6ua37vbnlkgpvwklntlqh5z5ohsby3tk5ep7oe/metadata.json"
Here is a sample of how the metadata should look:
{
"name": "art1.jpg",
"creator": "Jonstance",
"description": "Tribal Art",
"type": "image/jpg",
"format": "none",
"properties": {
"character": "Villager",
"theme": "War",
"level": "soldier"
},
"image": "ipfs://rjfybeghg35bheyqpi4qlnuljpok54u7h3bnp62fe6jit343hv3oxhgnbfm/art1.jpg"
}
Here are the CIDs for the metadata:
// IPFS Content Identifiers To Create NFTs
CID = [
"ipfs://gafyreie3ichhf4l4xa7e6xcy34tylbuu7763gnjf7c55trg3b6xyjr4bku/metadata.json",
"ipfs://gafyreicfldhlnndodwm4xecyy4j3s5u7kh88b5rvcyq4jao7f6kjttuvu/metadata.json",
"ipfs://gafyreia6ow6phsk7gdefzlgagfamxmujp47ue7rxymo6wr2qtenrzaeu/metadata.json"
];
Next, to create the token, we will use TokenCreateTransaction() to set the properties of the NFT.
// Create NFT with Royalty
let createNFT = await new TokenCreateTransaction()
.setTokenName("The Trybe")
.setTokenSymbol("TRYBE")
.setTokenType(TokenType.NonFungibleUnique)
.setDecimals(0)
.setInitialSupply(0)
.setTreasuryAccountId(treasuryId)
.setSupplyType(TokenSupplyType.Finite)
.setMaxSupply(CID.length)
.setCustomFees([nftRoyaltyFee])
.setAdminKey(adminKey)
.setSupplyKey(supplyKey)
.setPauseKey(pauseKey)
.setFreezeKey(freezeKey)
.setWipeKey(wipeKey)
.freezeWith(client)
.sign(treasuryKey);
let nftCreateTxSign = await nftCreate.sign(adminKey);
let nftCreateSubmit = await nftCreateTxSign.execute(client);
let nftCreateRx = await nftCreateSubmit.getReceipt(client);
let tokenId = nftCreateRx.tokenId;
console.log(`Created NFT with Token ID: ${tokenId} \n`);
// TOKEN QUERY TO CHECK THAT THE CUSTOM FEE SCHEDULE IS ASSOCIATED WITH NFT
var tokenInfo = await new TokenInfoQuery().setTokenId(tokenId).execute(client);
console.table(tokenInfo.customFees[0]);
Mint an NFT
What is the use of creating an NFT if you won't mint it? That is the next stage. We will mint a batch of NFTs for our NFT collection. We will using a for loop to make the process faster.
// Mint NFTs
nftTrybe = [];
for (var i = 0; i < CID.length; i++) {
nftTrybe[i] = await tokenMinterFunction(CID[i]);
console.log(`Created NFT ${tokenId} with serial: ${nftTrybe[i].serials[0].low}`);
}
Here is our Token Minter function:
// Token Minter Function
async function tokenMinterFunction(CID) {
mintTx = await new TokenMintTransaction()
.setTokenId(tokenId)
.setMetadata([Buffer.from(CID)])
.freezeWith(client);
let mintTxSign = await mintTx.sign(supplyKey);
let mintTxSubmit = await mintTxSign.execute(client);
let mintRx = await mintTxSubmit.getReceipt(client);
return mintRx;
}
And that's how we Mint an NFT on the Hedera network using the Hedera Token Service. You can see that creating and minting NFTs on the Hedera network is pretty easy. And the best part is you dont have to write a smart contract. If you have any questions regarding the article, feel free to share with me. I'll love to see them.
Subscribe to my newsletter
Read articles from Constance Osarodion Etiosa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by