Event Bubbling v/s Event Capturing

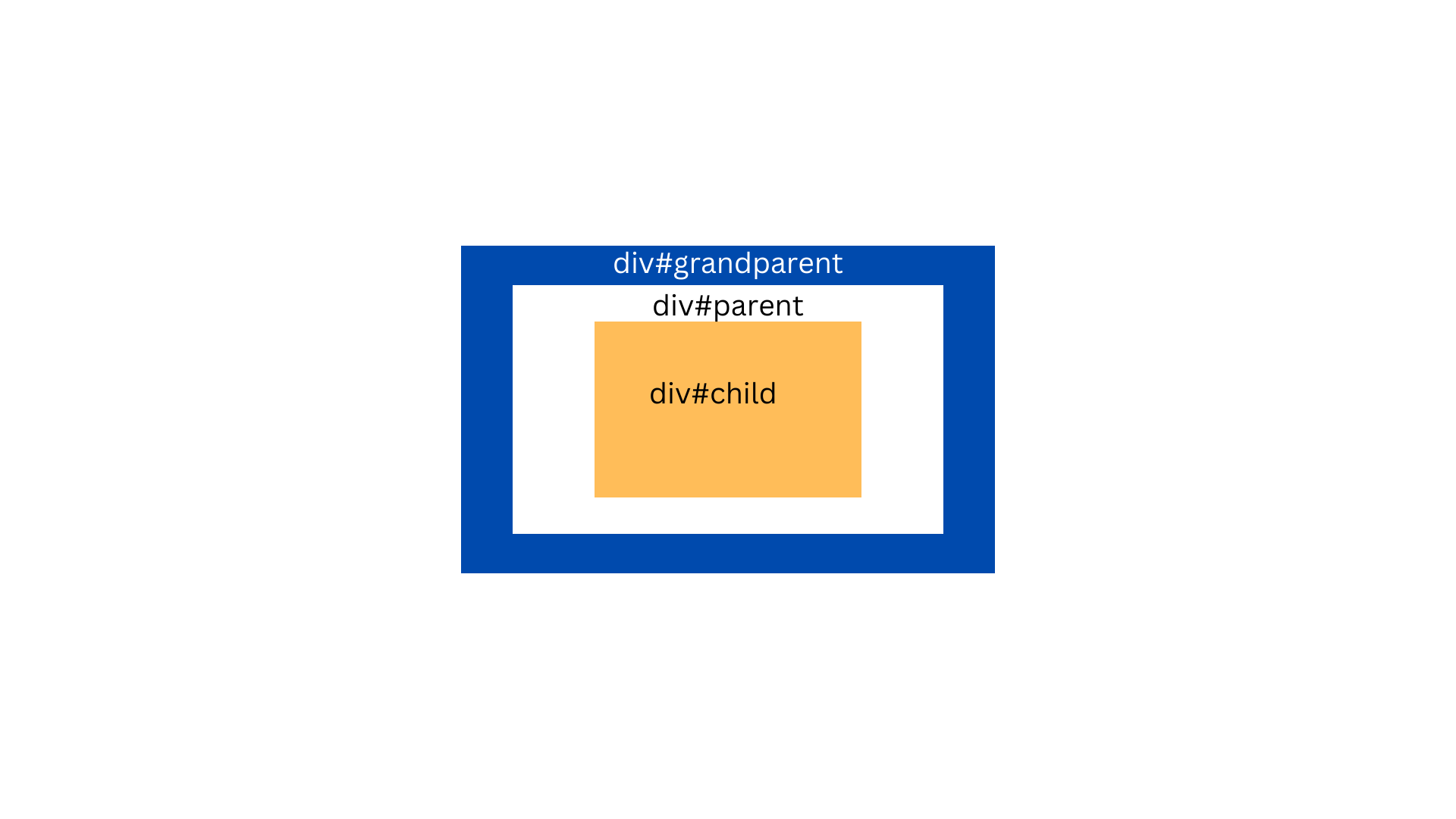
We will understand event listeners before understanding event bubbling & event capturing. An event listener is nothing but a function that gets executed when an event happens and that event can be anything like onclick event, onchange event, pressing key, etc.
How does Event Bubbling & Capturing work?
If we have a nested HTML element and every HTML element has an event attached. Now, what will happen if I click on the child element? This event will trigger onclick parent handler & onclick grand parent handler events too. This happens because of event bubbling.
Did not get it? No worries.
Let's understand by code
//html code
<div id="grandparent">grandparent
<div id="parent">parent
<div id="child"> child</div>
</div>
</div>
//javascript code
document.querySelector("#grandparent").addEventListener("click", () => {
console.log("grandparent");
});
document.querySelector("#parent").addEventListener("click", () => {
console.log("parent");
});
document.querySelector("#child").addEventListener("click", () => {
console.log("child");
});
- If we click on child event, the output will be
child, parent & grandparent
. Try yourself. This is happening because of the event bubbling. When the child div is clicked the parent & the grandparent div are indirectly clicked. Thus, event propagation is moving from inside to outside in the DOM or we can say the events are getting bubbled up.
That's why the propagation from the closest to the element which is far away in the DOM is called event bubbling.
Event Capturing is just opposite the event bubbling. In event capturing, the event will happen upward downside. Suppose we click on the grandparent element then the parent and child div click event will indirectly be triggered.
And, how this will happen?
Simply by just setting the useCapture value to true. And your code will look like this:
//javascript code
document.querySelector("#grandparent").addEventListener("click", () => {
console.log("grandparent");
}, true);
document.querySelector("#parent").addEventListener("click", () => {
console.log("parent");
}, true);
document.querySelector("#child").addEventListener("click", () => {
console.log("child");
}, true);
For more understanding, see what’s happening behind the scene
- Open your console
- Click on the element tool & then on any event in HTML code
- Now, go to the event listener tag and you will see that by default useCapture is false. But showing true because we set value as a true in the above code. That’s the reason event bubbling happens by default
See the image
How to stop event bubbling and event capturing?
We have just learned that all this happens because of the event propagation. So, to stop event bubbling & event capturing we have to stop event propagation. And our code will look like this:
document.querySelector("#grandparent").addEventListener("click", (event) => {
console.log("grandparent");
event.stopPropagation();
});
document.querySelector("#parent").addEventListener("click", (event) => {
console.log("parent");
event.stopPropagation();
});
document.querySelector("#child").addEventListener("click", (event) => {
console.log("child");
event.stopPropagation();
});
Conclusion
- Event bubbling means propagation of event happens from child element to ancestor element in DOM and event capturing means propagation of event happens from ancestor element to child element
- We can stop event propagation using
stop.propagation()
method
Hopefully, this article helped you learn about how event bubbling & event capturing. But, to make your understanding strong, write the code by yourself and use the debugger to check how your code works behind the scene. You can connect with me on LinkedIn and on GitHub
Subscribe to my newsletter
Read articles from Savita Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Savita Verma
Savita Verma
Everything you've ever wanted is on the other side of fear." - George Addair This quote sums up the significant pivot I have made in my professional life from the comfort of being in the not technical profession to getting on the roller coaster ride of programming and bugs. Prior to that, I was helping the CEO of NavGurukul in communication with students and partner companies & organisations. Built a few dashboards to help in students' academic, team productivity, and logistics work. Worked in strategy building for the volunteer and CodeStar program of NavGurukul.