Conditional statements in JavaScript

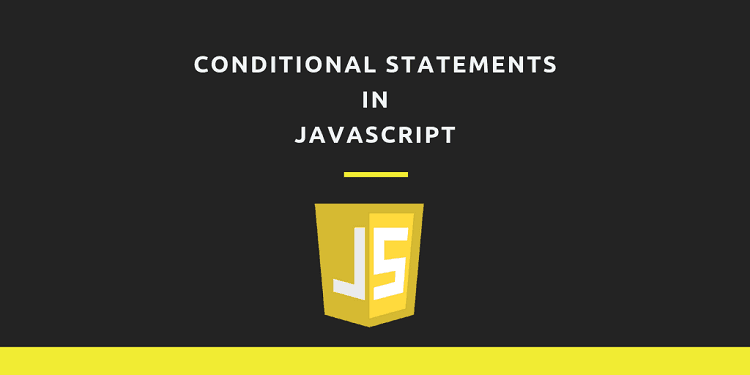
What are conditional statements in JavaScript?
In JavaScript conditional statement is used to execute one or more block of code based on a particular condition. Each condition works on a Boolean value that can be true or false.
Types of conditionals in JavaScript
if
In JavaScript if statement allows to execute a code block when the condition returns true.
Syntax :
if (condition) {
//Code be run when the condition is truthy
}
In the below example we have use two equality operator == and === where == is loosely equality operator just check the value where as === checks the data type as well so it tight equality operator.
Below example first if statement will be true second will be false.
Example :
const a = 3
if(a == '3')
{
console.log("True using ==")
}
if(a === '3')
{
console.log("True using ===")
}
if-else
An if-else statement executes one block if its condition is true and the other block if it is false.
Syntax :
if (condition) {
// If the condition is a truthy value, this code block will run
} else {
// If the condition is a falsie value, this code block will run
}
Example :
const a = 3
if(a === '3')
{
console.log("True using ==")
}
else
{
console.log("True using ===")// This gets executed
}
else-if
else-if statement executes the block that matches one among several conditions, or a default else block if no conditions are matched.
Syntax :
if (condition) {
// If the condition is truthy, this code block will run, and code execution will stop.
} else if (condition_2) {
// If the first condition is falsy, this code block will run if condition_2 will truthy.
} else if (condition_n) {
// If the previous conditions are both falsy, this code block will run if condition_n is truthy
} else {
// If all conditions are falsy, this code block will run
}
Example :
const a = 3
if(a === '3')
{
console.log("True using ==")
}
else if(a == 3)
{
console.log("True using ===")// This gets executed
}
else{
console.log("Not found")
}
Switch
Switch statement takes a input variable and tries to find the matching input variable with its all cases, which ever cases gets matched it executes block of code for matched case. If none of the cases matches a default case code block will be executed.
Syntax :
switch (expression) {
case 'first-case':
// executes code if the expression matches this case
break;
case 'case_2':
// executes code if the expression matches this case
break;
default:
// executes code if the expression doesn't match any case
}
Example :
const a = 3;
switch(a)
{
case 1: console.log('1')
break;
case 2: console.log('2')
break;
case 3: console.log('3')//gets executed
break;
default: console.log('not found')
}
Ternary Operator
Ternary operator is more like a if-else statement.
Syntax :
statement ? statement1: statement2;
In the above syntax if the statement value is true then statement1 will be executed if false statement2 will be executed
Example :
const a = 3;
a == 4 ? console.log('True') : console.log('False') //False will be printed
Subscribe to my newsletter
Read articles from Vignesh .V directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
