Increment and decrement in solidity
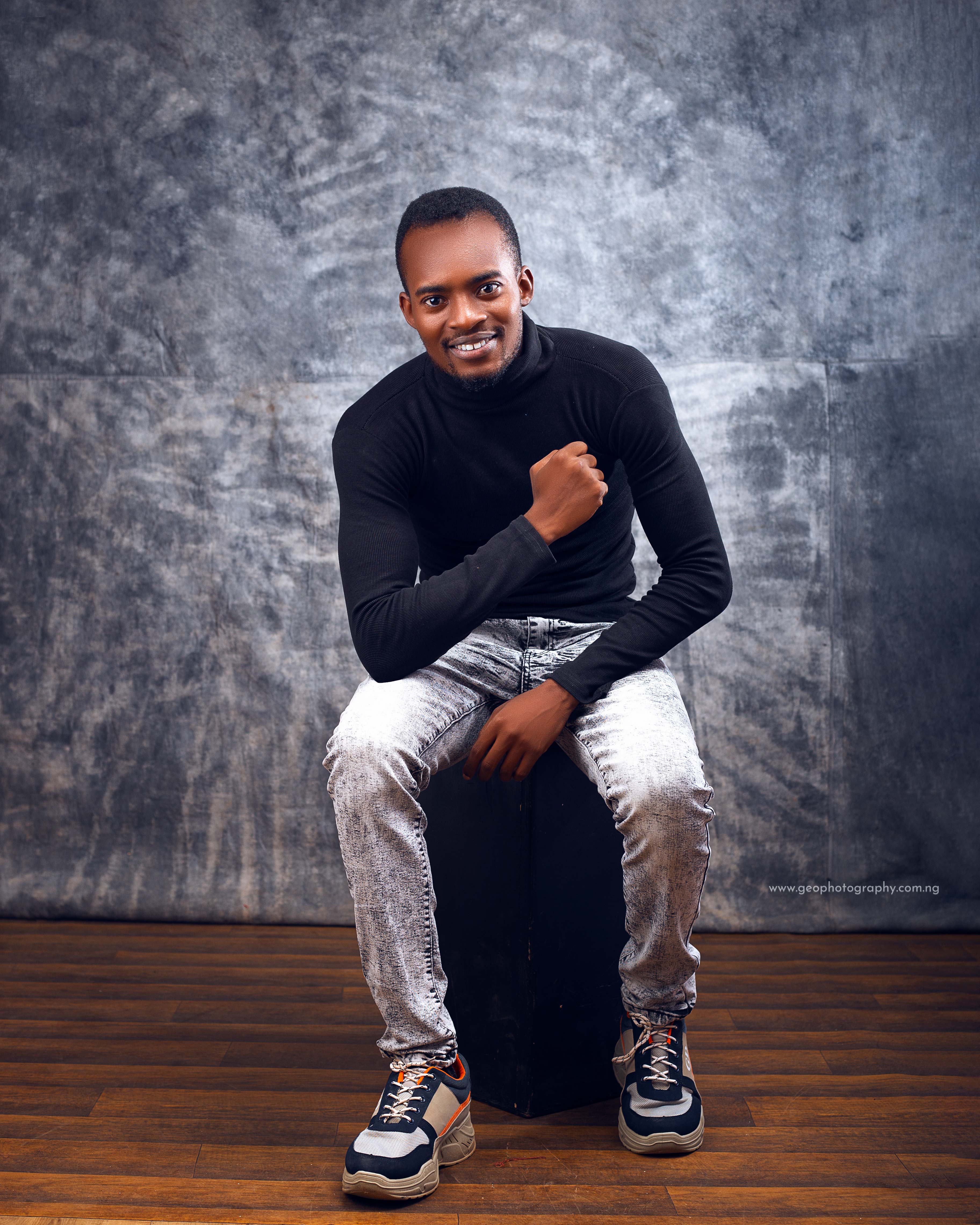
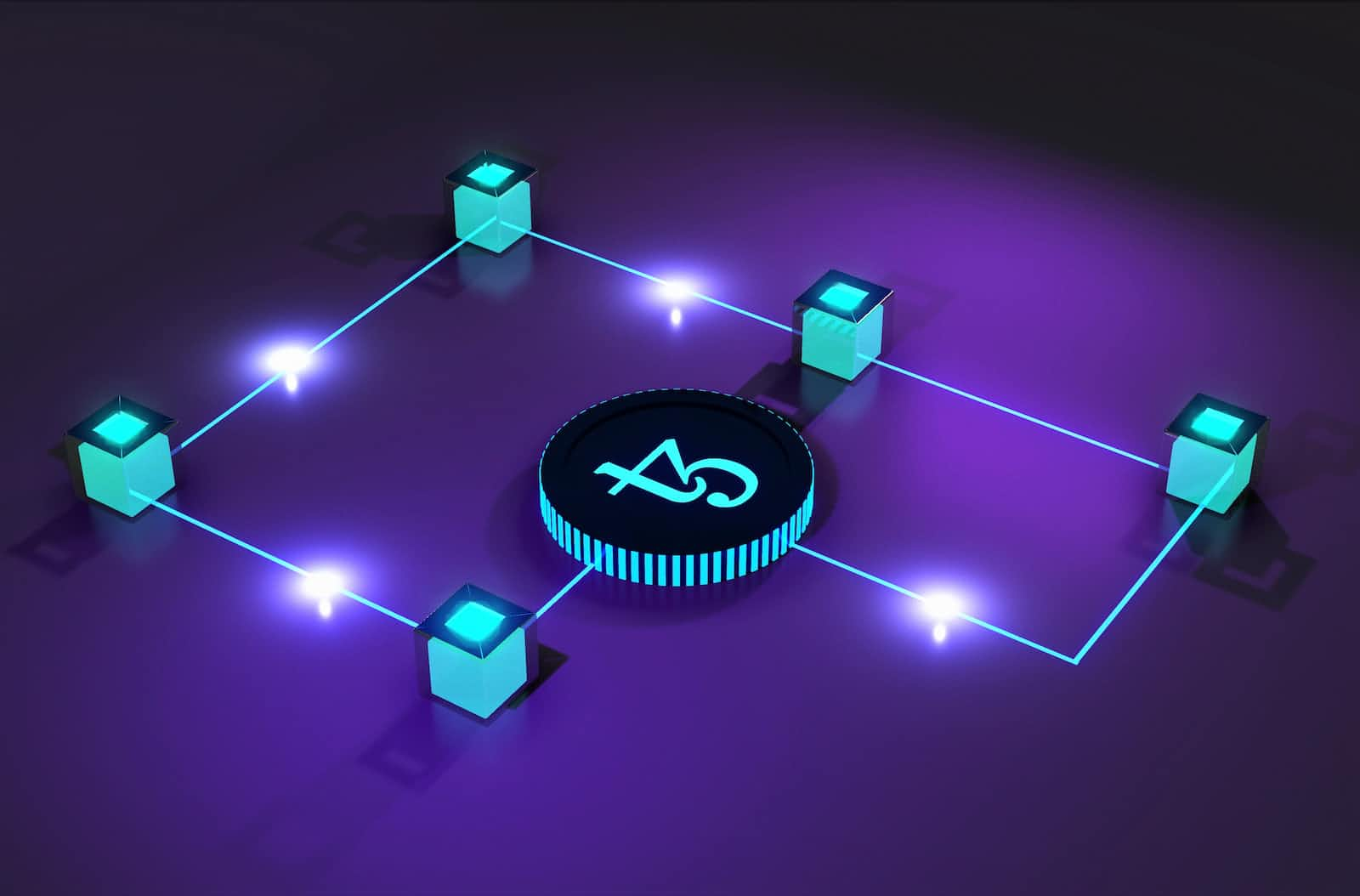
Hello guys, in this article, I will be showing us how to increment and decrement in solidity. First step is to declare the solidity compiler version followed by contract with name Michael.
pragma solidity ^0.5.0;
contract Michael{
}
Next step is to declare variable count with variable type uint and then set the visibility to public.
pragma solidity ^0.5.0;
contract Michael{
uint public count;
}
Brilliant, you are getting it.
Now let declare function called increase, set visibility to public
function increase() public {
}
Put this inside the function.
count +=1;
It simply means that the state variable count will be increased by 1 anytime the function increase is called.
There is another method which is method 2 to achieve thesame result as above code.
count = count +1;
Note: you cannot use the two methods together, you have to choose one.
See the full code below.
pragma solidity ^0.5.0;
contract Michael{
uint public count;
function increase() public {
count +=1;
}
}
We are done with increase function, Next is to crease function called decrease and set it to public.
function decrease() public {
}
Since we want to decrease count by 1, the code that will be inside the decrease function will be.
count -= 1;
The above function will decrease the value of count variable by 1. Another way of writing it is
count = count -1;
Note: only pick on of the methods, do not use the two together.
function decrease() public {
count -= 1;
}
Here is our full code.
pragma solidity ^0.5.0;
contract Michael{
uint public count;
function increase() public {
count +=1;
}
function decrease() public {
count -= 1;
}
}
Let compile and deploy. To compile, press control + s , look for deploy button and deploy to remix testnet.
Immediately you will see count, increase and decrease.
If you click on count, the result will be zero. If you click on increase, the value of count will increase by one and vise-versa.
Note: you can set count to a default value like this
uint public count =100;
Subscribe to my newsletter
Read articles from Michael Fawole directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
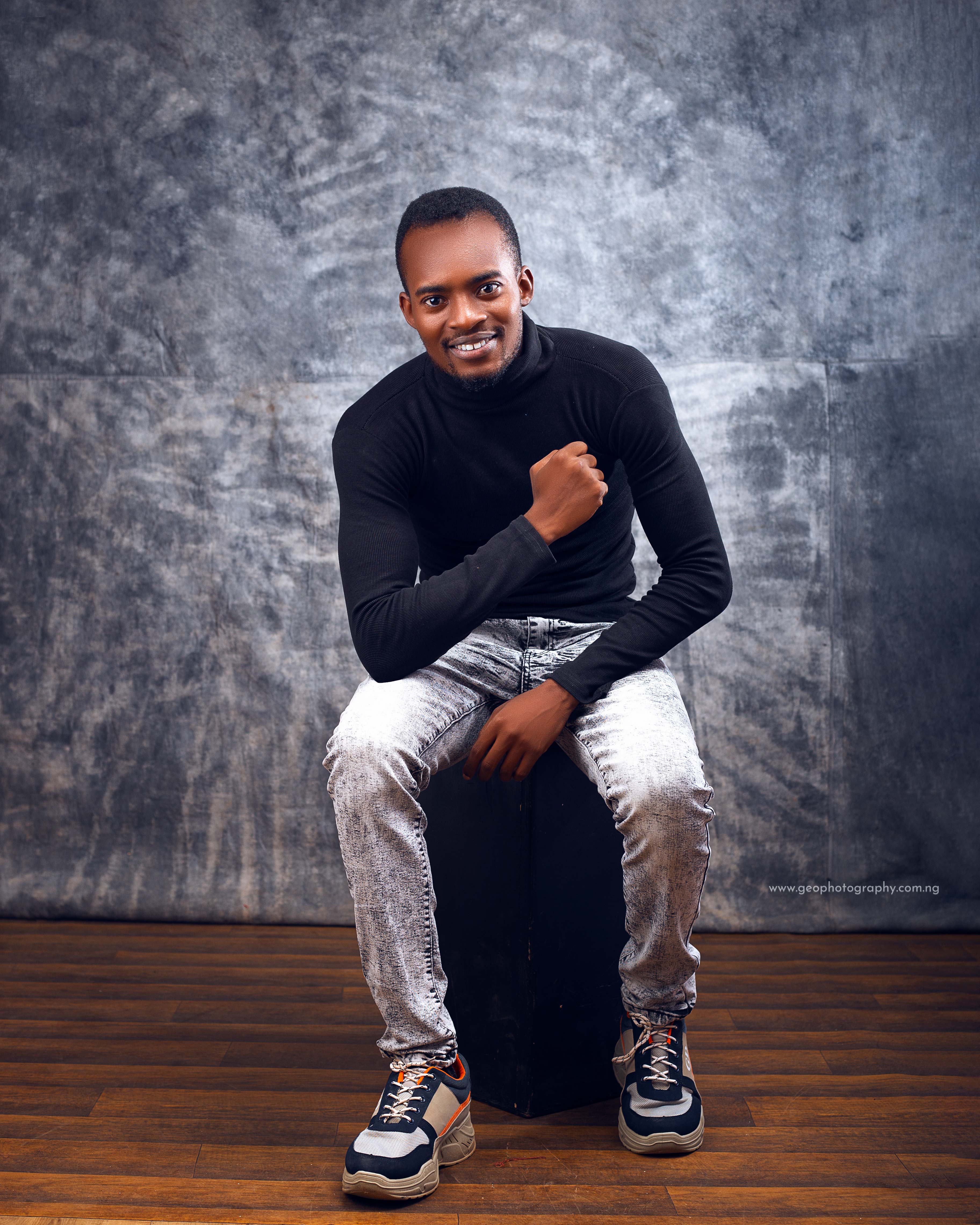
Michael Fawole
Michael Fawole
Michael Fawole is a Blockchain and web application developer with 7+ years of experience and 3+ years plus of experience in blockchain dapp development. He is also delivering training on blockchain ( Solidity, web3 and react js ) and php language. He also helps start-ups and companies to setup blockchain practice and incorporating blockchain into existing project. Techstack (Blockchain): Solidity, web3 js and react js. Techstack (Webapp): Php and Mysql.