Referral system with php and mysql
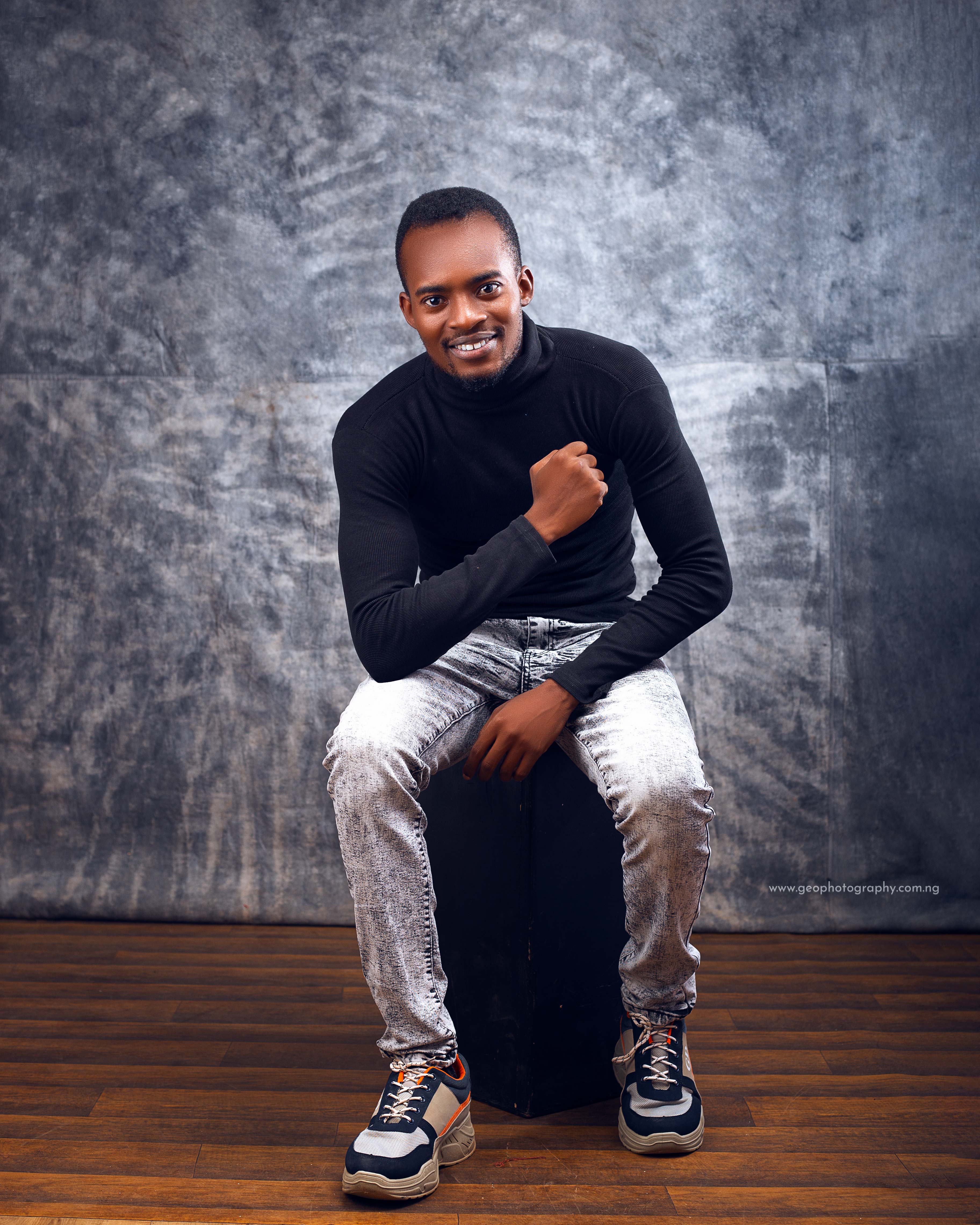
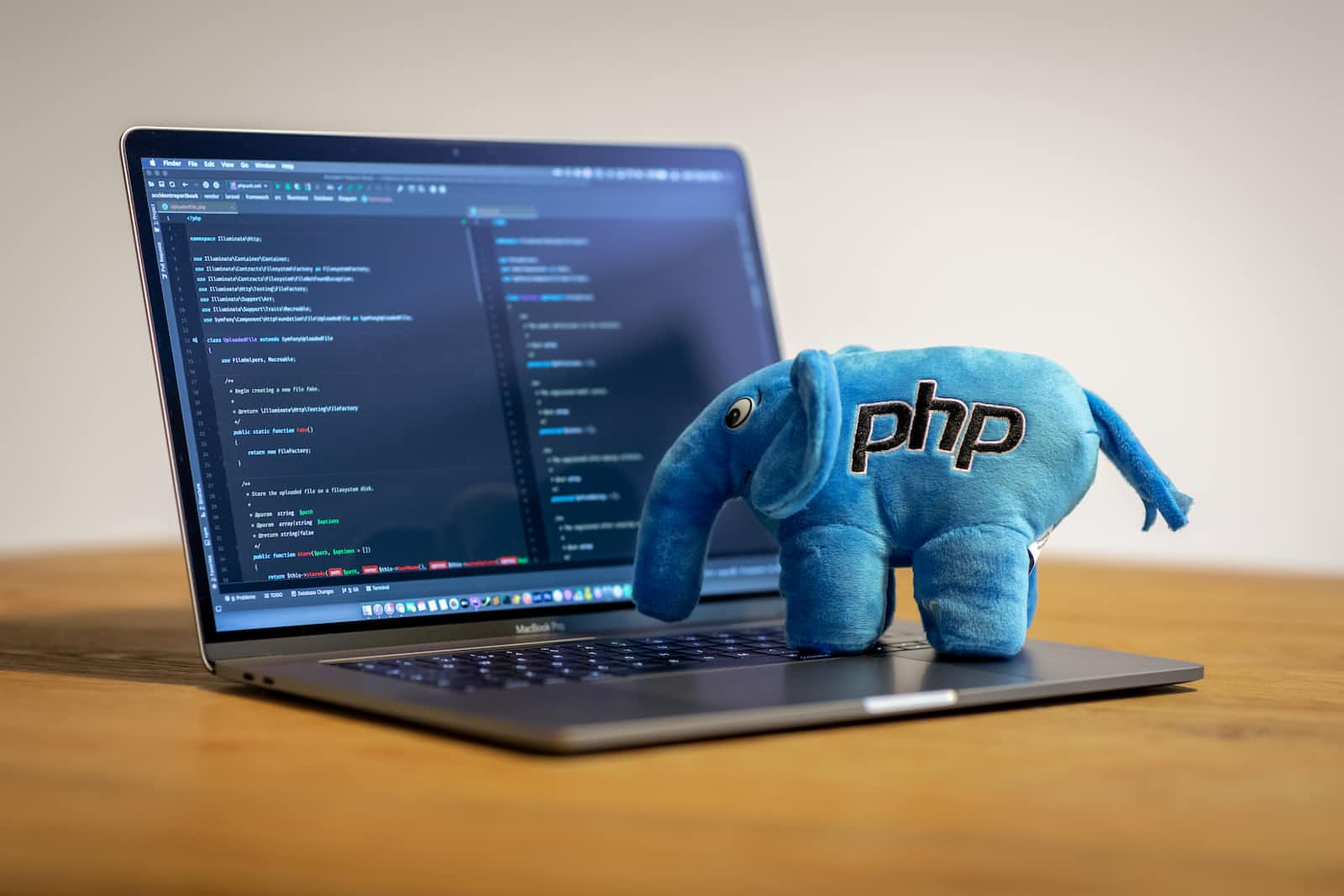
In this article, I will be showing you how to create a referral system with php and mysql
Creation of Database and table
Create database named hashnode. Next step is to create a table called users with the settings below
CREATE TABLE `users` (
`id` int(11) NOT NULL,
`username` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL,
`number_of_ref` int(11) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
Copy and paste the code above in the insert section of database hashnode, and the table will be created automatically.
Good job, we are already done with database and table.
Creation of register.php
After creating the register.php, open the file and create a form where users can enter their details and the referral id of the person that referred them.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Registration Page</title>
</head>
<body>
<h3>Enter Your details below</h3>
<?php
echo $_GET['message'];
?>
<form method="post" action="register_check.php">
<label>Username</label>
<input type="text" name="username"> <br>
<label>Password</label>
<input type="password" name="password"><br>
<input type="text" name="ref"><br>
<input type="submit" value="Submit">
</form>
</body>
</html>
The method is post while action point to register_check.php
We need to add text input for username, password and ref.
The ref is the field that will hold the referral id input from the user.
Let go to register_check.php
Creation of register_check.php
We start by importing or requiring database.php which is where our connection is, if you don't know how to setup connection in php kindly refer to my article here.
The next step is to receive the input values posted from register.php by using the code below.
<?php
require_once("database.php");
$username = $_POST['username'];
$password = $_POST['password'];
$ref = $_POST['ref'];
?>
Hope you are getting it. If you are not sure whether the values are posted or not, you can echo them.
Next is to check if the username already exists in our database.
$q = "SELECT * FROM users WHERE username='$username'";
$q = mysqli_query($conn,$q);
$total = mysqli_num_rows($q);
if($total < 1){
//this means that the username does not exist before in our database
$q="INSERT INTO `users` (`id`, `username`, `password`,`number_of_ref`) VALUES (NULL, '$username', '$password','$ref')";
mysqli_query($conn,$q);
header("location: index.php?message=You have registered successfully");
}else{
//this means that the username exists in our database
header("location: index.php?message=Username exist");
}
The above code loops through the table users and check if the username already exist, if yes then redirect them with message username already exist, if no then insert the user's details in our database.
Here is the full code of register_check.php
<?php
require_once("database.php");
$username = $_POST['username'];
$password = md5($_POST['password']);
$ref = $_POST['ref'];
//let check if the username does not exist in the database
$q = "SELECT * FROM users WHERE username='$username'";
$q = mysqli_query($conn,$q);
$total = mysqli_num_rows($q);
if($total < 1){
//this means that the username does not exist before in our database
$q="INSERT INTO `users` (`id`, `username`, `password`,`number_of_ref`) VALUES (NULL, '$username', '$password','$ref')";
mysqli_query($conn,$q);
header("location: index.php?message=You have registered successfully");
}else{
//this means that the username exists in our database
header("location: index.php?message=Username exist");
}
?>
Subscribe to my newsletter
Read articles from Michael Fawole directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
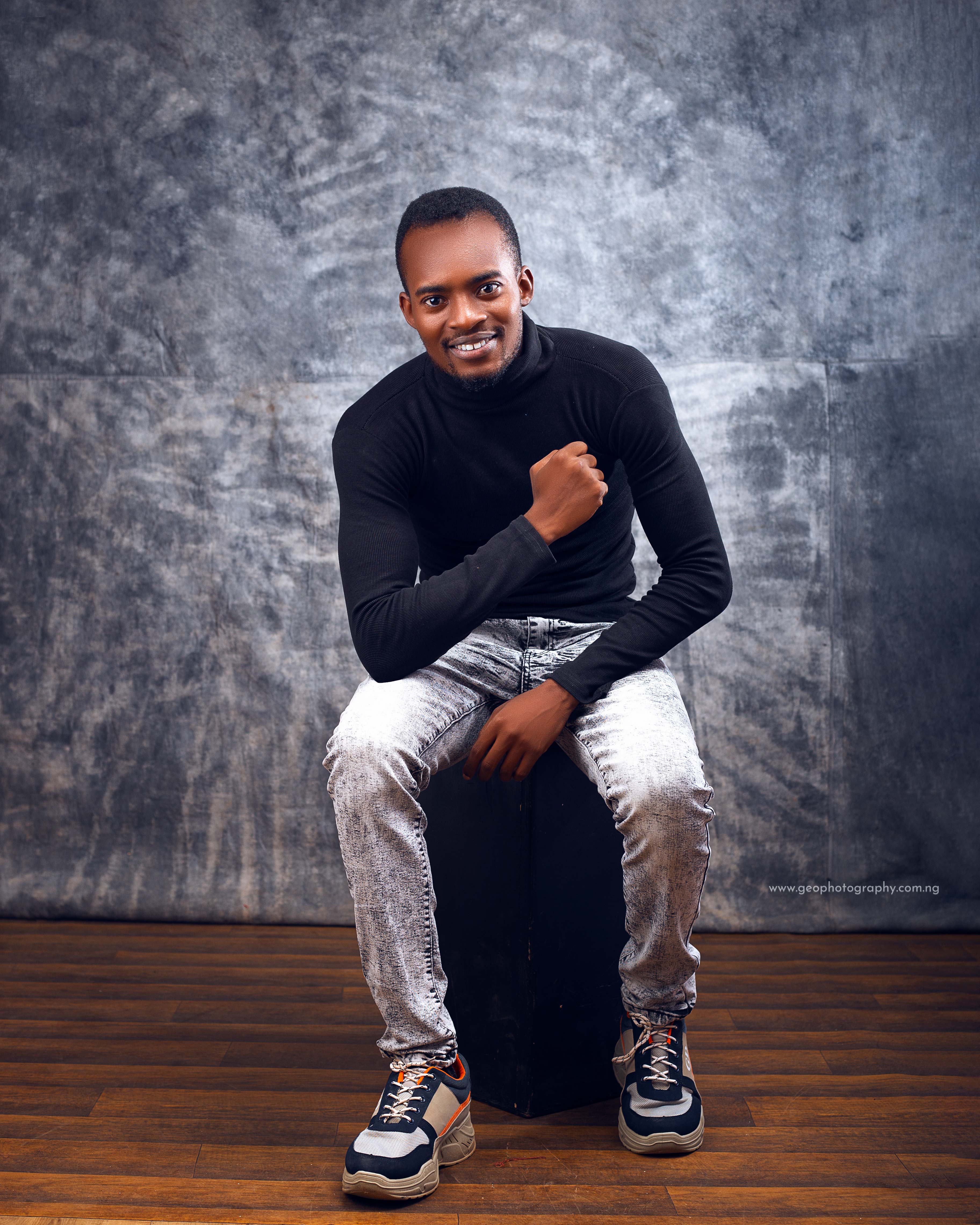
Michael Fawole
Michael Fawole
Michael Fawole is a Blockchain and web application developer with 7+ years of experience and 3+ years plus of experience in blockchain dapp development. He is also delivering training on blockchain ( Solidity, web3 and react js ) and php language. He also helps start-ups and companies to setup blockchain practice and incorporating blockchain into existing project. Techstack (Blockchain): Solidity, web3 js and react js. Techstack (Webapp): Php and Mysql.