Handling Form Has Never Been So Easy | Mantine Form
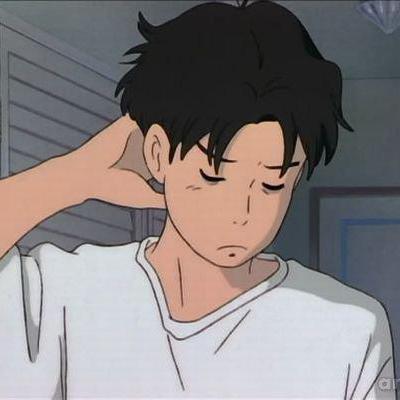
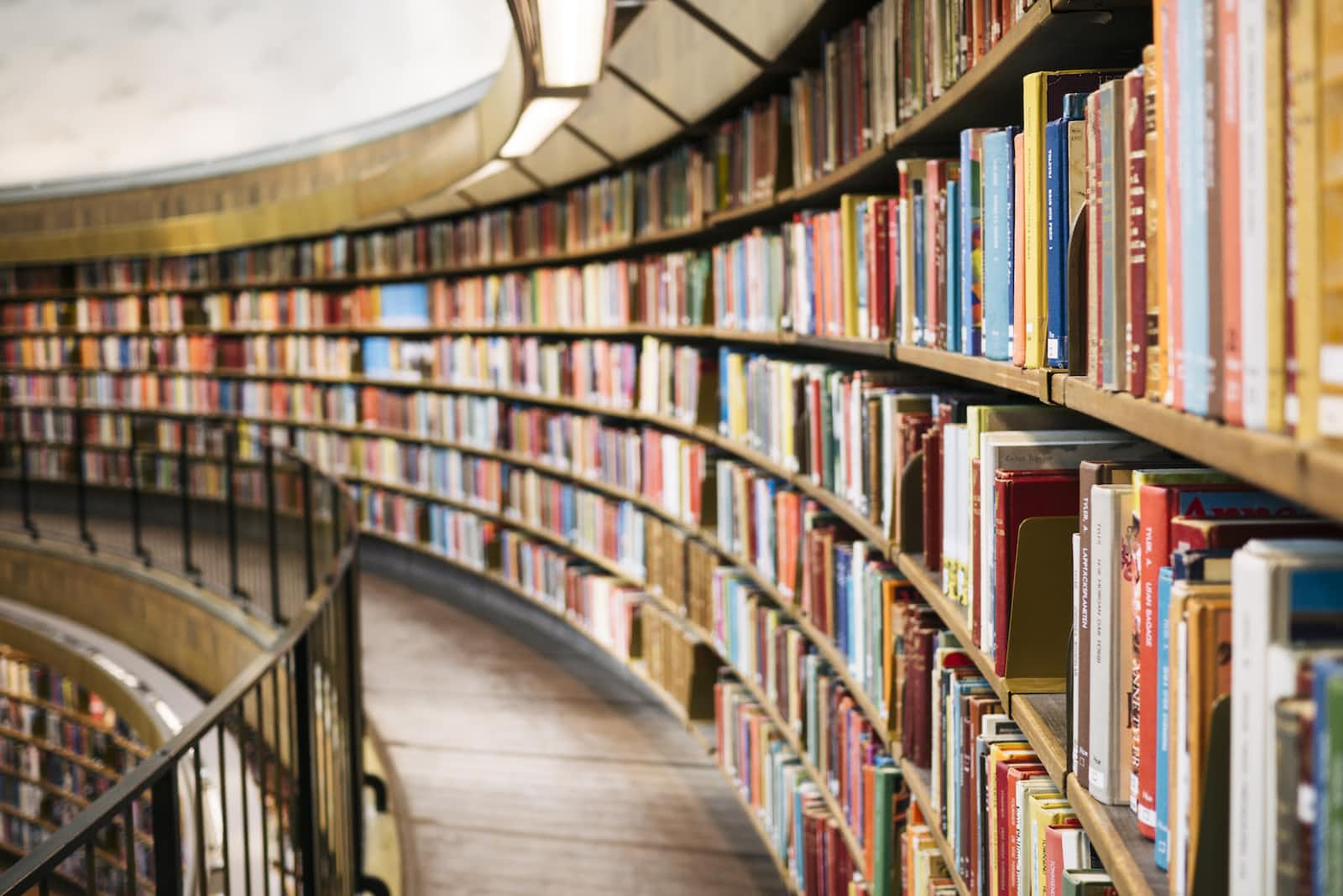
Handling Form with mantine use-form
A while ago when working on a project, my friend was searching for a good library to use on our new React based client-project. The project and he stumbled upon Mantine Library which was our savior right then and there.
Using use-form
To start with use-form from Mantine, you need to install use-form into your package.json first. @mantine/form does not depend on other libraries so you can use it as a standalone package.
npm install @mantine/form
yarn add @mantine/form
After that you can start using @mantine/form right away!
To get started, you need to import useform from @mantine/form into your JS file.
import { useForm } from '@mantine/form';
You can declare a variable and initialize it with useform like the example below:
const dog = useForm({
initialValues: {
name: '',
breed: '',
age: 0,
},
});
Accessing Value
<div>
<h2>{dog.name}</h2>
</div>
Setting form value You can set form value by using setFieldValue from useForm
<form>
<label htmlFor="name">Name</label>
<inputs
type="text"
value={dog.values.name}
onChange={(event) =>
dog.setFieldValue('name',event.currentTarget.value)}
/>
</form>
Reseting form values Useform has a reset api that you can call to reset the whole form value
<button type="reset" onClick={dog.reset}>Reset Form Value </button>
Validating the form Along with initialValues, you can also provide validate to easily validate your form with ease. To validate, you need to call a validate api from useForm like below:
const dog = useForm({
initialValues: {
name: '',
breed: '',
age: 0,
},
validate: {
name: (value) => (value !== null ? null : notify("Name can't be empty")),
breed: (value) =>
value !== null ? null : notify("Breed can't be empty"),
age: (value) => (value > 0 ? null : notify('Age must be greater than 0')),
},
});
Validating Function
const submit = () => {
//Before submitting, you can check if form is valid
if (dog.isValid) {
//Submit form
}
//Or the other way
const validated = dog.validate();
if (validated) {
validated.errors; //Object with errors
//Submit form
}
};
Conclusion Overall, from my personal opinion I really like Mantine's useForm just because it's really easy to use and beginner friendly. I seriously recommend checking out Mantine's document because there are a lot more stuff on their docs more than here. I hope this article spark your interest on checking Mantine Library out!
Subscribe to my newsletter
Read articles from Vatana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
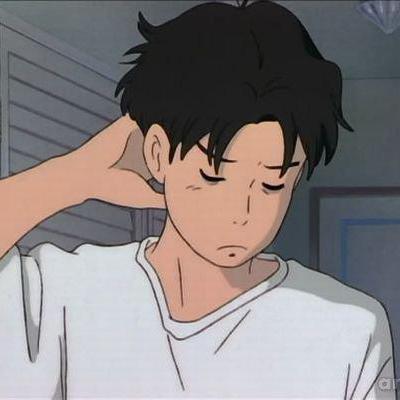
Vatana
Vatana
Software Engineer who's into fitness