Create a simple Desktop App in PySide6 with executable file
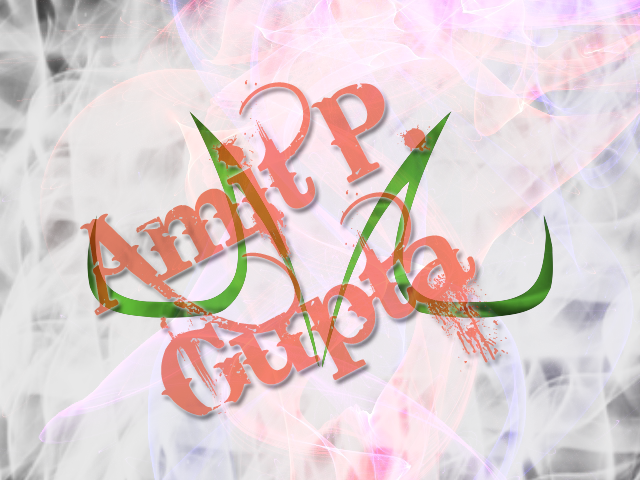
Pyside6 is a UI framework for Python used to create Desktop applications similar to Tkinter. It is based on QT Framework originally written for C++. In this concise post we would create an elementary PySide6 UI app and create an exe for it.
It is always a good practice to create a virtual environment which would house your Python packages before you begin your project. So let's start with creating a virtual environment first and activating it. I'd be developing this app in windows.
python -m venv venv
cd venv/Scripts
activate.bat
Next step would be to install packages, we would only install what is required for the very primitive version of the app to run. Let's install pyinstaller and Pyside6 packages.
pip install PySide6
pip install pyinstaller
Below is the complete snippet of the working main.py file. I'd explain what each of the lines do and the purpose of the packages we imported, just keep reading.
from PySide6.QtWidgets import QApplication, QWidget, QVBoxLayout, QLabel
# Only needed for access to command line arguments
import sys
app = QApplication(sys.argv)
# Create a Qt widget, which will be our window.
window = QWidget()
layout = QVBoxLayout()
labelWindow = QLabel("Another Window")
layout.addWidget(labelWindow)
window.setLayout(layout)
window.show()
# Start the event loop.
app.exec_()
QApplication class is used to create the main application. We started the app by calling the exec_() method on the object we just created. QWidget is like canvas on which we would have other widgets inside our app. We can apply horizontal or vertical layout to the widgets we add. Here, we are using a vertical layout by creating an object from QVBoxLayout.
This simple box would only contain a label, no fancy widgets, no buttons and events. If you now run the main file, you should see a very simple PySide6 app window popping up with a simple label. We won't be adding anything else for now. The purpose of this post is to get you started with PySide6 applications and quickly create executable files for your application. So, let's continue in that direction. We already installed pyinstaller earlier, let's make use of this package now. Type in your terminal the following command
pyinstaller --onefile --windowed main.py
I'd briefly explain what those '--onefile' and '--windowed' arguments mean while converting Python file into executable. One file command means that there should only be a single file, if we skip this, you would have several DLL files along with the executable file. Windowed means we do not wish command line to be opened while we open our app. Sometimes, this might be a desirable behaviour if we plan to pass command line arguments to the app, but in this case we would avoid that.
You should now see a folder named 'Dist' in the same app directory which would have your exe. It should run the program as executable file if everything went fine. I haven't explored how this build works on Linux operating system, but might add that case in future. The above script is tested in Windows and seems to work just fine. With that I'd be wrapping up for today. Thanks for the read!
Subscribe to my newsletter
Read articles from Amit PR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
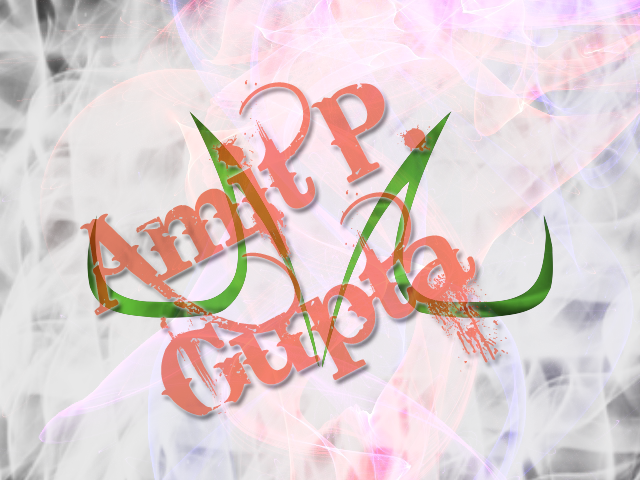