Functions in JavaScript- arguments
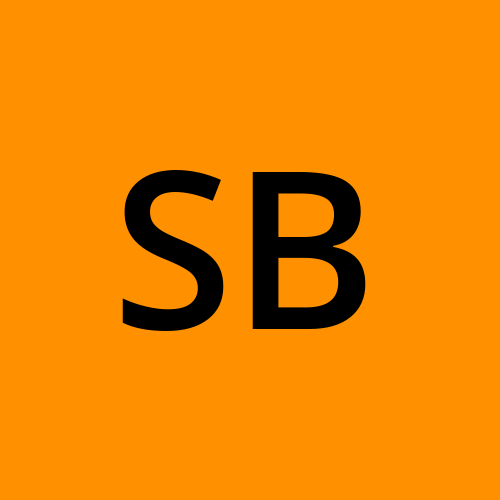

The previous article covered the basics of functions. Now, that doesn't even come close to covering everything about JS functions. Neither does this one for that matter, but I hope that the two taken together cover most of the use cases that you'll encounter out in the wild.
Formal parameters vs Actual Parameters
This is something that I find confusing even today. The terminologies make sense when I read them, however I invariably end up forgetting the difference between these two a couple of days later.
Formal parameters are the arguments that we define at the time of function definition. Actual parameters are the actual parameters that we pass to the functions-
// a and b are the formal parameters
function add(a, b){
return a+b;
}
// 2 and 3 are the actual parameters
console.log( add(2, 3) );
The arguments object
The arguments
object is an array-like object that contains the values of the arguments passed to that function. The arguments
object is 'Array-like'. It is not an array- hence we should be mindful while using array methods such as forEach()
with the arguments
object.
We can access the arguments passed to the function in question using array indexing.
function add(a, b){
console.log(arguments[0]); //logs 10
console.log(arguments[1]); //logs 20
return a+b;
}
let sum = add(10, 20); //sum holds 30
The arguments
object allows us to have variadic functions (functions that allow us to have a variable number of arguments). This is similar to how console.log()
accepts a variable number of arguments.
function add(){
let sum = 0;
for (let i=0; i<arguments.length; i++){
sum += arguments[i];
}
return sum;
}
let sum = add(10, 20, 30, 40); //sum holds 100
console.log(sum);
Rest parameters
ES6 introduced a new way to define variadic functions in JS with Rest parameters. Rest parameters allow us to accept a variable number of arguments as an array. Hence, we can use array methods such as forEach()
and map()
with rest parameters.
function add (a, b, ...remain){
let sum = 0;
sum += a + b;
for (i of remain){
sum += i;
}
return sum;
}
console.log( add(10, 20, 30, 40) ); //logs 100
Remember that the rest parameters can only be defined at the end of the argument list. They cannot be defined at the beginning-
// This is invalid. The rest parameter must be defined at the end.
function add(...remain, a, b){
let sum = 0;
sum += a+b;
for (i of remain){
sum += i;
}
return sum;
}
Default Parameters
Default parameters allow us to specify default values for arguments. These default values are used when no actual parameter is specified for a given formal parameter.
// b is the default parameter (Default value: 0)
function add (a, b=0){
return a+b;
}
console.log( add(5) ) //logs 5
function add (a, b){
return a+b;
}
console.log( add(5) ); //logs NaN as we have not specified a second parameter
//b is treated as undefined
Call by value
Before getting into what 'call by value' is, we need to know what JS primitives are. Primitives are data that are not objects and have no methods or properties. JS has 7 primitives- String
, Number
, BigInt
, Boolean
, undefined
, Symbol
and null
.
Call by value simply means that when a variable is passed to a function as a parameter, and changes are made to that parameter, these changes are not reflected in the original variable. All primitive data types are 'passed by value'-
function transform(a){
a = 10;
}
let a = 1;
transform(a);
console.log(a) //logs 1 (not 10)
Call by reference
Call by reference means that the address of the variable is passed to the function and hence any changes made to that variable within the function will be reflected in the original variable. All objects in JavaScript are 'passed by reference'-
//The array is transformed as JS arrays are objects
function transform(arr){
arr.push(10);
}
arr = [1, 2, 3];
transform(arr);
console.log(arr); //logs [1, 2, 3, 10] (not [1, 2, 3])
Hope this article provided a little clarity on JS function parameters. Stay tuned for more articles on JavaScript and Web Development.
Subscribe to my newsletter
Read articles from Senthooran B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
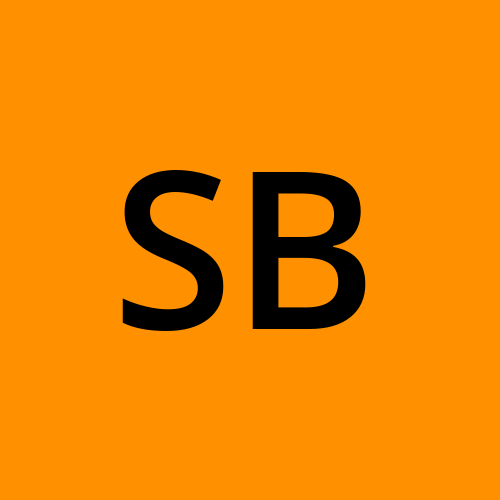