Working with Loops in JavaScript
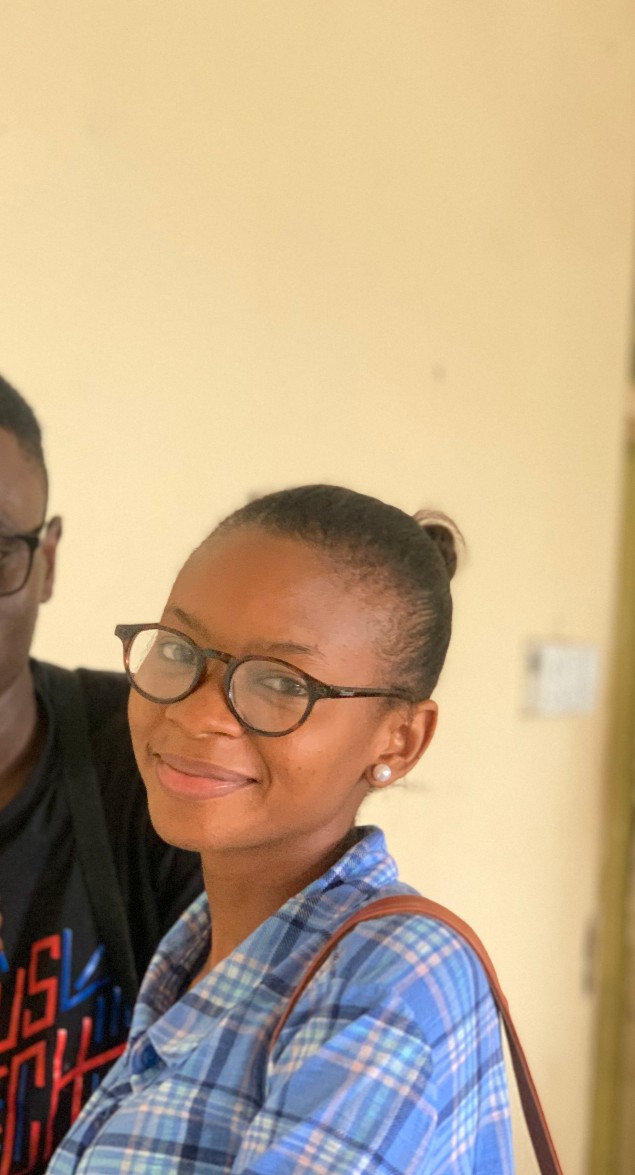
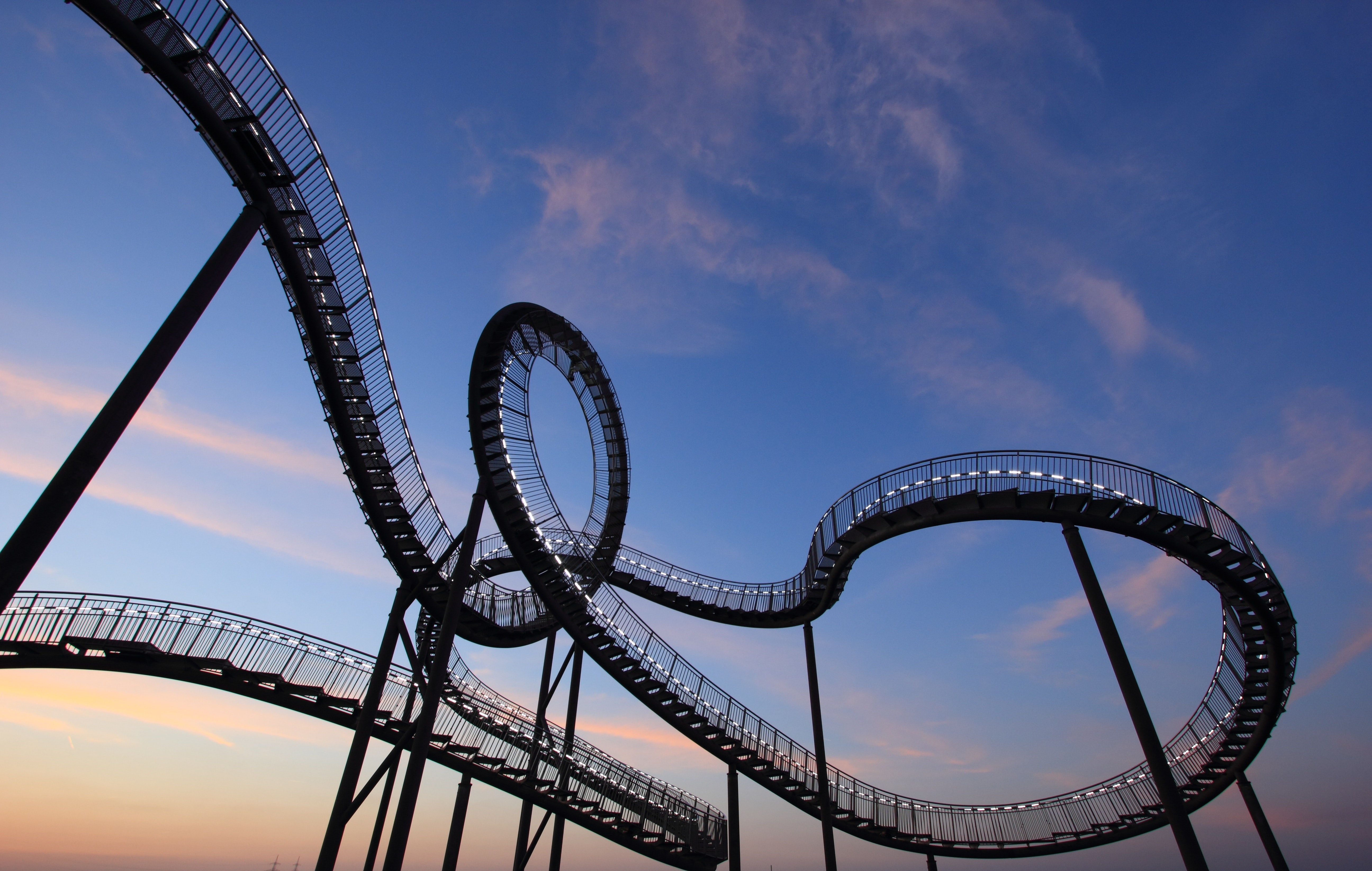
Hi guys! I hope you're well.
Welcome to another article where I will be talking about **Loops **in JavaScript. Before we dive right in, I would like to show you how code would have been written in the absence of Loops.
Let's say you want to print out a name x number of times. You would do this:
console.log("Somy");
console.log("Somy");
console.log("Somy");
console.log("Somy");
console.log("Somy");
Stressful right? Now let's dive right into Loops and their variations.
Definition of Loops
Loops repeat an action a given number of times. They come in handy in our previous example above. Let's review it this time using Loops:
for( let i = 0; i < 5; i++){
console.log("My name is ${Somy}"); //returns My name is Somy five times
}
It looks mind-boggling, right? Let's break it down into tiny bits.
The Different Variations of Loops
- for Loop:
In our example above, we used the for
keyword, the
Within the ```
()
``` parenthesis we have the three expressions guiding our loops like so:
for( [initial expression; condition; increment expression] )
When a ```
for
``` loop runs, the following occurs behind the scenes:
(**i**) Initial expression: According to MDN, the [initial expression](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Loops_and_iteration) usually initializes one or more loop counters. It is executed before the code block. It can also declare variables.
(**ii**) Condition: The code will continue to run as long as the given condition evaluates to true. It is a Boolean expression. In the example above, we had `i < 5` ( `i` must be less than 5) as our condition.
(**iii**) Increment expression: This is where the value of `i` is increased each time the loop executes. It could also be a decrement expression.
> Note: The letter `i` in the loop is optional and it stands for index. It is used as a temporary variable.
2 . **while Loop**: The while loop is similar to the `for` loop in which the loop continues to execute as the condition remains true.
Let's take an example:
let i = 1; while (i <= 5){ console.log("The number is ", i); i++; } //returns The number is 1 //returns The number is 2 //returns The number is 3 //returns The number is 4 //returns The number is 5
The loop above will continue to run as long as the variable `i` is less than or equal to 5. The variable `i` will increase each time the loop runs.
3 . ** for...of Loop**: The `for...of` loop is used to iterate over arrays and other iterable objects. Below is a syntax:
for (variable of iterable){ statement }
`variable`: For every iteration, the value of the next property is assigned to the variable.
`iteration`: An object that has iterable properties.
Let's take an example:
let classNames = ["Dave", "Tobi", "Peter", "Somy"];
for(let names of classNames){ console.log("The student name is: ",names); } //returns The student's name is Dave //returns The student's name is Tobi //returns The student's name is Peter //returns The student's name is Somy
4 . **Infinite Loops**: The infinite loop never stops executing just as the name implies. This situation could cause your browser or program to crash. Below is an example of an infinite loop:
for (let i = 10; i >= 0; i++){ console.log(i); }
For more resources on Loops in JavaScript, visit [MDN](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Loops_and_iteration#for...of_statement), [w3schools](https://www.w3schools.com/js/js_loop_for.asp) or [Freecodecamp](https://www.youtube.com/watch?v=24Wpg6njlYI).
Subscribe to my newsletter
Read articles from Somtochukwu Nwosu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
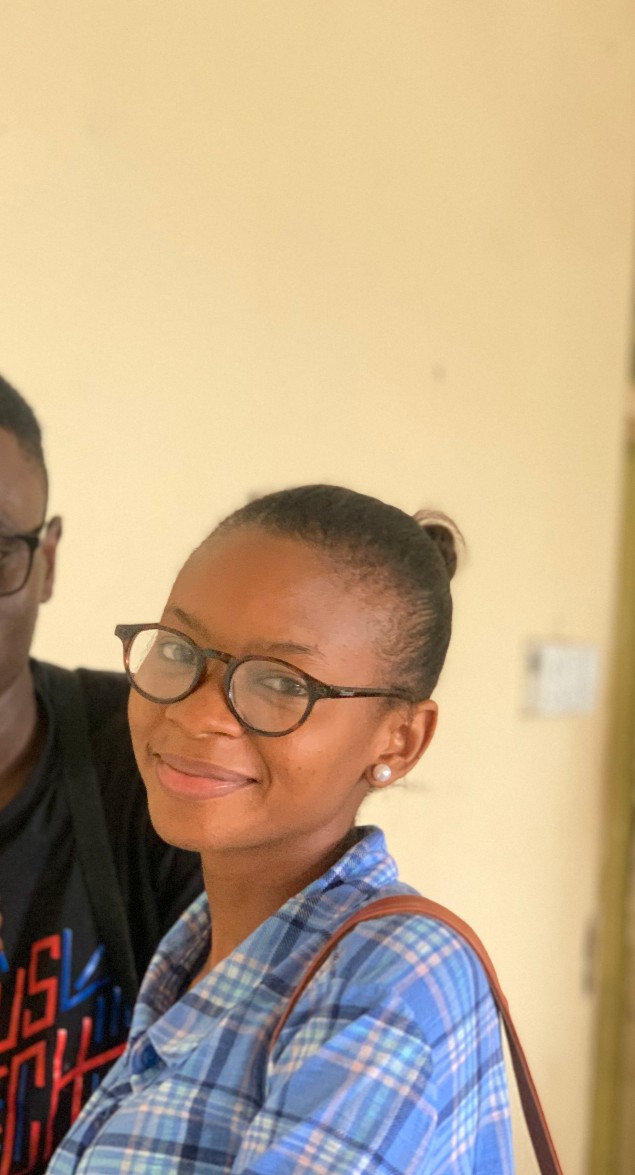
Somtochukwu Nwosu
Somtochukwu Nwosu
I'm passionate about learning and communicating that knowledge through my writing.